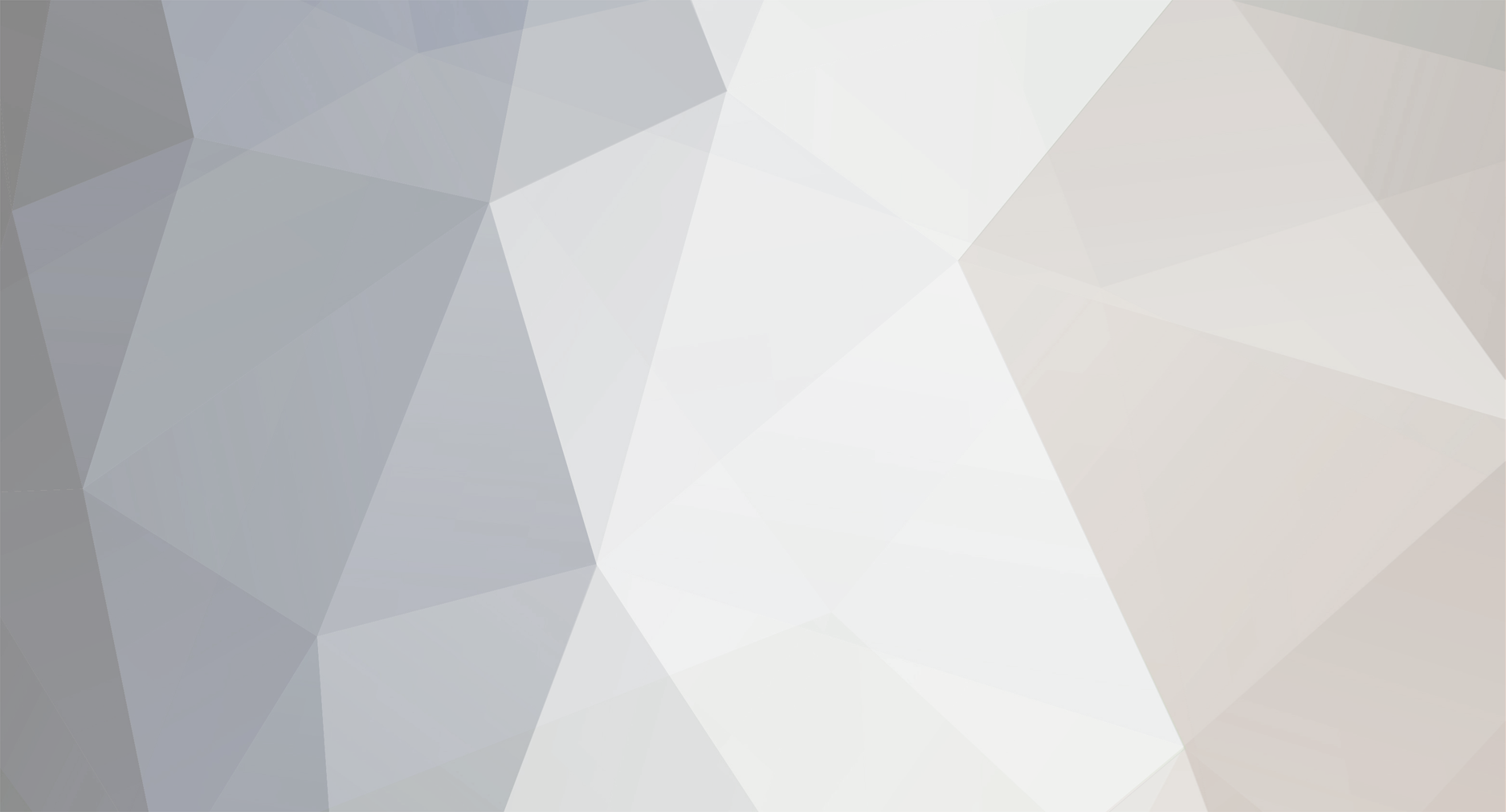
mallen
Members-
Posts
316 -
Joined
-
Last visited
Everything posted by mallen
-
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
The PDF function is a change to the way I displayed the images. When I get this working I will change that function too. error_reporting(E_ALL); ini_set("display_errors", 1); function get_folders () { //list category folders as list of links if ($handle = opendir('products')) { $blacklist = array('.','..','products', 'index.php'); while (false !== ($file = readdir($handle))) { if (!in_array($file, $blacklist)) { echo '<a href=/products_list.php?folder=' . $file .'>'. strtoupper($file) . '</a>'. '<br/>'; } } closedir($handle); } } function display_files_jpg () { //display list of jpg images foreach(glob('products/*/product2/*.jpg') as $image) { echo basename($image) . "<br />"; } } function display_files_pdf () { //display list of pdf file $dirs = 'my_desired_folder'; $dirs = $_GET['folder']; foreach(glob($dirs, GLOB_ONLYDIR) as $pdf) { echo basename($pdf) . "<br />"; } } echo display_files_pdf(); echo display_files_jpg(); echo get_folders(); -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
Yes this function echos a URL that includes the variable. Do I have to declare the $dirs ='some_value'; at the top of the page? function get_folders () { //list category folders as list of links if ($handle = opendir('products')) { $blacklist = array('.','..','products', 'index.php'); while (false !== ($file = readdir($handle))) { if (!in_array($file, $blacklist)) { echo '<a href=/DuraGuard/products_list.php?folder=' . $file .'>'. strtoupper($file) . '</a>'. '<br/>'; } } closedir($handle); } } -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
Thanks. I had something like that. I tried what you suggested and got "Notice: Undefined index: folder in...." -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
Anyone? I have searched over and over for a solution. All I can find is examples of static directories. Mine has to be based on a variable. Maybe something on the second page that is where "folder = $_GET["folder"] Thanks. -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
When this part runs the value that gets passed in the URL and displays on the page is only "category2" or "category1". It won't display the images and files for each. $dirs = $_GET['folder']; foreach(glob('dirs', GLOB_ONLYDIR) as $pdf) -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
Get_folders() and display_files_jpg() are working. -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
I used var_dump() to check that 'folder' has a value. But I need to figure how to make the folder directory a daynamic value. It doesn't return any images or files. Anyone have ideas how I can accomplish this? -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
I tried changing just the second funtion as a test using GET thinking since the 'folder' will be passed in the URL but didn't work. I think becuase product is a subfolder of category. function get_folders () { //list category folders as list of links if ($handle = opendir('products')) { $blacklist = array('.','..','products', 'index.php'); while (false !== ($file = readdir($handle))) { if (!in_array($file, $blacklist)) { echo '<a href=/products_list.php?folder=' . $file .'>'. strtoupper($file) . '</a>'. '<br/>'; } } closedir($handle); } } function display_files_jpg () { //display list of jpg images foreach(glob('products/*/product1/*.jpg') as $image) { echo basename($image) . "<br />"; } } function display_files_pdf () { //display list of pdf files $dirs = $_GET["folder"]; foreach(glob('dirs', GLOB_ONLYDIR) as $pdf) { echo basename($pdf). "<br />"; } } echo display_files_pdf(); echo display_files_jpg(); echo get_folders(); -
