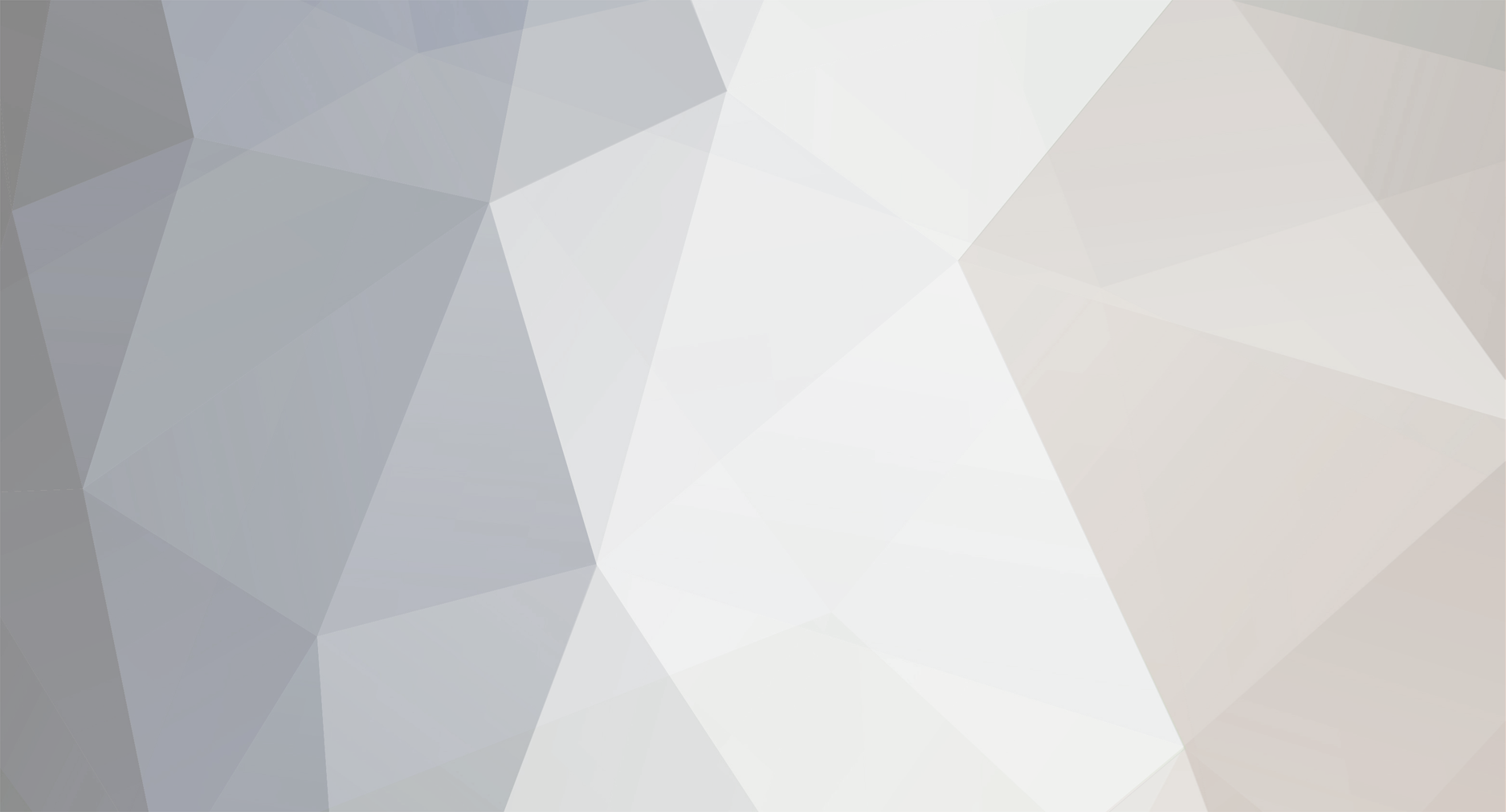
EternalSorrow
Members-
Posts
185 -
Joined
-
Last visited
Never
Everything posted by EternalSorrow
-
Find Links: str_replace vs. preg_replace
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Thanks for the tip! I also am at a loss on how to handle the regex, so anyone have any ideas? Tutorials? Further tips? -
I'm currently working on a not-so-simple way of displaying archived news information. Because the archive page is in a sub-folder whereas the news is normally placed in the main folder (this is for aesthetic purposes), I've run into a strange problem I'm having with the links. All the links point to the correct folders when the news is in the main folder, but since the archive page is in a sub-folder, I need to place double-period-slash (../) in front of all links to point them in the right direction. The links are strewn about the field $body, and this is where I need help. I need to be able to find any instance of <a href=" and replace is with <a href="../ so all links will point to the correct folder. I also need to find all links which have counter.php as their destination so I can make an exception to the double-period-slash rule (that page is in the same folder as the archive page, so it still points to the correction destination). I can't wrap my head around whether I need to use str_replace or preg_replace in these instances, or whether both are necessary. I also have the added difficulty of a preg_replace currently being used for the $body field to insert paragraph tags into the field. Anyone have any ideas or tutorials on how to solve this dilemma? Here's the short code for reference: <?php $query = "SELECT a.*, b.