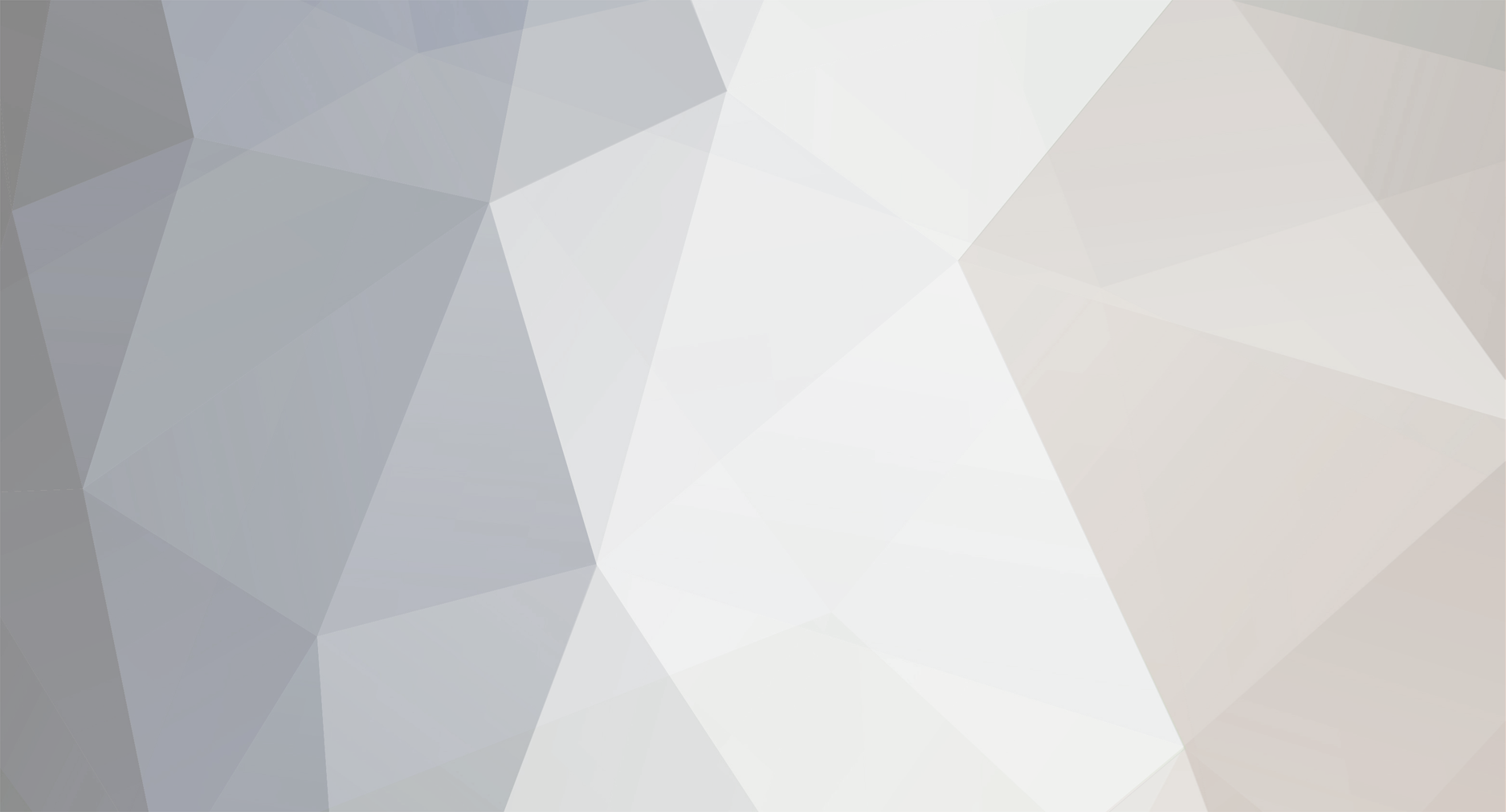
rhodesa
Staff Alumni-
Posts
5,253 -
Joined
-
Last visited
Everything posted by rhodesa
-
Try getting rid of the following line:
-
[SOLVED] can't get $_SERVER[’PHP_SELF’] to work with if ($submit)
rhodesa replied to matthewst's topic in PHP Coding Help
Let's break some of this down: Looking good so far... The default value for 'action' is itself. So, if you want the page to submit to itself, just leave it out. This makes your page more portable. Also, for this particular use, I would stick with the GET method. This way the search term will be in the URL. There are a few reasons for this, but the big reason is it gives the user a URL they can bookmark,email to a friead, etc. You are using the wrong mysql function here. Use mysql_fetch_assoc() instead of mysql_fetch_array() here. The function arguments should remain unchanged. The first PHP statement is just stating a variable, and is basically meaningless. You need to echo it like the second PHP statement does. Also, in the second PHP statement, you don't need the quotes around the variable. Furthermore, unless you can GUARANTEE the run_users will have no 'html characters', you should wrap the variables with the htmlspecialchars() function. Just to clarify, the line should be: <option value="<?php echo htmlspecialchars($run_user_selected);?>"><?php echo htmlspecialchars($run_user_selected);?></option> Looks good. But, I would remove the name="submit" attribute as it won't be needed, and will produce a cleaner url query string. If you were using POST, this should be $_POST['submit']. But, since we are change to GET, and the submit button will no longer be passed as data, it's easiest to just test for a value of runner_name. To do that, let's use: if(strlen($_GET['runner_name'])){ To access the value of variable passed, you should use $_GET['runner_name']. Also, for security reasons, let's use the mysql_real_escape_string() function to prevent people from passing malicious code. So, the query should be: $slacker_current_runners = mysql_query("SELECT run_user,run_distance,run_time,run_calories,run_date FROM run WHERE run_user = '".mysql_real_escape_string($_GET['runner_name'])."'", $db_link); Again, use mysql_fetch_assoc() This part looks fine. But, unless you have a specific reason for storing the values into new variables, you can just echo the values from $row_current_runners directly, like: echo $row_current_runners['run_user']; -
touché
-
[SOLVED] Displaying a picture which is being pulled from MySQL
rhodesa replied to PdVortex's topic in PHP Coding Help
No, your query isn't returning any rows, so nothing will be shown. Are there any rows in your table? If so, what are the values of location/destination for them? -
glob() will not work on remote files. the loop is the only way if you are using remote files.
-
