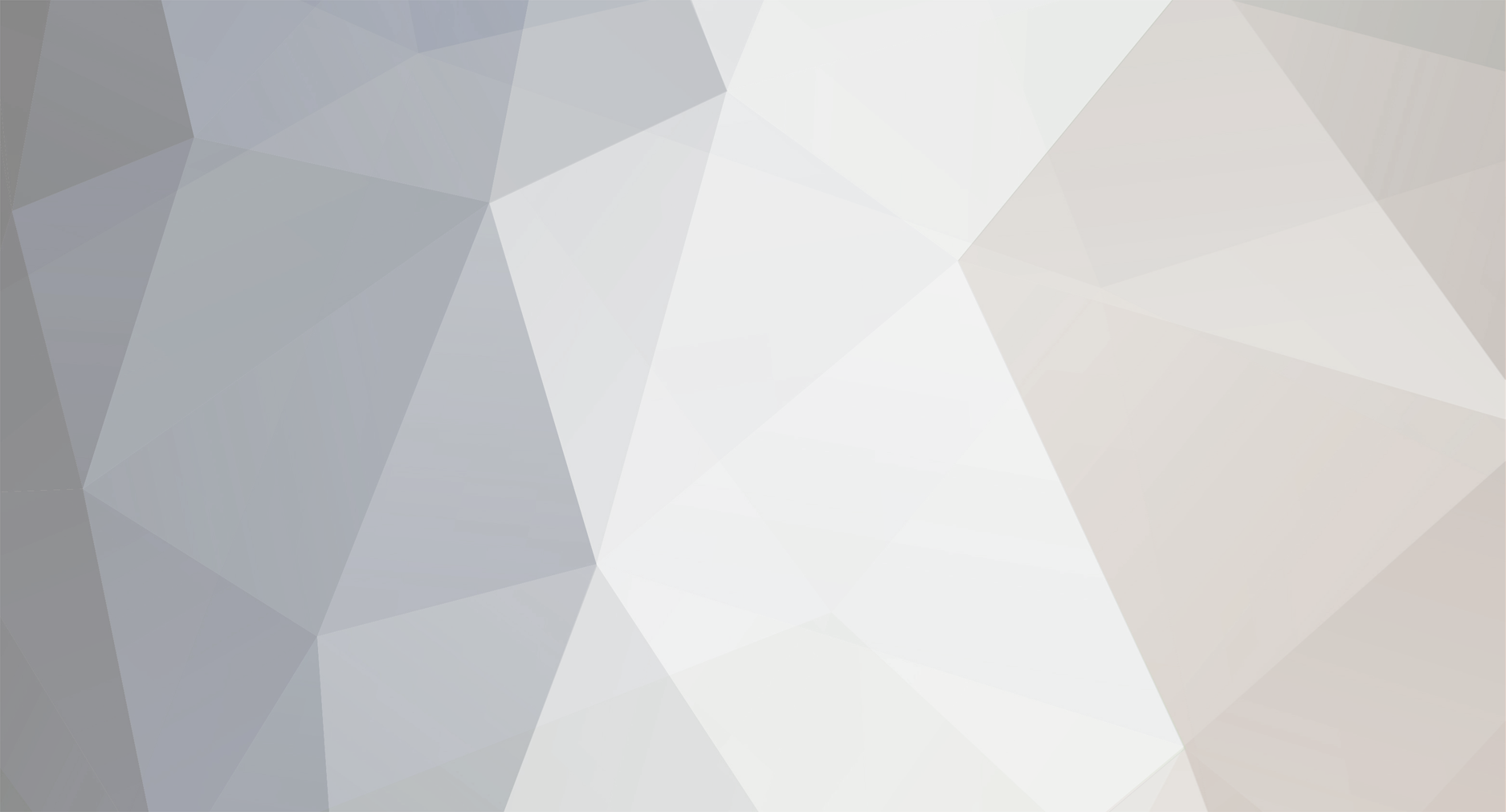
EternalSorrow
Members-
Posts
185 -
Joined
-
Last visited
Never
Everything posted by EternalSorrow
-
[SOLVED] IF Statement & Two Tables With Different Echoes
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I pieced together what I had with what you recommended, but for some reason no results will show on the page, nor is there an error message. When I place in a simple echo in place of the IFs the results do show. I've tried adjusting the CONDITION using various methods, but all failed to show anything other than an error message or a blank page. For reference, here's the full code (minus connection): <?php $query = "SELECT title, image, year, id, 'film' AS table1 FROM film UNION ALL SELECT name, img, id, occupation, 'people' AS table2 FROM people ORDER BY id DESC LIMIT 5 "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); if ($result[table1] == 'film') { echo '<li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li>'; } if ($result['table2'] == 'people') { echo '<li><a href="biography.php?name='.$name.'"><img src="images/thumbnails/'.$img.'.jpg" alt="" title="'.$name.' ('.$occupation.')"></a></li>'; } } ?> -
[SOLVED] IF Statement & Two Tables With Different Echoes
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I understand that I now have the defining name for each of the tables, but I'm still in the dark about how to write the condition in which I can identify the table and assign the correct echo to it. Anyone have any code snippets or links for me to learn from? -
[SOLVED] IF Statement & Two Tables With Different Echoes
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
If I'm using the IF statement as I've shown, with all the information passing through a single IF statement and then being either ignored to go to the ELSE or being implemented, then there shouldn't be any need for two IF statements. The problem I have is not how many IF statements to use but how to define the IF statement to find the table which the row has originated. -
I'm retrieving two tables in a single query using UNION ALL, like so: $query = "SELECT * FROM film UNION ALL SELECT * FROM people ORDER BY id DESC LIMIT 5 "; Now each of those tables, FILM and PEOPLE create links to two different pages for the different information stored in the said tables. Here are the two different echoes for the tables: FILM: <li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li> PEOPLE: <li><a href="biography.php?name='.$name.'"><img src="images/thumbnails/'.$img.'.jpg" alt="" title="'.$name.' ('.$occupation.')"></a></li> Is there an IF statement, or anything else for that matter, which will identify which table each row comes from and then assign the necessary link to that row, like so?: Here's my poor attempt at managing it (obviously doesn't work): <?php mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = "SELECT * FROM film UNION ALL SELECT * FROM people ORDER BY id DESC LIMIT 5 "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); $field_array = mysql_fetch_array($result); if (!in_array(`img`, $field_array)) { echo '<li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li>'; } else echo '<li><a href="biography.php?name='.$name.'"><img src="images/thumbnails/'.$img.'.jpg" alt="" title="'.$name.' ('.$occupation.')"></a></li>'; } ?>
-
[SOLVED] PHP, Arrays & A Lot Of Confusion
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
