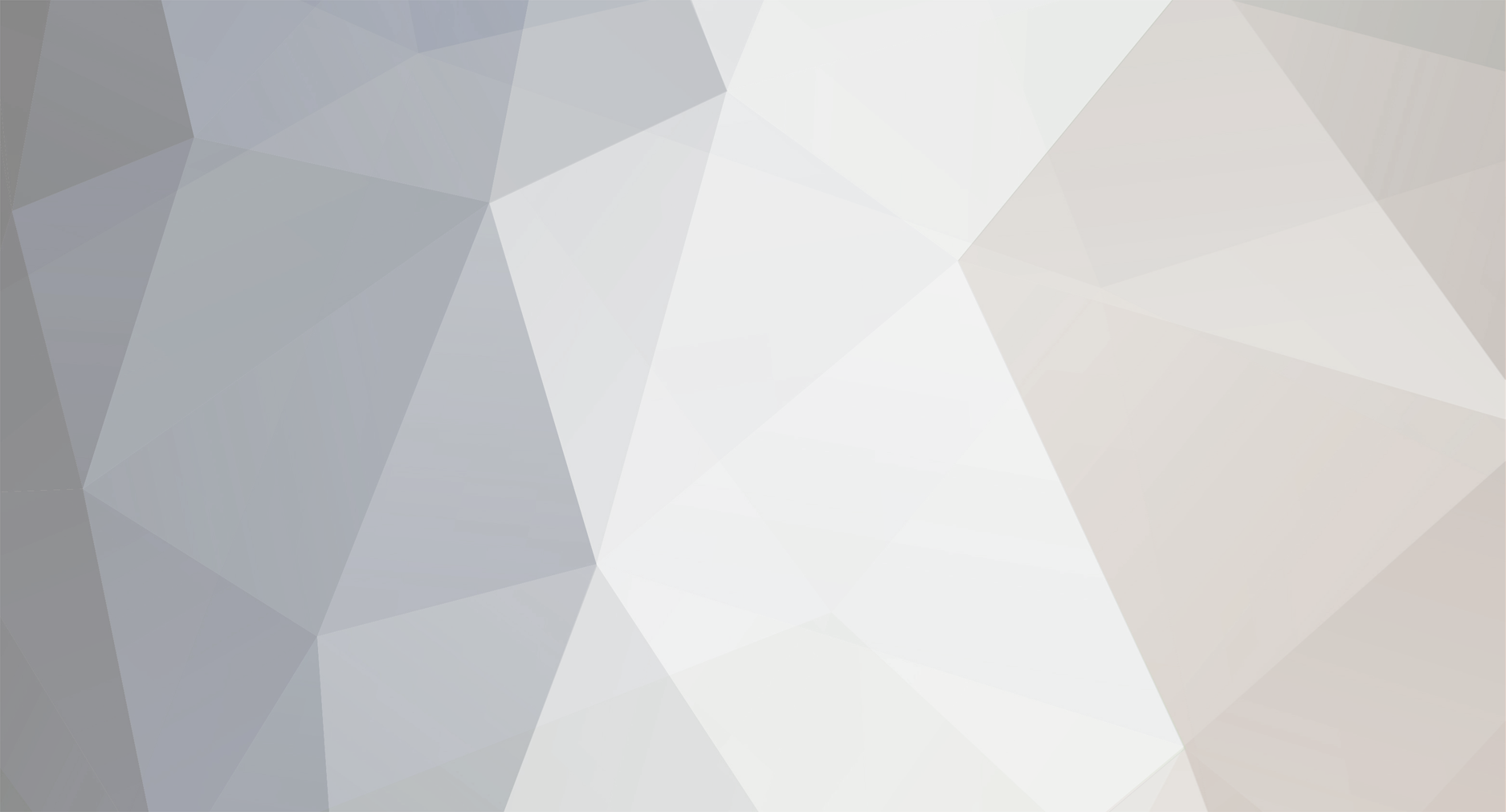
sqlnoob
-
Posts
173 -
Joined
-
Last visited
Posts posted by sqlnoob
-
-
I probably need to use a JOIN of some sort, but then I have all these WHERE clauses and a computation to consider. Plus it also needs to be updated and I'm not even sure wether that is possible.
