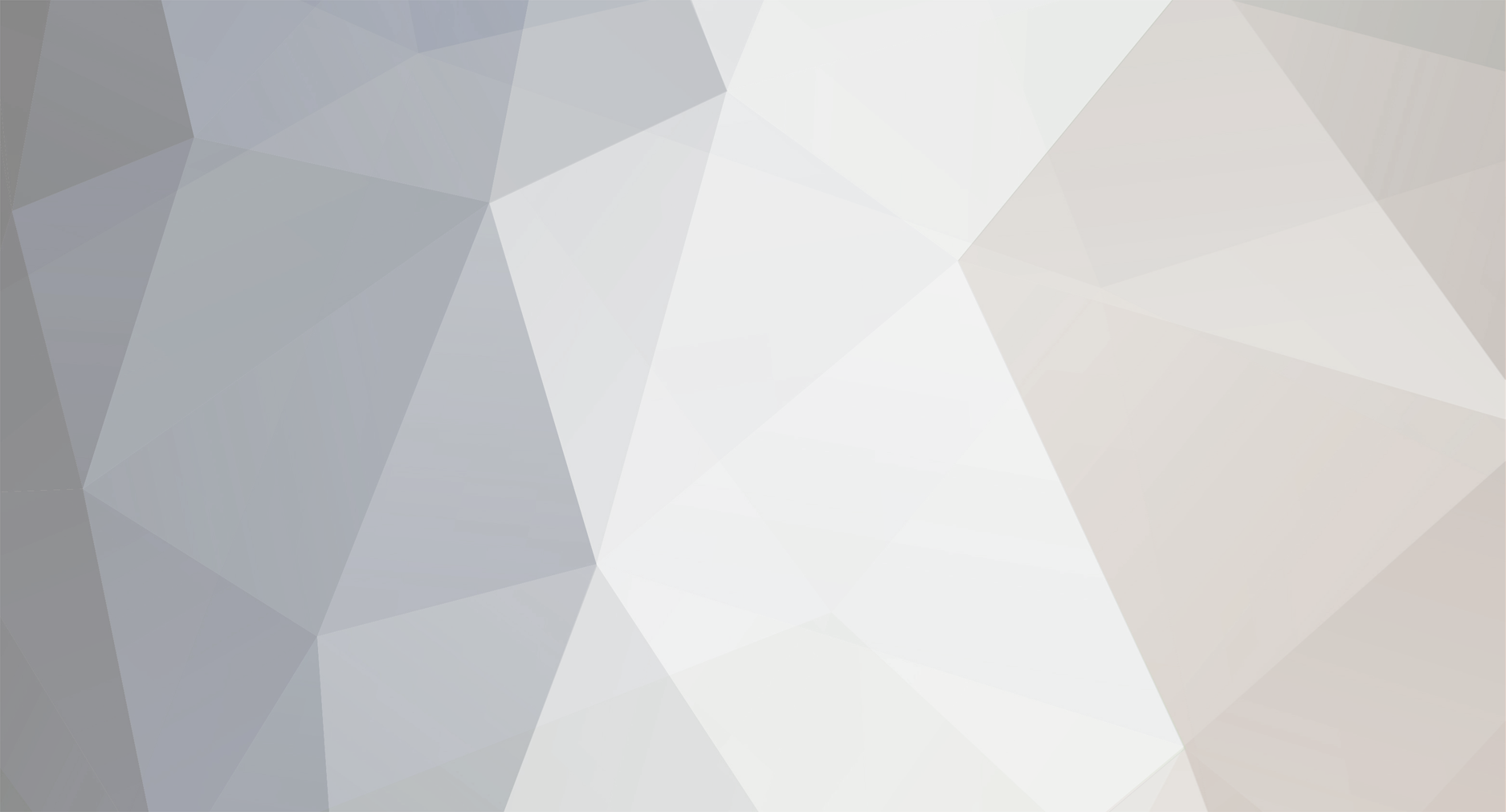
p2grace
-
Posts
1,024 -
Joined
-
Last visited
Never
Posts posted by p2grace
-
-
The errors are generated because the variables aren't set when you're trying to determine what they're values are.
Is this a public page that we can test?
-
There isn't a syntax issue... display php errors and see what you learn.
-
What is the code at those lines?
-
Try this:
setcookie('SITE_remember_me', '1', (time()+31536000),'/');
<?php session_start(); if(isset($_COOKIE['SITE_remember_me'] && $_COOKIE['SITE_remember_me']=='1'){ setcookie('logged_in', 1, 7200 +time()); $_SESSION['logged_in'] = '1'; $_SESSION['first_name'] = $_COOKIE['first_name']; } ?>
I added a folder path to the cookie.
You should put your site name in the front of your cookie so you don't have any conflict with other website cookies.
Let's start there, if it still doesn't work. Display php errors and we can go from there.
-
It's definitely possible.
$random_row = mysql_fetch_row(mysql_query("SELECT * FROM YOUR_TABLE ORDER BY RAND() LIMIT 1"));
-
Example of using if statement:
if($mean > $medium){ echo "mean is greater than medium"; }else{ echo "mean is not greater than medium"; }
-
Something like this should do the trick.