* FROM blog a INNER JOIN blog_catjoin c ON a.entry_id = c.entry_id INNER JOIN blog_category b ON b.fl_id = c.cat_id WHERE a.entry_id = '$entry_id2' GROUP BY a.entry_id ORDER BY a.entry_id DESC LIMIT 4"; $result = mysql_query($query) or die(mysql_error()); while ($row4 = mysql_fetch_array($result)) { extract($row4); $select_category = mysql_query("SELECT * FROM blog_category AS b INNER JOIN blog_catjoin AS c ON b.fl_id = c.cat_id WHERE c.entry_id = $entry_id ORDER BY b.fl_subject ASC ") or die (mysql_error()); $cat = ""; while ($row2 = mysql_fetch_array($select_category)) { $cat .= "<a href=\"archive_category.php?a=show&fl_subject=$row2[fl_subject]\">$row2[fl_subject]</a>, "; } $cat = substr($cat,0,-2); $select_table = "SELECT *, date_format(blog.added, '%M %d, %Y') AS datetime FROM blog WHERE entry_id = $entry_id "; $select_results = mysql_query($select_table) or die (mysql_error()); $tableArray = mysql_fetch_array($select_results) or die (mysql_error()); $body = preg_replace('#\r+#', '</p><p>', $body); echo '<div style="overflow: hidden;"> <h3>'.$title.'</h3> '.$body.' <p><b>Categories:</b> '.$cat.' <br><b>Added:</b> '.$tableArray['datetime'].' </div>'; } } ?>
-
SUBSTRING Field - treat as 'real' field?
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I removed the alphabetical listing from the second query, which holds the WHERE statement, because it needs to parse through all results from the 'design' table. The problem I'm now having is that the result from first code, which is all the rows of the table, is being retrieved by the second code, so all rows are being retrieved and displayed in the second code, too. I need only the rows corresponding to the $letter to be shown. Removing the first code (the alphabetical listing) fixes the problem, but deletion isn't a good solution in this case. My question is: how can I get the second code to retrieve the correct $letter from the URL while also being able to display the alphabetical list at the top? -
SUBSTRING Field - treat as 'real' field?