The best way (in my opinion) to approach this is with a variable passed by reference: <?php function Do_Something($what_to_do, &$errors = array()){ //Some checking script... $errors[]='error one happened'; //More checking script... $errors[]='error two happened'; //etc... return !count($errors); //Return true if no errors, false if there are } $errors = array(); if(!Do_Something('foo',$errors)){ echo "ERRORS:\n"; foreach($errors as $error){ echo " - {$error}\n"; } } ?>
-
http://us3.php.net/copy <?php copy($_POST['url'],'uploads/'.basename($_POST['url'])); ?> Obviously, the above is just an example. Any files being copied to your server should be thoroughly checked before they are put there. Also, the above will overwrite the file if it already exists.
-
You can load them all into an array (using a while loop). Then get the entries from the array throughout your page.
-
[SOLVED] Change Table Background Colour Using Javascript
rhodesa replied to stevesimo's topic in Javascript Help
try changing it to this: var mytable = document.getElementById('theTable'); -
It seems like a nested loop would be better: $s_sql = "SELECT `state` FROM `cities` WHERE `country` = 'US' ORDER BY `state`"; $s_result = mysql_query($s_sql); while($state = mysql_fetch_assoc($result)){ echo "Current state: {$state['state']}\n"; $c_sql = "SELECT cities from cities where country='US' AND state='$value'"; $c_result = mysql_query ($c_sql); while($city = mysql_fetch_assoc($c_result)){ echo " -{$city['cities']\n"; } }
-
It should look something like this: <?php $query = mysql_query("SELECT url FROM gallery") or die(mysql_error()); while ($row = mysql_fetch_assoc($query)){ echo $row['url']; } ?>
-
Before reinstalling, try starting FireFox in Safemode (FF safemode, not windows safemode). It may be one of your extensions. To start FF in Safemode: "C:\Program Files\Mozilla Firefox\firefox.exe" -safe-mode
-
Change <php? to <?php Why are you escaping the username? Do you do some DB call you aren't telling us about? You shouldn't trim passwords. Maybe the person wants a space at the end of it If you are storing the values into $username and $password, why are you still referring to the $_POST values? First, $username and $password should not have single quotes around them. PHP interprets that as a literal string, not the value of a variable. After that fix, this doesn't make any sense, because you are testing to see if a variable is equal to a copy of itself.
-
I think what you are looking for is something like this: $sql = "SELECT DISTINCT state from cities where country = 'US' ORDER BY state"; $result = mysql_query($sql); $states = array(); while($row = mysql_fetch_array ($result, MYSQL_ASSOC)){ $states[] = $row[state]; } $cities = array(); foreach ($states as $value) { $sql = "SELECT cities from cities where country='US' AND state='$value'"; $result = mysql_query ($sql); while($row = mysql_fetch_array ($result, MYSQL_ASSOC)){ $cities[] = $row[cities]; } } It would help if you specified exactly what you are trying to accomplish.
