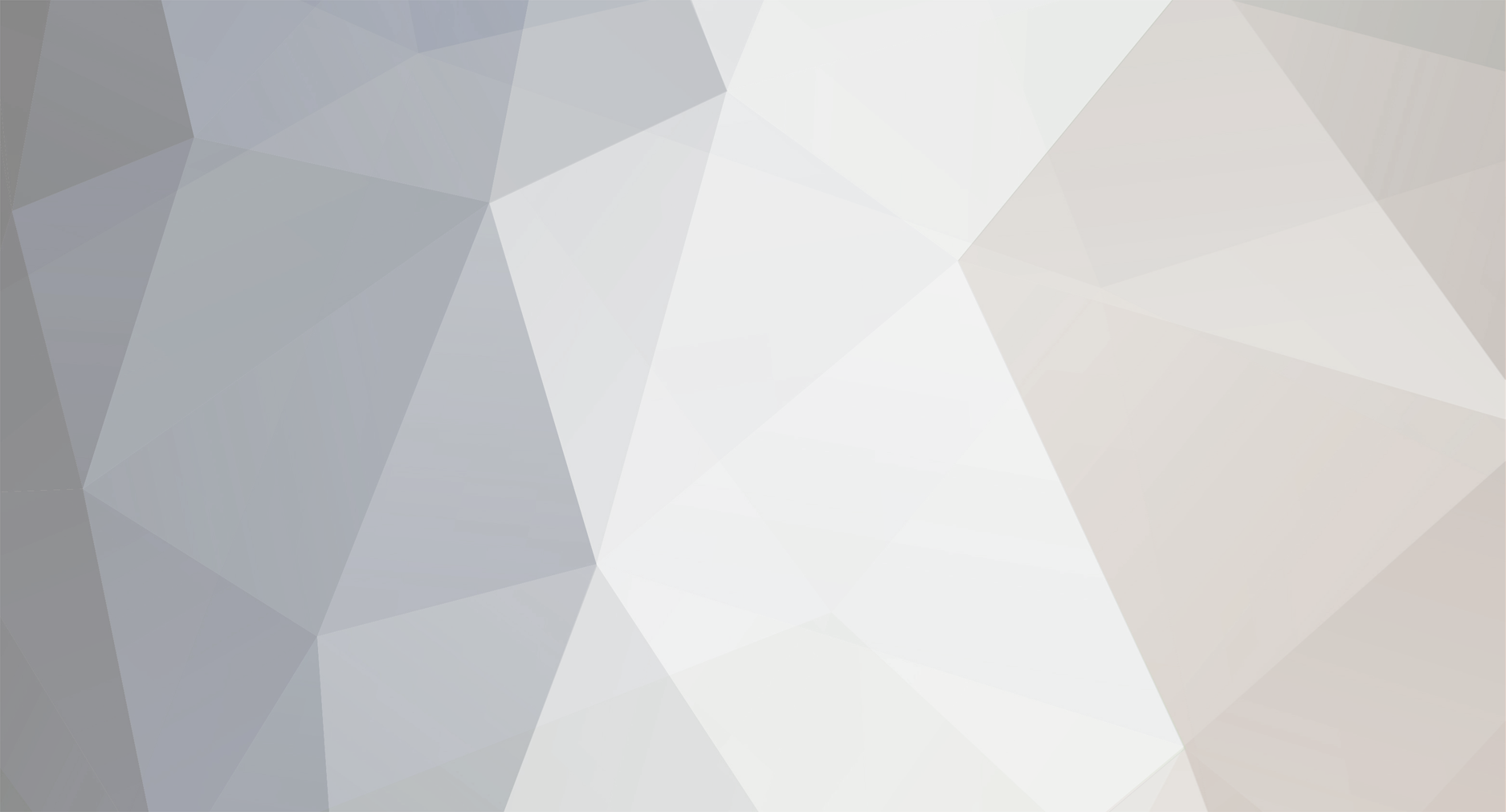
awpti
Members-
Posts
459 -
Joined
-
Last visited
Never
Everything posted by awpti
-
[SOLVED] wich encryption should I use for my passwords?
