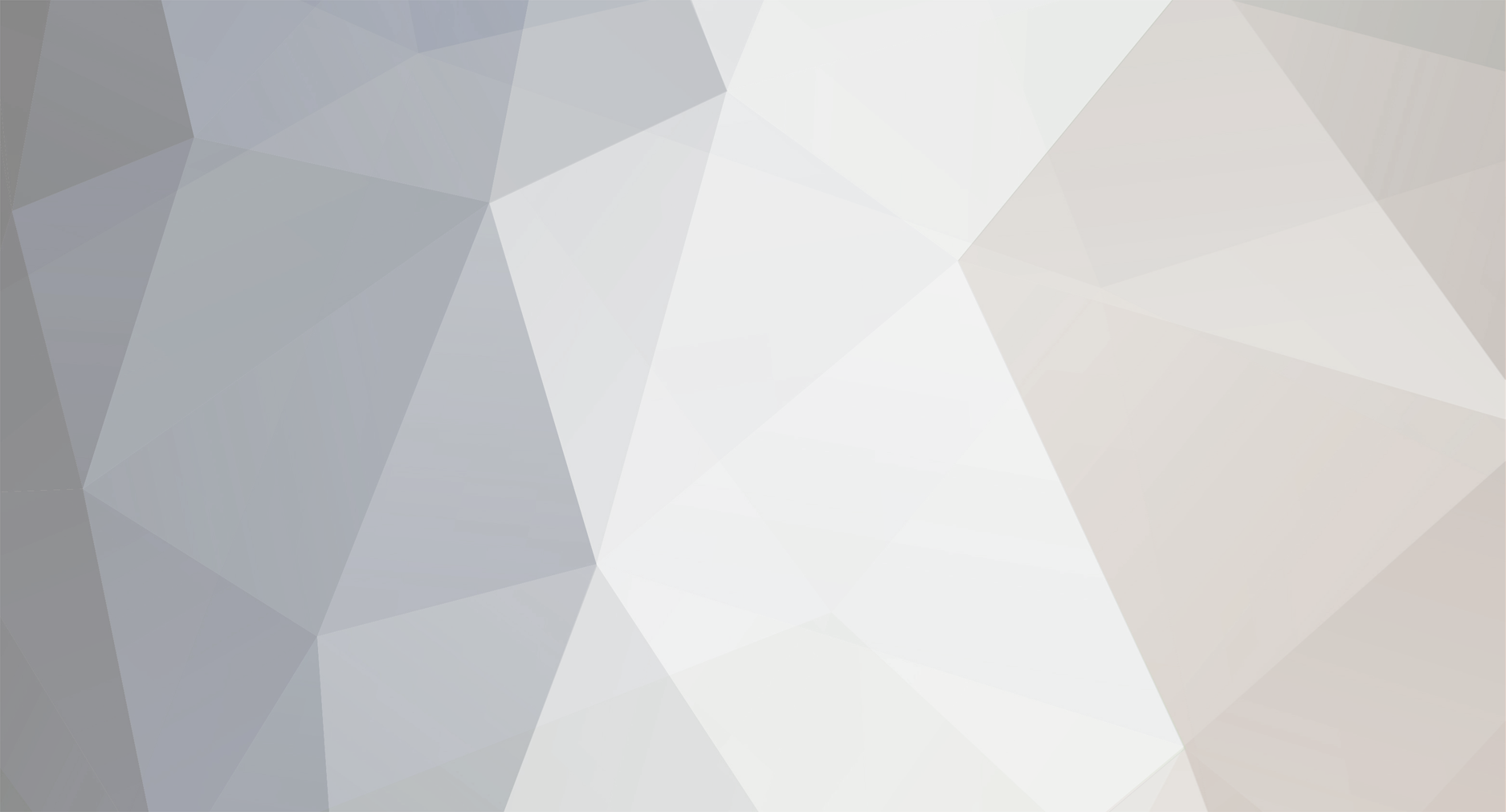
bschultz
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by bschultz
-
No two schools put things in the same order or with the same div or column names. The method I am using lets the end user select the order of the roster they are loading at that time based on which column is which. IE: column 1 is number, column 2 is name, column 3 is a picture of the payer (which we don't use and ignore), column 4 is their height. Next week, it might be column 1 name, column 2 number...etc. One week the school may only provide a Word document in table format... Like I said, there are no set formats of what is being copied and pasted in. As long as the info being gathered is in table form (which is the case 95+ percent of the time), my system can adapt. If you can show me how DOM or html_simple_dom would work better...I'd love to hear it.