I am a complete noob about these things, it would be my first time using such commands.
-
perhaps SUM and HAVING command are what you need, or perhaps you need to add a WHERE clause
I'm not terribly sure what you want to achieve with only those 2 columns and what values they can have. Can we assume that VotedFor holds a UserID?
Perhaps try GROUP BY UserID instead of VotedFor if that helps
Here is a good tutorial for those commands.
-
Not terribly sure where to ask this, but here goes...
WHAT I HAVE
Ok I have these 2 tables:
$sqlgang = "CREATE TABLE gangsters ( GangsterID int(4) NOT NULL AUTO_INCREMENT, PRIMARY KEY(GangsterID), Gangstername varchar(30) NOT NULL, Password varchar(10) NOT NULL, Gangname varchar(20) NOT NULL, City varchar( NOT NULL, Personality varchar(15) NOT NULL, Finances int(9) NOT NULL, Booze int(3) NOT NULL, Counterfeit int(3) NOT NULL, Status varchar(20) NOT NULL, Notoriety int(12) NOT NULL, Checkday int(3) NOT NULL, Officecheck int(1) NOT NULL, Recruitcheck int(1) NOT NULL, Residencecheck int(1) NOT NULL, Recruitcheck int(1) NOT NULL, Mapurl varchar(40) NOT NULL, Musiccheck int(1) NOT NULL, Ipad varchar(16) NOT NULL )"; mysql_query($sqlgang,$con); $sqlstreets = "CREATE TABLE streets ( BusinessID int(5) NOT NULL, PRIMARY KEY(BusinessID), Businesscategory varchar(10) NOT NULL, Businesstype varchar(20) NOT NULL, Owner varchar(30) NOT NULL, Extortionist varchar(30) NOT NULL, Landvalue int(6) NOT NULL, Businessclass int(1) NOT NULL, Operationalstatus varchar(20) NOT NULL, Statusday int(3) NOT NULL, Illegal varchar(20) NOT NULL, Income int(5) Default '0', Neighbourhood varchar(30) NOT NULL, Policeward int(2) NOT NULL, Churchward int(2) NOT NULL )"; mysql_query($sqlstreets,$con);
the Gangsters table and the streets table. Now the data in the Finances column of the gangsters table needs to be automatically updated each day. I know how to this manually each day for 1 record as shown by this:
//TIME CALCULATION $gamestart = mktime(0, 0, 0, 11, 14, 2008); $today = time(); $rawdifference = $today - $gamestart; $thisday = floor($rawdifference / 84600); //FETCHING TABLE TIME $dayresult = mysql_query ("SELECT Checkday FROM gangsters WHERE Gangstername ='$you' AND Status <>'dead'"); $dayrow = mysql_fetch_array($dayresult); $oldday = $dayrow['Checkday']; //CHECKING DATE OF LAST UPDATE if ($thisday > $oldday){ //FETCHING ILLEGAL INCOME $incomeresult = mysql_query ("SUM(Income) AS Incomesum FROM streets WHERE Owner ='$you' AND Operationalstatus ='running'"); $incomerow = mysql_fetch_array($incomeresult); $illegalincome = $incomerow['Incomesum']; //FETCHING AND CALCULATING LEGAL INCOME $bizzresult = mysql_query ("SUM(Landvalue) AS Bizzsum FROM streets WHERE Owner ='$you' AND Operationalstatus ='running' OR Operationalstatus ='open'"); $bizzrow = mysql_fetch_array($bizzresult); $tabincome = $bizzrow['Bizzsum']; $legalincome = $tabincome / 20; //FETCHING OLD MONEY $moneyresult = mysql_query ("SELECT Finances FROM gangsters WHERE Gangstername ='$you' AND Status <>'dead'"); $moneyrow = mysql_fetch_array($moneyresult); $oldmoney = $moneyrow['Finances']; //CALCULATING NEW MONEY $newmoney = $oldmoney + $legalincome + $illegalincome; //UPDATING NEW MONEY mysql_query("UPDATE gangsters SET Finances ='$newmoney' WHERE Gangstername ='$you'"); }
MY PROBLEM
I want to be able to automatically update the Finances field for all the records in the gangsters table that need updating each day. However I have no clue as to how to do that. Any help in solvng my problem would be very much appreciated.