Display List Of Folders And Link To Individual Pages
mallen replied to mallen's topic in PHP Coding Help
Thanks. I looked at glob() and came up with this. But I had to hard code each product folder. I need it to be dynamic. I plan to have it display a thumbnail, list of files to download and list of pdf files for each product. function get_folders () { //list category folders as list of links if ($handle = opendir('products')) { $blacklist = array('.','..','products', 'index.php'); while (false !== ($file = readdir($handle))) { if (!in_array($file, $blacklist)) { echo '<a href=/products_list.php?folder=' . $file .'>'. strtoupper($file) . '</a>'. '<br/>'; } } closedir($handle); } } function display_files_jpg () { //display list of jpg images foreach(glob('products/*/product1/*.jpg') as $image) { echo basename($image) . "<br />"; } } function display_files_pdf () { //display list of pdf files //pseudo code here //$file = $newCategory; foreach(glob('products/*/product1/*.pdf') as $pdf) { echo basename($pdf). "<br />"; } } echo display_files_pdf(); echo display_files_jpg(); echo get_folders(); -
I am trying to develop a page that will display a list of categories of products. And then when the user clicks on the category it passes to another page that will display a list of products for that category. I have folders for each category and inside the folder is a folder for each product. This way I can just dump files into the folders to make updates. So far I have it displaying the list of category folders I will use as my navigation. I need help getting the second page done. I need it to display the list of products and then a link to an individual page. I have written out the pseudo code what I am trying to do. function get_folders () { //list category folders as list of links if ($handle = opendir('products')) { $blacklist = array('.','..','products', 'index.php'); while (false !== ($file = readdir($handle))) { if (!in_array($file, $blacklist)) { echo '<a href=/products_list.php?folder=' . $file .'>'. strtoupper($file) . '</a>'. '<br/>'; } } closedir($handle); } } function display_category () { //display list of products based on category //pseudo code here $file = $newCategory; for each folder name that equals $newCategory { echo = '<a href=/single_product.php?folder=' . $newCategory .'>'. strtoupper($newCategory) . '</a>' ; } } echo get_folders();
-
I was able to get it fixed. I am sure it had to do with a cookie being set. I learned using _REQUEST includes $_COOKIE. I use some similar pages on other sites I have installed locally and there may be a conflict. I did a search with Dreamweaver and found no other function that could be sending me to another URL. I changed _REQUEST to _POST and to _GET and got a permissions error message. Then I cleared my history and cookies and set it back to _REQUEST. I noticed it was sending me to a URL with and extra '=' and found that in the function inside the javascript. Also I renamed the link on the onchange to make sure it was a unique function and it worked. function getBreadcrumbs() { $output =""; $totalPages = $this->totalLit / $this->numPerPage; if($totalPages > 2) { $output .= "<label>Page:</label>"; $output .= "<select id='breadcrumb_page' onchange='getNewBreadcrumbPageLiterature(this.value)';>"; $output .= "\");'>"; for($i=1; $i<=ceil($totalPages); $i++) { $output .= "<option value='$i'"; if(isset($_REQUEST['breadcrumb']) && $_REQUEST['breadcrumb'] == $i -1) $output.= " selected"; $output .= ">$i</option>\n"; } $output .= "</select>"; echo $output; } } and the Javascript file that is linked: function getNewBreadcrumbPageLiterature(p) { var newpage = p - 1; var location = document.location.href; var existing = gup('breadcrumb'); if(!existing) window.location = location + '&breadcrumb=' + newpage; else { window.location ="?page=edit-literature" +"&breadcrumb="+ newpage; } }
