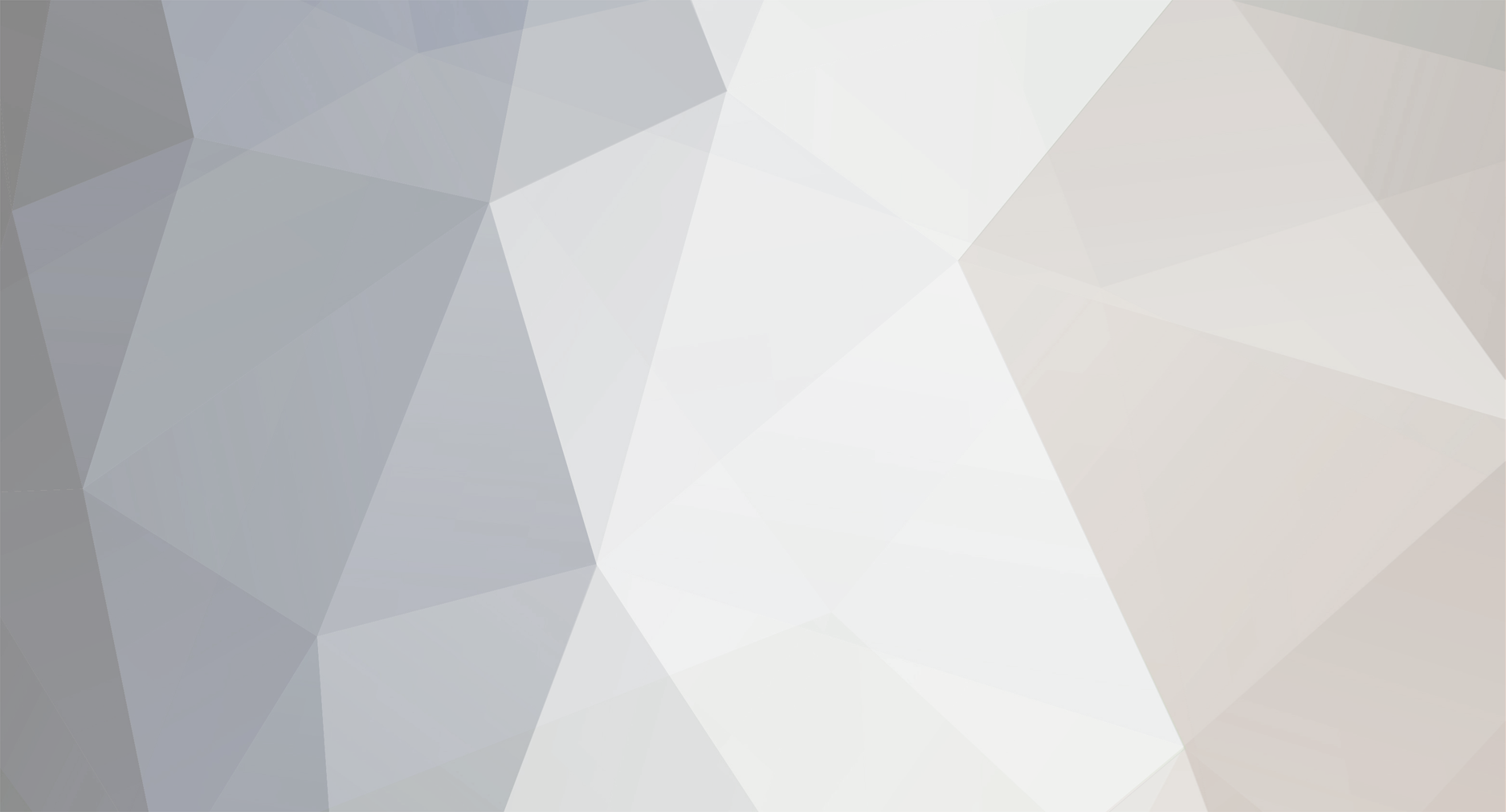
yuckysocks
Members-
Posts
32 -
Joined
-
Last visited
Never
Everything posted by yuckysocks
-
I did indeed; that's actually at the root of the foreach loop. Good suggestion though. Anyone else have any ideas?