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
The wild card WHERE option worked the best for the problem, as I'm not even sure how I could implement the HAVING clause in this example, but now I've run into another snag concerning the alphabetical listing versus the listing of relevant rows for the first letter. I would like the alphabet listing above where the retrieved rows, but because all letters are then listed and there is a wild card for the WHERE, more than just the single letter in the URL bar is retrieved. Is there any way for the second code (as I've split the alphabetical listing and letter query) to ignore the first snippet, or should I perhaps implement a (currently unknown) HAVING clause? Here's the modified code: <?php $sql = "SELECT *, UPPER(SUBSTRING(series,1,1)) AS letter FROM design ORDER BY series"; $query = mysql_query ($sql) or die (mysql_error()); echo '<ul class="alphabet">'; while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['series']] = $records['series']; } foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="series.php?letter='.$i.'">'.$i.'</a></li>' : '<li class="no_highlight">'.$i.'</li> '; echo ($i != 'Z') ? ' ':'</ul><h3>'.$letter.'</h3>'; } ?> <?php if (!is_numeric($_GET["letter"]) && !empty($_GET["letter"]) && $_GET["letter"]!="") { $letter = $_GET["letter"]; } $sql = "SELECT *, UPPER(SUBSTRING(series,1,1)) AS letter FROM design WHERE series LIKE '$letter%' ORDER BY series LIMIT 1"; $query = mysql_query ($sql) or die (mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['series']] = $records['series']; } foreach(range('A','Z') as $i) { if (array_key_exists ("$i", $alpha)) { foreach ($$i as $key=>$value) { $select_design = mysql_query("SELECT * FROM design WHERE series = '$value' ORDER BY id ASC ") or die (mysql_error()); $cat = ""; while ($row2 = mysql_fetch_array($select_design)) { $cat .= "<li><a href=\"graphics/preview.php?id=$row2[id]\"><img src=\"previews/$row2[image]\"></a></li> "; } $value = '<a name="'.$i.'"></a><h3>'.$value.'</h3><ul>'.$cat.'</ul>'; echo ''.$value.''; } } } ?> -
I'm piecing together a code which involves an alphabetized list and the ability to sort the table rows by first letter and series. This page is accessed by a user clicking on a link with the corresponding first letter of the series, a field which is created using the SUBSTRING command in the query statement. The problem I have is I would like to use the first letter (in my code simply named 'letter') to sort the table using the WHERE clause. However, I receive an error message stating the following: The above statement is true, as in my table there really isn't a field by the name of letter. However, I still need to sort the query by the first letter so when visitors click the link, they will be shown the page revealing all series beneath the relevant letter. Anyone have any idea how to make the 'imaginary' field $letter usable in a WHERE clause? Here's the code which produces the error effect: <?php if (!is_numeric($_GET["letter"]) && !empty($_GET["letter"]) && $_GET["letter"]!="") { $letter = $_GET["letter"]; } $sql = "SELECT *, UPPER(SUBSTRING(series,1,1)) AS letter FROM design WHERE letter = '$letter' ORDER BY series LIMIT 4"; $query = mysql_query ($sql) or die (mysql_error()); echo '<ul class="alphabet">'; while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['series']] = $records['series']; } foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="series.php?letter='.$i.'">'.$i.'</a></li>' : '<li class="no_highlight">'.$i.'</li> '; echo ($i != 'Z') ? ' ':'</ul><h3>'.$letter.'</h3>'; } foreach(range('A','Z') as $i) { if (array_key_exists ("$i", $alpha)) { foreach ($$i as $key=>$value) { $select_design = mysql_query("SELECT * FROM design WHERE series = '$value' ORDER BY id ASC ") or die (mysql_error()); $cat = ""; while ($row2 = mysql_fetch_array($select_design)) { $cat .= "<li><a href=\"graphics/preview.php?id=$row2[id]\"><img src=\"previews/$row2[image]\"></a></li> "; } $value = '<a name="'.$i.'"></a><h3>'.$value.'</h3><ul>'.$cat.'</ul>'; echo ''.$value.''; } } } ?>
-
