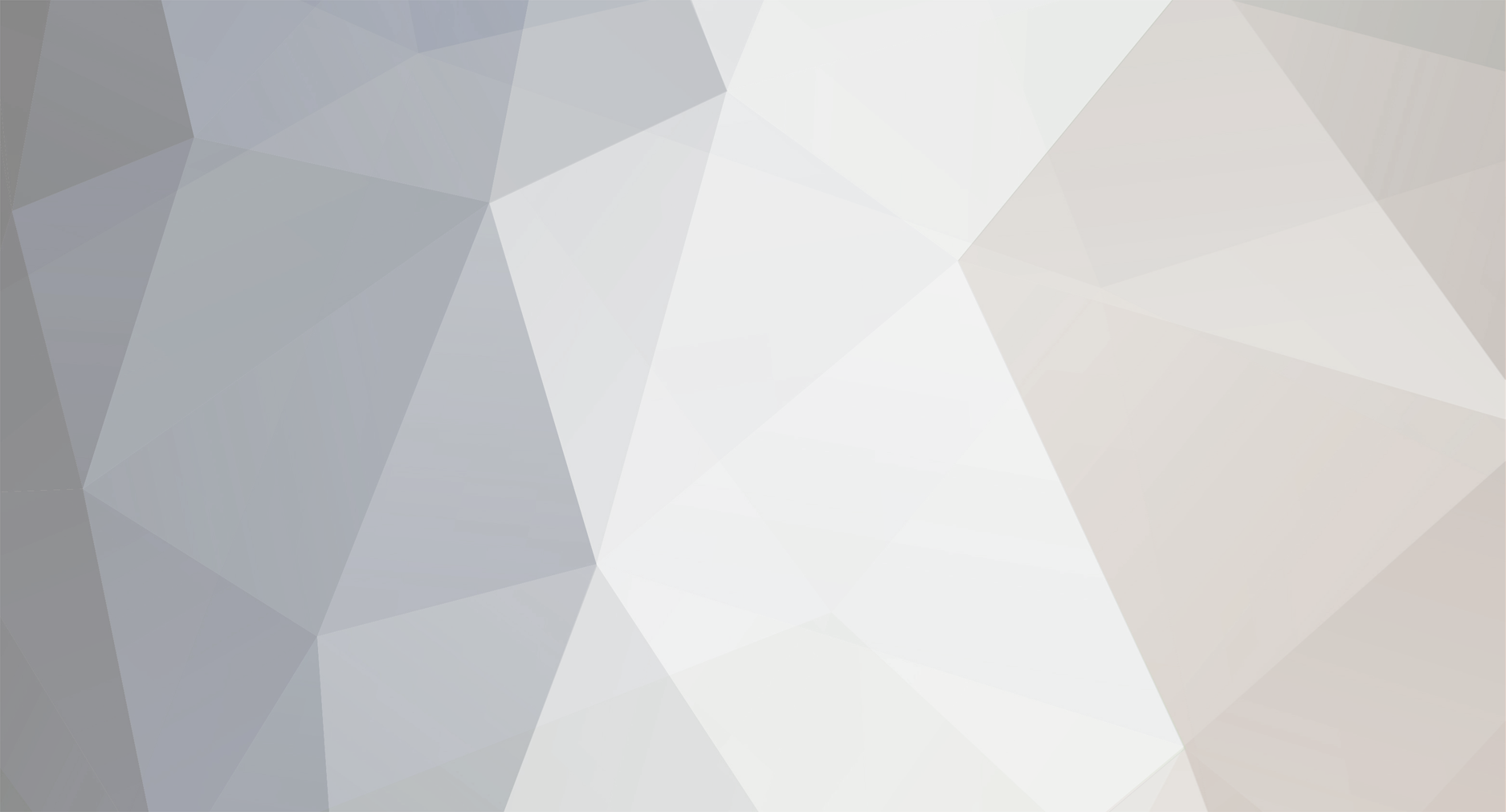
Goldeneye
Members-
Posts
315 -
Joined
-
Last visited
Never
Everything posted by Goldeneye
-
I'm not sure, but it looks like $sortedlist is a two-dimensional array. And you're just echoing the array in the Type-dimension. It looks like you have to do: echo "<h3>{$list['Type'][0]}</h3>\n"; (or whatever array-key you're trying to echo) instead of: echo "<h3>{$list['Type']}</h3>\n";
-
Put "yes" and "no" in the option-tag values: Example: <select name="menu"> <option value="yes">Yes</option> <option value="no">No</option> </select> And then Insert that value (using an SQL-INSERT query) into your database in a VARCHAR-type field.