-
Hi all. I'm trying to get data into a form that works with flot, the jQuery graphing library. I need to get UTC timestamps from Twitter into a javascript timestamp (=Unix timestamp * 1000). My code currently looks like this: foreach($decoded['results'] as $currentResult) { if($currentResult['from_user'] == $user) { $strippedTexts = $currentResult['created_at']; $bignumber = strtotime($strippedTexts); $biggerstring = bcmul($bignumber, 1000); $dates []= settype($biggerstring, "int"); } } This creates a dates[] array that contains the value "1", once. Clearly not what I want. I've tried multiplying by 1000 (resulted in a large number barf: -2147483648), multiplying by 1000.0 (to convert to a float, maybe it would take a bigger number). I've also tried concatenating "000" and using setype($var, "float") and that won't work either. Any idea how I can get the incoming array of UTC time stamps into "milliseconds since 1970" (which is what the flot library needs, as an integer, not a string)? If it helps, here's the entire code, soup to nuts: <?php $url = "http://search.twitter.com/search.json?q=%23runlogger"; $contents = file_get_contents($url); $decoded = (json_decode($contents, true)); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <head> <script type="text/javascript" src="jquery-1.3.2.min.js"></script> <script type="text/javascript" src="jquery.flot.js"></script> </head> <body> <form name="input" action="skeleton.php" method="get"> Twitter Username: <input type="text" name="user" /> <input type="submit" value="Go Get 'em, Tiger" /> </form> <?php if (isset($_GET['user'])) { $miles = array(); $dates = array(); $pairs = array(); $user = $_GET["user"]; foreach($decoded['results'] as $currentResult) { if($currentResult['from_user'] == $user) { $strippedTexts = explode(" ", $currentResult['text']); $miles []= $strippedTexts[0]; } } foreach($decoded['results'] as $currentResult) { if($currentResult['from_user'] == $user) { $strippedTexts = $currentResult['created_at']; $bignumber = strtotime($strippedTexts); $biggerstring = bcmul($bignumber, 1000); $dates []= settype($biggerstring, "int"); } } foreach($miles as $key=>$mile) { $pairs[$dates[$key]] = $mile; } ksort($pairs); foreach($pairs as $d=>$m) { $orderedpairs .= "[$d, $m], "; } echo " <div id='placeholder' style='width:600px;height:300px'></div> <script language='javascript' type='text/javascript'> $(function () { var data = [[$orderedpairs]]; var plotarea = $('#placeholder'); var options = { legend: { show: true } xaxis: { mode: time } } $.plot( plotarea , data , options ); }); </script> $orderedpairs "; } else { echo "Go ahead and search for a name! Try 'Tester2314' to see what an example could look like"; } ?>
-
Hi there, I just got a project to work, and want to know where I could have improved the code. Thanks for taking a look: This is a Cron every hour and sets up the database: <?php // displays all the file nodes $xml=simplexml_load_file('http://www.weather.gov/xml/current_obs/KBOS.xml'); if ($xml) { //sets up all the variables I need for the SQL addendum $weather = $xml->weather; $temp = $xml->temp_f; $wind = $xml->wind_mph; $date = $xml->observation_time; } //error catching else { echo "Oops, bad input"; } $db = mysql_connect('blah, blah, blah') or die(mysql_error()); mysql_select_db('table') or die(mysql_error()); mysql_query("INSERT INTO weatherdata (date, weatherstr, temp, wind) VALUES ('$date', '$weather', '$temp', '$wind')"); mysql_close($db); ?> And the next part fetches the data I want and makes it as 45, 48, 59, 64.... for the last 35 values. <?php //open connection $db = mysql_connect(blah, blah, blah) or die(mysql_error()); mysql_select_db('tables') or die(mysql_error()); $result = mysql_query("SELECT * FROM weatherdata ORDER BY id DESC LIMIT 35"); $row = (mysql_fetch_array($result)); while($row = (mysql_fetch_array($result))){ $output[] = $row['temp']; } $reverse = (array_reverse($output)); $csv = implode(", ", $reverse); ?> I want to know if this leaves memory open, if I could have done this more elegantly, or anything like that. I have the FUNCTION down, now I'm worried about learning good FORM. Thanks! -Alex
-
Hello. I have a query, and I want the results eventually to be variables that I serve to a JS library to make graphs. I have this currently: $result = mysql_query("SELECT * FROM weatherdata ORDER BY id DESC LIMIT 10"); $row = mysql_fetch_array($result) or die(mysql_error()); $row2 = array_reverse($row); foreach($row2 as $a){ echo $a."<br />"; } The table is 5 fields (including the key ID). The returned output is only the most recent entry, twice! 18<br />18<br /> 13<br />13<br /> 51<br />51<br /> Mostly Cloudy<br />Mostly Cloudy<br /> Last Updated on Mar 14, 4:54 pm EDT<br />Last Updated on Mar 14, 4:54 pm EDT<br /> With the actual DB entry looking like this: ID = 18 Wind = 13 temp = 51 weatherstr = Mostly Cloudy date = Last updated on Mar 14, 4:54 pm EDT I don't know why it only shows the most recent entry (DB has >50 entires so far, it's populated hourly), nor why it's shown twice. What I'm looking for, eventually, is to query the 10 most recent entries, and set the "temp" result for each as a variable ($a, $b, $c...) and then feed those variables to a JS library to make a graph. Any suggestions would be helpful!