Since it was apparent AJAX was going to be difficult with what I wanted to do with the $query, I settled for another content slider I found at Tutorialzine. The script is much more adept at user customization and I found I could place the $query statement within the page the slider was in, thus solving my database problem. -
[SOLVED] PHP, Arrays & A Lot Of Confusion
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
That did the trick wonderfully, but I've realized another problem which deals with my $query. I have the $query retrieving information from the database based upon the name of an individual who's page is being viewed, like so: $query = "SELECT * FROM related LEFT JOIN film ON related.title = film.title WHERE related.name = '$name' "; Because the PHP is being remotely pulled rom a separate page than the person's page, the $query cannot return any information based upon that WHERE statement. Is there any way to connect the separate PHP page to the page in which the jCarousel is embedded to make the WHERE statement functional? -
I've been doing basic PHP work on sites for a few years now, but I've never been tempted to learn how the ARRAY function worked, nor in what instances to use it. Now I've stumbled upon the jCarousel script, and I need to know how to use the ARRAY function to populate the jquery plugin. I have the dynamically driven AJAX version of the plugin downloaded and the example images work fine in the php file which is accessed by the AJAX. The only problem is I have no idea how to integrate the PHP coding I was using into the new PHP file which calls for the images to be placed (apparently manually?) into an array, like so: <?php // Array indexes are 0-based, jCarousel positions are 1-based. $first = max(0, intval($_GET['first']) - 1); $last = max($first + 1, intval($_GET['last']) - 1); $length = $last - $first + 1; // --- $images = array( 'http://static.flickr.com/66/199481236_dc98b5abb3_s.jpg', 'http://static.flickr.com/75/199481072_b4a0d09597_s.jpg', 'http://static.flickr.com/57/199481087_33ae73a8de_s.jpg', 'http://static.flickr.com/77/199481108_4359e6b971_s.jpg', 'http://static.flickr.com/58/199481143_3c148d9dd3_s.jpg', 'http://static.flickr.com/72/199481203_ad4cdcf109_s.jpg', 'http://static.flickr.com/58/199481218_264ce20da0_s.jpg', 'http://static.flickr.com/69/199481255_fdfe885f87_s.jpg', 'http://static.flickr.com/60/199480111_87d4cb3e38_s.jpg', 'http://static.flickr.com/70/229228324_08223b70fa_s.jpg', ); $total = count($images); $selected = array_slice($images, $first, $length); // --- header('Content-Type: text/xml'); echo '<data>'; // Return total number of images so the callback // can set the size of the carousel. echo ' <total>' . $total . '</total>'; foreach ($selected as $img) { echo ' <image>' . $img . '</image>'; } echo '</data>'; ?> Here's the PHP code I was using to display the images: <?php mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = "SELECT * FROM related LEFT JOIN film ON related.title = film.title WHERE related.name = '$name' "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); echo '<li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li>'; } ?> Is anyone able to explain to me how I'm supposed to integrate the above PHP script into the array? Am I going about this all wrong and need to dramatically change the first snippet of code in order to access the database and retrieve the data in the format I want? I sincerely apologize if I sound desperate, but after hours of looking at examples of arrays I still have no idea how I am supposed to use the code from the jCarousel in conjunction with my typical PHP style. Any help would be most appreciated.
-
Three Tables - Maybe Unusual Combination?