I'm currently trying to create a script for my design table from scratch, and I've run into an early problem. I have three different types for my designs, and each one has their own respective designs. My problem is on the index page I want to use a single script to show all three types, with three of their designs listed beneath each header type. It would appear something like this: What I'm having problems with is the initial query. I need to either group the types together, or use the DISTINCT command to group them together. The first difficulty is that with the DISTINCT command, my second query which gathers together and lists the respective designs won't have anything but the type field from which to retrieve table information, so I get a random picture for all results shown. The second difficulty is in using the GROUP BY command. Grouping by the type means the second query will only see one design for each type, because all others have been eliminated because of the common type. In summary, I need to group the designs under their respective types, but I can't figure out how to accomplish that with the code I have. Does anyone have any suggestions on how to get around this problem? Here's the code for reference: <?php $query = "SELECT * FROM design GROUP BY type ORDER BY type ASC"; $result = mysql_query($query) or die (mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); $select_single = mysql_query("SELECT * FROM design WHERE type = '$type' LIMIT 3"); $cat = ""; while ($row2 = mysql_fetch_array($select_single)) { $cat .= "<td><a href=\"graphics/preview.php?id=$id\"><img src=\"previews/$image\" class=\"tips\" title=\"$title|$series\" alt=\"\"></a>"; } echo '<ul class="head"> <li class="header">'.$type.'</li> <li class="last"><a href="type.php?type='.$type.'">View More?</a> </ul> <table align="center" cellpadding="6"><tr> '.$cat.' </table>'; } ?>
-
I'm currently trying to implement the cluetip jquery plugin into my site to use for my images. I can implement a stationary code no problem, but what I'm now trying to accomplish is use of the experimental mouse tracking. The example they give uses ajax, when I prefer to use the plugin without ajax. I've been able to create a mouse tracking title using the demo provided, but whenever I try to eliminate the rel in the link, the script stops working. Applying 'tracking' as 'true' to the stationary, working image does nothing, making think the code is somehow dependent upon there being both a link and the use of ajax. Anyone know how I might be able to use the mouse tracking without ajax?
-
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I have many questions about the data. They are in bold and are above the confusing area. Most of them deal with the fact that the code appears to have no connection with, or may be different from, previous code snippets I've given. The code also doesn't appear to group the now separated rows under the artificial character pound (#), which makes me wonder what heading they will be under in the fulling listing. Can this simply be done by allowing $symbols to equal pound (#), or am I simplifying this too much? -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Could you explain what the code is trying to do line by line? I truly can't figure out how to integrate the new foreach into the current snippet because I'm not sure where to the place the small code. -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Your question may have lost me, but I'll rephrase my previous explanation while defining the entirety of the code. The current code takes all first characters for the titles and assigns them as headers for an ordered list (under the <h3> tag). Then those titles which begin with that character are listed beneath the header character. Here's a sample table: So the output currently looks like this: What I want, however, is for the titles which begin with characters that are not letters to be grouped into a single category (represented by the pound (#)), so the total output would instead look like this: Possibly some sort of array would work which listed the non-alphabetical characters and then an IF statement used in the final foreach to find those titles which started with non-alphabetical characters and grouped them under the pound (#) character, but I'm just guessing here. And here's a slightly modified code (got rid of one now-useless line): <?php $sql = "SELECT *, UPPER(SUBSTRING(title,1,1)) AS letter FROM feudal WHERE `type` = 'Fanart' GROUP BY title, author ORDER BY title, author"; $query = mysql_query( $sql ) or die(mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']][] = $records; } echo '<ul class="alphabet"> <li><a href="#other">#</a></li>'; foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="#'.$i.'">'.$i.'</a></li>' : '<li>'.$i.'</li>'; } echo '</ul><ol class="listfanart">'; foreach($alpha as $i => $r) { echo '<a name="'.$i.'"></a><h3>'.$i.'<div style="font-size: 10px; float: right;"><a href="#">top</a></h3>'; foreach ($r as $records) { $url = $records['url']; $key = $records['author']; $value = $records['title']; echo '<li><a href="'.$url.'" target="_blank">'.$value.'</a> by '.$key.'</li> '; } } ?> -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