-
[SOLVED] I can't determine what the syntax error is
yuckysocks replied to yuckysocks's topic in PHP Coding Help
it was on 27, and with a different editor, I found some weird errors. I copied/pasted from a website, but something that showed up as a space in the browser was actually encoded as a weird E`. Hard to find! -
Easy fix, no doubt: $db = mysql_connect('xxxxx.xxxxxx.net','xxxxxx','xxxxxxxxxxx') or die(mysql_error()); mysql_select_db('xxxxxxxxxxxxx') or die(mysql_error()); mysql_query("INSERT INTO weatherdata (date, weatherstr, temp, wind) VALUES ('$date', '$weather', '$temp', '$wind')"); mysql_close($db); Unexpected T String Error. Gah! I don't THINK I have a syntax error, but of course, no one ever does...
-
That's all I needed to hear. Thanks for the help.
-
ok, so here is the code I have: Blah <?php // displays all the file nodes if(!$xml=simplexml_load_file('http://www.weather.gov/xml/current_obs/KBOS.xml')){ trigger_error('Error reading XML file',E_USER_ERROR); } $weather = $xml->current_observation->weather; echo $weather; ?> When I load up the page (not locally, for what it's worth) it only displays "Blah", not even the error specified. Can I not use the simplexml_load_file function on remote files? I know fopen works remotely... but that's not what I really want to do. Suggestions?
-
Hi all, I'm enjoying learning myself, but want to make sure my big picture steps are efficient for this project: I want to make a running http://sparkline.org/ of "good" weather vs. "bad" weather in my area. This will look at a federal XML file once a day with a cron job, parse the data, store it to my own database, then query the previous 10 entires in the DB to create the sparkline. Is this a reasonable way to execute this project? Also, I'm at the point where I can import the xml stream, but am not sure the best way to get at it. Xpath queries? Can't some function turn it into an array? I'm not so good at these things, so any hints would be great. Thanks!
-
hrmm. at -least a semi colon. (isn't it always a semi-colon?) but that still dumped out the errors. I don't know much about the functions (it's a hello world example, after all) file_handle looks like a variable, so I tried it with a $ in front: while (!feof($file_handle)); but that didn't reply with -anything- still stumped!
-
Hi there. Running examples from a webbots book. Here's the code: <? $target = "http://www.schrenk.com/nostarch/webbots/hello_world.html"; $file_handle = fopen($target, "r"); #fetch the file while (!feof(file_handle)) echo fgets($file_handle, 4096); fclose($file_handle); ?> I ran it from terminal on OS X 10.5 (FWIW) and it spat back infinite lines of: Warning: feof(): supplied argument is not a valid stream resource Instead of the expected line-by-line response from the targeted web server. It DOES show the source HTML after each error message, but when the source is done, the errors continue to infinity and I have to close Terminal. I'm a PHP newbie, so go ahead and talk down to me Thanks for explaining what's happening, Alex
-
Yes, Yes, 1000 times yes. It's amazing/frustrating/thrilling when just two characters make a script work or not work. Thanks for the continued help and support. Topic Solved.