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
The problem with that code is it won't choose people from the PEOPLE table who are related to the film; it will only retrieve the person who's link was clicked. Like so: What appears: And what should appear: I've been playing around with the coding since I posted, and was able to create two separate queries which output the total result I want. I've tried combining them, but when I do the PEOPLE table always duplicates the number of those people associated with the film and places the linked person's information in their places, like so: It should be like this: Does anyone have any ideas on how I can successfully join these two queries into one? <?php mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = "SELECT * FROM related LEFT JOIN film ON related.title = film.title WHERE related.name = '$name' "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); echo '<li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li>'; } ?> <?php mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = "SELECT * FROM related LEFT JOIN people ON related.name = people.name WHERE `title` = '$title' AND people.name != '{$name}' "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); echo '<li><a href="biography.php?name='.$name.'"><img src="images/thumbnails/'.$img.'.jpg" alt="" title="'.$name.' ('.$occupation.')"></a></li>'; } ?> -
[SOLVED] Field - Subtract First Word
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
We'll see how long the first post suggestion works on the site. Thanks for all the help and the great learning experience everyone! -
I'm setting up three tables, each with their own use: FILM table (used to store information on films): id title year info image PEOPLE table (used to store information on films): id name occupation bio image RELATED table (used to connect films to people): title name So those are the layouts of the three tables. I've connected the PEOPLE table to the FILM table, so that when someone clicks on a link of a person they can see a list of those films which relate to that person. Like so: <?php if (!is_numeric($_GET["name"]) && !empty($_GET["name"]) && $_GET["name"]!="") { $name = $_GET["name"]; } mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = "SELECT * FROM related INNER JOIN film ON related.title = film.title WHERE related.name = '$name' "; $result = mysql_query( $query ) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); echo '<li><a href="filmography.php?title='.$title.'"><img src="images/thumbnails/'.$image.'.jpg" alt="" title="'.$title.' ('.$year.')"></a></li>'; } ?> Here's where it gets tricky: I want to also show those people who are also connected to the same films, so that when a visitor clicks on a person's link they will view not only related films but those people they are related to through those films. Here's an example: PEOPLE table holds these people: Merle Oberon Leslie Howard FILM table holds this row: The Scarlet Pimpernel RELATED table holds these rows: The Scarlet Pimpernel | Merle Oberon The Scarlet Pimpernel | Leslie Howard So here's what the visitor should see when they click a link to Merle Oberon's bio page: Click Merle Oberon link --> And view these related links: The Scarlet Pimpernel (movie) Leslie Howard (person) I cannot wrap my mind around whether to create a new $query statement to show the connection between PEOPLE of like FILM, or to create some type of JOIN in the existing $query which will find the connection. Any help and/or advice would be much appreciated.
