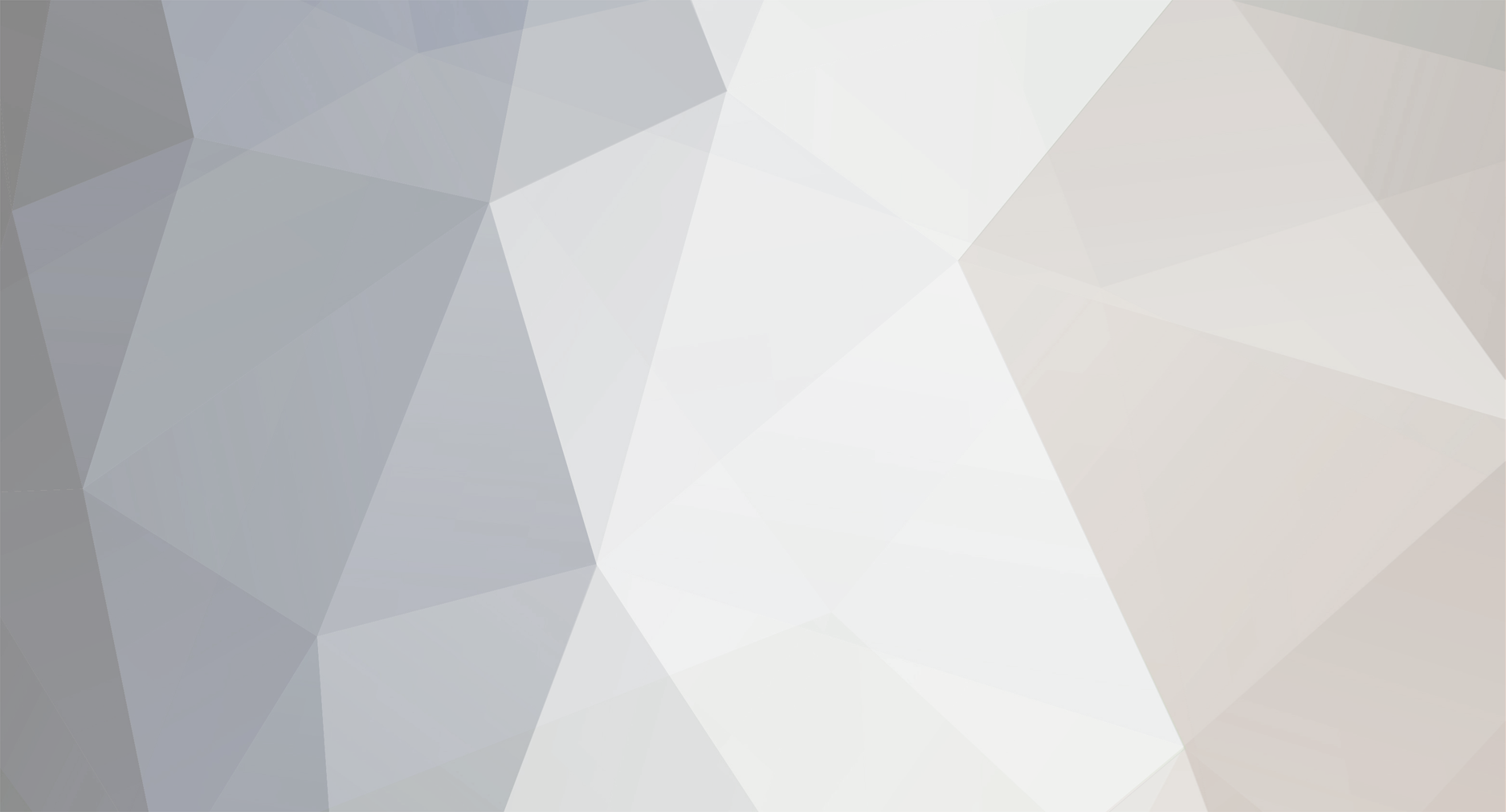
br0ken
Members-
Posts
421 -
Joined
-
Last visited
Never
Everything posted by br0ken
-
Stop fatal error on abstract class instantiation
br0ken replied to br0ken's topic in PHP Coding Help
Please ignore my question! Although I've been Googling this subject for a while, I didn't stumble across what I was looking for until just after I posted. If any one wants to know how to figure out whether or not a class is abstract at run time see the following link: http://php.net/manual/en/class.reflectionclass.php -
When I try to instantiate an abstract class a fatal error occurs. Is it possible to somehow suppress this error and return it as a string so that execution can continue? If this isn't possible, is there a way to test whether or not a certain class is abstract before trying to instantiate it? Thanks in advance!
