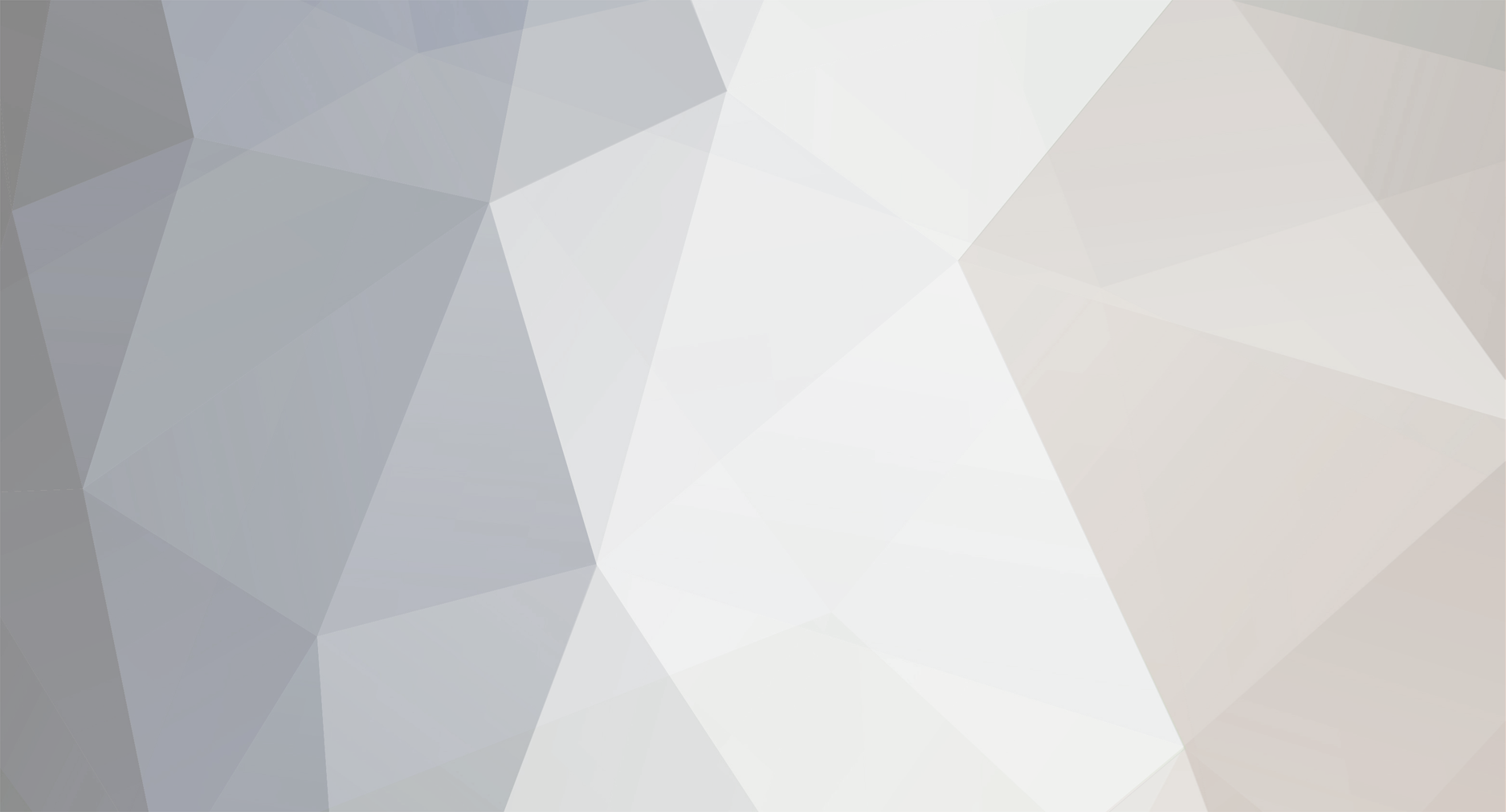
kjtocool
Members-
Posts
187 -
Joined
-
Last visited
Never
Everything posted by kjtocool
-
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
thorpe, did you get a chance to look at this again? -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
any ideas? -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
Maybe I should fully show my intentions: <?php ... code // Gets the last week_id with actuals, and the actual values $query = "SELECT * FROM bonanza_actuals ORDER BY week_id DESC LIMIT 1"; $result = mysqli_query($databaseConnect, $query); $row = mysqli_fetch_assoc($result); $week_id = $row['week_id']; $movie_1_actual_name = $row['movie_1_name']; $movie_1_actual_gross = $row['movie_1_gross']; $movie_2_actual_name = $row['movie_2_name']; $movie_2_actual_gross = $row['movie_2_gross']; $movie_3_actual_name = $row['movie_3_name']; $movie_3_actual_gross = $row['movie_3_gross']; $movie_4_actual_name = $row['movie_4_name']; $movie_4_actual_gross = $row['movie_4_gross']; $movie_5_actual_name = $row['movie_5_name']; $movie_5_actual_gross = $row['movie_5_gross']; $movie_6_actual_name = $row['movie_6_name']; $movie_6_actual_gross = $row['movie_6_gross']; $movie_7_actual_name = $row['movie_7_name']; $movie_7_actual_gross = $row['movie_7_gross']; $movie_8_actual_name = $row['movie_8_name']; $movie_8_actual_gross = $row['movie_8_gross']; $movie_9_actual_name = $row['movie_9_name']; $movie_9_actual_gross = $row['movie_9_gross']; $movie_10_actual_name = $row['movie_10_name']; $movie_10_actual_gross = $row['movie_10_gross']; mysqli_free_result($result); $actuals_array = array("$movie_1_actual_name" => $movie_1_actual_gross, "$movie_2_actual_name" => $movie_2_actual_gross, "$movie_3_actual_name" => $movie_3_actual_gross, "$movie_4_actual_name" => $movie_4_actual_gross, "$movie_5_actual_name" => $movie_5_actual_gross, "$movie_6_actual_name" => $movie_6_actual_gross, "$movie_7_actual_name" => $movie_7_actual_gross, "$movie_8_actual_name" => $movie_8_actual_gross, "$movie_9_actual_name" => $movie_9_actual_gross, "$movie_10_actual_name" => $movie_10_actual_gross); asort($actuals_array, SORT_NUMERIC); $rank = 10; foreach($actuals_array AS $name => $gross) { $${'movie_' . $rank . '_actual_name'} = $name; $${'movie_' . $rank . '_actual_gross'} = $gross; $rank--; } ?