-
I've been searching for several hours for an answer to this simple problem, but no luck, so I've come groveling here to seek guidance. All I want to know is when a $field is retrieved from the database, how can I subtract, skip, trim or essentially eliminate the first word from a string? Here's an example of what I want with the string of the $field and the output I'm going searching for: $string = "The Scarlet Pimpernel"; $output = "Scarlet Pimpernel"; Be aware the first word could be anything, not necessarily the word 'the.'
-
Apparently the best way to perform this is to do an inner query and set the series value to the $value field, such as this: $select_table = "SELECT * FROM series WHERE series = '$value'"; $select_results = mysql_query($select_table) or die (mysql_error()); $tableArray = mysql_fetch_array($select_results) or die (mysql_error());
-
Due to the lack of replies I scoured the internet for another option to listing the series, and found this code snippet. The only problem I now have is how to create a URL for each of the listed series which contains their ID, because I'd like for them to be linked as such: <a href="counter.php?id='.$id.'">'.$series.'</a> Merely using the above code, with the field $series replaced by the field $value, fetches the same random ID from the database for all listed entries. So my question is now: how do I make sure the id chosen for the $value is the actual ID related to the listed series? Here's the updated code: <?php $sql = "SELECT UPPER(SUBSTRING(series,1,1)) AS letter, series, id FROM series ORDER BY series"; $query = mysql_query ($sql) or die (mysql_error()); while ($records = @mysql_fetch_array ($query)) { $alpha[$records['letter']] += 1; ${$records['letter']}[$records['series']] = $records['series']; } foreach(range(6,6) as $i) { echo (array_key_exists ("$i", $alpha)) ? '<a href="#'.$i.'" title="'.$alpha["$i"].' results">#</a>' : "$i"; echo ($i != 'Z') ? ' | ':''; } foreach(range('A','Z') as $i) { echo (array_key_exists ("$i", $alpha)) ? '<a href="#'.$i.'" title="'.$alpha["$i"].' results">'.$i.'</a>' : "$i"; echo ($i != 'Z') ? ' | ':'</div>'; } foreach(range('6','6') as $i) { if (array_key_exists ("$i", $alpha)) { echo '<h2><a name="'.$i.'">#</a></h2>'; foreach ($$i as $key=>$value) { $value = '<a href="counter.php?id='.$id.'">'.$value.'</a>'; echo '<p>'.$value.'</p>'; } } } foreach(range('A','Z') as $i) { if (array_key_exists ("$i", $alpha)) { echo '<h2><a name="'.$i.'">'.$i.'</a></h2>'; foreach ($$i as $key=>$value) { $value = '<a href="counter.php?id='.$id.'">'.$value.'</a>'; echo '<p>'.$value.'</p>'; } } } ?>
-
I'm currently working on constructing a "counter page" which will update clicks for a link and reroute the visitor to the appropriate series page, but I've hit a snag. I want to use the series as the identifier for the counter page, and I use the correct link for the series, such as: <a href="counter.php?series='.$series.'">'.$series.'</a> but when the link is clicked I'm sent to an empty counter page. Apparently the counter php code isn't properly reading the series name and updating the information, but I can't figure out what the problem is because no mysql error message appears. So does anyone know what may be causing the problem in using a name to update the database hit counter and reroute visitors to the appropriate page? Here's the full code for the counter.php page: <?php mysql_connect(localhost,'name','pw'); @mysql_select_db(db) or die( "Unable to select database"); if(!isset($_GET['series']) || empty($_GET['series']) || !is_numeric($_GET['series'])) { die(mysql_error()); } else { $series=$_GET['series']; $query=mysql_query("SELECT series, count FROM series WHERE series='$series") or die(mysql_error()); if(mysql_numrows($query) == "0") { die(mysql_error()); } else { while($r=mysql_fetch_array($query)) { $clicks=$r[count]; $clicks++; mysql_query("UPDATE series SET count='$clicks' WHERE series='$r[series]'") or die(mysql_error()); header("Location: about.php?series=$r[series]"); } } } ?>