-
This isn't really a Wordpress forum but have you read this page: http://codex.wordpress.org/Template_Tags/get_posts That tells you everything you need to know and more
-
Arg!!! I am going crazy with header function !!
br0ken replied to jtorral's topic in PHP Coding Help
You need a semi colon after Location. <?php header("Location: http://www.google.com"); exit; // Also, always add an exit after your header ?> -
Ignace is correct that a Student and Teacher are separate entities and therefore both need a separate class, but you are also right in noticing they share a common factor; they are both a human! The best way to do this would be to have a base class Human (or Person) and have both Teacher and Student extend from them. <?php abstract class Human { protected $_name = ''; protected $_gender = ''; protected $_role = ''; public function getName() { return $this->_name; } } class Teacher extends Human { public function __construct($name) { $this->_name = $name; $this->_role = 'Teacher'; } } class Student extends Human { public function __construct($name) { $this->_name = $name; $this->_role = 'Student'; } } $teacher = new Teacher('Mrs Smith'); $student = new Student('Jack Adams'); echo $teacher->getName(); //Returns Mrs Smith echo $student->getName(); // Returns Jack Adams ?> The benefit of this method is that both Teacher and Student can use the Human functions. This will save you duplicating code between the two objects.
-
writing a function based on if it is the index file or not?
br0ken replied to kade119's topic in PHP Coding Help
Actually you won't have to add the folder into the array as the $_SERVER['REQUEST_URI'] has the basename function wrapped around it. This function takes a filename and path as a string and returns the filename portion. This means that any folder on it will be removed and therefore you won't have to check it. To test your script, try printing out the value of basename($_SERVER['REQUEST_URI']) and see what you get on different pages. You can add which ever values are displayed on the homepage to the array. -
Based on the question, I imagine Zend is too complex for what this user requires. Also, with no knowledge of Zend I imagine the code sample you posted makes no sense what so ever.
-
I defined my array manually but you could parse yours dynamically easily enough. You could write this functionality manually using either substr and strpos or regular expressions. Alternatively, you could use one of the many pre-written PHP CSS parsers. http://www.google.co.uk/search?q=php+css+parser&ie=utf-8&oe=utf-8&aq=t&rls=org.mozilla:en-US:official&client=firefox-a
-
There are various different methods to creating your 'environment' (for the lack of a better word). The method you have described will work fine, however you will need to watch out for naming collisions. By asking whether your method is good practise, as opposed to just coding and hoping shows that you are on the right track. Keep asking questions and you'll be fine!
-
Prevent Spoofed Contact Form Submission_Security of Web form
br0ken replied to dingi's topic in PHP Coding Help
You could install a capctha box. They're one of those crazy things that force a user to write some scrambled words before allowing them to post. You can get some free ones and they're easy enough to install. http://www.captcha.net/ (There's load more, just Google Free Captcha) -
how you make a mysql query to be exactly accurate
br0ken replied to dbradbury's topic in PHP Coding Help
the equality operator (equals sign) checks for an exact match. If you have space on the end or the case is different then a match will not be returned. To get inexact matches you could convert the text to lower case using LOWER or use the LIKE operator. -
If I was doing something like that, I would parse the CSS into an array. Each element in the array would have the selector as the key and the values as the value. For example: <?php $css = array('body' => 'font-family:Arial;font-size:12px;', 'a' => 'color:#000;', 'a.big' => 'font-size:18px; font-weight:bold;', ); $selectorToFind = 'a.big'; $css[$selectorToFind] = str_replace('font-size:18px;', 'font-size:30px;', $css[$selectorToFind]); ?>
-
is this logical? '$l = ($_GET($row['forum_id']));'
br0ken replied to dbradbury's topic in PHP Coding Help
That is a little confusing! I won't pretend to know what you're trying to achieve but I did notice that you're trying to use the $album variable in your query, but you haven't actually initialised it yet. Where you have: if(isset($_GET['album'])) { Change it to if($album = isset($_GET['album'])) { -
writing a function based on if it is the index file or not?
