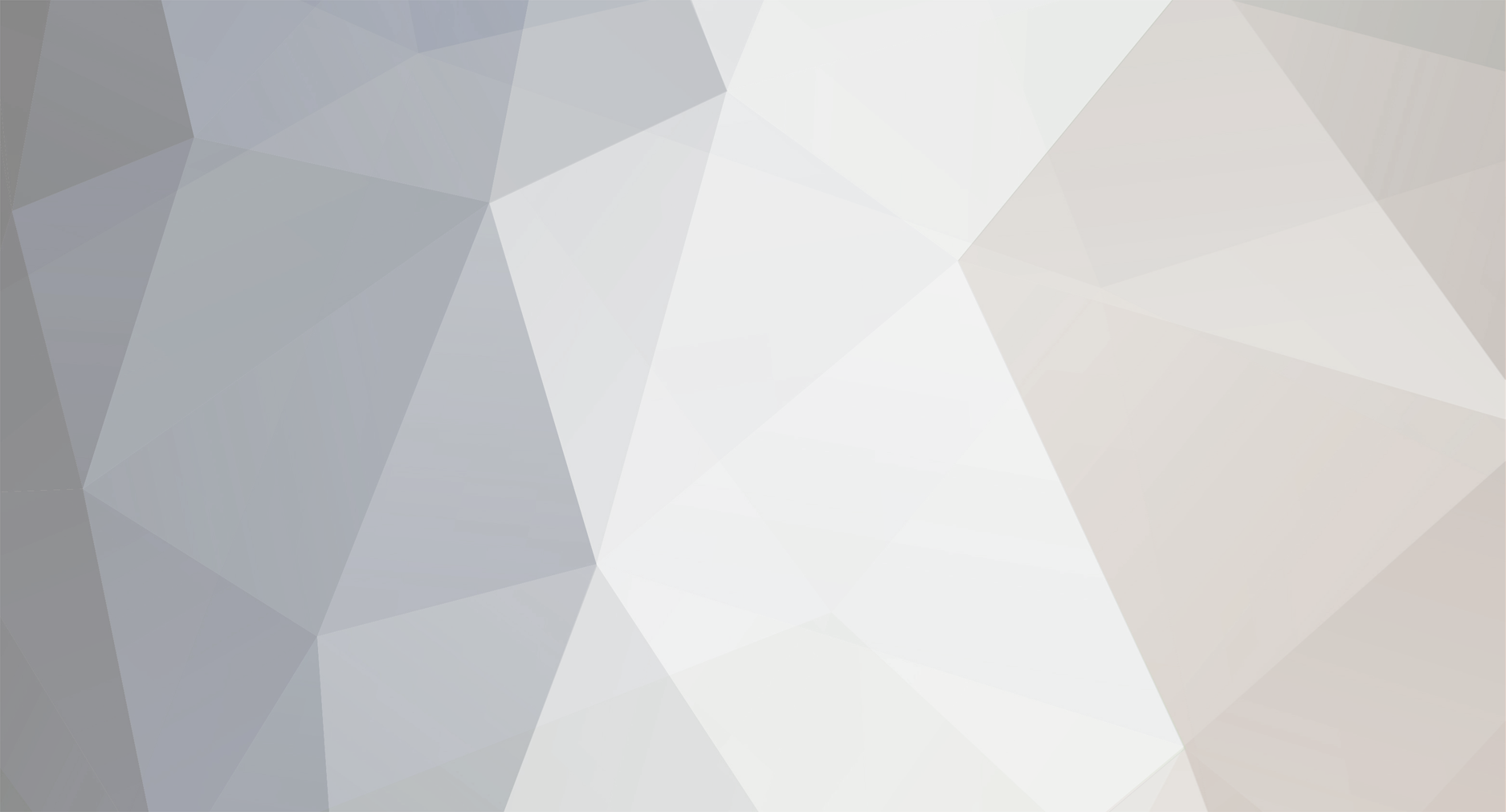
kjtocool
Members-
Posts
187 -
Joined
-
Last visited
Never
Everything posted by kjtocool
-
How do you decide which functions to use, mysql_ or mysqli_ ? I see most people on these forums using mysql_ , though I personally tend to use mysqli_ . In general, I was wondering your thoughts on which to use, and when.
