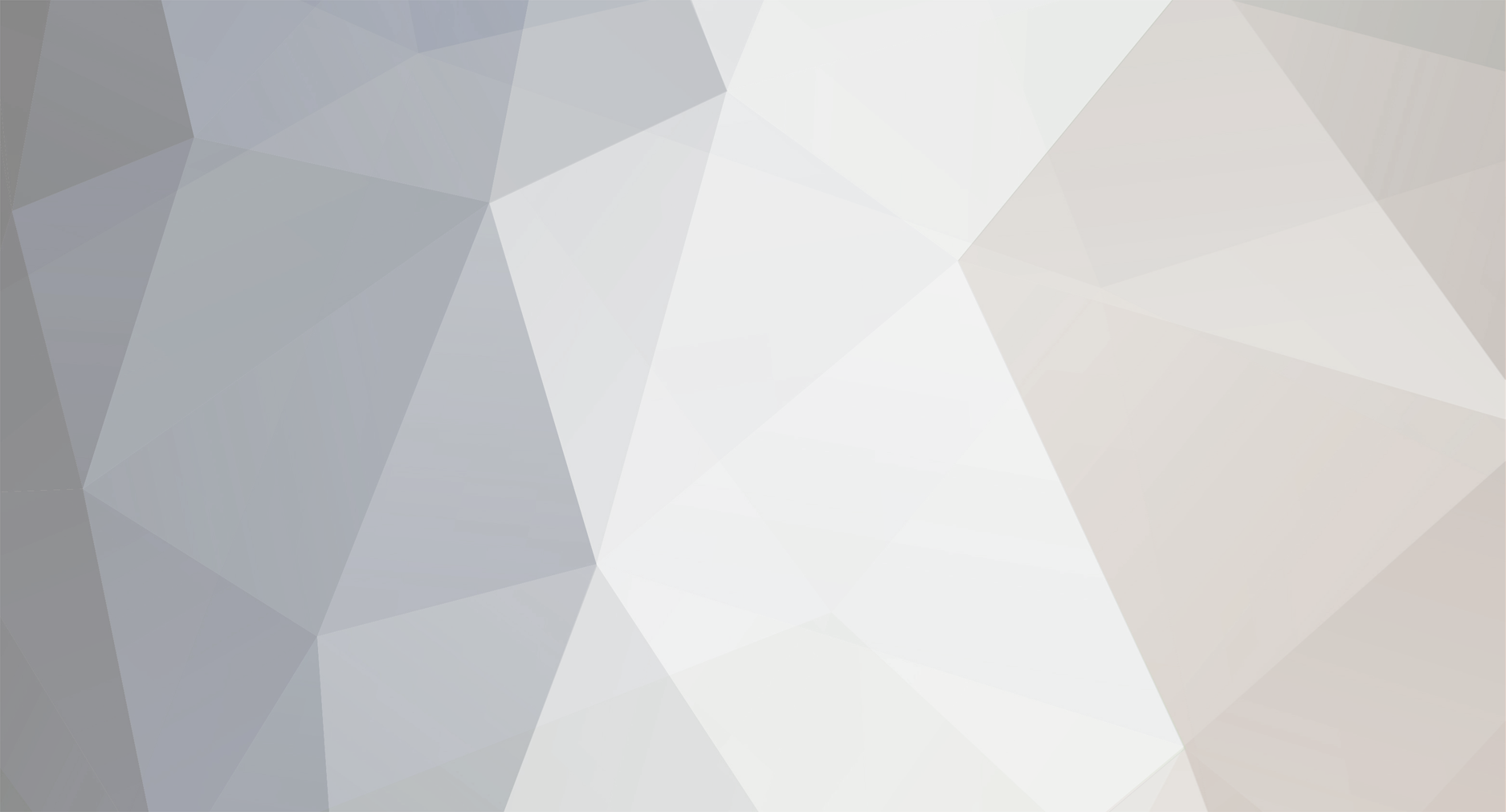
waynew
Members-
Posts
2,405 -
Joined
-
Last visited
Everything posted by waynew
-
writes to the database but does not upload file
waynew replied to sandbudd's topic in PHP Coding Help
If the files are too large, then the settings in your php.ini file could be restricting them. -
Could you give us all the schema (structure - column types etc) of your database table? In PHP, you can get the current timestamp by using the function mktime() Then, you could add whatever seconds onto the current timestamp.