-
Unfortunately, the second header is still displayed as text at the start of the email (the text/html char encoding one). Any other ideas? This is not a huge deal, but I want the resulting email to be as useful as possible to the recipient (ie, just click a link instead of copy/paste it in). Thanks again for the help, Alex
-
I implemented Craygo's code. The variable is converted to the proper word, but the second header (Content-Type: text/html; charset=ISO-8859-1) is just displayed, as opposed to being used as a header. This prevents the link from being converted into a proper anchor element. Thoughts? Thanks so much, Alex
-
Hi, I'm looking to get this function to work: mail('[email protected]', 'A new case study has been posted!', 'Go to <a href="admin.php">the admin page</a> to look it over. It is information regarding $schoolname', 'From: [email protected]'); The mail gets sent properly, but the link and the variable aren't treated properly. The message is in fact going to gmail, so maybe it has something to do with how they treat html in messages, but at least the variable ought to get filled in, eh? It's a POSTed variable, do I need to call it that? Anyhow, thanks for your always prompt help. Alex
-
[SOLVED] Can someone troubleshoot a simple script?
yuckysocks replied to yuckysocks's topic in PHP Coding Help
Well, at least you're blind and not a total newbie who didn't recognize that at all. I printed out the file, and went through it line by line, writing in English what each part did, and next to $sql = QUERY I wrote "performs operation". Shows how much I still have to learn! Thanks so much for all the help. -
[SOLVED] Can someone troubleshoot a simple script?
yuckysocks replied to yuckysocks's topic in PHP Coding Help
What I have right now is <?php // Connecting, selecting database $link = mysql_connect('localhost', 'xxxx', 'xxxx') or die('Could not connect: ' . mysql_error()); mysql_select_db('case_studies') or die('Could not select database'); if(!isset($cmd)) { // Performing SQL query FOR UNAPPROVED CASES $query = 'SELECT id, schoolname, schoolcity, state FROM casestudies WHERE approved = 0'; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); // Printing results in HTML echo "<table class=\"sortable\">\n"; echo "<th>School Name</th> <th>School City</th> <th>State</th> <th>Approval Link</th>"; while ($line = mysql_fetch_assoc($result)) { $id = $line["id"]; $schoolname = $line["schoolname"]; $schoolcity = $line["schoolcity"]; $state = $line["state"]; echo <<<END \t<tr>\n; \t\t<td><a href='casestudy.php?id=$id'>$schoolname- Check me for Accuracy</a></td>\n; \t\t<td>$schoolcity</td>\n; \t\t<td>$state</td>\n; \t\t<td><a href='admin2.php?cmd=approve&id=$id'>Approve the $schoolname case study and make it public</a>; \t</tr>\n END; } echo "</table>\n"; } if($_GET["cmd"]=="approve") { $id = $_GET["id"]; $sql = "UPDATE casestudies SET approved = 1 WHERE id = $id"; } // Closing connection mysql_close($link); ?> Clicking on the links still won't update my data... Maybe my query is wrong? Also, fyi, I stripped the semi-colons from the echo-->HTML section. I ended up with 5 semis x 3 records = 15 semi colons in a line up top. Any hints would be great. Thanks! -
Ok, but right around now, people are putting up "solved" posts. Is this feature now included? How are these showing up?
-
Hi, My goal is to show a table of user-posted results to an admin, who can then approve the data and change the value of the "approved" column from 0 to 1. <?php // Connecting, selecting database $link = mysql_connect('localhost', 'xxxx', 'xxxx') or die('Could not connect: ' . mysql_error()); mysql_select_db('case_studies') or die('Could not select database'); if(!isset($cmd)) { // Performing SQL query FOR UNAPPROVED CASES $query = 'SELECT id, schoolname, schoolcity, state FROM casestudies WHERE approved = 0'; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); // Printing results in HTML echo "<table class=\"sortable\">\n"; echo "<th>School Name</th> <th>School City</th> <th>State</th> <th>Approval Link</th>"; while ($line = mysql_fetch_array($result, MYSQL_ASSOC)) { echo "\t<tr>\n"; echo "\t\t<td><a href='casestudy.php?id=".$line['id']."'>".$line['schoolname']."- Check me for Accuracy</a></td>\n"; echo "\t\t<td>".$line['schoolcity']."</td>\n"; echo "\t\t<td>".$line['state']."</td>\n"; echo "\t\t<td><a href='admin.php?cmd=approve&id=".$line['id']."'>Approve the $schoolname case study and make it public</a>"; echo "\t</tr>\n"; } echo "</table>\n"; } if($_GET["cmd"]=="approve") { $id = $_GET["id"]; $sql = "UPDATE casestudies SET approved = 1 WHERE id = $id"; } // Closing connection mysql_close($link); ?> Some ideas are cobbled together from different sample scripts, so I don't really know where the issue lies. When I click on the "Approve" link, it goes to a blank page, but the data remains unchanged. Help! Also, thanks so much to everyone in the forum, you all are VERY helpful. -Alex
