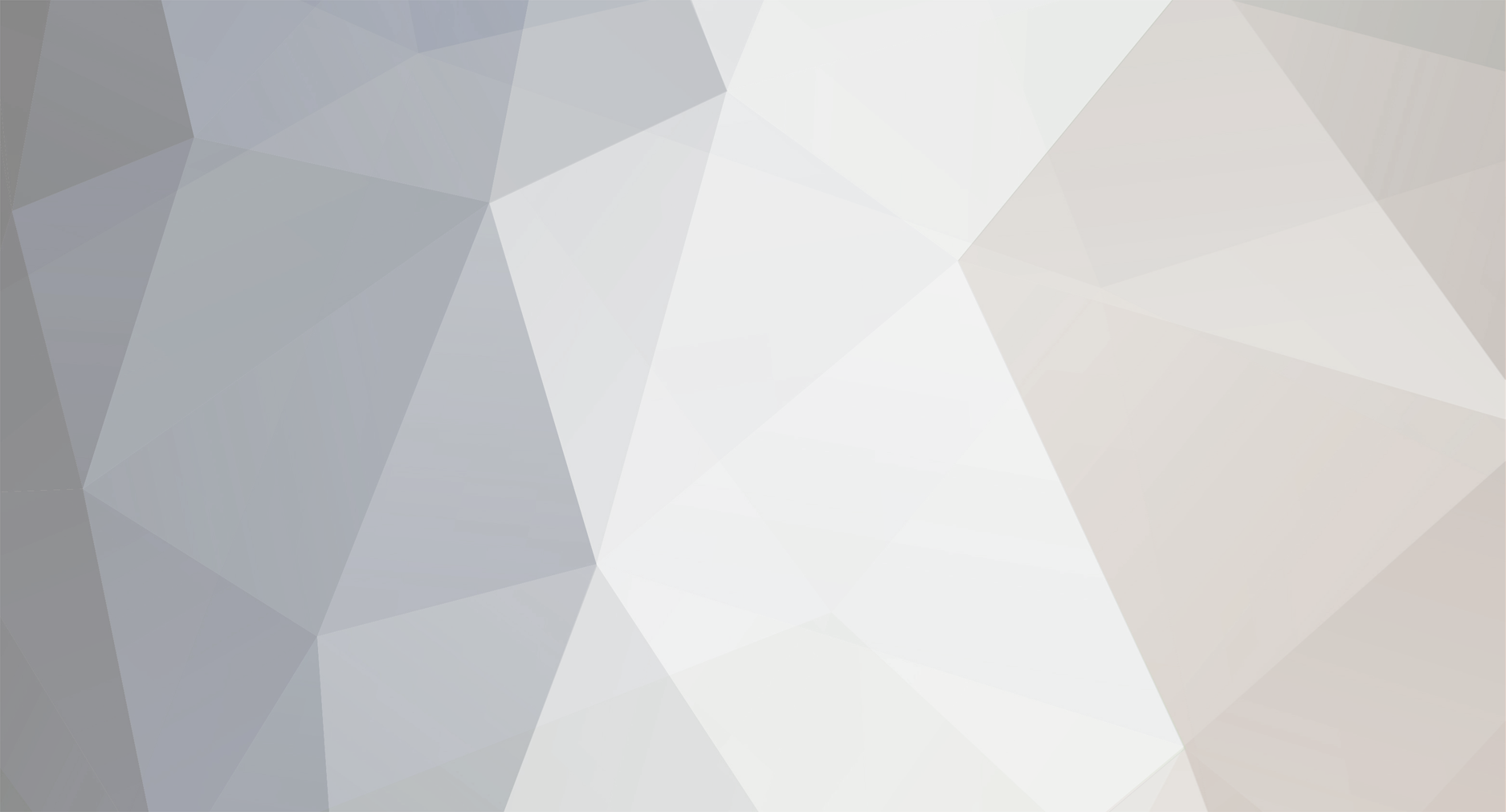
p2grace
-
Posts
1,024 -
Joined
-
Last visited
Never
Posts posted by p2grace
-
-
It'll look like <img src="getImage.php?id=55">. They will be able to copy it. If a user is required to login to access the page, you can restrict access to the image if and only if the session is active, but you can't prevent a user from being able to copy source code.