<?php session_start(); include("checklogin.php"); $username = $_SESSION['myusername']; $query = mysql_query("SELECT * FROM members WHERE username='$username'"); if (mysql_num_rows($query)==0){ die("User not found!"); }else{ $row = mysql_fetch_assoc($query); $location = $row['imagelocation']; if($location == ""){ echo "<img src ='/profileimages/box.png' width='225' height='275'>"; }else{ echo "<img src ='$location' width='225' height='275'>"; } } ?>
-
Heredoc is a much easier way to do this.
<?php echo <<<HTML <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link href="jjrq.css" rel="stylesheet" type="text/css" /> </head> <body> <form method="post" name="table" id="table"> <select name="select" id="select"> <option value="one">1</option> <option value="two">2</option> <option value="three">3</option> </select> </form> </body> </html> HTML; ?>
No need to escape quotes.
-
Glad it was a simple fix
Could you mark the topic as solved?
-
Hi imimin, it's good to see you're still making your way through some typical coding challenges
If you look at the html surrounding your variable within your heredoc statement, you closed your <td> and then tried adding content after it (through the db variable). You need to put the closing </td> after the db variable. Try the following:
echo <<<HTML <input type='hidden' name='name' value='$prod_name' /> <input type='hidden' name='price' value='$price' /> <input type='hidden' name='sh' value='$weight' /> <input type='hidden' name='img' value='https://secure.impact-impressions.com/poj/includes/img_resize3.php?src=$sitelocation$img&width=100&height=100&qua=50' /> <input type='hidden' name='img2' value='' /> <input type='hidden' name='return' value='http://www.patternsofjoy.com/order_more.php' /> <input type='RADIO' name='custom20' value='Style: $selected_style' checked='checked' style='display: none' /> <strong>Garment Style: </strong>$selected_style <br /> <a href="http://www.patternsofjoy.com/order_more.php" TITLE="Click here to change Garement Style">***Change Garment Style***</a> </TD> </TR> <TR> <TD> <B>Size Range:</B><BR> <INPUT TYPE="RADIO" NAME=custom30 VALUE="Size Range: Adult +$2.00"> Adult (Add $2.00) <BR> <INPUT TYPE="RADIO" NAME=custom30 VALUE="Size Range: Child"> Child<BR> *****NEW TABLE HERE VIA DB($adult_size_chart)***** </td> </tr> <tr> <td> <strong>Garment Size:</strong> <select id="custom40" name="custom40" size='5'> <option value="Size: Small (S)">Small (S)</option> <option value="Size: Medium (M)">Medium (M)</option> <option value="Size: Large (L)">Large (L)</option> <option value="Size: Extra Large (L)">Extra Large (L)</option> <option value="Size: Toddler (T)">Toddler (T)</option> </select><br /> <a href="javascript:newwindow2();" >Garment Measuring Tips</a> </td> </tr> HTML;
-
Variables need to be within double quotes.
header("Location: index.php?page=message&forum=$forum&id=$forumpostid&pagenum=last");
-
Two ways:
Method 1:
SELECT * FROM `news` `n`, `news_comments` `c` WHERE `n`.`newsID` = `c`.`newsID`
Method 2:
SELECT * FROM `news` AS `n` INNER JOIN `news_comments` AS `c` USING ( `newsID` )
-
If you're using sendmail, multiple recipients need to be separated by a comma not a semicolon. What are you using as a delimiter?
-
Can you echo out the original $sku_init, and then the $sku results and post them. Your code should work.
-
You need quotes around the array key.
replace=array(","); $sku_init=$row_itm['itm_sku']; $sku=str_replace($replace," ",$sku_init);
-
It doesn't appear because of the <input type="text" value="valuewith"" /> (notice the double quotes). One method would be to use str_replace() to replace aprostrophe's and leave the escaped quotations. There's probably a better way to do it but that's the first idea that popped in my head.
-
Query the db using that id, then use mysql_num_rows() to determine if there were any results. If there was display the output, if there isn't produce an error.
-
-
Do you have a url we can look at?
-
That typically happens when the original quote or apostrophe was copied out of Microsoft Word, and they are not actual quotes or apostrophe's, but rather Microsoft symbols that look similar. This method is used so the quotes and apostrophes resemble the corresponding font in Microsoft applications, however it is also the reason all text should be copied into a text editor (to convert the Microsoft symbols) before being entered into the database.
-
Is there supposed to be an equal number of values in each array? If so you could just do an array_shuffle to each and then one for loop to get the value of each key.
-
I don't see where that file is included.
-
That was the opening to the heredoc, each heredoc needs to be closed as well.
opening: <<<HTML
closing: HTML;
-
Yeah... the logic to calculate the price cannot be contained within heredoc. So I ended the first heredoc on line 155 with the HTML;. Then the $price is calculated, and a new heredoc is started, ending at line 205.
I'd recommend reading over the examples of heredoc at the url below.
http://us.php.net/manual/en/language.types.string.php#language.types.string.syntax.heredoc
whats wrong with this code it shows a blank page only
in PHP Coding Help
Posted
There are few logic issues here:
You're calling $res outside of the function scope, so the variable doesn't actually exist.
You're $html variable isn't being used.
You're trying to print an array where I assume you actually want to echo html.