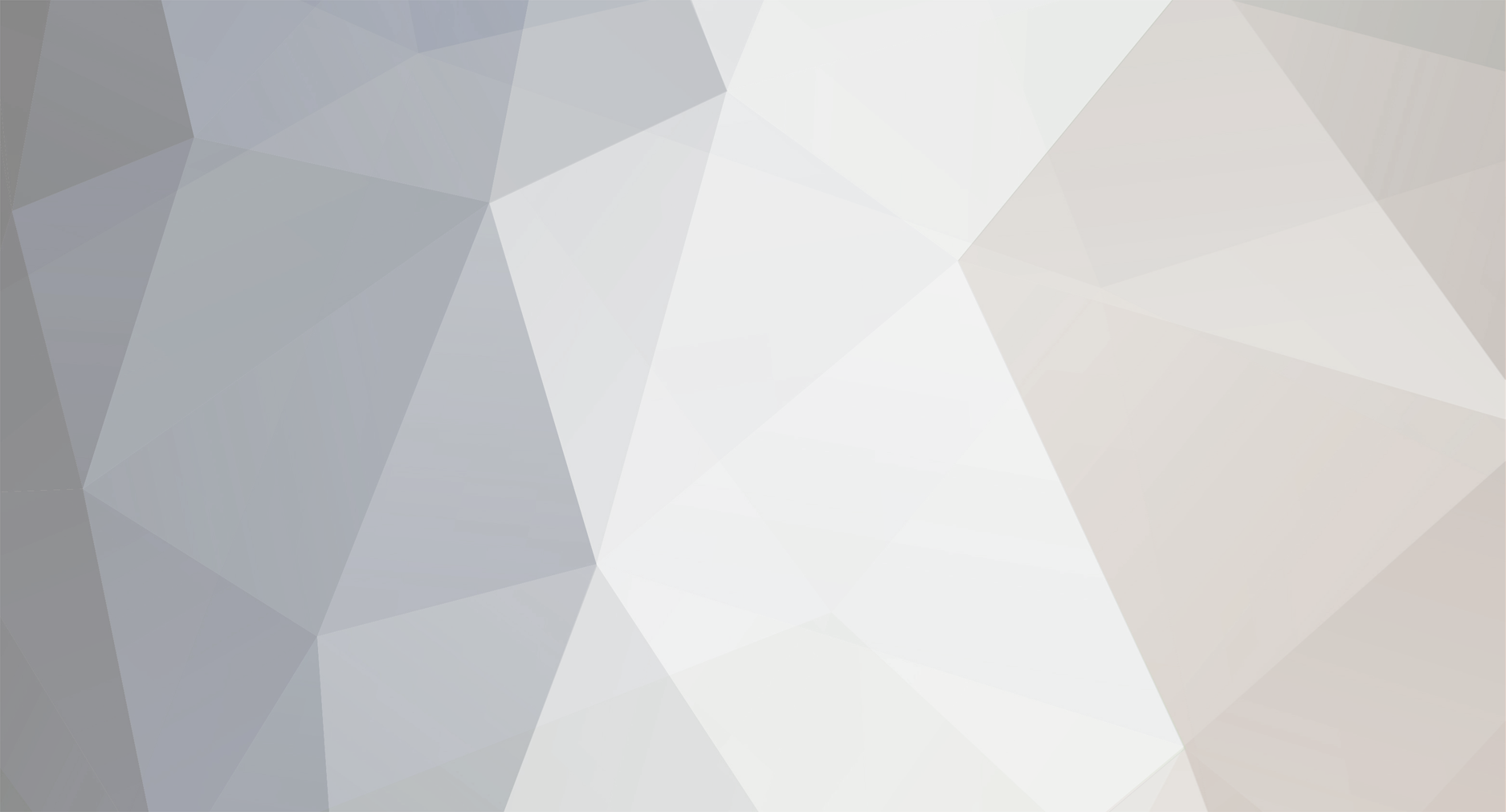
awpti
Members-
Posts
459 -
Joined
-
Last visited
Never
Everything posted by awpti
-
Try these: ini_set('memory_limit', '512M'); Or in your .htaccess: php_value memory_limit 512M Otherwise there's not much you can do short of partial reads/writes until you've looped through it.