-
Your variables are different.
Your code:
if (isset($_POST['submit'])) { $id = $_POST['id']; $newStatus = $_POST['newstatus']; newStatus($id, $newstatus); exit(); }
Should be:
if (isset($_POST['submit'])) { $id = $_POST['id']; $newStatus = $_POST['newstatus']; newStatus($id, $newStatus); exit(); }
To catch these types of issues easily, it's always a good idea to display errors while in development (development only).
error_reporting(E_ALL); ini_set('display_errors','on');
-
If you are trying to secure the file, I would recommend using php to load the image through a get variable, that way you can ensure the session exists. Then that $_GET variable loads the image, and sets the header to an image. e.g. (http://www.site.com/image/?id=34kjdolskfjl2342lk) would lookup that id, load the image, and change the header of the php script to display an image.
-
I don't see where you are specifying the user status in the query. If it isn't specified, it'll set it to the default (0).
-
That syntax is called shorthand or ternary if/else statements.
Explanation: http://snippets.dzone.com/posts/show/76
Basically it's saying set $device equal to "hands" if $coffeeMaker is null, otherwise set it to the value of $coffeeMaker.
-
You're not returning a true/false statement in your second code. Also, you're using a header to redirect, which only works if the browser hasn't received data yet (if the header has been established). Turn on errors with the code below, more then likely the redirect isn't working because the header is already established.
error_reporting(E_ALL); ini_set('display_errors','on');
-
You can post data to any server (despite its server side language) with action="http://destination_url/file.php". So yes, your form could sit on an asp box and could post to a php server.
-
When you say it "doesn't work" what does that imply? Does it produce a php syntax error or warning, or behave unexpectedly?
-
You would use cookies if you want the redirects to be remembered throughout each visit. As far as tracking goes, a database would be the best option. Like I said before, you'll need to do some research and googling to learn the information you need. The link below demonstrates how to connect to a mysql database (assuming you have one created).
http://www.siteground.com/tutorials/php-mysql/php_mysql_connection.htm
-
There are a couple ways of achieving this. One method would be that all links are redirect links, and you use session variables to determine number of clicks. Then in the redirect, if it shouldn't redirect again (based off of session variable check) just send to initial link, otherwise redirect to new link.
There isn't a straight simple answer for this one, some digging and understanding of php methods (and javascript most likely) will be required.
Very easy way.
Set a cookie then if $_COOK['xxx'] > 5
header(Location: bla bla);
This could work, however if a user refreshes their page this will also redirect, and this assumes the content is templatized. You also wouldn't be able to track to which link they are referring from if that data was necessary. Also, I wouldn't recommend using cookies for this, but rather sessions that way you can use multi-dimensional arrays.
-
There are a couple ways of achieving this. One method would be that all links are redirect links, and you use session variables to determine number of clicks. Then in the redirect, if it shouldn't redirect again (based off of session variable check) just send to initial link, otherwise redirect to new link.
There isn't a straight simple answer for this one, some digging and understanding of php methods (and javascript most likely) will be required.
-
There might be an easier way, but something like this should do the trick off the top of my head.
<?php $day1 = "05-12-2009 13:44:31"; $day2 = "05-12-2009 17:55:31"; $result = strtotime($day1) - strtotime($day2); $diff = $result / 31556926; $h = date("h",$diff); $i = date("i",$diff); $h = (substr($h,0,1) == 0) ? substr($h,1) : $h; $i = (substr($i,0,1) == 0) ? substr($i,1) : $i; echo "$h hours $i minutes"; ?>
-
If it's in double-quotes it'll pass the value of the variable, not the variable itself. If its in single quotes, it'll only pass the variable string. Make sure it's in double quotes.
-
First, to get the last insert id, just execute the following:
$id = mysql_insert_id();
That will return the id of the last insert statement performed.
Then to redirect just do the following:
header("Location: company.php?id=$id");
-
Basically I'm providing both ids separated by a colon. Then I use php's explode() method to convert that string into an array where the first element in the array is ID_A and the second element in the array is ID_E. Make sense?