-
Queuing up and then letting through data
yuckysocks replied to yuckysocks's topic in PHP Coding Help
Hi! I'm unable to upload/test things right now, but I'm sure there are bugs here that people can work out for me: <?php // Connecting, selecting database $link = mysql_connect('localhost', 'neepor', 'energy') or die('Could not connect: ' . mysql_error()); mysql_select_db('case_studies') or die('Could not select database'); if(!isset($cmd)) { // Performing SQL query FOR UNAPPROVED CASES $query = 'SELECT id, schoolname, schoolcity, state FROM casestudies WHERE approved = 0'; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); // Printing results in HTML echo "<table class=\"sortable\">\n"; echo "<th>School Name</th> <th>School City</th> <th>State</th> <th>Approval Link</th>"; while ($line = mysql_fetch_array($result, MYSQL_ASSOC)) { echo "\t<tr>\n"; echo "\t\t<td><a href='casestudy.php?id=".$line['id']."'>".$line['schoolname']."- Check me for Accuracy</a></td>\n"; echo "\t\t<td>".$line['schoolcity']."</td>\n"; echo "\t\t<td>".$line['state']."</td>\n"; echo "\t\t<td><a href='admin.php?cmd=approve&id=$id'>Approve the $schoolname case study and make it public</a>"; echo "\t</tr>\n"; } echo "</table>\n"; } if($_GET["cmd"]=="approve") { $sql = "UPDATE casestudies SET approved = 1 WHERE id = $id"; // Free resultset mysql_free_result($result); // Closing connection mysql_close($link); ?> I think I'm on the right track, but need to be sure. Thanks! -
Excellent, that works well! Now, this is just for my own education... This table has 74 fields. Is there another way to do that without copying and pasting each field? Like, step through each field and check for "isset"? Anyhow, thanks for your time, once again. Alex
-
I'm not sure that will do what I want. The code I have now looks like this: <?php $id = intval($_GET['id']); echo '<div id="content">'; echo '<h3 id="title">Case Study Details</h3>'; $link = mysql_connect('localhost', 'xxxxxxx', 'xxxxxxx') or die('Could not connect: ' . mysql_error()); mysql_select_db('case_studies') or die('Could not select database'); $query = "SELECT * FROM casestudies WHERE id = $id"; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); $row = mysql_fetch_assoc($result); echo "<h4>{$row['schoolname']}</h4>"; foreach ($row as $fld => $val) { echo '<p class="caseresult"><b>' . $fld . '</b> : ' . $val . '</p>'; } ?> So they've clicked on a link that gives PHP the id value for what they want to look at. It then selects all from the row WHERE id=$id. Then it spits it all out with the $fld : $val format. What I want is, when there is no $val entered, for the associated $fld to also not be shown. Test example is here: http://www.neep.org/HPSE/csstemplate/casestudy.php?id=17. I want all of the blank fields to not be shown at all (such as projectstart, constructionend, constructionstart, and masterplan). Thanks for the help, Alex
-
How can I call for all values from a table row, but exclude blanks? What I show now is this: 1. Alex 2. Boston 3. 4. 5. Male 6. Orange What I'd prefer is this: 1. Alex 2. Boston 5. Male 6. Orange Do I change my query, or how I echo my data once I have it? I know this is a basic question, so thanks for the help!
-
Queuing up and then letting through data
yuckysocks replied to yuckysocks's topic in PHP Coding Help
I like that solution. Before I read it, the basic idea I had come up with was: 1. Post all data to a table called "pending" 2. Admin views a (private) page with all "pending" cases. 3. When a button is pressed, it copies the data from "pending" into the public "show" database. Is this a reasonable way to accomplish this also? Is this "expensive" to do, as far as server load goes or anything like that? We are low traffic so it's moot anyhow, but I'd like to only use well-tuned options. Thanks for any critique! -
I have a form that posts data to a mysql database, which is then pulled out into a table. The form will never be publicly linked, so I didn't bother with logins or anything like that, but I'm wondering 1. What's the easiest way to have the data being posted held, then checked by an administrator for accuracy, and then allowed to be displayed on the public page? 2. Are there significant risks of running an unsecured form if it's never linked anywhere except from e-mails sent to select people? Thanks a lot for the info, Alex