> Essentially I do the following: First I get the movie names and how much money they made from the database. They are stored there in no particular order. Second, I sort the array numerically (it will give the lowest number first, so they come out in reverse order, thus why $rank starts at 10). I want to loop through each item of the array, in reverse order, set the first item out of the array to the previously defined variable, $movie_10_actual_name, and $movie_10_actual_gross. And then so on through the 10 values in the array all the way down to #1. Thus giving me the values, ordered properly from highest to lowest. But it doesn't assign the value to the variable, and that's my issue. -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
I can't say for sure, I got it from thorpe. It seems to create some type of concatenated variable, but I can't seem to call it. -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
Anyone? -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
Having an issue with the loop now: <?php while ($row) { $${'place_' . $place . '_score'} = $row['score']; echo $place_1_score . "<br />"; $place++; $row = mysqli_fetch_assoc($result); ?> The above will echo blank lines all the way down, but if I replace $place_1_score in the echo statement with: $${'place_' . $place . '_score'}, then it echo's fine. What is going wrong? -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
Worked like a charm, thanks so much, never would have got that. -
[SOLVED] Question on looping variables - Variable won't increment!
kjtocool replied to kjtocool's topic in PHP Coding Help
<?php $rank = 1; $variable = "movie"; foreach($actuals_array AS $name => $gross) { $variable . $rank = $name; echo $variable1; } ?> I tried the above, it echo's a blank. I want $variable1 then $variable2 then $variable3, etc. -
I want to increment a php variable in a loop ... hard to explain ... let me show you $rank = 1; foreach (something) { $variable[$rank] = something; $rank++; } So basically, I want it to assign the first value of an array to $variable1, the second to $variable2, etc. I can't figure out the correct syntax?
-
Help sorting numerically: Can I change sort order?