awpti replied to Yeodan's topic in PHP Coding Help
Easiest method is md5(). It's a one-way hash and is secure enough for most purposes. If you want true security, wrap the password in a 128 char "key", eg; keyprefix_password_keypostfix This makes your md5()'d password damned near unbreakable. Caveat; if you lose either key, all users will have to reset their passwords. -
So, here's the sample code: It assumes no string is longer than 3 characters for the ord() function at the end. <form method="post" action="test.php"> <textarea name="test"></textarea><br /> <input type="submit" name="testing" value="what the f!" /> <?php $res = explode("\n", $_POST['test']); foreach($res as $val) { $fix[] = str_replace("\n", '', $val); } echo '<pre>'; var_dump($fix); echo '</pre><br />'; echo 'Ordinal Value of last char: '.ord($fix[4]{3}); Weird thing? You'll notice that the var_dump() shows each array entry as having a newline character except for the last one. If you echo the ordinal value of that extraneous character, it comes back as: 0 (0 == NULL) My question: What the HELL? Why is this kicking back as a newline character when ord() clearly shows it's null? It shouldn't even display, let alone have an ordinal value.
-
Close. If you have more than one table in the 'busfleet' database, you need to add a WHERE clause to it. ex; SELECT TABLE STATUS FROM 'database' WHERE Name = 'my_table_name'; That will only return status from one table rather than multiple. Otherwise, that should work fine.
-
Try to add a little more detail. Error logs, etc.
-
Here's a nice little form handler I wrote some time ago. It can be used with either _GET or _POST. You can set the REDIRECT and SUBJECT in your Form or just set it at the top of the script here. This form handler sends the message to a single address and that's it - set in the script. <?php // $mail_to and $mail_from must be set. $mail_to = 'to.someone@someplace.com'; // Who is the E-Mail going to? ex: me@mydomain.com $mail_from = 'where@i.am.from.com'; // Where does the E-Mail appear to be from? ex; webform@mydomain.com // OPTIONAL SETTING $redirect_url = ''; // Example: http://domain.com/thankyou.html - must be a FULL URL. ############################ # DO NOT EDIT BELOW THIS # ############################ // Fail if _POST and _GET are empty. Nothing to process. if(count($_POST) == 0 AND count($_GET) == 0): echo 'This form handler does nothing if visited directly. You must submit form data to this script.'; exit; endif; // Fail if $mail_to or $mail_from are not set. if(empty($mail_to) OR empty($mail_from)): echo 'You must edit this script and set the appropriate values for $mail_to and $mail_from.'; exit; endif; // Set $fields to whichever method is being used. $fields = (count($_POST) > 0 ) ? $_POST : $_GET; $message_body = "Form Submission \n\n"; foreach ($fields as $field => $value): switch(strtolower($field)): case 'redirect': $redirect = $value; break; case 'subject': $subject = $value; break; endswitch; if (strtolower($field) != 'redirect' AND strtolower($field) != 'submit' AND strtolower($field) != 'subject'): $message_body .= strtoupper($field) . ": {$value}\r\n"; endif; endforeach; // Set the redirect URL from the form (if set). $host_url is a default action if $redirect isn't set $redirect = (empty($redirect_url)) ? $redirect : $redirect_url; $host_url = $_SERVER['HTTP_HOST']; // Set the message subject based upon a subject field being set or not. $message_subject = (!empty($subject)) ? $subject : 'Message from '.$_SERVER['HTTP_HOST']; $headers = "From: {$mail_from}\r\n Reply-To: {$mail_from}\r\n X-Mailer: PHP/" . phpversion(); if (!mail($mail_to, $message_subject, $message_body, $headers)): echo 'Messge Send Failed.'; endif; if(empty($redirect)): header("Location: http://{$host_url}"); else: header("Location: {$redirect}"); endif;
-
[SOLVED] PHP MySQL Statement not being executed with no feedback
awpti replied to ialsoagree's topic in PHP Coding Help
That would imply one off two things, one of which has been ruled out: 1.) Mal-formed statement (Ruled out) 2.) Uncaught exception. make use of mysql_error(). That's where your error is going to hide. If there are no errors, go back to #1. -
You start with the most common development method: Documentation. Here's my method: Document what you want the social network to provide. Document the basic "path" of features (visual side - just the basics) example: User Registration We collect the following information: Example Info 1 Example Info 2 User clicks on Register and the following happens: Entry is added to Database Validation E-Mail is sent I'm quite verbose in my documentation, but it gives me real direction vs. just jumping into the mix in full-retard mode. Back-adding features will give you a headache, not counting all the potential forced complete dumps and rewrites because your idea/methodology suddenly doesn't work out for a new feature without sacrificing performance or security. Most people start with a framework-design once they have the general idea down and then start pushing code around (Concept->Development of Concept->Concept Validation->Production Development->Production Deployment)
-
Please, keep an eye on the "Last Updated:" part Last Update: Aug 28 2003 This project has been abandoned. Don't use abandonware - it'll get you nowhere and is likely outdated and outclassed by other software available.