-
Shows up fine on FireFox 2.0.0.11 on Windows XP
-
This code is untested and written on the fly...but it should look something like this: <script type="text/javascript"> function loadFields ( ele ) { var fieldNode = document.getElementById('formFields'); fieldNodes.innerHTML = ''; if(!ele.value.length) return; for(var n=0;n < ele.value;n++) fieldNodes.innerHTML += '<input type="text" name="name_' + n + '" /><br />'; } </script> <form action="POST"> Select the number of people: <select name="num_people" onchange="loadFields();"> <option value=""></option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> <option value="10">10</option> </select> <div id="formFields"></div> </form>
-
on another note, instead of recursively calling a function with setTimeout, i would look into setInterval http://www.w3schools.com/htmldom/met_win_setinterval.asp
-
It should be more like: <?php require("config.php"); $connection = mysql_connect($host,$usr,$pwd); mysql_select_db($db_name,$connection); //Added select db function here if(!$_SESSION['login'] and !$username and !$password) { include("login.html"); exit(); } elseif (!$_SESSION['login']) { if($username == $admin_username and $password == $admin_password) { $_SESSION['login'] = true; } else { include("login_error.html"); exit(); } } if(!isset($action) or $action == 'login') { displayLinks($connection); //Removed $db here...also remove it from your function } ?>
-
This is a function I use all the time to convert a PHP array into the code for a JSON object: <?php /** * $arr - The array to convert * $trim - True will trim whitespace off the values * $r - True will add whitespace to the output to make it readable * $l - Ignore this variable */ function php_json_encode ( $arr, $trim = false, $r = false, $l = 0 ) { $json_str = ""; if(is_array($arr)){ $pure_array = true; for($i=0;$i < count($arr);$i++){ if(!isset($arr[$i])){ $pure_array = false; break; } } if($pure_array){ $json_str = "[\n".(($r)?str_repeat(' ',$l + 1):""); $temp = array(); for($i=0;$i< count($arr);$i++) $temp[] = sprintf("%s", php_json_encode($arr[$i],$trim,$r,$l + 1)); $json_str .= implode(",\n".(($r)?str_repeat(' ',$l + 1):""),$temp); $json_str .= (($r)?"\n".str_repeat(' ',$l):"")."]"; }else{ $json_str = "{\n".(($r)?str_repeat(' ',$l + 1):""); $temp = array(); foreach($arr as $key => $value) $temp[] = sprintf("\"%s\":%s", $key, php_json_encode($value,$trim,$r,$l + 1)); $json_str .= implode(",\n".(($r)?str_repeat(' ',$l + 1):""),$temp); $json_str .= (($r)?"\n".str_repeat(' ',$l):"")."}"; } }elseif(is_int($arr)){ $json_str = $arr; }elseif($arr === true){ $json_str = 'true'; }elseif($arr === false){ $json_str = 'false'; }else{ mb_internal_encoding("UTF-8"); $convmap = array(0x80, 0xFFFF, 0, 0xFFFF); if($trim) $arr = trim($arr); $str = ""; for($i=mb_strlen($arr)-1;$i >= 0;$i--){ $mb_char = mb_substr($arr, $i, 1); $str = (mb_ereg("&#(\\d+);", mb_encode_numericentity($mb_char, $convmap, "UTF-8"), $match)) ? sprintf("\\u%04x", $match[1]) . $str : $mb_char . $str; } $str = addslashes($str); $str = str_replace("\n", '\n', $str); $str = str_replace("\r", '\r', $str); $str = str_replace("\t", '\t', $str); $str = str_replace("&", '\&', $str); $str = preg_replace('{(</)(script)}i', "$1'+'$2", $str); $json_str = "'{$str}'"; } return $json_str; } $my_arr = array( 'foo' => 'bar', 'my_num' => 123 ); print '<script type="text/javascript"> myJSON = '.php_json_encode($my_arr).';</script>'; ?> Hope this helps
-
i guess if it was the only code in the script it would display the image. but you are probably looking for these functions: http://us.php.net/gd specifically check out: imagecreatefromgif() imagecopyresampled() imagegif()
-
Oh...and instead of mysql_db_query, you would just use mysql_query()
-
As long as you are only working with one database, you should use the mysql_select_db() function after your mysql_connect() function. Then, all you need is the $connection variable. As far as argument vs global, it's a matter of preference. I use global, but that is just me.
-
Sorry, but this code makes no sense. You can't include a GIF file. Please try to elaborate on what you are trying to do.
-
[SOLVED] another php question, in my coding >.<
rhodesa replied to robotman321's topic in PHP Coding Help
If get_magic_quotes_gpc is TRUE you want to REMOVE slashes, not add them....