-
image rollover is doable but I have about 65 provinces, that's a lot of possible combinations i'd get
there'sd got to be a better way than that?
-
Oh btw before I forget, my site uses phpadmin and I'm not too sure my host has a gd library installed (probably not).
-
OK I'm not too sure where to ask this, but bear with me.
WHAT I HAVE:
I've made a small online multiplayer game (you can watch it at www.shugo.nl). In here I have this map of Japan, with 65 provinces. I use a red background for the .php file containing the map of Japan. For each of the 65 provinces there is a capital (shown as a large dot). In the map (which is .gif file), these dots of the .gif file containing the map are transparent, so that you see the red background color through the map. Whenever a player of the game owns a certain province, I use a php script, to show a blue square image in a -1 z-index (so that it becomes a background image, behind the map image). I use this php script also to position this blue background image below the capital, so that I get a blue dot.
The result is that any player who owns a province gets to see that by a blue dot, where as the other provinces that player doesn't own are shown with red dots. To give you an idea of the situation:
http://www.shugo.nl/daimyo.htm
I use an sql table to store the owner of a province and such and another table to store the diplomatic stances.
THE TROUBLE
What I want to do is change is this entire situation. Instead of just some dots (the province capitals) changing color, I want to change the color of the entire province, but keep the the black borders of the provinces and of course the same color for the sea and ocean.
I want to change it in such a way, that for example I get 3 different maps.
1. a map showing provinces in blue who are player controlled and red as non-player controlled
2. a map showing the various clans (there are 32 in the game, plus a number of minor clans and possible rebels) with a diffirent color for each clan (probably not going to implement this one, because 34 different colors is hard to notice)
3. a map showing the diplomatic settings of the clan. For instance red means war and blue means peace etcetera.
HELP/SOLUTION
Anyone know of good and (preferably easy way, though I doubt that) of doing this.
Any suggestions, hints possible solutions are very welcome.
Thanks in advance for any help.
-
oh shoot
forgot to change that reference
thanks alot all, it works now
Thread closed!
-
now this is the echo i get:
select sum(Wealth) as sumofwealth FROM provinces WHERE Owner ='Kikkawa' GROUP BY Owner
taxes have been collected
still no SUM
???
-
can you show me what exactly you're doing
-
yup 100% sure I have a value there, it echoes the clans name, so that's allright.
either the way I'm querying it, or the way I'm fetching it, or the table is wrong somehow.
???
-
I should have, but let me echo it, just to be sure
-
it is this SUM that I don't get, neither in the echo, nor in my updating part of my economy.php file.
So that means it somehow is not fetching it, but what exactly am I doing wrong there. I can't seem to figure that out.
-
ok I want to do something like this:
http://www.w3schools.com/sql/sql_groupby.asp
In my case I want to have the SUM of all wealth of a certain clan whichever is logged in. I get the clan name from the cookie, which I use to search the table called provinces to get all the provinces Wealth of this clan.
I want the user/clan to be able tax the wealth of all the provinces they own.
So lets say Hosokawa is logged in at the moment, then I need to fetch the SUM of all their Wealth of the provinces they own.