-
[SOLVED] My Code Won't UPDATE the Database -- NEEDED ASAP
awpti replied to skiingguru1611's topic in PHP Coding Help
Just a little commentary: Don't ever suppress error messages using @. Either write an exception handler or disable the display of errors. The @ slows down the speed of code parsing/lexing in the engine. Plus, it's just bad practice. -
I'm assuming it's an HTML Email.. Wrap it up in tables and let the tables sort it out.
-
PHP is a parsed language. Trying to 'include' a PHP file from another site will get you the final resultant output of that script, not the content of said script. Yes, you can pull DB content from one server to your own - provided you have the username/pass stored locally or in a flat format file you have access to.
-
[SOLVED] how can i make this if else statement work?
awpti replied to shadiadiph's topic in PHP Coding Help
You've got some pretty obvious typos/syntax errors here. I'll assume you copied/pasted this as-is. We can't magically determine which line is really 168, so why not point that one out for us? -
[SOLVED] another question for everyone who knows what there doing
awpti replied to Reaper0167's topic in PHP Coding Help
Yup, just have to use PHP to echo it out first. PHP is server-side, JS is clientside. You can use PHP to spit out javascript which should then be executed by the browser. -
Selecting only specified 'entries' in a database with images?
awpti replied to cs.punk's topic in PHP Coding Help
SELECT * FROM some_table WHERE id IN(12345,213123,3412,4512,534647). As far as attaching images to users? Data normalization. Link the image to the user ID. eg; CREATE TABLE my_images ( `id` int not null primary key auto_increment, `user_id` int, #references users.id `image_name` varchar(128), ); -
That's the Smarty Template file and does no work on any files. You will need to modify the code behind the file-handler to resize files. It's perfectly possible to do, but not if you don't know PHP fairly well. You could always ask the original developers where file uploads are handled and make your modifications there.
-
So, what is the content of $data['date']? It's obviously not what it expects.
-
Why not just specify the engine time in the CREATE TABLE line? CREATE TABLE blah ( .... .... .... ) ENGINE=INNODB;
-
Address this in their forums.
-
I would highly suggest making use of the PHPMailer class to handle this. Also, you don't need to quote variables ("$to_email").. get rid of 'em. http://sourceforge.net/projects/phpmailer
-
This has nothing to do with PHP and everything to do with CSS.
-
[SOLVED] Folder view works but can the output be formatted?
awpti replied to toxictoad's topic in PHP Coding Help
Replace the \n with <br /> or just add a <br /> after or before the \n -
Don't forget to validate that. You can do that easily, since you're pulling data by an integer.. <?php if(ctype_digit($_GET['member_id'])): // do something else: // Fail - it's not an integer value! endif; Addendum: You don't need a while loop if you're only fetching 1 row of data.
-
Parse error, unexpected T_STRING, expecting ',' or ';'
awpti replied to LostAngel's topic in PHP Coding Help
If that paste is correct, your spacing is all sorts of screwed up. And that doublequote is in the wrong place. Where's your closing PHP tag? -
RewriteRule /member/(.*)$ member-profile.php?member=$1 Roughly.. my syntax is probably off. Only use (.*) if you have no character limitations. If all users are purely alphanumeric and you want to (and should) enforce that.. use this: RewriteRule /member/([a-zA-Z0-9])$ member-profile.php?member=$1
-
Use code tags and tab that beast out.