-
I solved it using this: $title = the_title('', '', false); I guess since it was a WordPress function I should of looked there first.
-
Correct that is my issue. Its null and therefore not switching according to my logic. the_title() function holds a value but $title does not.
-
Yes I var_dump($title) and it has a value of NULL and original title for each page shows unchanged.
-
hanks for the info. I will leave the above as it is. I now realize the similarity of if/else and a switch. How about this example for a another part of my site. Same type of issue. I am printing a page title and I need to change the name dynamically. All I get is the page name its not changing based on my logic. $title = the_title(); if ($title == 'Contact Info'): { echo ("Contact Us"); } else: switch($title){ case 'Products Pages': echo ("Our Products"); break; case 'Find An Agent': echo ("Locate an Agent"); break; } endif;
-
I got it to work but Ihave to hard code each page. I was trying to get something more dynamic to switch the name. function corepages() { $pages = get_pages(); global $blog_id; foreach ($pages as $p) { if ( file_exists( compat_get_plugin_dir( 'dir' ) . '/icons/' . $p['icon'] ) ) { $image = compat_get_plugin_url( 'dir' ) . '/icons/' . $p['icon']; } else { $image = compat_get_upload_url() . '/images/' . $p['icon']; } if ($p['post_title'] == "Products Pages") : { echo ('<li><a href="' . get_permalink($p['ID']) . '"><img src="' . $image . '" alt="icon" />' . 'Our Products' . '</a></li>'); } else : switch($p['post_title']){ case 'Contact Our Company': echo ('<li><a href="' . get_permalink($p['ID']) . '"><img src="' . $image . '" alt="icon" />' . 'Contact Us' . '</a></li>'); break; } case 'Our Locations': echo ('<li><a href="' . get_permalink($p['ID']) . '"><img src="' . $image . '" alt="icon" />' . 'Locations' . '</a></li>'); break; endif; } }
-
I am trying to do switch where it changes the name of the post title. I just can't seem to get the format correct. I tired the variable $P also and it doesn't work. function corepages() { $pages = get_pages(); global $blog_id; foreach ($pages as $p) { if ( file_exists( compat_get_plugin_dir( 'dir' ) . '/icons/' . $p['icon'] ) ) { $image = compat_get_plugin_url( 'dir' ) . '/icons/' . $p['icon']; } else { $image = compat_get_upload_url() . '/images/' . $p['icon']; } if ($p['post_title'] !== "") : { echo('<li><a href="' . get_permalink($p['ID']) . '"><img src="' . $image . '" alt="icon" />' . __($p['post_title']) . '</a></li>'); } else : switch($p['post_title']){ case 'Products Pages': $p = "Our Products"; break; case 'Contact Our Company': $p = "Contact Us"; break; } endif; } }
-
Thanks Jazzman1. Not sure what I should use instead of _REQUEST. I tired your code and yes it works by itself. But the URL is not changing. Also my total pages will need to be dynamic. Its displaying data to the javascript pop up window. But in the complete set up it still fails after you select a second page. In any order. My url reads ?page=edit-literature&breadcrumb=1 Then I choose and page My url reads ?page=edit-literature&breadcrumb=2 And the second page in any order whether its page 1 again or page 3 it goes : ?page=different_page_alltogether&breadcrumb=0 And of course the admin page and pagination is all gone because its on another page.