In this example Hosokawa owns the provinces Izumi, Kawachi and Settsu, which respectively should get Hosokawa 304 + 185 + 459 = a SUM of 948.
I'm new at this and I haven't used aggregate functions before, so clearly I'm doing something wrong, but what? ???
-
tried it and nope still doesn't work
this turned out to be more difficult then expected
-
changed it to this. Now it no longer returns an fatal error, but it still doesn't echo my result, nor updates it the way it supposed.
$receiptresult = mysql_query ("SELECT SUM(Wealth) FROM provinces GROUP BY Owner WHERE Owner = '$taxuser'");
$taxrowreceipt = mysql_fetch_assoc($receiptresult);
$taxreceipt = $taxrowreceipt['SUM(wealth)'];
why?
-
line 84 is this line:
errrr
$receiptresult = mysql_query ("SELECT SUM(Wealth) FROM provinces WHERE Owner = '$taxuser'");
-
Fatal error: Unsupported operand types in www/economy.php on line 84
-
Is anyone able to help me and see what's wrong with it.
Any help is much appreciated
-
these are the tables I'm using to get the data from:
<?php if ($con = @mysql_connect('localhost', 'shugonl', 'password')) { mysql_select_db('shugonl', $con); echo 'connected to the database'; } else { echo 'connection failed'; } mysql_select_db("shugonl", $con); $sqlpersons = "CREATE TABLE persons ( Password varchar( NOT NULL, Clan varchar(10) NOT NULL, Username varchar(20) NOT NULL, Coffer int( NOT NULL, Taxheight int(1) NOT NULL, Yari int(6) NOT NULL, Dachi int(5) NOT NULL, Yumi int(5) NOT NULL, Daisho int(5) NOT NULL, Teppo int(5) NOT NULL, Honjin int(2) NOT NULL, Taxyear int(2) NOT NULL, Attackp int(1) NOT NULL, Lastyear int(2) NOT NULL, Religion int(1) NOT NULL, Insurgent int(2) NOT NULL )"; mysql_query($sqlpersons,$con); $startday = idate("d"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Akita', 'unused', '0', '2', '0', '0', '0', '0', '0', '2', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Amako', 'unused', '0', '2', '0', '0', '0', '0', '0', '44', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Arima', 'unused', '0', '2', '0', '0', '0', '0', '0', '59', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Asakura', 'unused', '0', '2', '0', '0', '0', '0', '0', '18', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Chiba', 'unused', '0', '2', '0', '0', '0', '0', '0', '0', '14', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Chosokabe', 'unused', '0', '2', '0', '0', '0', '0', '0', '56', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Hatakeyama', 'unused', '0', '2', '0', '0', '0', '0', '0', '32', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Hatano', 'unused', '0', '2', '0', '0', '0', '0', '0', '49', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Hojo', 'unused', '0', '2', '0', '0', '0', '0', '0', '13', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Hosokawa', 'unused', '0', '2', '0', '0', '0', '0', '0', '33', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Imagawa', 'unused', '0', '2', '0', '0', '0', '0', '0', '17', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Ishida', 'unused', '0', '2', '0', '0', '0', '0', '0', '27', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Isshiki', 'unused', '0', '2', '0', '0', '0', '0', '0', '50', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Ito', 'unused', '0', '2', '0', '0', '0', '0', '0', '63', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Jinbo', 'unused', '0', '2', '0', '0', '0', '0', '0', '19', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Kikkawa', 'unused', '0', '2', '0', '0', '0', '0', '0', '43', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Kikuchi', 'unused', '0', '2', '0', '0', '0', '0', '0', '61', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Kono', 'unused', '0', '2', '0', '0', '0', '0', '0', '54', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Maeda', 'unused', '0', '2', '0', '0', '0', '0', '0', '22', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Matsudaira', 'unused', '0', '2', '0', '0', '0', '0', '0', '25', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Miyoshi', 'unused', '0', '2', '0', '0', '0', '0', '0', '53', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Mori', 'unused', '0', '2', '0', '0', '0', '0', '0', '37', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Nagao', 