br0ken replied to kade119's topic in PHP Coding Help
<?php // You should probably move this into your functions file function is_homepage() { return in_array(basename($_SERVER['REQUEST_URI']), array('', 'index.php', 'index.html', '/')); } $flash = 'http://www.testsite.com/banner.swf'; if (is_homepage()) { ?> <object width="550" height="400"> <param name="movie" value="<?php echo $flash ?>"> <embed src="<?php echo $flash ?>" width="550" height="400"> </embed> </object> <?php } else { echo '<img src="banner.jpg" alt="Image Banner"/>'; } ?> -
I just Google 'PHP Simple Calendar' and got quite a lot of results. This one looks pretty simply: http://php.about.com/od/finishedphp1/ss/php_calendar.htm
-
After a quick Google I found no stats but take a look at your own data and see how often it happens there.
-
is this logical? '$l = ($_GET($row['forum_id']));'
br0ken replied to dbradbury's topic in PHP Coding Help
This would work if $row['forum_id'] contain a string that was also a parameter name in the query string but I doubt it is what you're trying to do. What are you trying to achieve? -
I'm not sure what you're trying to do but when you do $variable = ''; you're assigning an empty value to $variable and aren't actually outputting anything.
-
writing a function based on if it is the index file or not?
br0ken replied to kade119's topic in PHP Coding Help
Yeah sure... Web servers can be set up to issue a default page if a user tries to access the domain with no URI. If this didn't happen, any user that went to http://www.testsite.com/ would see a blank page. The default page is usually either index.html, index.php or default.asp (for IIS machines). If a user accesses http://www.testsite.com or http://www.testsite.com/index.php, they are accessing the same page as the former will user will simply be shown the default page. Because of this, there are two possible request URI's for the homepage and you will need to check for both of them. Also, just thinking about it, you may sometimes find a backslash ( / ) as your request uri. You could use the following to check if your user is on the homepage: <?php function is_homepage() { return in_array(basename($_SERVER['REQUEST_URI']), array('', 'index.php', 'index.html', '/')); } ?> -
Unfortunately I'm not great with regular expressions (I'm terrible actually!) but I know you can specify strings that need to appear before and/or after a variable. Hopefully someone else on here can shed some light on this for you.
-
Each time you try to add a new array to your session you actually overwrite the current one. Consider the following: <?php // Assign an array to filename session variable // No matter how many times you do this filename will only contain 1 array $_SESSION['filename'] = array(); // Assign an empty array to filename session variable $_SESSION['filename'] = array(); // And then assign values to that array... $_SESSION['filename'][] = array('First Array'); $_SESSION['filename'][] = array('Second Array'); $_SESSION['filename'][] = array('Third Array'); $_SESSION['filename'][] = array('Fourth Array'); ?>
-
You could either use a complex combination of substr, strpos and str_replace or use a regular expression function: http://php.net/manual/en/function.preg-replace.php
-
im not sure whether this is possible or not...
br0ken replied to dbradbury's topic in PHP Coding Help
Another option (I did this a few years ago) is to write a publishing script that goes through each of your pages and publishes a static file for each. You can have them published in the URL structure you desire (LETTER/ARTIST). Then, on your current URL's, you simply add a 301 redirect (permanent redirect) to the new static HTML page. Eventually, Google will forget about your old URL's and you can remove your legacy script. -
writing a function based on if it is the index file or not?
br0ken replied to kade119's topic in PHP Coding Help
That's my bad... I've modified it in case anyone copies it in the future! Thanks! -
Getting a list of all subdirectories and storing them inside an array
br0ken replied to sh0wtym3's topic in PHP Coding Help
You will need to write a recursive function (a recursive function is a function that calls itself). The function will be passed a directory, which it will open, iterate through each subdirectory and call itself on that directory. See the following link: http://www.webcheatsheet.com/PHP/working_with_directories.php http://www.php.net/opendir http://www.php.net/readdir