-
In that case... Try $file_handle = fopen('/path/to/MS_Word_document.doc', 'ab'); instead of $file_handle = fopen('/path/to/MS_Word_document.doc', 'a');
-
I don't know exactly how to edit a Microsoft Word document through PHP, but I can outline how it might be done. <?php $str = 'The text to append to the Microsoft Word Document'; $file_handle = fopen('/path/to/MS_Word_document.doc', a); fwrite($file_handle, $str); fclose($file_handle); ?> I'm not sure that would work, but it should be a push in the right direction.
-
Well I was beaten to the answer, but I'll expand on, and complement the post by Anzeo. Setting session-variables are easy. <?php session_start(); if(isset($_POST['action'])){ $_SESSION['username'] = $_POST['username']; $_SESSION['password'] = $_POST['password']; echo 'Hello, '.$_SESSION['username']; } echo '<div><form action="'.$_SERVER['PHP_SELF'].'" method="post">'; echo '<input type="text" name="username"><br />'; echo '<input type="text" name="password"><br />'; echo '<input type="submit" name="action" value="Go"><br />'; echo '</form></div>'; ?> A couple of things about this code: 1) session_start must at the beginning of the script (or at least before ANY output or $_SESSION variables are used) 2) You should NEVER store a user's password inside a $_SESSION variable. Doing so is very a insecure practice.
-
Do something like this: <?php $images = array('image1.jpg', 'image2.png', 'image3.jpg'); ?> <a href="#"><img src="<?php bloginfo('stylesheet_directory');?>/images/<?php $images[array_rand($images, 1)]; ?>" alt="image file #2" width="125" height="125" /></a> $images can contain any file-name and file-type as long as it actually exists. With that code you can do this
-
Yes, as Gayner suggested, it's from a hypothetical database field named `dst` with a value of either '1' for TRUE or '0' for FALSE. IF `dst` equals '1', then add 1-hour to the UTC-offset. So if the value of $row['timezone'] for yourself was -5 and you had $row['dst'] set to '1' then your UTC-offset would actually be -4. This is because when you enter Daylight-Saving Time, you set your clock back 1-hour. IF you had $row['dst'] set to '0' then you would not add 1 to your value of $row['timezone'] so your UTC-offset would just -5.
-
Try: header('Content-Type: application/octet-stream'); instead of: header("Content-Type: $mtype");
-
Searching & sorting files in a directory using DIR function
Goldeneye replied to jetsettt's topic in PHP Coding Help
It sounds like you're looking for pagination. For array-pagination you basically take the entire array of filenames and walk through it using offsets. Basically, this is the procedure: 1) Get the total number of elements in the array (using count -- I'll call it $count) and decide how many elements you want to display on each page (I'll call it $perpage) 2) Get the number of pages needed to display all the filenames by dividing $count by $perpage and round-up using ceil. (If you round down, some elements of the array will not get displayed if $count and $perpage don't divide evenly). I'll call this $total_pages; 3) calculate the offset based on the page-number ($_GET['page'] -- comes from http://foo.bar/filenames.php?page=2) subtract 1 (so it aligns correctly with the array-keys of the array you're paginating) and multiply by $perpage (to get the page numbers as opposed to just number of array-elements). I'll call this $offset. 4) use array_slice to "walk" through the array-elements. array_slice($array, $offset, $perpage); ($array is the array of filenames) Next... We're not done yet, you have to generate links for the pages <?php $page = $_GET['page']; $query_string = $_SERVER['query_string']; if(stristr($query_string, 'page=') === false){ $query_string .= strlen($query_string) > 0 ? '&page=1' : 'page=1'; $page = 1; } $links = array(); for($i = 1; $i <= $total_pages; ++$i){ if($i == $page) $links[] = $i; else $links[] = '<a href="?'.str_replace('page='.$page, 'page='.$i, $query_string).'">'.$i.'</a>'; } $output = implode(', ', $links); ?> I just provided the code for that as I was getting tired of verbalizing the process. That should work. -
This topic should probably be in the PHPFreaks CSS-Help Forum. Are you certain you edited the correct CSS-class for the table? The font-family property is what you'd use to change a font-face through CSS. You could do it in a CSS-file or right inside the HTML code (which I don't recommend).