-
Try this:
preupload.php
<?php include("config.inc.php"); // initialization $photo_upload_fields = ""; $counter = 1; // default number of fields $number_of_fields = 6; // If you want more fields, then the call to this page should be like, // preupload.php?number_of_fields=20 if( $_GET['number_of_fields'] ) $number_of_fields = (int)($_GET['number_of_fields']); // Firstly Lets build the Category List $result = mysql_query( "SELECT id_A,A_nombre,id_E FROM t_atracciones ORDER BY A_nombre" ); while( $row = mysql_fetch_array( $result ) ) { $photo_category_list .=<<<__HTML_END <option value="{$row[0]}:{$row[2]}">$row[1]</option>\n __HTML_END; } mysql_free_result( $result ); // Lets build the Photo Uploading fields while( $counter <= $number_of_fields ) { $photo_upload_fields .=<<<__HTML_END <tr> <td> Photo {$counter}: <input name=' photo_filename[]' type='file' /> </td> </tr> <tr> <td> Caption: <textarea name='photo_caption[]' cols='30' rows='1'></textarea> </td> </tr> __HTML_END; $counter++; } // Final Output echo <<<__HTML_END <html> <head> <title>Lets upload Photos</title> </head> <body> <form enctype='multipart/form-data' action='upload.php' method='post' name='upload_form'> <table width='90%' border='0' align='center' style='width: 90%;'> <tr> <td> <select name='category'> <option value="">Seleccione una atracción</option> $photo_category_list </select> </td> </tr> <tr> <td> <p> </p> </td> </tr> <!-Insert the photo fields here --> $photo_upload_fields <tr> <td> <input type='submit' name='submit' value='Add Photos' /> </td> </tr> </table> </form> </body> </html> __HTML_END; ?>
upload.php
<?php include("config.inc.php"); // initialization $result_final = ""; $counter = 0; // List of our known photo types $known_photo_types = array( 'image/pjpeg' => 'jpg', 'image/jpeg' => 'jpg', 'image/gif' => 'gif', 'image/bmp' => 'bmp', 'image/x-png' => 'png' ); // GD Function List $gd_function_suffix = array( 'image/pjpeg' => 'JPEG', 'image/jpeg' => 'JPEG', 'image/gif' => 'GIF', 'image/bmp' => 'WBMP', 'image/x-png' => 'PNG' ); // Fetch the photo array sent by preupload.php $photos_uploaded = $_FILES['photo_filename']; // Fetch the photo caption array $photo_caption = $_POST['photo_caption']; while( $counter <= count($photos_uploaded) ) { if($photos_uploaded['size'][$counter] > 0) { if(!array_key_exists($photos_uploaded['type'][$counter], $known_photo_types)) { $result_final .= "File ".($counter+1)." is not a photo<br />"; } else { $cat = explode(":",$_POST['category']); $id_A = $cat[0]; $id_E = $cat[1]; mysql_query( "INSERT INTO t_gallery_photos(`photo_filename`, `photo_caption`, `id_A`,`id_E`) VALUES('0', '".mysql_real_escape_string($photo_caption[$counter])."', '".mysql_real_escape_string($id_A)."', '".mysql_real_escape_string($id_E)."')" ); $new_id = mysql_insert_id(); $filetype = $photos_uploaded['type'][$counter]; $extention = $known_photo_types[$filetype]; $filename = $new_id.".".$extention; mysql_query( "UPDATE t_gallery_photos SET photo_filename='".mysql_real_escape_string($filename)."' WHERE photo_id='".mysql_real_escape_string($new_id)."'" ); // Store the orignal file copy($photos_uploaded['tmp_name'][$counter], $images_dir."/".$filename); // Let's get the Thumbnail size $size = GetImageSize( $images_dir."/".$filename ); if($size[0] > $size[1]) { $thumbnail_width = 100; $thumbnail_height = (int)(100 * $size[1] / $size[0]); } else { $thumbnail_width = (int)(100 * $size[0] / $size[1]); $thumbnail_height = 100; } // Build Thumbnail with GD 1.x.x, you can use the other described methods too $function_suffix = $gd_function_suffix[$filetype]; $function_to_read = 'ImageCreateFrom' . $function_suffix; $function_to_write = 'Image' . $function_suffix; // Read the source file $source_handle = $function_to_read($images_dir . '/' . $filename); if ($source_handle) { // Let's create a blank image for the thumbnail $destination_handle = ImageCreateTrueColor($thumbnail_width, $thumbnail_height); // Now we resize it ImageCopyResampled($destination_handle, $source_handle, 0, 0, 0, 0, $thumbnail_width, $thumbnail_height, $size[0], $size[1]); } // Let's save the thumbnail $function_to_write($destination_handle, $images_dir . '/tb_' . $filename); $result_final .= "<img src='".$images_dir. "/tb_".$filename."' /> File ".($counter+1)." Added<br />"; } } $counter++; } // Print Result echo <<<__HTML_END <html> <head> <title>Photos uploaded</title> </head> <body> $result_final </body> </html> __HTML_END; ?>
