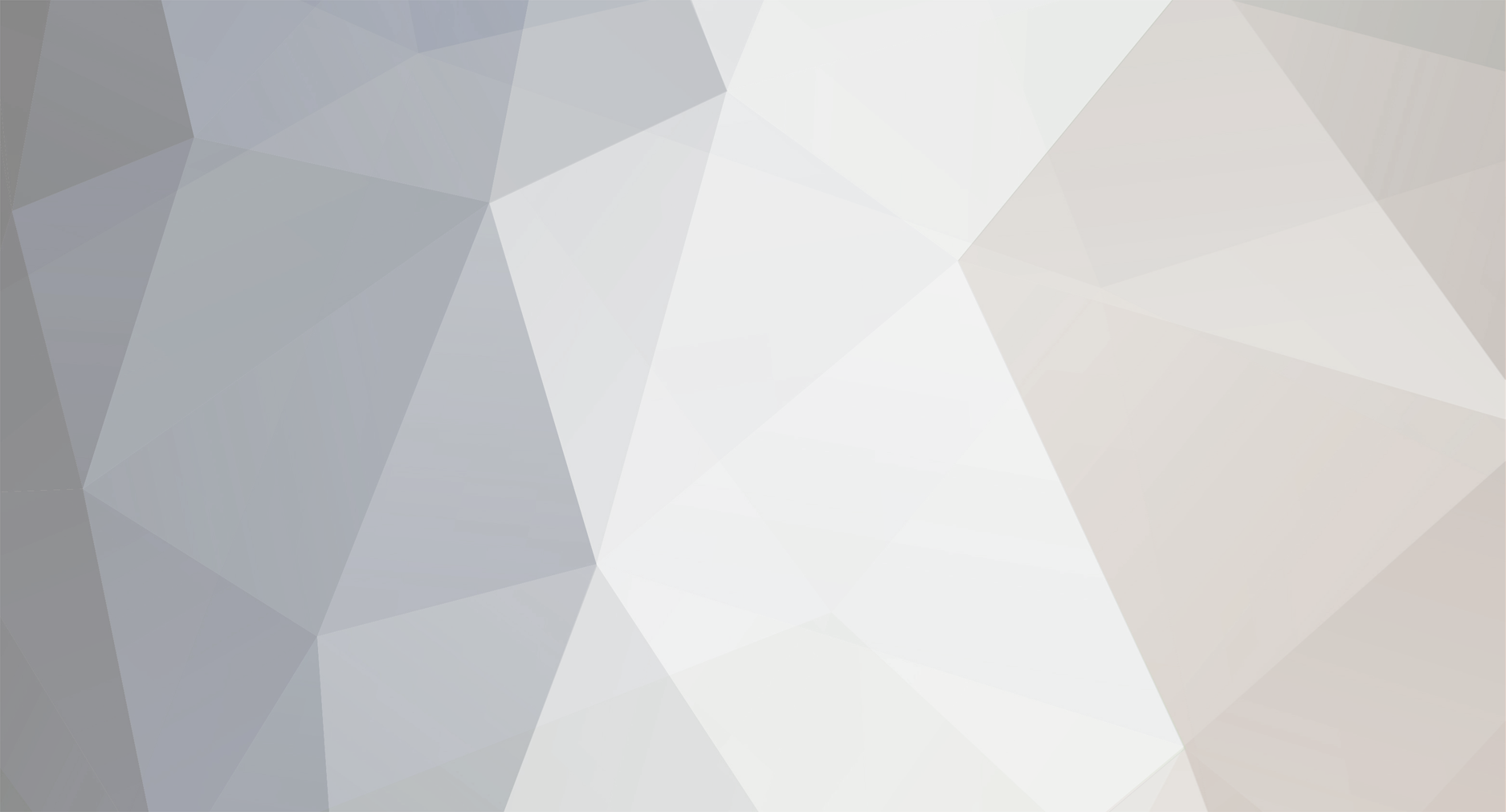
inactive
-
Posts
97 -
Joined
-
Last visited
Posts posted by inactive
-
-
Thanks again. Only minor issue is that it doesn't handle an empty field very well (will show up as a double-quote in the array instead of NULL). For some reason this isn't a problem for the last field.
