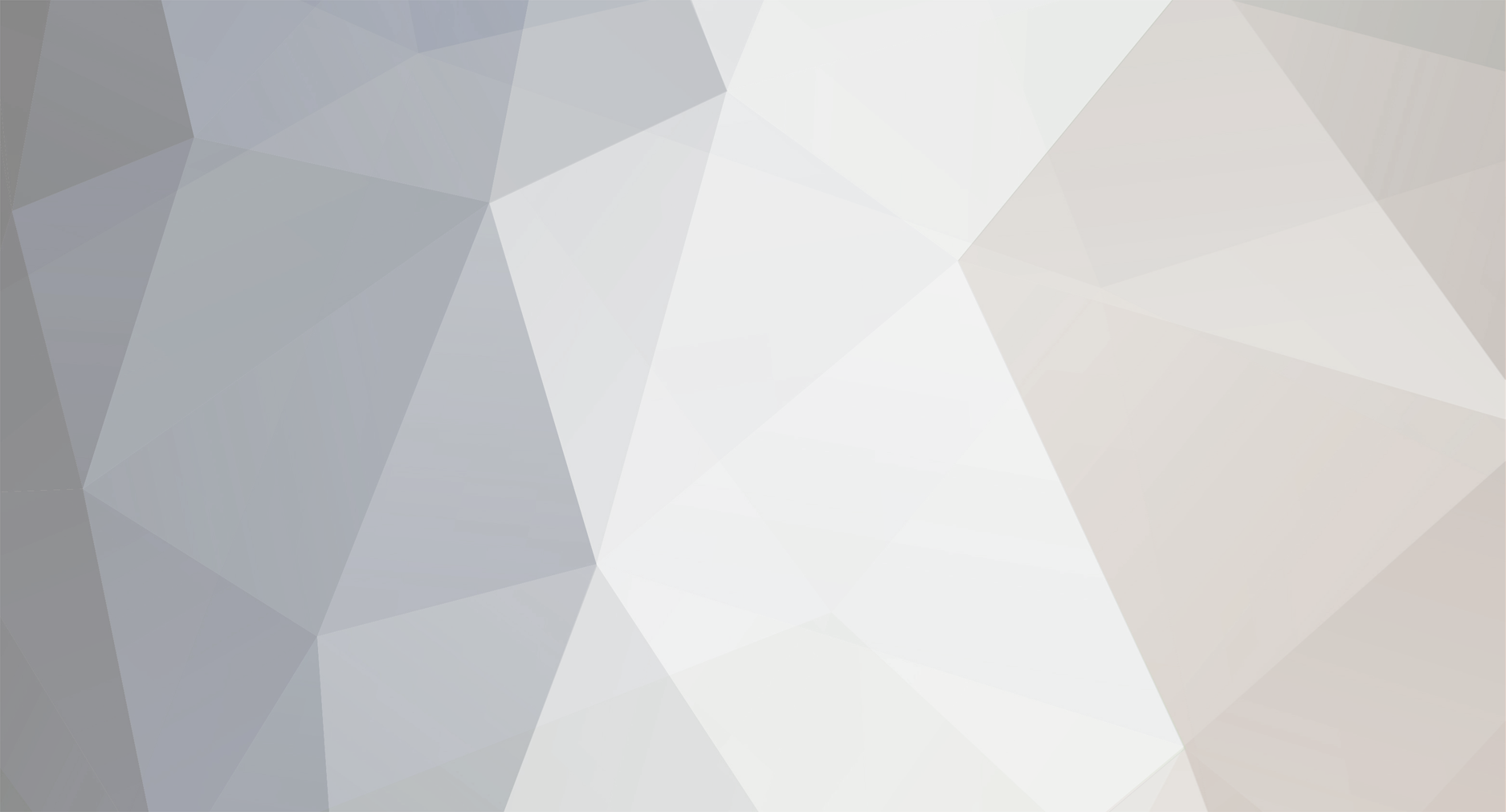
seanlim
Members-
Posts
355 -
Joined
-
Last visited
Everything posted by seanlim
-
try using a absolute URL instead? i.e. header("Location: http://yousitehere.com/home/test.htm");
-
Array Functions: Whats the difference between implode and explode?
seanlim replied to eldan88's topic in PHP Coding Help
Nope. The explode function breaks up a string. In your example, $array1 is an array. If you had a string "4,8,15,16,23,42", you could call the explode function on it to break it down into the equivalent of $array1. i.e. <?php $array1 = array(4,8,15,16,23,42); $string = "4,8,15,16,23,42"; $array2 = explode($string); //$array1 is the same as $array2 echo $array1[1]; // 8 echo $array2[1]; // 8 echo $string[1]; // will not work as expected ?> -
see the notes on session_destroy, the example there tells you exactly what you need to do to remove global session variables.
-
i'm pretty sure contentEditable objects are not sent together with the other form elements when the form is submitted. what you'll need to do is to use javascript to "copy" the contents of the contentEditable element, and add it into the form request e.g. by "pasting" into a hidden input element. So the entire process is: [*]User enters data into the div and clicks submit [*]Javascript copies data from the div [*]Pastes it into the a hidden input [*]Continue with form submission
-
I might be totally wrong here, but I don't see a point of passing the username through a get request if you are already storing it in a session variable! Instead of session_register, do a $_SESSION['myusername'] = $myusername; and on the second page, just do <?php echo $_SESSION['myusername']; ?> No need to play with GET requests!
