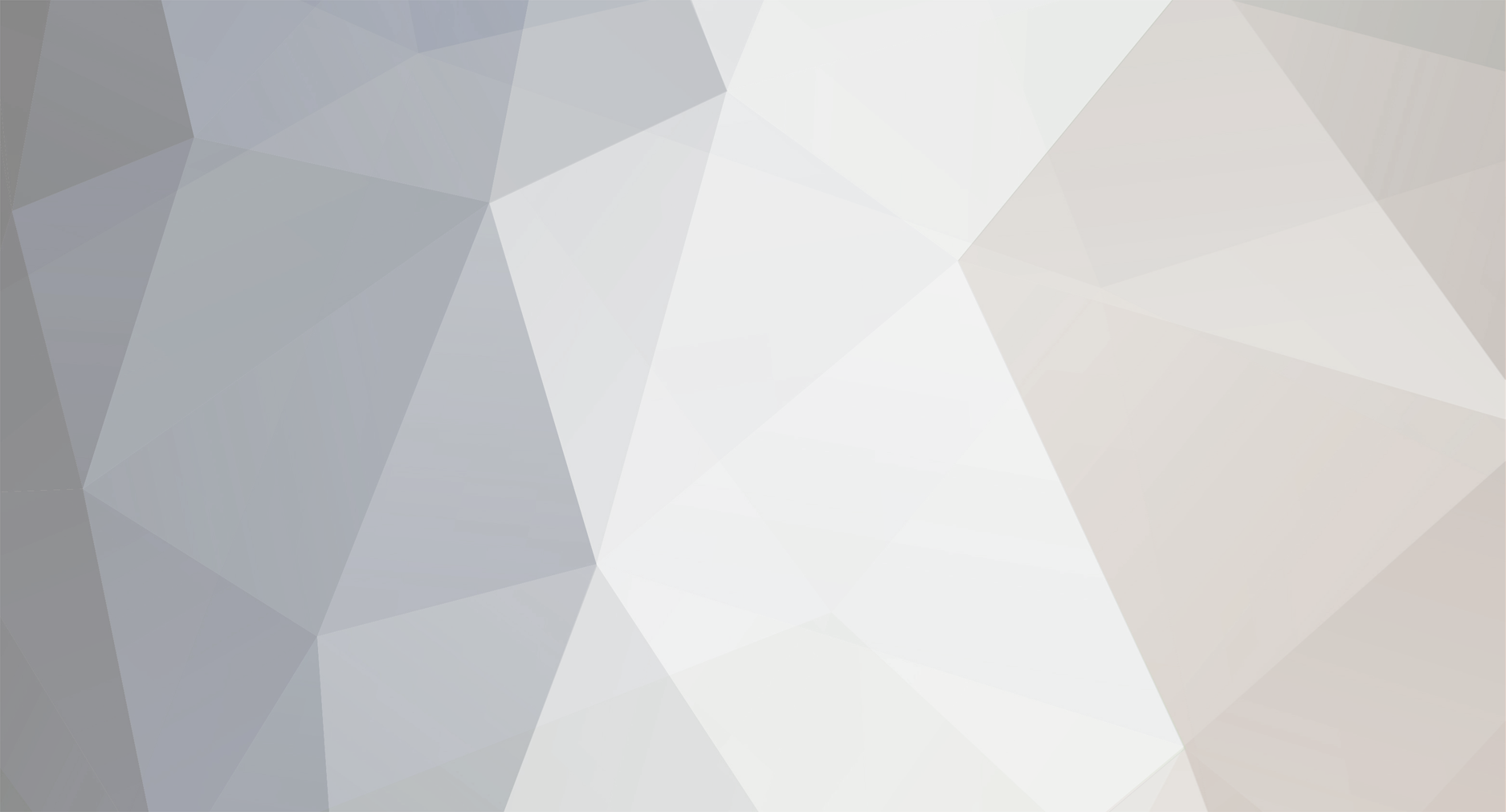
samona
Members-
Posts
232 -
Joined
-
Last visited
Never
Everything posted by samona
-
you didnt close your quotations on the die() function on line 37. Use the code below and try again. <?php include "connect.php"; if(isset($_POST['submit'])) { $username=$_POST['username']; $password=$_POST['password']; $email=$_POST['email']; if(strlen($username)<1) { print "You did not enter a username."; } else if(strlen($password)<1) { print "You did not enter a password."; } else { $insert="Insert into users (username,password,email) values('$username','$password','$email')"; mysql_query($insert) or die("Could not insert comment"); } } ?>
-
You could use regex.
-
How about right before the <?php tag? Just add another <option value="">Example</>
-
When you use for loops you would use it in the php code not the template file. And the way you would use it depends on the template class.
-
Yes it's possible.