-
Do you have other PHP scripts on your server that end up getting downloaded instead of executed or is this the only script that does?
-
To complement the last post (by c-o-d-e), I have written what his post suggested. Since I use this system myself, I know it works to do exactly what you want it to do. The array I provided in y.php is the exact array I use for my projects. In the provided link for a ZIP-File, there are two files. The two files are just two examples of how you would use the seconds-offset with gmdate. y.php exemplifies how you'd implement it into a form so the user can change it. z.php exemplifies how you'd assign it to a $_SESSION variable as if you were pulling it out of a database. It may or may not be a lot of work to implement depending on how you setup your code and how much code you have. I hope these are clear enough examples. If by chance you need some clarification, feel free to send me a private-message. Download it (it's hosted on my personal virtual-directory) http://eltanin.net/~elyk/dev/php/timezone_code.zip
-
Strange characters showing up in text field
Goldeneye replied to sincityalex's topic in PHP Coding Help
You are right, it most likely has to do with the character encoding. Show us the text with these "strange" characters you speak of AND some code. -
Sorry, the reason my function didn't work was because I unknowingly changed a variable-name in the middle of a function... Try this function, instead, I should work: <?php function page($name){ $uri = basename($_SERVER['REQUEST_URI']); if(strpos($uri, '?') !== false) $uri = reset(explode('?', $uri)); return $name == $uri ? true : false; } ?>
-
date_default_timezone_set() sets the timezone used for those date()-type functions so you can just use date() and the correct offset will be set for the user. Unfortunately, both methods require work. You don't have to set the timezone with date_default_timezone_set() or putenv('TZ=...') on every page, you can just put it in a common page and include that page. The PHP5 method does have support for Daylight Saving Time. Also different timezones set in date_default_timezone_set() shouldn't clash. The method I use has backward compatibility and it's not significantly hard to implement -- just store the $dst value in the database under an enum-field or a tinyint-field, and use a checkbox in a form to have the use set it to either true or false. If neither of those do it for you, it might be an alternative to look for a timezone-class but at that point you're just reinventing the wheel.
-
This might be helpful <?php function page($name){ $uri = basename($_SERVER['REQUEST_URI']); if(strpos($uri, '?') !== false) $uri = reset(explode('?', $uri)); return $page == $uri ? true : false; } ?> And then to use it just do if(page('home.php')) include('middle.php');
-
First off, $subject, $message, and $email are undefined. It might help to define them. Either this line: <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> is the culprit or the server you're using does not support PHP. At least, that's what it sounds like.
-
Like this: <?php $timezone = -1; //This is the timezone from the UTC see http://en.wikipedia.org/wiki/List_of_time_zones //$timezone can be anything between +12 and -12 $_SESSION['timezone'] = $timezone * 3600; //And then do this: gmdate(time(), $_SESSION['timezone']; ?> You don't have to use a Session variable but I do. One thing this doesn't support is the use of Day-Light Saving Time. What you could do is give the user an option to +1 to $timezone if that user is in Daylight-Saving Time. <?php $dst = 1; //1 evaluates to boolean TRUE, 0 evaluates to boolean FALSE $_SESSION['timezone'] = ($dst == 1) ? ($timezone + 1) * 3600 : $timezone * 3600; ?> Alternatively, if you're using PHP 5, you can use, date_default_timezone_set. The only downfall this way is having to make a list/array of the timezones you need.