For example the following string:
"Home","","Wingrove"," Lyn"," ","2/125 Albatross"
Will show come out in the array as:
[0] => Home [1] => " [2] => Wingrove [3] => Lyn [4] => " [5] => 2/125 Albatross
However:
"Home","","Wingrove"," Lyn"," ","2/125 Albatross",""
Will show come out in the array as:
[0] => Home [1] => " [2] => Wingrove [3] => Lyn [4] => " [5] => 2/125 Albatross [6] =>
(Last empty quote goes into array correctly)
A little more tricky than you suspected?
Thanks heaps.
-
Hey thanks benjaminbeazy, yeah I thought I'd have to split( or explode( or something. Just a quick query, but what were you trying to achieve with the foreach loop? It doesn't seem to do anything at the moment. I assume you were trying to get rid of the extra quotes left over? Being a noob and all, I have no idea if there's a typo or anything lol...
Thanks mate.
-
Sorry yes, one other thing is that this file I access using curl is then saved as a .CSV file on the server, so yes it is a CSV file. Sorry for confusion.
So apart from regex or split (splitting by ","), no other suggestions?
-
heard of snoopy? it has a text only setting i'm pretty sure. have a look around sourceforge for it.
-
Good suggestions thanks BillyJim and cooldude, however as previously indicated I do not have any control over the data, so I cannot change the delimiter or dump it into Excel first.
A bit of background might clear this up slighly (sorry for being confusing previously):
I will be using php to access a CSV file located on another server (http access, using curl) which I have no control over. I have to then convert this CSV file into an array for further processing. This process will run every 10 minutes, permanently, and the CSV file is about 3Mb, so as you can see it is not possible to jump in half way with Excel. Besides, as the file is already in CSV format, Excel reads is incorrectly anyway (no fault of Excel, just bad CSV formatting, but unfortunately I cannot change that fact).
What am I left with?
-
Very good point, especially as there are standards associated with proper CSVs
However unfortunately I do not have control over the data I have to process, and as such both the delimeter char and start/end field char may be somewhere in the field values, and I need to be able to work around this.
Any suggestions?
-
Hey guys,
I've seem a million different scripts/functions out there that build on fgetscsv, but they don't do exactly what I want.
I'm looking to convert a CSV file to an array, where the field end char is " (double quote) and decimeter is is , (comma), but where field data may contain commas or double quotes, but this should not indicate end of field, unless it is specifically end-of-field,delimiter,start of field (i.e. "," [double-quote, comma, double-quote]).
So for example the following data:
"james","foo,bar","baby"face","trollop"
Would produce the following array:
[0] => james [1] => foo,bar [2] => baby"face [3] => trollop
The data would always be in this format (i.e. each field will be surrounded by double quotes, and then delimited by a comma).
Any ideas? As I would prefer not to trim of the start and end double-quotes and then split by "," if you know what I mean.
Thanks guys. Looking forward to response.
-
-
yeah thats what i ended up using (roughly).
what about using preg_match?
-
tks rhodesa, very helpful idea.
but out of curiosity, does anyone know how i would do what i wanted without fgetscsv?
-
hey guys just doing my first preg_match to look for a valid email address.
im using "^([a-zA-Z0-9_\-\.]+)@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.)|(([a-zA-Z0-9\-]+\.)+))([a-zA-Z]{2,4}|[0-9]{1,3})(\]?)$^"
which works, but the thing is i need it to pick out an email out of a csv-like string.
i.e. the sting may be:
"foo","bar","foobar","foo@bar.com"
and i need it to pick out the foo@bar.com, and just cant work out how to do it.
any ideas?
probably a kinda lame question i know, but all the regex tutes i find are so complex...
cheers.
-
ha wicked.
legendary thanks mmarif4u.
-
thanks mmarif4u, you are quite correct. when i removed the get vars (i.e. dropped the question mark and all that followed), the include worked perfectly.
so i cant use ? in the file name...but is thers another way to perhaps pass some vars through to monkey.php? again, i'm not sure if i have the right idea here, or even if this is possible.
the reason i was using monkey.php?trace=blah is because perhaps in another script i might try to include monkey.php?trace=foobar and i would like it to behave differently.
i.e. in monkey.php:
$trace = $_GET['trace']; if (trace == 'blah') { do whatever } elseif (trace == 'foobar') { do something else }
so i need some way to pass a variable or sorts to monkey.php as i include it, so it will behave differently. is this possible?
from what Kris said, maybe before i write the include i just set $trace then,
i.e. in index.php
$trace = 'blah'; include('monkey.php');
and then in some other file
$trace = 'foobar'; include('monkey.php');
is this a better idea?
thanks everyone for your help.