'unused', '0', '2', '0', '0', '0', '0', '0', '3', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Oda', 'unused', '0', '2', '0', '0', '0', '0', '0', '28', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Otomo', 'unused', '0', '2', '0', '0', '0', '0', '0', '57', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Ouchi', 'unused', '0', '2', '0', '0', '0', '0', '0', '46', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Shimazu', 'unused', '0', '2', '0', '0', '0', '0', '0', '65', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Shoni', 'unused', '0', '2', '0', '0', '0', '0', '0', '62', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Takeda', 'unused', '0', '2', '0', '0', '0', '0', '0', '9', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Uesugi', 'unused', '0', '2', '0', '0', '0', '0', '0', '11', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Urakami', 'unused', '0', '2', '0', '0', '0', '0', '0', '39', '$startday', '0', '$startday', '0', '0')"); mysql_query("INSERT INTO persons (Password, Clan, Username, Coffer, Taxheight, Yari, Dachi, Yumi, Daisho, Teppo, Honjin, Taxyear, Attackp, Lastyear, Religion, Insurgent) VALUES ('demo', 'Yamana', 'unused', '0', '2', '0', '0', '0', '0', '0', '42', '$startday', '0', '$startday', '0', '0')"); mysql_select_db("shugonl", $con); $sqlprov = "CREATE TABLE provinces ( Identifier int(2) NOT NULL, Province varchar(10) NOT NULL, Capital varchar(11) NOT NULL, Castle varchar(10) NOT NULL, Owner varchar(20) NOT NULL, Wealth int(4) NOT NULL, Region int(2) NOT NULL, Attitude int(2) NOT NULL, Machi varchar(7) NOT NULL )"; mysql_query($sqlprov,$con); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('1', 'Sado', 'Sado', 'none', 'neutral', '50', '1', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('2', 'Dewa', 'Sakata', 'none', 'Akita', '430', '2', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('3', 'Echigo', 'Niigata', 'none', 'Nagao', '451', '2', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('4', 'Hitachi', 'Ishioka', 'none', 'neutral', '605', '2', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('5', 'Mutsu', 'Wakamutsu', 'none', 'neutral', '1520', '2', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('6', 'Shimotsuke', 'Utsunomiya', 'none', 'neutral', '380', '2', '1', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('7', 'Awa', 'Tokushima', 'none', 'neutral', '177', '9', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('8', 'Izu', 'Tagata', 'none', 'neutral', '89', '3', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('9', 'Kai', 'Kofu', 'none', 'Takeda', '207', '3', '1', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('10', 'Kazusa', 'Otaki', 'none', 'neutral', '96', '3', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('11', 'Kozuke', 'Maebashi', 'none', 'Uesugi', '608', '3', '1', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('12', 'Musashi', 'Edo', 'none', 'neutral', '2557', '3', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('13', 'Sagami', 'Odawara', 'none', 'Hojo', '506', '3', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('14', 'Shimousa', 'Sakura', 'none', 'Chiba', '101', '3', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('15', 'Shinano', 'Matsumoto', 'none', 'neutral', '402', '3', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('16', 'Suruga', 'Sumpu', 'none', 'Imagawa', '245', '3', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('17', 'Totomi', 'Hamamatsu', 'none', 'Imagawa', '288', '3', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('18', 'Echizen', 'Fukui', 'none', 'Asakura', '180', '4', '3', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('19', 'Etchu', 'Takaoka', 'none', 'Jinbo', '260', '4', '2', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('20', 'Hida', 'Takayama', 'none', 'neutral', '120', '4', '2', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('21', 'Kaga', 'Kanazawa', 'none', 'Maeda', '835', '4', '3', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('22', 'Noto', 'Nanao', 'none', 'Maeda', '215', '4', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('23', 'Iga', 'Ueno', 'none', 'neutral', '95', '5', '3', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, machi) VALUES ('24', 'Ise', 'Suzuka', 'none', 'neutral', '440', '5', '3', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('25', 