-
PHP header redirect? header("Location: new_page_here.php");
-
i highly doubt that is possible with a normal textarea, as it is meant for plain, unformatted text. what you want is almost like a wysiwyg editor. You could try an editable <div> instead of a textarea and append a inner <span> to reverse the text direction.
-
First off, there's no Java in there. it's Javascript. Totally different. Secondly, i don't think you are passing the object right from the onclick handler. I have not done "pure" javascript in awhile, but from what I recall, you can't simply pass the object by id as you have done in hideLayer(thisone). I would suggest trying onclick="hideLayer('thisone')" and in your function, do a document.getElementById("thisone").style.display = "none";
-
In jQuery, you can simply use the removeClass('globalClass') method. Ideally (as you mentioned), you would modify only the CSS in the spirit of separating presentation from the markup. However, there will be trade-offs if you are adamant about sticking to this rule and don't want to go down the "#wrapper"-path mentioned by CPD. There are a host of selectors available http://www.w3.org/TR/CSS2/selector.html to define styles only for specific elements and even the "not" selector which you could use to prevent the new class from being styled. However, this might result in long and hard to maintain CSS code. I usually go somewhere in the middle, not overdoing the "unneeded" HTML elements but putting them in when they make sense. This should help prevent overly verbose CSS. Edit: the "not" selector is only available in CSS3
-
Validating and retaining state of a dynamic checkboxes
seanlim replied to femiot's topic in PHP Coding Help
It would help greatly if you indented your code nicely! $result = mysql_query("SELECT * FROM course") or die(mysql_error()); if ($result) while ($row = mysql_fetch_array($result)) { print "<input type=\"checkbox\" name=\"courses[]\" value=\"$row[cid]\"".(isset($_POST['courses']) and in_array($row['cid'],$_POST['courses'])) ? ' checked' : '') .">$row[cname]\n"; } *untested code The basic idea here is that you check if the id of the checkbox exists in the $_POST['courses'] array. If it does, you assume that the box was checked in the form submission, and print the "checked" attribute within the checkbox's HTML tag. -
Yes, that is entire normal and to be expected, if you have used the mysql_fetch_array function. The documentation states that it will "fetch a result row as an associative array, a numeric array, or both". When the optional second parameter is left out, it defaults to retrieving both. If you just want a numerial array, you can use mysql_fetch_row, or if you want a associative array, you can use mysql_fetch_assoc.
-
you probably have one or more invalid characters in your header("location: ..."); maybe try urlencode, you probably want to pass all your fields through that function before adding them to the URL, i.e. header("Location: blahblah.php?field1=".urlencode($field1));
-
The like buttons will each have to be "linked" to a different URL, so as to separate the likes. Take a look at the Facebook Developers site where they have the generator for their like buttons. You should be able to to figure out where the article's URL should go and insert that dynamically using PHP
-
Maybe, instead of $("document").on('submit', 'form.response', function() { Try $("form").submit(function(){
-
It is not even a Javascript problem! Try css: button{ width: 20em; } or, if you are using input buttons, input[type=button]{ width: 20em; }
-
Probably need to see the page in action to properly debug it, but if I were to hazard a guess, I would say that the variable you are applying ".closest()" to is actually a collection of elements. So jQuery is basically calling closest() on all the forms on your page. I might be totally wrong here, just guessing. Is this page hosted live where we can have a look?
-
They are effectively the same. However, as mentioned above, you will want to separate the logic from the presentation as much as possible to make your code readable and easy for maintenance and debugging. Take a look at the some templating options or even the MVC architecture. So, to answer your question they will provide the same results. However, if you are just printing plain HTML, it might be *slightly* faster if you do it outside of the <?php ?> tags (compared to using echo "...") as it won't have have to run through the PHP parser.
-
var_export seems to fit your requirements quite well
-
The AS keyword will allow you to "rename" a column, possibly into something more friendly. I don't know what the name of your columns are, but the select statement should look something like: SELECT s.*, h.name AS home_team_name, a.name AS away_team_name FROM... This will select all columns in the schedules table, and allow you to read the name columns from the two joined tables. In PHP, you can read it from the array as $row['home_team_name'] and $row['away_team_name']. HTH
-
Of course they are the same! You have: $field2 = $row["full"]; $field3 = $row["full"]; I would be shocked if they were different! Take a look at his thread that was just resolved: http://www.phpfreaks.com/forums/index.php?topic=356057.msg1682808#msg1682808
-
If that's the case, you'll probably need something to the effect of... SELECT * FROM schedules s LEFT JOIN teams h ON s.home = h.id LEFT JOIN teams a ON s.away = a.id
-
Maybe you should var_dump() the SQL query, just to make sure that the POST variables are getting passed properly, you SQL syntax is well-formed, and that the SQL database is returning the desired results.
-
Too much code. Your login script could just post to itself and use a header to redirect on success. The basic flow would be: <?php // login.php if(isset($_POST['login'])) { // do login here, on success: header("Location: main.php"); } ?> <html> ... <body> <!-- form here --> </body> </html> <?php //main.php if(**not logged in**) header("Location: login.php") // print success message ?>
-
can you clarify if the data is in multiple "DBs" as stated in your question or "Tables" as stated in your title? they are actually quite different as Muddy_Funster was trying to point out. Data can be easily joined when they are in the same DB on different tables, but it will probably get messy if they are in totally separate databases
-
use the AS keyword! SELECT d.value AS username, c.value AS postcode, d.user_id, you can then use mysql_fetch_assoc to fetch $list['username'] and $list['postcode']