-
I found this nice snippet of code to limit the output of my series descriptions to the first sentence, but I've run into a snag. I want to be able to use the code in a "repeat region," but I receive this error message on the attempt: Is there any way to make this snippet of code repeat friendly? Here's what I have so far: <?php $query = "SELECT * FROM series WHERE category = 'Animanga' ORDER BY id desc LIMIT 2 "; $result = mysql_query($query); while ($row = mysql_fetch_array($result)) { extract($row); function first_sentence($plot) { $pos = strpos($plot, '.'); return substr($plot, 0, $pos+1); } echo '<li><a href="counter.php?id='.$id.'">'.$series.'</a><br> '.first_sentence($plot).''; } ?>
-
Having to post again since I can't seem to edit my previous posts. The pattern for the id appearances have changed, so here's what it's supposed to do: A ----- Ack - id=40 Aclaim - id=32 B ----- Bread - id=17 Bub - id=50 versus what it does: A ----- Ack - id=40 Aclaim - id=40 B ----- Bread - id=17 Bub - id=17 So now you see that it's taking the id of the first series in that alphabet letter and assigning it to them all within that letter.
-
Thanks for the reminder, can't believe how foolish that was. However, the inclusion of a URL is turning out to be quite difficult because of what I want to place in the URL. I want to place the series' ID numbers within the URL, as such: Unfortunately I can't seem to find a way to correlate the proper number to the proper series. Currently the only number retrieved is the one assigned is to the first series listed in the A section (for some reason the listing for 6 isn't the first id captured). So here's an example of my problem. The right way: A ----- Ack - id=40 Aclaim - id=32 B ----- Bread - id=18 Bub - id=50 And here's how it is showing: A ----- Ack - id=40 Aclaim - id=40 B ----- Bread - id=40 Bub - id=40 Any ideas on how to assign the correct id to its proper series? Here's the code as it now stands: <?php mysql_connect("localhost", "name", "pw"); @mysql_select_db(db) or die( "Unable to select database"); $sql = "SELECT SUBSTRING(series,1,1) as init, GROUP_CONCAT(series ORDER BY series SEPARATOR '<li>') FROM series GROUP BY init"; $res = mysql_query($sql); while (list($init, $seriess) = mysql_fetch_row($res)) { $seriess = "<li><a href=\"counter.php?id=$id\">$seriess</a>"; echo '<div class="accordionButton"><h2>'.$init.'</h2></div> <div class="accordionContent"> <ul class="secondnav">'.$seriess.' </ul> </div>'; } ?>
-
I found this article explaining how to implement the alphabetical system of listing using PHP, but I want to add a URL to all the list entries. I need to do this by modifying the SEPARATOR, but no matter what I try it won't allow the <a href=""> within it's area. Here's the simple code I have: <?php mysql_connect("localhost", "name", "pw"); @mysql_select_db(db) or die( "Unable to select database"); $sql = "SELECT SUBSTRING(series,1,1) as init, GROUP_CONCAT(series ORDER BY series SEPARATOR "<li><a href="">") FROM series GROUP BY init"; $res = mysql_query($sql); while (list($init, $seriess) = mysql_fetch_row($res)) { echo '<div class="accordionButton"><h2>'.$init.'</h2></div> <div class="accordionContent"> <ul class="secondnav"><li>'.$seriess.' </ul> </div>'; } ?> I get the error: for the line which holds the SEPARATOR and its contents. Any ideas on how to implement the URL?
