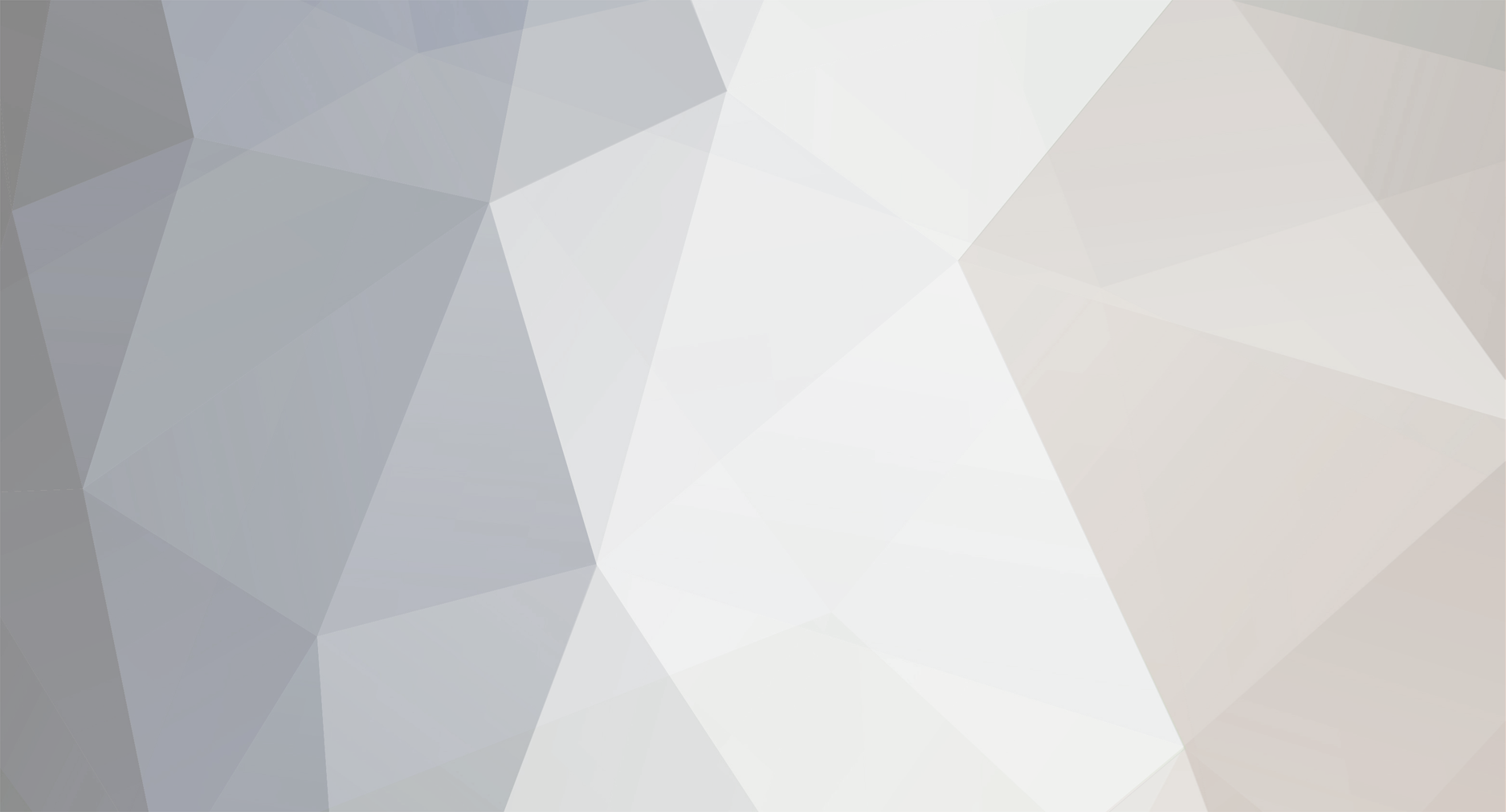
moon 111
Members-
Posts
198 -
Joined
-
Last visited
Never
Everything posted by moon 111
-
I'm working on a shoutbox using OOP but my addMessage function isn't working. Also, can you tell me if this is the correct way of writing it? I'm rather new to OOP and I'm not sure whats what. Would there be a better/easier way? Thanks class_lib.php <?php class shoutbox { public $file = 'shoutbox.txt'; public function __construct() { } public function parseFile() { $tempfile = file($this->file); foreach($tempfile as $value) { $temp = explode(':-:', $value); $file[] = array($temp[0], $temp[1], $temp[2]); } return $file; } public function displayShoutBox() { $file = $this->parseFile(); foreach($file as $value) { $date[] = $value[0]; $name[] = $value[1]; $message[] = $value[2]; } $content .= "<table border='0' width='500' cellspacing='0' cellpadding='5' bgcolor='#FFFFFF'>"; $i = 0; foreach($name as $value) { $content .= "<tr><td>" . $date[$i] . "<td><td><b>" . $name[$i] . ":</b> " . $message[$i] . "</td></tr>"; $i++; } $content .= "</table>"; return $content; } public function addMessage($name, $message) { $handle = fopen($this->file, "rw"); if(filesize($this->file) > 0) $content = fread($handle, filesize($this->file)); else $content = ""; $date = date("H:i:s"); $content = $date . ":-:" . $name . ":-:" . $message . "\r\n" . $content; fwrite($handle, $content); } } ?> shoutbox.php (main) <?php include_once('class_lib.php'); $shoutbox = new shoutbox; $shoutbox->addMessage("Moshe Teutsch", "Testing..."); echo $shoutbox->displayShoutBox(); ?> The text file has the following format: 10:19:50:-:Moshe Teutsch:-:Testing... 10:26:26:-:Moshe Teutsch:-:Test number two... etc...