-
I was going on what he said here, where he makes it sound like he wants only the last 25 rows. You are right though, my code only is faulty.
-
If the table is auto_incremented, does it not make more sense to simply get the last incremented value, and use it to get the exact values to not delete?
-
Try simplifying: <?php if (isset($_GET['username'])) { $username = $_GET['username']; $query = "SELECT * FROM users WHERE Username = '$username' LIMIT 1"; $result = mysql_query($query) if ($result) { if (mysql_num_rows($result)) { $array = mysql_fetch_assoc($result); $pID = $array['ID']; $puser = $array['Username']; echo $puser . " " . $pID; } else { echo "No users found with id $id<br />"; } } else { echo "Query failed<br />$sql<br />" . mysql_error(); } } else { echo "No ID passed"; } ?> You had at least 1 syntax error in the last block of code you posted.
-
Or <?php $databaseConnect = mysqli_connect("localhost", "username", "password", "database"); $result = mysqli_query($databaseConnect, "SELECT LAST_INSERT_ID()"); $last_row_to_delete = $result - 26; $query = "DELETE FROM table_name WHERE column_id >= $last_row_to_delte"; if (mysqli_query($databaseConnect, $query)) echo "Success"; else echo "Failure"; ?>
-
It all depends on the ID. Assuming you used an auto_incrementing ID, starting at 1, which also serves as a primary key, and you know the ID you want to not delete, you could do something like this: DELETE FROM table_name WHERE column_id > 74 If you don't know the ID, you should first do a statement to select the last inserted value, then subtract 26.
-
[SOLVED] MySQL query to pull usernames based on user_id
kjtocool replied to drummer101's topic in MySQL Help
First off, your using mysql_ functions, which I am not familiar with. I tend to use the updated mysqli_ functions. If I were using those, I would try the following: <?php $databaseConnection = mysqli_connect("localhost", "username", "password", "database") Or die("Unable to connect to the database."); $query = "SELECT phpbb_user_group.user_id, phpbb_users.username FROM phpbb_user_group, phpbb_users WHERE phpbb_user_group.group_id = 6 AND phpbb_users.user_id = phpbb_user_group.user_id"; $result = mysqli_query($databaseConnect, $query); $row = mysqli_fetch_assoc($result); while ($row) { $username = $row['username']; $user_id = $row['user_id']; echo "Username = " . $username . " - User_ID = " . $user_id; $row = mysqli_fetch_assoc($result); } ?> -
[SOLVED] Help! Error while looping through query results.
kjtocool replied to kjtocool's topic in MySQL Help
The $userID and $user_ID issue was what was giving me the error. Thanks! I think you're right about the join, I'll try that out and see if I can't get it to work. EDIT: The join worked great. Thanks again for your help. -
[SOLVED] MySQL query to pull usernames based on user_id
kjtocool replied to drummer101's topic in MySQL Help
Couldn't you do something like: SELECT phpbb_user_group.user_id, phpbb_users.username FROM phpbb_user_group, phpbb_users WHERE phpbb_user_group.group_id = 6 AND phpbb_users.user_id = {$i[user_id]} -
Hey guys, any help is greatly appreciated. In short, I make a $databaseConnect variable earlier in the code. I then run a query on the database, and get a results set. I enter into a while loop to go through the results, and each time through the loop, I want to query the database again to find something else. I use the same $databaseConnect variable. I am getting an error when trying to query the database the second time through: <?php $query = "SELECT * FROM Comments WHERE article_ID = $article_ID"; $result = mysqli_query($databaseConnect, $query); $row = mysqli_fetch_assoc($result); while ($row) { $userID = $row['user_ID']; $commentID = $row['comment_ID']; $comment = $row['comment']; $query2 = "SELECT username FROM Users WHERE user_ID = $user_ID"; $result2 = mysqli_query($databaseConnect, $query2); $row2 = mysqli_fetch_assoc($result2); $userName = $row2['username']; mysqli_free_result($result2); echo '<table width="70%" border="0" align="center" cellpadding="0" cellspacing="1">'; echo '<tr>'; echo '<td bgcolor="#F4FAFF" class="style6">Author: ' . $userName . ' ( ' . $userID . ' )</td>'; echo '</tr>'; echo '<tr>'; echo '<td bgcolor="#F4FAFF" class="style6">' . $comment . '</td>'; echo '</tr>'; echo '</table><br />'; $row = mysqli_fetch_assoc($result); } mysqli_free_result($result); mysqli_close($databaseConnect); ?