-
I'm currently working on a dropdown menu using jquery, and I've run into a snag about how I want it to look. I'd like the top link of the menu to remain "hovered" even when I browse the lower list. Does anyone know of a way to retain the hover for the top <li> even when the mouse is moving down the row to a sub <ul>? Here's a model for practical reference: link here Here's my simple listing for a part (two of the columns as an example) of the navigation: <ul class="tabs"> <li><a href="fanfiction">F</a> <ul class="dropdown"> <li><a href="fanfiction/stories.php?series=Black Blood Brothers">Black Blood Brothers</a> <li><a href="fanfiction/stories.php?series=Gankutsuou">Gankutsuou</a> <li><a href="fanfiction/stories.php?series=Hellsing">Hellsing</a> <li><a href="fanfiction/stories.php?series=Inuyasha">Inuyasha</a> <li><a href="fanfiction/stories.php?series=Rurouni Kenshin">Rurouni Kenshin</a> <li><a href="fanfiction/stories.php?series=Trigun">Trigun</a> </ul> </li> <li><a href="design">D</a> <ul class="dropdown"> <li><a href="design/type.php?type=Divider">Dividers</a></li> <li><a href="design/type.php?type=Table">Tables</a></li> <li><a href="design/type.php?type=Iframe">Iframes</a></li> </ul> </li> </ul> And here's the CSS for the dropdown: ul.tabs { display: table; margin: 0; padding: 0; list-style: none; position: relative; } ul.tabs li { list-style: none; display: table-cell; float: left; position: relative; text-align: center; width: 117px; font-size: 20px; font-weight: bold; padding: 2px 0px 0px 1px; line-height: 40px; } ul.tabs a { position: relative; display: block; } ul.tabs a:hover { background: url(images/navhover.png) top center no-repeat; } /* dropdowns *************************/ ul.dropdown { margin: 0px padding: 0px; display: block; position: absolute; z-index: 999; width: 116px; display: none; background: url(images/lowernavhover.jpg) top center no-repeat; background-color: #ccc; } ul.dropdown li { margin: 3px; padding: 0; float: none; position: relative; list-style: none; display: block; font-size: 12px; font-weight: normal; text-align: left; text-indent: 3px; width: 116px; line-height: 18px; } ul.dropdown li a { display: block; } ul.dropdown a:hover { background: none; }
-
It's a little difficult to tell without any code (other than looking at your source), but I'd say you should place the footer in a separate div and float it to the left. Do the same with the header and body content (encase in div and float to left) and that should take care of your problem.
-
IF Statement With Logged In Feature - specifying the query
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
As a bump, here is a question I gave which is in a similar vein: link here And here are a few related links, such as where I got the basics for this script. -
I have implemented an (old) login system into my site which I found here. The difficulty I'm having is with the content guests and users are allowed to view. I've created a field, here called $nom, which when identified in the database signifies a guest is allowed to view the information. The login script uses the simple code snippet if(!$session->logged_in) { to signify what the users can view, and an ELSE for what guests can view. What I can't figure out is a way to execute a query, here using an IF statement, which will show what users are allowed to view, and a simple ELSE which will show guests what they are allowed to view. Here's the IF statement I've put together to try to specify the guests from the users, but when viewed logged in or not the result is always the same: if(!$session->logged_in) { echo '<li><a href="info.php?author='.$author.'&title='.$title.'">'.$title.'</a></li>'; } else { $result_section = "SELECT * FROM archives WHERE nom = 'no' LIMIT 1 "; $results = mysql_query( $result_section ) or die(mysql_error()); while ($section = mysql_fetch_assoc($results)) { echo '<li><a href="info.php?author='.$author.'&title='.$title.'">'.$title.'</a></li>'; } } I can't figure out what's wrong, and no error message appears telling me my code is wrong. Anyone have any ideas? Here's the code in its entirety: <?php if (!is_numeric($_GET["author"]) && !empty($_GET["author"]) && $_GET["author"]!