-
<div id="adminBreadCrumbs"> <div class='alignRight'> <label>Page:</label><select id='breadcrumb_page' onchange='getNewBreadcrumbPage(this.value)';><option value='1'>1</option> <option value='2'selected>2</option> <option value='3'>3</option> </select>2.8888888888889 </div>
-
I removed if(isset($_REQUEST['breadcrumb']) && $_REQUEST['breadcrumb'] == $i -1) And that didn't work. Got the same results. It needs a dynamic variable for the bread crumb that gets fed to the switch statement.
-
I think this line may be the issue output .= "<select id='breadcrumb_page' onchange='getNewBreadcrumbPage(this.value,\""; I can't tell what is getting stuck. For example it give choice of 1,2, 3. You can pick any one the first time. If you pick any other page after this first selection it loads another page. Seems it loses track of what page and breadcrumb its on. The page is page=edit-literature&breadcrumb=2 and it will leave this.
-
I set the total per page to 9. The drop down shows 3 pages. I echo $totalPages; and get 2.8888888888889 I have 26 files
-
2.8888888888889
-
I have this list of files that are dynamically listed on a admin page. It lists all the files and I can control the amount per page. Also I can add and delete. So I know all those functions work fine. Here is the problem. I am trying to use a pagination system. It has a drop down box with page numbers. But it will only alow me to select the page once. It lists for example all 4 pages. It doesn't matter if I select page two or four or what order. If I select another page it launches a different page and exits the pagination ect.. breadcrumb=1 Is there anything in my code that could cause this? class literature { protected $numPerPage, $getLits, $totalLit; function __construct() { $this->numPerPage = 9; $this->getLit = $this->getLits(); $this->totalLit = count($this->getLits(true)); } function getLits($count = false) { $curPage = 0; $ltCategory = NULL; if(isset($_REQUEST['breadcrumb'])) $curPage = $this->numPerPage * $_REQUEST['breadcrumb']; if($count) $curPage = -1; return $this->getLitQuery($curPage); } function getLitQuery($curPage = 0) { global $wpdb; $litQuery = "SELECT * FROM literature ORDER BY lit_id ASC"; if($curPage !== -1) $litQuery .= " LIMIT $curPage,". $this->numPerPage; return $wpdb->get_results($wpdb->prepare($litQuery), ARRAY_A); } function getLitInfo($id) { global $wpdb; $query = $vals = $wpdb->get_row($wpdb->prepare("SELECT * FROM literature WHERE `lit_id`='$id' LIMIT 1"), ARRAY_A); return $vals; } function getBreadcrumbs() { $output =""; $totalPages = $this->totalLit / $this->numPerPage; if($totalPages > 2) { $output .= "<label>Page:</label>"; $output .= "<select id='breadcrumb_page' onchange='getNewBreadcrumbPage(this.value,\""; $output .= "\");'>"; for($i=1; $i<=ceil($totalPages); $i++) { $output .= "<option value='$i'"; if(isset($_REQUEST['breadcrumb']) && $_REQUEST['breadcrumb'] == $i -1) $output.= "selected"; $output .= ">$i</option>\n"; } $output .= "</select>"; echo $output; } } } And here are the javascript funtions: function getNewBreadcrumbPage(p, c) { var newpage = p - 1; var location = document.location.href; var existing = gup('breadcrumb'); if(!existing) window.location = location + '&breadcrumb=' + newpage; else { window.location ="?page=edit_literature&productCategory=" + c +"&breadcrumb="+ newpage; } } function gup( name ) { name = name.replace(/[\[]/,"\\\[").replace(/[\]]/,"\\\]"); var regexS = "[\\?&]"+name+"=([^]*)"; var regex = new RegExp( regexS ); var results = regex.exec( window.location.href ); if( results == null ) return false; else return true; }
-
If I change to : ORDER BY agent.country, cty.name, states.state_name ASC I get Mexico, then United States and so on. The agent_countries table is US id is 1, Canada is 2, Mexico is 3 and Puerto Rico is 4. I changed it to ORDER BY cty.id ASC and its in the order I needed.