-
Try this for the display:
<?php // Firstly Lets build the Category List $result = mysql_query( "SELECT id_A,A_nombre,ID_E FROM t_atracciones ORDER BY A_nombre" ); while( $row = mysql_fetch_assoc($result)){ $photo_category_list .=<<<__HTML_END <option value="{$row['id_A']}:{$row['id_E']}">{$row['A_nombre']}</option>\n __HTML_END; } ?>
And this for the insert
<?php $id = mysql_real_escape_string(explode(":",$_POST['category'])); $id_A = $id[0]; $id_E = $id[1]; mysql_query( "INSERT INTO t_gallery_photos(`photo_filename`, `photo_caption`, `id_A`,`id_E`) VALUES('0', '".addslashes($photo_caption[$counter])."', '$id_A','$id_E)" ); $new_id = mysql_insert_id(); $filetype = $photos_uploaded['type'][$counter]; $extention = $known_photo_types[$filetype]; $filename = $new_id.".".$extention; mysql_query( "UPDATE t_gallery_photos SET photo_filename='".addslashes($filename)."' WHERE photo_id='".addslashes($new_id)."'" ); ?>
Also note, that you shouldn't use addslashes here, but rather mysql_real_escape_string().
http://us2.php.net/manual/en/function.mysql-real-escape-string.php
-
There are a few ways of doing this. The easiest way would probably to set the value of the option to be id_a:id_e and then use explode() to convert the string into an array where element 0 of the array is the id_a and element 1 of the array is id_e. Make sense?
-
The ampersand in front of a variable instructs apache to pass the variable's reference instead of the variable's value.
-
One option would be instead of literally creating all of these files just to fill their contents from the database, is to have only one file in the root, which would then load the contents from the db based off of the path specified in the url. You'd have to use a series of mod_rewrites in an htaccess file to make it work, but there are plenty of examples in google to help with that.
-
I would start by verifying the contents of the $contents array. The query fails because $id is empty, which means the contents of the array aren't correct.
-
If it's an xml string you can use SimpleXMLElement():
http://us2.php.net/manual/en/simplexml.examples-basic.php
If its a file you can use simplexml_load_file():
http://us2.php.net/manual/en/function.simplexml-load-file.php
-
print_r() displays the results of an array, which is why it's displaying like that. If you want to display in a table you'll need to loop through the results and construct the html.
-
$delq = "DELETE FROM '$tablename' WHERE id='$id'";
will this work?
as far as I knew, variables don't show up inside single quotes. this is how I make queries (lots of ways of doing it, this is my method)
$delq = 'DELETE FROM ' . $TableName . ' WHERE id="' . $id . '"';
try that, i think your variable isn't pasting into the query because you're using single quotes
In this case the variable will show up within the single quotes because it's wrapped by double quotes.
$delq = "DELETE FROM '$tablename' WHERE id='$id'";
will this work?
as far as I knew, variables don't show up inside single quotes. this is how I make queries (lots of ways of doing it, this is my method)
$delq = 'DELETE FROM ' . $TableName . ' WHERE id="' . $id . '"';
try that, i think your variable isn't pasting into the query because you're using single quotes
In this case the variable will display within the single quotes because it's wrapped within a double quote. AlexWD is correct that the single quotes are the problem.
is it possible to rename an image after displaying ?
in PHP Coding Help
Posted
Can it be done, yes. But it is overkill. Like mjdamato mentioned, nothing can prevent the user from doing a screenshot.