="") { $author = $_GET["author"]; } mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query = sprintf("SELECT DISTINCT title, url, archives.nom FROM archives LEFT JOIN author ON author.id = archives.id WHERE `author`= '%s' ORDER BY title ", mysql_real_escape_string($author)) or die(mysql_error()); $result = mysql_query( $query ) or die(mysql_error()); $i=1; echo '<h1>'.$author.'</h1><ol> <li>Authored Titles: <ol class="titles">'; while ($row = mysql_fetch_array($result)) { extract($row); if(!$session->logged_in) { echo '<li><a href="info.php?author='.$author.'&title='.$title.'">'.$title.'</a></li>'; } else { $result_section = "SELECT * FROM archives WHERE nom = 'no' LIMIT 1 "; $results = mysql_query( $result_section ) or die(mysql_error()); while ($section = mysql_fetch_assoc($results)) { echo '<li><a href="info.php?author='.$author.'&title='.$title.'">'.$title.'</a></li>'; } } } echo '</ol></li>'; ?> </ol>
-
[SOLVED] PHP Within PHP: IF Statement
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
That worked perfectly! Thanks for your help! I'll be sure to study the PHP for this statement, as I've numerous cases where this can be used. -
[SOLVED] PHP Within PHP: IF Statement
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
I use the word 'embed' here because I can think of no better word which describes placing one object within another, without of course using that long string of words from it's definition. And here's the entirety of the code. By the length you can tell why I wish to, um, place the logged_in statement within the echo as otherwise I would be forced to duplicate this twice on the same page, one for logged_in users to view and another for guests. <?php mysql_connect(localhost,user,pw); @mysql_select_db(db) or die( "Unable to select database"); $query=sprintf("SELECT * from fanfiction WHERE `story`= '%s' ORDER BY id asc ", mysql_real_escape_string($story)); $result = mysql_query( $query ) or die(mysql_error()); $screen = $_GET['screen']; $PHP_SELF = $_SERVER['PHP_SELF']; $rows_per_page=1; $total_records=mysql_num_rows($result); $pages = ceil($total_records / $rows_per_page); $last = $pages -1; if (!isset($screen)) $screen=0; $start = $screen * $rows_per_page; $query .= "LIMIT $start, $rows_per_page"; $result= mysql_query($query) or die ("Could not execute query : $query." . mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); echo '<h3>' . $title . ($session->logged_in) ? ' - <a href="edit.php?cmd=edit&title=' . $title . '">Edit</a>' : '' . '</h3>'.$chapter.''; } echo '<ul class="series">'; if ($screen == 0) { } else { echo "<li><a href=\"$PHP_SELF?story=$story&screen=0\">First</a>"; } // Put Code C Here // create the dynamic links if ($screen > 0) { $j = $screen - 1; $url = "$PHP_SELF?story=$story&screen=$j"; echo "<li><a href=\"$url\">Previous</a>"; // } // page numbering links now $p = 3; // number of links to display per page $lower = $p; // set the lower limit to $p $upper = $screen+$p; // set the upper limit to current page + number of links per page while($upper>$pages){ $p = $p-1; $upper = $screen+$p; } if($p<$lower){ $y = $lower-$p; $to = $screen-$y; while($to<0){ $to++; } } if(!empty($to)) { for ($i=$to;$i<$screen;$i++){ $url = "$PHP_SELF?story=$story&screen=" . $i; $j = $i + 1; echo "<li><a href=\"$url\">$j</a>"; } } for ($i=$screen;$i<$upper;$i++) { $url = "$PHP_SELF?story=$story&screen=" . $i; $j = $i + 1; echo "<li><a href=\"$url\">$j</a>"; } if ($screen < $pages-1) { $j = $screen + 1; $url = "$PHP_SELF?story=$story&screen=$j"; echo "<li><a href=\"$url\">Next</a>"; } if ($screen == $last) { } else { echo "<li><a href=\"$PHP_SELF?story=$story&screen=$last\">Last</a>"; } ?> -
[SOLVED] PHP Within PHP: IF Statement
EternalSorrow replied to EternalSorrow's topic in PHP Coding Help
@thorpe I'm afraid the code you gave me doesn't work for some reason. The logged_in portion does appear, but the $title field at the head does not. I've tried numerous ways of adjusting it, but all end in either error or still a blank area where the $title should be. @roopurt18 You are misunderstanding something about PHP because that statement makes little logical sense. Perhaps if you clarify what you meant we can steer you in the right direction. If I am misunderstanding something about PHP, I do wish you'd tell me what this is. Your remark is cryptic and, to put it frank, I have no idea what you're talking about nor asking.