-
i tried changing my include_path to htdocs within the apache install dir obviously), but same errors, just with obviously the changed include_path listed.
any ideas guys?
-
lol i figured i had NFI, but from what you've explained, thats fine and would still work great. only it doesnt work... ???
-
hmmm ok well i've seen a few similar threads to this around, but none of them have been closed, so i thought the best idea was to start from scratch.
ok well i have two files, index.php and monkey.php. both are in the same directory (which happens to be htdocs, as im running apache).
in index.php we have:
<?php include('monkey.php?trace=blah'); ?>
and in monkey, we effectively have:
<?php if(isset($_GET['trace'])) { $trace = $_GET['trace']; echo 'some stuff'; echo $trace; echo 'perhaps some more stuff'; } ?>
monkley.php is slightly more cmplicated than that, but i think all that stuff is unnecesary.
basically, what i'm trying to do is parse monkey.php?trace=blah as though all that php code was actually within index.php.
now i'm reli new to this, so maybe include is even the wrong thing to use, i dunno (i'm hoping someone here might be able to help).
instead, this is what is showing up in my apache error logs:
[Wed Jan 16 16:40:41 2008] [error] [client 127.0.0.1] PHP Warning: include(monkey.php?trace=blah) [<a href='function.include'>function.include</a>]: failed to open stream: No error in C:\\server\\Appache\\htdocs\\index.php on line 3 [Wed Jan 16 16:40:41 2008] [error] [client 127.0.0.1] PHP Warning: include() [<a href='function.include'>function.include</a>]: Failed opening 'monkey.php?trace=blah' for inclusion (include_path='.;C:\\php5\\pear') in C:\\server\\Appache\\htdocs\\index.php on line 3
any ideas? i've even tried placing ./ or ../ before monkey.php?trace=blah, but nothing.
any help appreciated muchly guys.
~mc.
-
yep, as you said Kris it was just the spelling of the post var.
thanks heaps.
-
gah! i think thats it. thanks Kris. i guess thats why they say you should never proff your own code.
and yes i could probably get rid of the ssl stuff too...
i'll try it tonight. thanks again.
-
hmmm yeah thats what i suspected, however i used that snoopy class to pull the site, and it works fine...i dont know whether snoopy automatically spoofs the referer header...i doubt it... any other suggestions? i would prefer not to have to use snoopy, thats all...
-
hey guys kinda new to this
trying to use curl to submit some post vars to a page, and then print the results.
i've tested submitting to my own page, and the vars are coming through fine, but
when i submit to the actual page im trying to use (the tracking page for australian
air express), the retuned page is just the normal page as though no post vars went
through. it actually says 'Please enter a Consignment/Article number before
requesting a search,' which i take to mean it know its been submitted to, but didnt
pull the var thats meant to be looked up (enquirynumber).
Ok so heres my code
$url = 'http://203.43.1.230/scripts/cgiip.exe/WService=wtsaae/inquiry.w'; $params = "searchbutton=Search&enquirynumber=UXW81825"; $user_agent = "Mozilla/4.0 (compatible; MSIE 5.01; Windows NT 5.0)"; $ch = curl_init(); curl_setopt($ch, CURLOPT_POST,1); curl_setopt($ch, CURLOPT_POSTFIELDS,$params); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2); curl_setopt($ch, CURLOPT_USERAGENT, $user_agent); curl_setopt($ch, CURLOPT_RETURNTRANSFER,1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); $result=curl_exec ($ch); curl_close ($ch); echo $result;
and you can test whats meant to happen by going to
http://203.43.1.230/track/inquiry.html and entering
UXW81825.
Is there anything wrong with my code? or is something
different betwen refferencing to an external file, rather
that the local file i tested it on?
I know that the site is not filtering out based on referer
or anything, because i have managed to do this before
using snoopy (php class).
Hope i havent been too confusing here, and would love
any comment or suggestions, or help! lol
Thanks guys
mitch.
[SOLVED] CSV to array
in PHP Coding Help
Posted
Thanks ben. Your code works well with the data you provided. However my data will not contain spaces after each field.
The data I'm likely to get could be as bad as this:
In which case the following code works best:
Which returns:
Which is 100% correct.
So basically I'm exploding by "," (which will always be between each field), and then removing the the quotes (") from before the first field and and also from the end of the last field.
I thought I may have to do this in the first place, but I was hoping there was a more elegant solution. However this works 100% so I will settle for this.
Thanks heaps to all those who helped out and suggested ideas.
~Mitch.