kjtocool replied to kjtocool's topic in PHP Coding Help
OK, Now I am wondering, how do I sort DESC? My sort is working fine, but it's sorting smallest to biggest, I want it to sort biggest to smallest. Is this possible? Current code: <?php asort($scores_array, SORT_NUMERIC); $rank = 0; foreach ($scores_array as $user_id => $score) { echo "UID: " . $user_id . " = Score: ." . $score . " Rank: " . $rank . "<br />"; $rank++; }?> Prints: UID: 2529 = Score: .8.65 Rank: 0 UID: 539 = Score: .10.172 Rank: 1 UID: 1140 = Score: .10.717 Rank: 2 UID: 74 = Score: .13.333 Rank: 3 UID: 2 = Score: .14.444 Rank: 4 UID: 4150 = Score: .21.381 Rank: 5 UID: 107 = Score: .21.692 Rank: 6 UID: 2809 = Score: .30.333 Rank: 7 UID: 66 = Score: .32.477 Rank: 8 UID: 2519 = Score: .35.167 Rank: 9 UID: 4153 = Score: .35.775 Rank: 10 UID: 3200 = Score: .38.684 Rank: 11 UID: 52 = Score: .40.638 Rank: 12 UID: 268 = Score: .40.881 Rank: 13 UID: 149 = Score: .46.728 Rank: 14 UID: 34 = Score: .52.452 Rank: 15 UID: 13 = Score: .53.271 Rank: 16 UID: 33 = Score: .62.935 Rank: 17 UID: 7 = Score: .65.381 Rank: 18 UID: 2400 = Score: .71.954 Rank: 19 UID: 535 = Score: .83.06 Rank: 20 UID: 38 = Score: .86.27 Rank: 21 -
Yep, that's exactly what I was looking for. Thanks.
-
That's a good question. I suppose yes I do. Example: 9.126709098 I would want: 9.127
-
Can I use substr() on numerical float variables? For example, if I have 9.33333333333333333333333333333333333333 repeating, and I want to only keep the first 5 characters (including decimal point), how do I go about doing so?
-
[SOLVED] Opinions wanted on which way to code a program
kjtocool replied to kjtocool's topic in PHP Coding Help
Database structure is like so: Weeks - week_id - prediction_end - weekend_start_date - weekend_end_date Predictions - week_id - movie_1_name - movie_1_prediction . . . - movie_10_name - movie_10_prediction Actuals - week_id - movie_1_name - movie_1_gross . . . - movie_10_name - movie_10_gross So each week, each user predicts what the top 10 movies will make. I store their guesses in a database. Later on, when the numbers come out, I store the real values in a separate table. I want to show results ... so ... show which users have predicted the best over the course of a month, year, each specific week, etc. As I see it I can either: A) Update the Prediction table and storing the score the user gets. To do this I would have to run a script every week. Then, instead of having to select all the data, and running calculations on it, I could just pull the score, or in the case of a yearly chart, pull all the scores from a user and average them. Much simpler than pulling all 10 names and the prediction for each, and running it through a function to get the score, and doing it for every user. or B) I don't store the score, don't have to run an update script every week, and just pull all the data every time anyone wants to see it. I really can't decide. -
[SOLVED] Opinions wanted on which way to code a program
kjtocool replied to kjtocool's topic in PHP Coding Help
I would say I can expect somewhere around 200-600 predictions each week. And probably around 600-1500 participating users overall. I like your idea of a table for live display, but in general, once the values are set, there set for good. They only need to be updated once, and then there fixed (with the exception of a users overall average, which needs updated each week. So I would need hundreds of results tables, which I'd have to update all the time. -
For the past few weeks I have been building a web application / text based game coded in php. The game involves movies, and predicting how much money the top 10 movies will make week to week at the box office. I was doing a bit of work on the game tonight, and ran into a fundamental issue that I became a bit worried about, and I wanted to get your opinion. The basic deal is this: There will be a section where users can view results of a specific week, a month, a year, all time, etc. Each time someone queries any type of chart ... like the past week's results ... a month ago's results ... essentially any type of chart showing and ranking scores ... I was planning on querying all the relevant predictions, calculating their score, and then displaying the results ... each and every time someone queried some result. My question is, do you think this is OK, or do you think it will create too large a server load? The site has it's own dedicated server. Each time I display a result, I would have to: - Run a query to select all predictions - Create a result set - Iterate through the results - Pass the 20 actual values and the 20 predicted values to a function which returns the users score - Run another query pulling the user's name from a separate database based on the user_id - Create an array with all username's and the corresponding score - Sort the array - Print out the results I'm not so worried about the load for Weekly Chart's, but when it comes to things like a yearly chart, where I'd have to calculate 52 predictions for every user ... well the load could get nasty. Same goes for the overall chart ... where I'd have to find the average for every user's prediction. I suppose the alternate is adding a new column to the table where I store each user's prediction, and then running a script to update each prediction with a score, each week. That was what I wanted to avoid, but the more I thought about it, the more worried I became about the load it would create on the server. So I thought I would ask what you all thought ...
-
SELECT * FROM bonanza_weeks WHERE bonanza_weeks.week_id NOT IN ( SELECT bonanza_actuals.week_id FROM bonanza_actuals WHERE bonanza_actuals.week_id = bonanza_weeks.week_ID ) Works perfectly. Thanks a bunch!