-
I would definitely use a cron job. The whole point of a cron job is running code repeatedly. If hes running one long script why would he need the cron job? He only needs to run it once!
-
For some reason the following code isn't working. Any ideas? ($file is defined) <?php function addMessage($name, $message) { $handle = fopen($file, "rw"); if(filesize($file) > 0) $contents = fread($handle, filesize($file)); else $content = ""; $date = date("H:i:s"); $content = $date . ":-:" . $name . ":-:" . $message . "\r\n" . $content; fwrite($handle, $content); } ?> EDIT: found the mistake with $contents/$content but its still not working.
-
I have a multidimensional array. It is organized like so: $file = array(array("title1", "message1"), array("title2", "message2")); etc... I'm trying to move the "title" part and the "message" part into two different arrays so that I have $title = array("title1", "title2"); $message = array("message1", "message2"); Would this work? foreach($file as $value) { $title[] = $value[0]; $message[] = $value[1]; }
-
It is sorting the information character by character. 1-10 1-11 1-12 1-1 1-2 1-3 You need to sort everything after the dash though I'm not sure how to do that...
-
I'll be more specific: I have a table 'site' that has information such as id, title, url etc. I have another table 'rating' which has the cols sid, uid, rating. sid reffers to the site that was rated. How can I order the information in the table 'site' by the average rating of all the rows where the sid (in rating) equals id (in site)?
-
Would something like this work? (there is also a row rating.) SELECT * FROM site AS s, rating AS r WHERE s.id = r.sid ORDER BY AVG(r.rating) DESC
-
LIMIT must be at the end of the query: $sql = mysql_query("SELECT * FROM tblNews ORDER BY NewsDateAdded DESC LIMIT ".$limits.",".$max) or die(mysql_error());
-
There is probably a much better way of doing this but here is my code: <?php $txtfile = 'file.txt'; $file1 = "file1.swf"; $file2 = "file2.swf"; $handle = fopen($txtfile, 'rw'); if(filesize($txtfile) > 0) $contents = fread($handle, filesize($txtfile)); else $contents = ""; if(!empty($contents)) { $date = explode(',', $contents); if(date('D') == "Mon") { if($date[0] != date('d')) { if($date[1] == $file1) $file = $file2; else $file = $file1; fwrite($handle, date('d') . ',' . $file); } } } else { $file = $file1; fwrite($handle, date('d') . ',' . $file); } echo $file; // Display the swf's name. Change this to whatever. ?> You need an empty file 'file.txt' (you can change the name in the code).
-
Oh, sorry I fixed the code. The "code" tags
-
SQL injection has nothing to do with POST or GET. What it means is that if you have somecode like: <?php mysql_query("SELECT * FROM users WHERE username = '".$_POST['username']."'". " AND password = '" . $_POST['password']."'"); ?> Someone can insert into username and password something like ' or 1=1-- which would turn the query into: <?php mysql_query("SELECT * FROM users WHERE username = '' OR 1=1--'". " AND password = '' OR 1=1--'"); ?> which will probably log him in as the first user.
-
If the file is .png it gives me one of those squares with red symbol (what you get when you can't display the image). Read the code again - I edited it.