-
You'll need a datetime column in your blog post table. date_posted DATETIME NOT NULL When inserting a new blog post into your database table, you'll need to give the datetime, which is: $date_posted = date("Y-m-d H:i:s"); When selecting from your blog post table, you'll need to order it like so: $result = mysql_query("SELECT * FROM blog ORDER BY date_posted DESC") or trigger_error(mysql_error()); If you're only wanting to show the first 35 characters of something, do this (assuming the main text of the blog post is in a column called "body") <?php while($row = mysql_fetch_assoc($result)){ if(strlen(trim($row['body'])) > 35){ echo substr($row['body'],0,35).' [...]'; } else{ echo $row['body']; } } ?>
-
writes to the database but does not upload file
waynew replied to sandbudd's topic in PHP Coding Help
Have you got error reporting and notices on? -
Change $_SESSION['username'] = '$username'; $_SESSION['password'] = '$password'; to $_SESSION['username'] = $username; $_SESSION['password'] = $password; In your code, you're encapsulating your variables in single quotes. In PHP, single quotes will give you the literal of what is between those single quotes, meaning your username and password session variables will always literally be $username and $password and not what is actually in those variables. As opposed to double quotes. Also - why are you storing their password in a session variable? That is a major security concern.
-
writes to the database but does not upload file
waynew replied to sandbudd's topic in PHP Coding Help
Is your script slow? Or is just not showing up on your FTP client straight away? -
To assign a variable to a $_SESSION variable, you need to do something like this: <?php //assuming $username has been gotten from the DB $_SESSION['username'] = htmlentities($username,ENT_QUOTES,"utf-8"); ?> In your code, you're not assigning anything to your session variables.
-
I'm guessing that the OP is only new to PHP and therefore doesn't know much about common security risks such as XSS.
-
Yes. But make sure that you use htmlentities in order to sanitise your output. You don't want the user entering HTML or JavaScript as their username, only to see it being executed everytime your script outputs the username. echo htmlentities($_SESSION['username'],ENT_QUOTES,"utf-8"); You should also make sure that the session variable actually exists: <?php if(isset($_SESSION['username'])){ //print it out } ?>
-
writes to the database but does not upload file
waynew replied to sandbudd's topic in PHP Coding Help
Also please note that are two main security flaws in your script. 1: You're not checking to see if the filetype is acceptable, which means that any old file could be uploaded to your server. What if somebody uploaded a .php file? They'd be able to upload it to your server and then execute it. 2: You're not using the function is_uploaded_file(). is_uploaded_file() makes sure that the file in question has actually been uploaded. Otherwise, an attacker could give you the file location of a sensitive file outside your root... only to have that sensitive file copied to a publicly viewable location. -
writes to the database but does not upload file
waynew replied to sandbudd's topic in PHP Coding Help
Try this and see what happens: <?php //This is the directory where images will be saved $target = 'images/'.$_FILES['photo']['name']; //This gets all the other information from the form $pic= $_FILES['photo']['name']; // Connects to your Database mysql_connect("", "", "") or die(mysql_error()) ; mysql_select_db("") or die(mysql_error()) ; //Writes the information to the database mysql_query("INSERT INTO `alabama` VALUES ('$pic')") ; //Writes the photo to the server if(move_uploaded_file($_FILES['photo']['tmp_name'], $target)) { //Tells you if its all ok echo "The file ".$_FILES['photo']['name']. " has been uploaded, and your information has been added to the directory"; } else { //Gives and error if its not echo "Sorry, there was a problem uploading your file."; } ?> -