-
SELECT * FROM bonanza_weeks WHERE bonanza_weeks.week_id NOT IN ( SELECT * FROM bonanza_actuals WHERE bonanza_actuals.week_id = bonanza_weeks.week_ID ) Returns Error: #1241 - Operand should contain 1 column(s)
-
I tried: SELECT * FROM bonanza_weeks LEFT JOIN bonanza_actuals ON bonanza_weeks.week_id = bonanza_actuals.week_id It doesn't work properly, it just returns every row in bonanza_weeks. In each table, week_id is a unique value, only appearing once per table.
-
I have two tables: weeks and actuals. I need to write a query which selects all rows from the weeks table that aren't in the actuals table. Both tables share a week_id which should be used for the comparison. So I need something like: SELECT * FROM weeks WHERE weeks.week_id doesn't exist in actuals ...
-
http://us.php.net/reserved.variables Look at SCRIPT_FILENAME or SCRIPT_NAME, maybe that will help?
-
I'm not sure, but I do something like the following: <?php include($_SERVER['DOCUMENT_ROOT'] . "/header.php"); ?> Maybe there is a similar statement for something above the root directory?
-
Help sorting numerically: Can I change sort order?
kjtocool replied to kjtocool's topic in PHP Coding Help
Bah, it's because I forgot to return $array in my function. -
Help sorting numerically: Can I change sort order?
kjtocool replied to kjtocool's topic in PHP Coding Help
Yeah, thanks. Silly mistake. Now it's throwing another error: <?php function order_movies($name1, $gross1, $name2, $gross2, $name3, $gross3, $name4, $gross4, $name5, $gross5, $name6, $gross6, $name7, $gross7, $name8, $gross8, $name9, $gross9, $name10, $gross10) { $array = array("$name1" => $gross1, "$name2" => $gross2, "$name3" => $gross3, "$name4" => $gross4, "$name5" => $gross5, "$name6" => $gross6, "$name7" => $gross7, "$name8" => $gross8, "$name9" => $gross9, "$name10" => $gross10); asort($array, SORT_NUMERIC); print_r($array); echo "<br /><br />"; } if (isset($_SESSION['user_name'])) { $user_name = $_SESSION['user_name']; $user_id = $_SESSION['user_id']; $week_id = $_POST['week_id']; $movie_1_name = $_POST['movie_1_name']; $movie_1_prediction = $_POST['movie_1_prediction']; $movie_2_name = $_POST['movie_2_name']; $movie_2_prediction = $_POST['movie_2_prediction']; $movie_3_name = $_POST['movie_3_name']; $movie_3_prediction = $_POST['movie_3_prediction']; $movie_4_name = $_POST['movie_4_name']; $movie_4_prediction = $_POST['movie_4_prediction']; $movie_5_name = $_POST['movie_5_name']; $movie_5_prediction = $_POST['movie_5_prediction']; $movie_6_name = $_POST['movie_6_name']; $movie_6_prediction = $_POST['movie_6_prediction']; $movie_7_name = $_POST['movie_7_name']; $movie_7_prediction = $_POST['movie_7_prediction']; $movie_8_name = $_POST['movie_8_name']; $movie_8_prediction = $_POST['movie_8_prediction']; $movie_9_name = $_POST['movie_9_name']; $movie_9_prediction = $_POST['movie_9_prediction']; $movie_10_name = $_POST['movie_10_name']; $movie_10_prediction = $_POST['movie_10_prediction']; $array = order_movies($movie_1_name, $movie_1_prediction, $movie_2_name, $movie_2_prediction, $movie_3_name, $movie_3_prediction, $movie_4_name, $movie_4_prediction, $movie_5_name, $movie_5_prediction, $movie_6_name, $movie_6_prediction, $movie_7_name, $movie_7_prediction, $movie_8_name, $movie_8_prediction, $movie_9_name, $movie_9_prediction, $movie_10_name, $movie_10_prediction); $movie_rank = 10; // The next line is 265 and throwing an error foreach ($array as $name => $gross) { if ($movie_rank == 10) { $movie_10_name = $name; $movie_10_prediction = $gross; } else if ($movie_rank == 9) { $movie_9_name = $name; $movie_9_prediction = $gross; } else if ($movie_rank == { $movie_8_name = $name; $movie_8_prediction = $gross; } else if ($movie_rank == 7) { $movie_7_name = $name; $movie_7_prediction = $gross; } else if ($movie_rank == 6) { $movie_6_name = $name; $movie_6_prediction = $gross; } else if ($movie_rank == 5) { $movie_5_name = $name; $movie_5_prediction = $gross; } else if ($movie_rank == 4) { $movie_4_name = $name; $movie_4_prediction = $gross; } else if ($movie_rank == 3) { $movie_3_name = $name; $movie_3_prediction = $gross; } else if ($movie_rank == 2) { $movie_2_name = $name; $movie_2_prediction = $gross; } else if ($movie_rank == 1) { $movie_1_name = $name; $movie_1_prediction = $gross; } $movie_rank--; } echo $movie_1_name . " " . $movie_2_name . " " . $movie_3_name . " " . $movie_4_name. " " . $movie_5_name . " " . $movie_6_name . " " . $movie_7_name . " " . $movie_8_name . " " . $movie_9_name . " " . $movie_10_name; ?> Error: Warning: Invalid argument supplied for foreach() in /home/worldofk/public_html/bonanza_entryVal.php on line 265 -
Help sorting numerically: Can I change sort order?