-
This is the syntax I use for arrays: <?php $my_array = array ( 'some_key' => 'some_value', 'another_key' => 'another_value' ); Can't miss the closing parenthesis if you do it that way.
-
Of course you can run multiple language sites in a single server, just not as a single file as was suggested in your original post (from my interpretation). File is just read based on it's file extension or AddType Better to just refactor the entire mess to avoid that (creates unnecessary overhead).
-
[SOLVED] make gold decrease when people buy wood
awpti replied to berry05's topic in PHP Coding Help
He wants us to write it for him. -
Technically, yes. Realistically, no. That would also be completely retarded. Not even going to sugar-coat that statement. EDIT: I should say: Technically, NO. No current webserver can instantiate two parsing engines at once. But, if you were to develop a solution, yes. And it would still be retarded. It would be full retard.
-
Here's a start for you, so you understand indenting (assuming you don't, for obvious reasons): <?php $crew_check =mysql_query("SELECT * FROM crews"); while($k = mysql_fetch_object($crew_check)){ $user=mysql_fetch_object(mysql_query("SELECT * FROM users WHERE username='$k->owner'")); $underboss=mysql_fetch_object(mysql_query("SELECT * FROM users WHERE username='$k->underboss'")); $rhm=mysql_fetch_object(mysql_query("SELECT * FROM users WHERE username='$k->rhm'")); $lhm=mysql_fetch_object(mysql_query("SELECT * FROM users WHERE username='$k->lhm'")); if ($user->status == "Dead" || $user->status == "Banned"){ if ($k->underboss != "0" && $underboss->status == "Alive"){ mysql_query("UPDATE crews SET owner='$k->underboss', underboss='0' WHERE name='$k->name'"); if ($underboss->status == "Dead" || $underboss->status == "Banned"){ if ($k->rhm != "0" && $rhm->status == "Alive"){ mysql_query("UPDATE crews SET underboss='$k->rhm', rhm='0' WHERE name='$k->name'"); if ($rhm->status == "Dead" || $rhm->status == "Banned"){ if ($k->lhm != "0" && $lhm->status == "Alive"){ mysql_query("UPDATE crews SET rhm='$k->lhm', lhm='0' WHERE name='$k->name'"); } elseif ($k->lhm == "0" || $lhm->status == "Dead" || $lhm->status == "Banned"){ mysql_query("UPDATE `users` SET `crew`='0' WHERE `crew`='$k->name'"); mysql_query("DELETE FROM crews WHERE name='$k->name'"); } } } ?> I think we can begin to see what a horrendous mess this application is. This beast needs some SERIOUS refactoring. Yikes. I'm not going to tab anything else out for you. Good luck.
-
You're beyond help at this point, Petchy. Tab out your code and you will find the missing braces.
-
Nothing wrong with that code. Problem is with your query.
-
Polly want a cracker?
-
Let me put it this way: cat junk.php | grep '{' | wc -l 85 cat junk.php | grep '}' | wc -l 76 You are missing 9 closing brackets. And they make fun of me for using alternative syntax. HAH!
-
Custom CSS files (username+random chars? or name it the same as the subdomain). The less you have in your database, the more performance you'll be able to squeeze out of it. The file system is always faster than a database (for, hopefully, obvious reasons) for storing relatively flat content. Your idea will work fine - it's how WordPressMU works (though less than elegantly). I've never seen online articles discussing this specifically, but look into a framework like CodeIgniter, Kohana or Cake to reduce development time and give you more time to sit back and consider viable options.
-
Yup, again.. Go to their forums and ask how to integrate. They probably have documentation on custom integration. If not, their users can help you. This really isn't the right place for it (even though PHPFreaks has a Third-Party software forum)
-
Tab that garbage out. Indentation will lead to enlightenment.