-
copy and paste the roster from the schools website into a wysiwyg text box on my site. That entry runs through HTML Purifier to clean up formatting other than the table tr and td tags...then put into an array. The array is then split by < td > to find each inidividual roster entry (number, name, height, weight etc.). I was using strip_tags to get rid of everything other than table tr and td...but a new school had embedded p tags inside the td tag...which killed the import. HTML Purifier is cleaning up the entered code...but I don't know where the extra whitespace is coming from. Still haven't had a chance to play with typecast, but will soon.
-
I tried trim, ltrim and rtirm last night...none seemed to work. I'll try typecast today...thanks!
-
Ever heard of HTML Purifier adding whitespace at the end of tags? I'm trying to import sports jersey numbers into a DB...as int. Whitespace is killing the import.
-
That explains it...I put the allowed tags in brackets...thanks!
-
HTML Purifier didn't remove the inner tags either...
-
I need to strip some html tags out of an uploaded string of code. I need to keep the <td> tags...but some code that is being uploaded include <p> tags INSIDE the <td> tag. How would I go about stripping ALL other tags inside these allowed tags: <td> <tr><table>
-
Why is it you always find the right Google phrase AFTER you post the questions? ${'pos_' . $i}
-
I'm have a hard time figuring out variable variables. if (${$pos_$i} == '1234') So, the $i is in a loop... The full variables are $pos_1, $pos_2, $pos_3 etc. How can get this to work? The code above throws a Parse error: syntax error, unexpected T_VARIABLE in... error. Thanks!
-
That makes sense...thanks!
-
The following code ran as true on Friday at 11:45pm...and $showname did not equal anything...anyone know why? if ((($dow != 'Friday' || $dow != 'Saturday') && $hour == '23' && $minute == '45') || $showname == 'livingthecountrylife') This should only run on Sunday, MOnday, Tuesday, Wednesday and Thursday night at 11:45pm...or when run from command line passing the variable Showname the value of livingthecountrylife. The date and time variables are set like this: $year = date('Y'); // 4 digit $month = date('m'); // with leading zeros $date = date('d'); // with leading zeros $dow = date('l'); // Sunday Monday Tuesday...etc $hour = date('H'); // 24 hour with leading zeros $minute = date('i'); // with leading zero $now = date('Y-m-d'); Thanks!
-
I changed plans and went with GET variables to set the start date and the end date of the time frame wanted....more flexible anyway. Thanks!
-
I tried... $sql = 'SELECT * FROM table WHERE WEEK(birthdate) = WEEK(DATE_ADD(NOW(), INTERVAL 7 DAY)) AND WEEK(birthdate) = WEEK(DATE_ADD(NOW(), INTERVAL -7 DAY))'; And I got back results from March...
-
I have a column (DATE) for bithdays...how can I select in MYSQL which birthdays were last week...and the upcoming week? I found this...but it's only for the upcoming week...not last week too... $sql = 'SELECT * FROM table WHERE WEEK(birthdate) = WEEK(DATE_ADD(NOW(), INTERVAL 7 DAY))'; How can I adjust this to include last week too? Thanks.
-
Thanks... by the way, here's the current code snipit...with column names... mysql_select_db($db,$dbc); $sql = "SELECT * FROM downloads"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $row = $row['row']; $name = $row['name']; $min = $row['min']; $hour = $row['hour']; $dom = $row['dom']; $mon = $row['mon']; $dow = $row['dow']; $type = $row['type']; $filetype = $row['filetype']; $url = $row['url']; $username = $row['username']; $password = $row['password']; $local_path = $row['local_path']; $remote_path = $row['remote_path']; $a1037 = $row['a1037']; $a983 = $row['a983']; $a921 = $row['a921']; $a1360 = $row['a1360']; $a1300 = $row['a1300']; // rest of code here }
-
Monkeypaw...that's correct, 1 cron job running 1 script. I've found a problem in both of our logic, though. if(date("i") == $minute || $minute == '*') { $doirun = TRUE; } if(date("H") == $hour || $hour == '*') { $doirun = TRUE; } If the download should occur at 0:10 of each hour...hour will be * in the database...meaning that it would execute every minute (date("H") == $hour || $hour == '*') when the cron job runs...since hour = * How can I account for all of the different if statements that will be needed to act in a similar fashion as cron?