What I'm aiming for is an array which lists all ascii characters which are not letters, and if a row happens to start with one of those characters they would be placed under a heading called #. Here's an example: and have the output be like this: -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
That code actually greatly improved the previous snippet by allowing all ascii characters to be shown rather than just those which started with a letter. Thanks a bunch for the improvement! Now another question (hopefully my last): I have several rows which start with ascii characters such as plus signs (+), numbers, and colons. Is there any way for me to group specified characters under a single symbol, such as the pound (#)? Here's the new code as it now stands: <?php $sql = "SELECT *, UPPER(SUBSTRING(title,1,1)) AS letter FROM feudal WHERE `type` = 'Fanart' GROUP BY title, author ORDER BY title, author"; $query = mysql_query( $sql ) or die(mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']][] = $records; } echo '<ul class="alphabet"> <li><a href="#other">#</a></li>'; foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="#'.$i.'">'.$i.'</a></li>' : '<li>'.$i.'</li>'; } echo '</ul><ol class="listfanart">'; foreach($alpha as $i => $r) { // if (array_key_exists ("$i", $alpha)) { echo '<a name="'.$i.'"></a><h3>'.$i.'<div style="font-size: 10px; float: right;"><a href="#">top</a></h3>'; foreach ($r as $records) { $url = $records['url']; $key = $records['author']; $value = $records['title']; echo '<li><a href="'.$url.'" target="_blank">'.$value.'</a> by '.$key.'</li> '; } } ?> -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Anyone have any ideas? -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I am remotely familiar with arrays (translation: barely grasping the concept), but to be honest I've never heard of using a '2d array' nor making an 'object.' How does one do these things (especially with the current mess I've given as code)? -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Isn't that a little like rearranging the deck chairs on the Titanic? The problem isn't with the existing variables, but the fact that the $url field has no correlation to either $value or $key ($title and $author, respectively). What I need is to find is some way to relate the $url field to the $record which is outputted in the final echo of the code. -
Inserting Another Field - relational trouble
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
How would I do this without interfering with the intent of the code? -
I've created an alphabetical listing code which more or less works, but I would like to expand on the information it outputs to the viewer. Currently it only outputs two fields, the $title and $author. I would also like to add the $url field to the output, but simply adding this: doesn't created a relationship to the outputted information. What that means if I do insert the $url into the echo, it will simply retrieve the $url for the final outputted row and assign that to all of the outputted lists, like so: table title1, author1, url1 title2, author2, url2 title3, author3, url3 Right now it outputs this: How would I create a relationship between the outputted rows and their respective $url using the code below? <?php $sql = "SELECT *, UPPER(SUBSTRING(title,1,1)) AS letter FROM feudal WHERE `type` = 'Fanart' GROUP BY title, author ORDER BY title"; $query = mysql_query( $sql ) or die(mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['author']] = $records['title']; $url= $records['url']; } echo '<ul class="alphabet"> <li><a href="#other">#</a></li>'; // Create Alpha link Listing foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="#'.$i.'">'.$i.'</a></li>' : '<li>'.$i.'</li>'; } echo '</ul><ol class="listfanart">'; // Create Data Listing foreach(range('A','Z') as $i) { if (array_key_exists ("$i", $alpha)) { echo '<a name="'.$i.'"></a><h3>'.$i.'<div style="font-size: 10px; float: right;"><a href="#">top</a></h3>'; foreach ($$i as $key=>$value) { echo '<li><a href="'.$url.'" target="_blank">'.$value.'</a> by '.$key.'</li> '; } } } ?>
-