> Any explanation as to why I can't query while still using the results of the same query would be great, as well as how I can get around this. KJ
-
I never define $userID. I defene $_SESSION['userID'] in loginVal.php here: if (mysqli_num_rows($result) == 0) echo "Username or password is incorrect."; else { $row = mysqli_fetch_assoc($result); $_SESSION['userID'] = $row['user_ID']; echo "Successful Login"; echo '<META HTTP-EQUIV="refresh" CONTENT="0.0; URL=http://www.thelink.com/options.php?' . SID . '">'; } In any case, apparently my problem was that this: if (!isset($userID)) should have been: if (!isset($_SESSION['userID'])) So it works now. Thanks a bunch!
-
Hi there, I am having a problem creating a session and holding it across pages. I start with a simple form that requests username and password. When the user clicks submit, it sends them to loginVal.php: <?php session_start(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Xtinct CMS</title> <link href="main.css" rel="stylesheet" type="text/css"> <style type="text/css" > <!-- .style1 { font-family: Arial; font-size: 16px; color: #0F45FF; font-weight: bold; } .style6 { font-family: Arial; font-size: 12px; } --> </style></head> <body> <table width="670" border="0" align="center" cellpadding="0" cellspacing="0"> <form id="form1" name="form1" method="post" action=""> <tr> <td background="images/top.jpg" height="50"> </td> </tr> <tr> <td background="images/middle.jpg" height="200" valign="top"> <table width="670" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="75"> </td> <td><p align="center"><span class="style1">Login</span></p> <p align="left" class="style6"> <?php $userName = $_POST["userName"]; $_SESSION['userName'] = $userName; $passwordHash = md5($_POST["password"]); $_SESSION['passwordHash'] = $passwordHash; $databaseConnect = mysqli_connect("localhost", "username", "password", "database_name") Or die("Unable to connect to the database."); $query = "SELECT user_ID FROM Users WHERE username = '$userName' AND password = '$passwordHash' LIMIT 1"; $result = mysqli_query($databaseConnect, $query); if (mysqli_num_rows($result) == 0) echo "Username or password is incorrect."; else { $row = mysqli_fetch_assoc($result); $_SESSION['userID'] = $row['user_ID']; echo "Successful Login"; echo '<META HTTP-EQUIV="refresh" CONTENT="0.0; URL=http://www.thelink.com/options.php?' . SID . '">'; } mysqli_free_result($result); mysqli_close($databaseConnect); ?> </p> <br /> </td> <td width="75"> </td> </tr> </table> </td> </tr> <tr> <td background="images/bottom.jpg" height="35"> </td> </tr> </form> </table> </body> </html> As you can see, I use session_start() to start the page. I then get values for username and password, hash the password, and make sure the login is valid. I pass values to a few $_SESSION variables, and if the login was valid, I redirect them to another page, options.php. On options.php I run the following check: <?php session_start(); if (!isset($userID)) { header("Location: http://www.thelink.com/index.php"); } ?> Even though I set the $_SESSION['userID'] variable, it always re-directs. While troubleshooting, I tried echoing the SID in the loginVal.php file, and it came up as an empty string. So for whatever reason, I am not generating a session ID. I am new to sessions, can someone tell me what I am doing wrong? KJ