-
I have this function: [code] <?php function watermarkImage ($SourceFile, $WaterMarkText, $DestinationFile, $x, $y, $font_size) { if(stripos($SourceFile, ".png")) { $ext = ".png"; } elseif(stripos($SourceFile, ".jpg") OR stripos($SourceFile, ".jpeg")) { $ext = ".jpg"; } list($width, $height) = getimagesize($SourceFile); $image_p = imagecreatetruecolor($width, $height); if($ext == ".png") $image = imagecreatefrompng($SourceFile); elseif($ext == ".jpg") $image = imagecreatefromjpeg($SourceFile); imagecopyresampled($image_p, $image, 0, 0, 0, 0, $width, $height, $width, $height); $black = imagecolorallocate($image_p, 0, 0, 0); $font = 'arial.ttf'; imagettftext($image_p, $font_size, 0, $x, $y, $black, $font, $WaterMarkText); if ($DestinationFile<>'') { if($ext == ".png") imagepng ($image_p, $DestinationFile, 100); elseif($ext == ".jpg") imagejpeg ($image_p, $DestinationFile, 100); } else { if($ext == ".png") { header('Content-Type: image/png'); imagepng($image_p, null, 100); } elseif($ext == ".jpg") { header('Content-Type: image/jpeg'); imagejpeg($image_p, null, 100); } } imagedestroy($image); imagedestroy($image_p); } ?> But it only works on .jpegs. Want I want to do is make it work with pngs too. I tried this but it didn't work: <?php function watermarkImage ($SourceFile, $WaterMarkText, $DestinationFile, $x, $y, $font_size) { if(stripos($SourceFile, ".png")) { $ext = ".png"; } elseif(stripos($SourceFile, ".jpg") OR stripos($SourceFile, ".jpeg")) { $ext = ".jpg"; } list($width, $height) = getimagesize($SourceFile); $image_p = imagecreatetruecolor($width, $height); if($ext == ".png") $image = imagecreatefrompng($SourceFile); elseif($ext == ".jpg") $image = imagecreatefromjpeg($SourceFile); imagecopyresampled($image_p, $image, 0, 0, 0, 0, $width, $height, $width, $height); $black = imagecolorallocate($image_p, 0, 0, 0); $font = 'arial.ttf'; imagettftext($image_p, $font_size, 0, $x, $y, $black, $font, $WaterMarkText); if ($DestinationFile<>'') { if($ext == ".png") imagepng ($image_p, $DestinationFile, 100); elseif($ext == ".jpg") imagejpeg ($image_p, $DestinationFile, 100); } else { if($ext == ".png") { header('Content-Type: image/png'); imagepng($image_p, null, 100); } elseif($ext == ".jpg") { header('Content-Type: image/jpeg'); imagejpeg($image_p, null, 100); } } imagedestroy($image); imagedestroy($image_p); } ?> Help please[/code]
-
I have a table. One of the fields is title. I want to sort it by title in LOWERCASE becasue some are in lower case and others in uppercase and the lower case ones are gettting listed on the top when I sort them. This is my code now: SELECT * FROM site ORDER BY title ASC Could I write something like this? SELECT * FROM site ORDER BY LOWER(title) ASC thanks
-
This doen't work either: SELECT * FROM site, rating WHERE site.id = rating.sid ORDER BY AVG(rating.rating) DESC And yes I understood. There is a field rating in the table rating.
-
Would this work: SELECT * FROM site, rating WHERE site.id = rating.sid ORDER BY AVG(rating) DESC
-
I have a table sites that has the cols id, title, description, uid. I also have a table rating with the rows sid, uid, rating. sid reffers to the site. Now I want to sort 'sites' by the average of all of the ratings reffering to that site. This is a want I would do if I could (obviously not legal: SELECT * FROM sites ORDER BY AVG(SELECT rating FROM rating WHERE sid = '".$sid."') DESC . Clear?
-
I kind've need the help quickly....
-
No, I need to order the table 'site' by the row 'rating' in the table 'rating'
-
Well if all you want is something like that (I assume 20kg costs 20 pounds by Air etc.) you can just have an array like this: <?php $costs = array("Air" => 1, "Fedex" => 0.5); $kg = 10; $method = "Air"; echo "It costs $" . $kg * $costs[$method] . " to ship " . $kg . "kg by " . $method; ?>
-
If I understand you correctly, this should work: <?php // I assume you have two rows: method (air, fedex etc.) and cost (1:2.00,2:4.00 etc.) // I also assume you have already connect to the database. $method = "air"; $q = "SELECT * FROM shipping WHERE method ='".$method."'"; $r = mysql_query($q); $list = mysql_fetch_array($r); $arr = explode(",", $list); foreach($arr as $value) { $arr2 = explode(":", $value); echo $arr[0] . "kg costs $" . $arr2[1] . " to ship by " . $method . "<br>"; } ?>
-
I have two relevent tables: sites and rating. Rating has these rows: sid (site id), uid (userid) and rating. When someone votes on a website it adds to rating the sid of the site their uid and their rating. What I need to do is sort the site table by rating. How can I do that?
-
No problem. Enjoy!
-
Heres something that I wrote: <?php $q = "SELECT * FROM site"; $r = mysql_query($q); $num = ceil(mysql_num_rows($r) / $limit2); if($num != 1) { for($i = 1; $i <= $num; $i++) { if($page / $limit2 != $i) echo "[<a href='index.php?p=list&page=".$i."'>".$i."</a>] "; else echo "[<b>".$i."</b>] "; } } ?> $limit2 is the number of page you want to display per page and $page is the current page. Basicly it takes the number of pages that its displaying and deviding them into pages ($num = ceil...). Then it checks if there is more then one page (no need for pagnation with 1) and loops thru all of the pages echoing them