kjtocool replied to kjtocool's topic in PHP Coding Help
OK, so I fixed the above issue, but am now having a different problem: <?php // Returns the movies in reverse order, starting with the smallest, and going to the biggest. function order_movies($name1, $gross1, $name2, $gross2, $name3, $gross3, $name4, $gross4, $name5, $gross5, $name6, $gross6, $name7, $gross7, $name8, $gross8, $name9, $gross9, $name10, $gross10) { $array = array("$name1" => $gross1, "$name2" => $gross2, "$name3" => $gross3, "$name4" => $gross4, "$name5" => $gross5, "$name6" => $gross6, "$name7" => $gross7, "$name8" => $gross8, "$name9" => $gross9, "$name10" => $gross10); asort($array, SORT_NUMERIC); $movie_rank = 10 // The next line is # 263 throwing an error foreach ($array as $name => $gross) { if ($movie_rank == 10) { $movie_10_name = $name; $movie_10_prediction = $gross; } else if ($movie_rank == 9) { $movie_9_name = $name; $movie_9_prediction = $gross; } else if ($movie_rank == { $movie_8_name = $name; $movie_8_prediction = $gross; } else if ($movie_rank == 7) { $movie_7_name = $name; $movie_7_prediction = $gross; } else if ($movie_rank == 6) { $movie_6_name = $name; $movie_6_prediction = $gross; } else if ($movie_rank == 5) { $movie_5_name = $name; $movie_5_prediction = $gross; } else if ($movie_rank == 4) { $movie_4_name = $name; $movie_4_prediction = $gross; } else if ($movie_rank == 3) { $movie_3_name = $name; $movie_3_prediction = $gross; } else if ($movie_rank == 2) { $movie_2_name = $name; $movie_2_prediction = $gross; } else if ($movie_rank == 1) { $movie_1_name = $name; $movie_1_prediction = $gross; } } } $movie_1_name = $_POST['movie_1_name']; $movie_1_prediction = $_POST['movie_1_prediction']; $movie_2_name = $_POST['movie_2_name']; $movie_2_prediction = $_POST['movie_2_prediction']; $movie_3_name = $_POST['movie_3_name']; $movie_3_prediction = $_POST['movie_3_prediction']; $movie_4_name = $_POST['movie_4_name']; $movie_4_prediction = $_POST['movie_4_prediction']; $movie_5_name = $_POST['movie_5_name']; $movie_5_prediction = $_POST['movie_5_prediction']; $movie_6_name = $_POST['movie_6_name']; $movie_6_prediction = $_POST['movie_6_prediction']; $movie_7_name = $_POST['movie_7_name']; $movie_7_prediction = $_POST['movie_7_prediction']; $movie_8_name = $_POST['movie_8_name']; $movie_8_prediction = $_POST['movie_8_prediction']; $movie_9_name = $_POST['movie_9_name']; $movie_9_prediction = $_POST['movie_9_prediction']; $movie_10_name = $_POST['movie_10_name']; $movie_10_prediction = $_POST['movie_10_prediction']; $array = order_movies($movie_1_name, $movie_1_prediction, $movie_2_name, $movie_2_prediction, $movie_3_name, $movie_3_prediction, $movie_4_name, $movie_4_prediction, $movie_5_name, $movie_5_prediction, $movie_6_name, $movie_6_prediction, $movie_7_name, $movie_7_prediction, $movie_8_name, $movie_8_prediction, $movie_9_name, $movie_9_prediction, $movie_10_name, $movie_10_prediction); ?> The above is throwing an error: Parse error: syntax error, unexpected T_FOREACH in /home/worldofk/public_html/bonanza_entryVal.php on line 263