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Well, perhaps a change in code is necessary considering all the variety of ascii characters which are housed in the database (everything from a period to parenthesis, which means ascii 32 through 90 will be needed). I've managed to get a page set up from a tutorial I found here. It does have advice on how to deal with those odd ascii characters at the end, but I cannot figure out how to implement that into the main list. Anyone have any tutorials or advice on how to implement this bit of code: for ($i=32; $i< =90; $i++) { $letter = chr($i); ...code... } into the main code below?: <?php $sql = "SELECT *, UPPER(SUBSTRING(title,1,1)) AS letter FROM feudal WHERE `type` = 'Fanart' GROUP BY title, author ORDER BY title LIMIT 50"; $query = mysql_query( $sql ) or die(mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['author']] = $records['title']; } echo '<ul class="alphabet"> <li><a href="#other">#</a></li>'; // Create Alpha link Listing foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<li><a href="#'.$i.'">'.$i.'</a></li>' : '<li>'.$i.'</li>'; } echo '</ul><ol class="listfanart">'; // Create Data Listing foreach(range('A','Z') as $i) { if (array_key_exists ("$i", $alpha)) { echo '<a name="'.$i.'"></a><h3>'.$i.'<div style="font-size: 10px; float: right;"><a href="#">top</a></h3>'; foreach ($$i as $key=>$value) { echo '<li><a href="" target="_blank">'.$value.'</a> by '.$key.'</li> '; } } } ?> </ol> -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I did find this tutorial which on the last page addresses special characters using ASCII, but I am not sure how to implement it into the current code (always a problem of mine). -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I'm afraid it still repeats incorrectly (skipping first, etc.). -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I placed the echo within the IF statement but the results are still the same. Here's the code as it now stands (now minus the $query, which hasn't changed): $words = array('1','2','3','4','5','6','7','8','9','+',':'); $firstLetter = ''; while ($row = mysql_fetch_assoc($result)) { $url = $row[url]; $author = $row[author]; $title = $row[title]; if(in_array($firstLetter,$words)){ if($firstLetter != '#'){ $firstLetter = '#'; echo '<h3><a name="'.$firstLetter.'">'.$firstLetter.'</a></h3>'; } } else if (ucwords($firstLetter) != ucwords($row['firstLetter'])) { //Assign category to holding var $firstLetter = $row['firstLetter']; //echo firstLetter heading echo '<h3><a name="'.$firstLetter.'">'.$firstLetter.'</a></h3>'; } -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
The results are still (frustratingly) repeating. -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
The code produced some strange results. As far as I can tell the $words skips the first instance of the special character or number, but rightly adjusts the second. It repeats this pattern, like this: Table +miss +this +yes +zis Those lines read like this: I am not really sure why the code would be skipping every other instance. It does, however, work when a single instance occurs. -
Alphabetical Organizing - some snags
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
That worked perfectly, thanks for the tip. Now does anyone have any idea on how to manage the special characters and numbers? I'm thinking some sort of IF statement where if the first letter isn't alphabetical they can be grouped, or using regex (always tricky, though), but I've never seen any examples explaining how this might be done. -
I've got a code which allows me to take the first letter of every title in a database and create an alphabetical listing, like so: Table 'feudal' holds these titles: + some guys 3000 Guys bed bugs Bed bugs The titles are correctly assigned to their proper titles and outputted on the page, but too thoroughly. The code differentiates between capital and lowercase letters, when I want all letters to be grouped together. Here's an example: What I want: What I get: The other problem I have is some of the titles start with characters and numbers, which are then given their own heading. I would like those characters andnumbers to fall under a generic symbol, such as the pound (#). Here's an example: What I want: What I get: So in summary, can anyone help me figure out how to group upper and lower case letters together, and how to place all characters and numbers under a single heading? Here's the code for reference (minus connection, of course): <?php $query = "SELECT *, substring(`title`,1,1) AS firstLetter FROM feudal WHERE `type` = 'Fanart' GROUP BY title, author ORDER BY title"; $result = mysql_query( $query ) or die(mysql_error()); $firstLetter = ''; while ($row = mysql_fetch_assoc($result)) { $url = $row[url]; $author = $row[author]; $title = $row[title]; if ($firstLetter != $row['firstLetter']) { //Assign category to holding var $firstLetter = $row['firstLetter']; //echo firstLetter heading echo '<h3><a name="'.$firstLetter.'">'.$firstLetter.'</a></h3>'; } echo '<li><a href="'.$url.'" target="_blank">'.$title.'</a> by '.$author.'</li>'; } ?>