-
I'd currently work for a radio station. On a daily and weekly basis we need to download certain files (mp3) for playback later. I currently have about 50 cron jobs scheduled to download the files at a given time or day. I'd like to consolidate these files and jobs. I'd like to have one cron job set to run every minute to execute a php script that will do the download if the time is a database is correct. How can I write the logic of the if statements in the php script to follow similar logic as cron (0 10 * * *) like 10am every day? I have the datatype in mysql set as varchar so that I can include the * wildcard. Here's what I've come up with. Is there a "better" way to accomplish this? $run = 0; if ($min == $current_minute || $min == '*') { $run = 1; } if ($hour == $current_hour || $hour == '*') { $run = 1; } if ($dom == $current_dom || $dom == '*') { $run = 1; } if ($mon == $current_mon || $mon == '*') { $run = 1; } if ($dow == $current_dow || $dow == '*') { $run = 1; } if ($run = 0) { exit; ) elseif ($run == 1) { execute rest of script here }
-
The database is structured as name...not first name and last name. Since that's the way the rosters are formatted, that's the way the db was formatted. I did a little research on full text search and it looks promissing. I've only tested for the player I refferenced above, D. Kroeplin...and it worked. I'm guessing it's slow though. Is it? And is there any way to keep the db as is...and still use LIKE like you suggested?
-
Alright, so levenshtein didn't work at all... Kroeplin, D. (as taken from the stats page of the team---which is Dustin Kroeplin) came back as Dustin Dowd using levenshtein. Back to square one I guess!
-
This is for a football stats system. The user enters the teams roster (which goes into the database. Then they enter the player stats. All of this information is listed on the schools website. Problem is, so schools put stats online in first last order...some put last, first...some put last, first initial (2 initials if there's a John Smith and a Jim Smith (Jo. and Ji.). I just need to match the full name that's in the database. If there's a mulitple possible match, I suppose I could add a check for the user to choose which player it is. It's VERY rare that a team has more than one player with a same last name. I won't have time to work on this project til the weekend...I'll probably be back then with further questions.
-
Metaphone didn't work for the examples I listed...but Levenshtein-Distance did. There I go again...learning something new! Thanks!
-
I need to write a select statement where Jo. Doe Doe, Jo Doe John will ALL match John Doe... and where Ja. Doe Doe, Ja Doe Jane will ALL match Jane Doe. So, Initials and full last name are given...is this possible? I've only worked with LIKE...and that didnt' work. Is there another function I'm not aware of?
-
Step two of this project requires building the html form (dynamically) based on what the user has already selected. I thought there was a way to do this in php, but my searching on Google lead me to jquery and ajax (of which I know neither of them!). I want to have an array of options for a form select box. Once the user selects the first select box, a second select box shows up with every option EXCEPT WHAT WAS SELECTED IN THE FIRST BOX...and repeats it's self until the array is empty (every array item has been selected). Can this be done in PHP? This is what I have so far...which does work...but it's only 2 select boxes...of 20! I can only hope it can done simpler than this: <?php $fields = array('Number', 'Name', 'Picture', 'Position', 'Height', 'Weight', 'Year', 'Home', 'Last School'); $submitted = array("$_POST[column1]", "$_POST[column2]", "$_POST[column3]", "$_POST[column4]", "$_POST[column5]", "$_POST[column6]", "$_POST[column7]", "$_POST[column8]", "$_POST[column9]", "$_POST[column10]"); ?> <form name="form1" method="post" action=""> Column 1 - <label> <select name="column1" id="column1"> <option value="">Select The Value of Column 1</option> <?php foreach ($fields as $field) { echo "<option value='$field'"; if ($_POST['column1'] == $field) { echo " selected"; } echo ">$field</option>\n"; } ?> </select> </label><br> <br> <?php if (isset($_POST['column1'])) { echo 'Column 2 - <select name="column2" id="column2">'; $column_array[] = $_POST['column1']; $array_diff = array_diff($fields,$column_array); if (isset($_POST['column2'])) { echo "$_POST[column1]"; } else { foreach ($array_diff as $fields) { echo "<option value='$fields'"; if ($_POST['column2'] == $fields) { echo " selected"; } echo ">$fields</option>\n"; } } } echo "</select>"; ?> <br /><br /> <label> <input type="submit" name="button" id="button" value="Submit"> </label> </form>
-
That's what I'm looking for...thanks for the quick reply!
-
Is there a function to determine which $_POST variable = foo? I have a form with 20 elements...and need to know which one equals another variable that's set in the script...and don't want to write a 400 layer deep if statement. Thanks!