-
Oldish internet meme. Look it up. Specifically: I accidentally the economy (G.W. Bush actually said this in a speech due to his lack of pacing)
-
I can only tell you that ZF makes my eyes melt and brain explode. On a very random basis. I can't even determine from your code why it would throw the errors your seeing, let alone why it was previously kicking back an empty array. That's weird. It LOOKS fine..
-
This is generally done using regular expressions to look at the content and match valid URLs. This tends to be slow compared to using tags since ereg/preg functions are, by their very nature, slow. Better to keep with the tagging system and start making use of, say, FCKEditor or TinyMCE to make posting easier.
-
Absolutely. I'd recommend going to the vBulletin Support Forums for information on how to do this.
-
FTP is a protocal. Doesn't matter if you connect via IP or some random hostname as long as that hostname reverses to the same IP that the actual FTP Service lives on. ex: geeklan.com www.geeklan.com ftp.geeklan.com All reverse to: 208.109.240.109 I can connect via FTP to any of those addresses. As far as other programs timing out.. sounds like an issue with the application. By the way.. wrong forum.
-
I had to do something like this a long time ago (years): <?php $quotes = file('quotes.txt'); $rand = rand(0, count($quotes) -1); echo $quotes[$rand]; quotes.txt: This is a quote! -Cite This is another quote! -Cite This is yet another quote! -Cite Wow! More quotes! -Cite Obviously, the less you have as far as number of quotes, the less random it's going to be. If you are belching out a number of quotes and they must be unique, you'll need to keep track of keys that have been echo'd and make sure they aren't pushed out again.
-
<?php include 'config.php'; mysql_select_db("exembar_site")or die("Cannot find specified Database!"); $user = mysql_real_escape_string($_POST['user']); $pass = mysql_real_escape_string($_POST['pass']); $result = mysql_query("SELECT * FROM `staff` WHERE `username`='{$user}' AND `password`='{$pass}'"); $count = mysql_num_rows($result); if($count == 1): echo 'Login Successful'; else: echo 'Login failed!'; endif; //commented out below and fixed syntax/logic. /* if($count == 1): //Since you only expect one result, a while() loop is not necessary) $info = mysql_fetch_assoc($result); session_start(); $_SESSION['id'] = $info['id']; $_SESSION['user'] = $info['user']; header("location:http://team.exembarstudios.exofire.net"); else: session_start(); $_SESSION['error'] = "Invalid Username or Password"; header("location:http://team.exembarstudios.exofire.net/login.php"); endif; */ ?> You have a lot of needless repetition in your code. I've fixed the logic a bit. If it spits out "Login Successful!", then it's a problem with your index page on that domain. Alternative PHP Syntax For the WIN!
-
Utilize FTP functions built in to PHP. It'll look messy, that's about all I can say.
-
Ouch, that hurts my head. Here's one I wrote up: <?php class awpnav { function build_links($input_menu, $css_id, $css_id_ns, $uri) { if(!is_array($input_menu)) { return FALSE; } else { foreach($input_menu as $key => $value) { $class = ''; if($uri == str_replace('/', '', $key)) { $class = 'id="'.$css_id.'"'; } elseif($css_id_ns !== '') { $class = 'id="'.$css_id_ns.'"'; } $menu_final[] = '<li '.$class.'><a href="'.$key.'">'.$value.'</a></li>'; } } //Self-explanitory class. Returns a simple array or FALSE return $menu_final; } } Here's how you use it: <?php $menu = array ( '/' => 'Home', '/hosting' => 'Web Hosting', '/services' => 'Services', '/about' => 'About' ); $uri = ....; // Code to figure out the KEY of the array $css_id = 'current'; $css_id_ns = ''; //unselected item has no CSS ID $nav = new awpnav; $result = $nav->build_links($menu, $css_id, $css_id_ns, $uri); Essentially: build_links(array $menu, string $css_id, string $css_id_ns, string $uri); It figures out which menu option to highlight based on the array key. The value of the key is what I actually pass to the page title before rendering the view (content). This needs to be manually edited each time you add a new menu item, but you don't have to add 5-6 lines of HTML+some code. You can see it working here: http://awpti.org/