-
[SOLVED] Automatically anchoring URLs that are *not* inside [code] tags
Goldeneye replied to Goldeneye's topic in Regex Help
Thank you, thebadbad! I had no idea it was more complex than just writing a regular-expression. This sort of task seems like something a reuglar-expression would be great for. But anyway, thank you, again. -
The background: I have a function that formats text with bold, italics, underlines, images, and automatically anchored URLs. I have a tag (I call it the [raw] tag as in raw-text) for leaving anything inbetween the tags unformatted. The problem: URLs inside the [raw]*[/raw] tags still get automatically anchored. The code: $strs = array('This is a [i]string[/i] with a link: http://foobar.com/ -- no twix for you.', '[raw]This is a [i]string[/i] with a link: http://foobar.com/ -- no twix for you[/raw]' ); foreach($strs as $str){ preg_match_all('/\[raw\](.*?)\[\/raw\]/si', $str, $code); foreach($code[1] as $stri) $str = '<span style="font-family: monospace; white-space: pre;">'.str_replace('[', '[', $stri).'</span>'; // THIS IS WHERE THE RAW-TAG REPLACEMENT ENDS // ################################## // NOW FOR PARSING THE REGULAR TAGS THAT HAVEN'T HAD THEIR LEFT-SQUARE BRACKETS REPLACED: $search = array('/^[\&\#91\;raw\]]((mailto:|(http|ftp|nntp|news):\/\/).*?)(\s|<|\)|"|\\\\|\'|$)^[\&\#91\;\/raw\]]/', '/\[i\](.*?)\[\/i\]/si'); $replace = array('<a href="$1" rel="nofollow" target="_blank">$1</a>$4', '<i>$1</i>'); $str = preg_replace($search, $replace, $str); echo '<p>'.$str.'</p>'; } What I expect to happen is that the element of the array will be: This is a <i>string</i> with a link: <a href="http://foobar.com/">http://foobar.com/</a> -- no twix for you. What I expect the second element of the array to look like: This is a [i]string[/i] with a link: http://foobar.com/ -- no twix for you The breakdown of my automatically anchoring Regular-Expression: '/^[\&\#91\;raw\]] ((mailto:|(http|ftp|nntp|news):\/\/).*?)(\s|<|\)|"|\\\\|\'|$) ^[\&\#91\;\/raw\]]/' What this should do is that it looks for URLs not in-between [raw]*[/raw] tags and anchors them. I use ^[\&\#91\;\/raw\]] instead of ^[\[\/raw\]] because the raw-tag works by replacing the first square bracket ([) with it's HTML-entity equivalent ([). A preemptive thanks to any input/advice.
-
[SOLVED] Inserting a check in checkbox from array value
Goldeneye replied to jmr3460's topic in PHP Coding Help
Okay, a few things that can changed: 1) Remove the commas and array-keys from your array values in $formats (believe me, they aren't necessary) like so: $formats = array("Discussion", "Basic Text Study", "It Works Study", "IP Study", "Topic", "Step Study", "Tradition Study", "Candlelight", "Speaker", "Birthday", "Men", "Women", "Varies", "Other"); 2) I noticed that you implode your arrays with a space and explode them with a comma-and-space. Just stick to one type of 'adhesive' it makes things quite a bit easier. <?php // taking the formats from the database $form = explode(', ', $result_meeting['format']); // when putting the formats into the database $format = $_POST['format']; $format = implode(', ', $formats); ?> 3) Your foreach loop will now look like this (if you use the 'cleaner' array I provided in #1): <?php foreach($formats as $format) { if (in_array($format, $formatted)){ $select = " checked"; } else { $select = " "; } // output row from foreach echo "<td><input type=\"checkbox\" name=\"format[]\" value=\"" . $format . "\"" . $select . ">" . $format . "</td>\n"; } ?> [/code] -
Basing Administrator status on what the user-ID is, isn't the best way to have an administration echelon. Instead, have an ENUM field in your database called `admin` with either 0 (or not admin) and 1 (or is admin. That is a very primitive way, as well but it is leaves room for more flexibility. Also, member_id should be $member_id.
-
If you're looking to perform a page redirect, you can use the following code: <?php if(...) //check if user is logged in header('Location: the_page_you_want_to_redirect_to.php') and exit; ?> That code will redirect to the page you specify. You must place this in your script before anything is outputted with echo or print.
-
Method 1: Use serialize on the array you want to store in the database and then use unserialize when pulling that same value from the database -- or, as lemmin suggests -- Method 2: implode the array before inserting it into the database. And then explode that string when pulling it from the database.
-
[SOLVED] Inserting a check in checkbox from array value
Goldeneye replied to jmr3460's topic in PHP Coding Help
<?php $str = 'Discussion, Men, Speaker'; $array = explode(', ', $str); print_r($array); ?> Assuming your code is like that, it should produce Array ( [0] => Discussion [1] => Men [2] => Speaker ) What exactly does your code look like?