I added lines this time: <?php $charset = 'A,B,C,D,E,F,G,H,K,L,M,N,P,R,S,T,U,V,W,Y,Z,1,2,3,4,5,6,7,8,9,!'; $char_array = explode(",",$charset); $size_of_array = sizeof($char_array) - 1; $num_chars_in_capthca = 6; $captcha_string = ""; $i = 0; while($i < $num_chars_in_capthca){ $index = rand(0,$size_of_array); $captcha_string .= $char_array[$index]; $i++; } $_SESSION['captcha_code'] = $captcha_string; $img_number = imagecreate(125,50); $backcolor = imagecolorallocate($img_number,000,000,000); $textcolor = imagecolorallocate($img_number,255,255,255); $linecolor = imagecolorallocate($img_number,232,128,240); imagefill($img_number,0,0,$backcolor); imagestring($img_number,10,35,17,$captcha_string,$textcolor); $num_lines = 3; $i = 0; while($i < $num_lines){ imageline ($img_number,rand(1,62),rand(1,25),rand(63,125),rand(26,50),$linecolor); $i++; } header("Content-type: image/jpeg"); imagejpeg($img_number); ?>
-
I made your captcha a little bit tougher: <?php $charset = 'A,B,C,D,E,F,G,H,K,L,M,N,P,R,S,T,U,V,W,Y,Z,1,2,3,4,5,6,7,8,9,!'; $char_array = explode(",",$charset); $size_of_array = sizeof($char_array) - 1; $num_chars_in_capthca = 6; $captcha_string = ""; $i = 0; while($i < $num_chars_in_capthca){ $index = rand(0,$size_of_array); $captcha_string .= $char_array[$index]; $i++; } $_SESSION['captcha_code'] = $captcha_string; $img_number = imagecreate(75,25); $backcolor = imagecolorallocate($img_number,0xcc,0xcc,0xcc); $textcolor = imagecolorallocate($img_number,255,255,255); imagefill($img_number,0,0,$backcolor); imagestring($img_number,3,5,5,$captcha_string,$textcolor); header("Content-type: image/jpeg"); imagejpeg($img_number); ?>
-
file_get_content() isn't a function (you're thinking about file_get_contents()) so you'd get a syntax error. Other than that, I can't see anything else wrong with what you're doing.
-
Instead of foreach($email_message as $e) { $email_message .= $e."\n"; } You're appending the array value to the actual array? <?php $message = implode("\n",$lines_array); ?>
-
Firstly; why are you doing this? Are you trying to prove that you can? If you do follow through with this and store the number in a file, you're going to have to make sure that you lock the file in question while you're updating it, as you don't want two updates happening concurrently. But I have to say that I just don't see the point in what you're doing.
-
Use a hidden field: <form action = "postage.php" method = "POST"> <input name="total_price" type="hidden" value="<?php echo $total_price; ?>" /> <center> <input type = "submit" value = "procceed to checkout" name= "grandT"> </center> <form> But bear in mind that you will have to check to see if $_POST['total_price'] is the correct price (on postage.php) as users could tamper with the form values before submitting it.
-
I should probably just go with BBC then. What Hotmail and Gmail etc does is their own business. As long as the system is sending emails and the server isn't being stretched by an overuse of the mail function, things should be fine.
-
What is going wrong? What do you expect to happen? And what is actually happening? Also, you didn't post the entire code block. What's in that last else statement?
-
I have to create a newsletter system for a client. Problem is, I don't want to rape the mail() function. How should I go about doing this? Keeping in mind that looping the mail function a thousand times isn't really a solution.
-
IE 9 only 3 weeks old, and already increases acid test 3 results
waynew replied to nrg_alpha's topic in Miscellaneous
IE still rules all. -
Doesn't changing DB encoding mid-connection create a security hazard?
-
By placing a user_id in the words column and then linking that word back to the specific user? I'm not 100% sure what you're talking about.
-
That's what she said. Yes Gayner; I'm sure she did.
-
Code injected into website - is there a program to remove it?
waynew replied to tibberous's topic in Miscellaneous
As a student - I'm not willing to pay $30-60 a month.