-
you can't use the header() function when you have already sent output
-
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
OK, I just put this on my server and ran it. Should work now. The problem was the name for the submit button you had was "Submit". I changed the form so the name of the submit button is "submit" and I included an else statement. Actually, you dont need the else statement. I'll revise it... <?php if (isset($_POST['submit'])) { // Define your username and password $post_username = trim($_POST['txtUsername']); $post_password = trim($_POST['txtPassword']); $username = "test"; $password = "admin"; if ($post_username == $username && $post_password == $password) { header("Location: welcome.php"); } else { header("Location: error.php"); } } //If the user hasn't pressed the submit button then display the form ?> <h1>Login</h1> <form name="form" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p><label for="txtUsername">Username:</label> <br /><input type="text" title="Enter your Username" name="txtUsername" /></p> <p><label for="txtpassword">Password:</label> <br /><input type="password" title="Enter your password" name="txtPassword" /></p> <p><input type="submit" name="submit" value="Login" /></p> </form> -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
By the way. What are the name of your pages? -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
Try the code below. I changed the if statement to AND instead of OR <?php if (isset($_POST['submit'])) { // Define your username and password $post_username = $_POST['txtUsername']; $post_password = $_POST['txtPassword']; $username = "test"; $password = "test"; if ($post_username == $username && $post_password == $password) { header("Location: mainlogin.php"); } else { header("Location: mainlogin.php"); } } //If the user hasn't pressed the submit button then display the form ?> <h1>Login</h1> <form name="form" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p><label for="txtUsername">Username:</label> <br /><input type="text" title="Enter your Username" name="txtUsername" /></p> <p><label for="txtpassword">Password:</label> <br /><input type="password" title="Enter your password" name="txtPassword" /></p> <p><input type="submit" name="Submit" value="Login" /></p> </form> -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
For example <?php if (isset($_POST['submit']) { // Define your username and password $post_username = $_POST['txtUsername']; $post_password = $_POST['txtPassword']; $username = "someuser"; $password = "somepassword"; if ($post_username == $username && $post_password == $password) { session_start(); $_Session["login_name"] = $username; header("Location:next_page"); } else { header("Location:error_page"); } } //If the user hasn't pressed the submit button then display the form <h1>Login</h1> <form name="form" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p><label for="txtUsername">Username:</label> <br /><input type="text" title="Enter your Username" name="txtUsername" /></p> <p><label for="txtpassword">Password:</label> <br /><input type="password" title="Enter your password" name="txtPassword" /></p> <p><input type="submit" name="Submit" value="Login" /></p> </form> Then the next page would be <?php session_start(); if (!isset($_SESSION["login_name"])) { //revert them to error page if they have not logged in. header("Location:error_page.php); } //Contine with rest of your code -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
I agree with deskit. If you want a login system than sessions are the way to go. -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
Sorry, I forgot a paranthesis. Change it to this if (isset($_POST['submit'])) { // Define your username and password -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
Try the following <?php if (isset($_POST['submit']) { // Define your username and password $post_username = $_POST['txtUsername']; $post_password = $_POST['txtPassword']; $username = "someuser"; $password = "somepassword"; if ($post_username == $username || $post_password == $password) { header("Location:next_page"); } else { header("Location:error_page"); } } //If the user hasn't pressed the submit button then display the form <h1>Login</h1> <form name="form" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p><label for="txtUsername">Username:</label> <br /><input type="text" title="Enter your Username" name="txtUsername" /></p> <p><label for="txtpassword">Password:</label> <br /><input type="password" title="Enter your password" name="txtPassword" /></p> <p><input type="submit" name="Submit" value="Login" /></p> </form> -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
Oh, you want to go to another page if the password is correct. Sorry! Ummm give me a few minutes. -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
Try the code below and let me know if it works. <?php // Define your username and password $username = "someuser"; $password = "somepassword"; if ($_POST['txtUsername'] != $username || $_POST['txtPassword'] != $password) { header("Location:page_to_display_error"); else { ?> <h1>Login</h1> <form name="form" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p><label for="txtUsername">Username:</label> <br /><input type="text" title="Enter your Username" name="txtUsername" /></p> <p><label for="txtpassword">Password:</label> <br /><input type="password" title="Enter your password" name="txtPassword" /></p> <p><input type="submit" name="Submit" value="Login" /></p> </form> <? } ?> -
[SOLVED] go to other page if password is correct
samona replied to ma5ect's topic in PHP Coding Help
You can't output data before the header() function. -
try including the full path name
-
I was wondering, does an application have to be written in a certain way for it to be available on a wireless network? FOr example, are there applications that don't work on wireless?
-
I check but thats not the problem. When I mean I have to switch folders for the inbox to update I mean that it doesn't make a different if I wait 1 minute or 1 hour. The inbox updates only when i switch folders.
-
Hi all, I have a problem with outlook using the IMAP protocol. Whenever I receive email in outlook I have to switch to another folder in order for my inbox to update. As an example, if someone sends me an email. Outlook does not contain the new email. However, when I switch to another folder and back to inbox the email will finally appear. Has anyone experienced this or know of a fix?
-
thank you all!!!!!!!!!!!!!!!!!!!!!
-
When I right click on the Google logo and select view image, an image containing all the images on the page gets produced instead of viewing just the Google logo. Is that CSS?
-
Is it possible to connect one network card to two networks?