'Mikawa', 'Okazaki', 'none', 'Matsudaira', '252', '5', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('26', 'Mino', 'Gifu', 'none', 'Oda', '135', '5', '2', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('27', 'Omi', 'Odani', 'none', 'Ishida', '194', '5', '2', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('28', 'Owari', 'Kiyosu', 'none', 'Oda', '200', '5', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('29', 'Shima', 'Toba', 'none', 'neutral', '51', '5', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('30', 'Izumi', 'Izumi', 'none', 'Hosokawa', '304', '6', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('31', 'Kawachi', 'Fujidera', 'none', 'Hosokawa', '185', '6', '1', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('32', 'Kii', 'Wakayama', 'none', 'Hatakeyama', '376', '6', '3', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('33', 'Settsu', 'Osaka', 'none', 'Hosokawa', '459', '6', '3', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('34', 'Yamashiro', 'Kyoto', 'none', 'neutral', '704', '6', '3', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('35', 'Yamato', 'Nara', 'none', 'neutral', '200', '6', '3', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('36', 'Aki', 'Hiroshima', 'none', 'Mori', '800', '7', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('37', 'Bingo', 'Fuchu', 'none', 'Mori', '130', '7', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('38', 'Bitchu', 'Soja', 'none', 'Hosokawa', '114', '7', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('39', 'Bizen', 'Okayama', 'none', 'Urakami', '574', '7', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('40', 'Harima', 'Himeji', 'none', 'neutral', '220', '7', '3', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('41', 'Hoki', 'Kurayoshi', 'none', 'neutral', '103', '7', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('42', 'Inaba', 'Tottori', 'none', 'Yamana', '125', '7', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('43', 'Iwami', 'Hamada', 'none', 'Kikkawa', '53', '7', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('44', 'Izumo', 'Matsue', 'none', 'Amako', '106', '7', '2', 'plain')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('45', 'Mimasaka', 'Tsuyama', 'none', 'Urakami', '68', '7', '2', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('46', 'Nagato', 'Shimonoseki', 'none', 'Ouchi', '236', '7', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('47', 'Suwa', 'Hofu', 'none', 'Ouchi', '209', '7', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('48', 'Tajima', 'Hidaka', 'none', 'Yamana', '60', '7', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('49', 'Tamba', 'Kameyama', 'none', 'Hatano', '81', '7', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('50', 'Tango', 'Maizuru', 'none', 'Isshiki', '208', '7', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('51', 'Wakasa', 'Obama', 'none', 'neutral', '112', '7', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('52', 'Awaji', 'Minami', 'none', 'neutral', '56', '8', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('53', 'Awa', 'Miyoshi', 'none', 'Miyoshi', '90', '3', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('54', 'Iyo', 'Matsuyama', 'none', 'Kono', '93', '9', '2', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('55', 'Sanuki', 'Takamatsu', 'none', 'Miyoshi', '155', '9', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('56', 'Tosa', 'Nankoku', 'none', 'Chosokabe', '222', '9', '3', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('57', 'Bungo', 'Kokokufu', 'none', 'Otomo', '125', '10', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('58', 'Buzen', 'Fukuoka', 'none', 'neutral', '370', '10', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('59', 'Chikugo', 'Kurume', 'none', 'Arima', '132', '10', '1', 'forest')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('60', 'Chikuzen', 'Dazaifu', 'none', 'neutral', '304', '10', '1', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('61', 'Higo', 'Kumamoto', 'none', 'Kikuchi', '225', '10', '2', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('62', 'Hizen', 'Yamato', 'none', 'Shoni', '1447', '10', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('63', 'Hyuga', 'Saito', 'none', 'Ito', '107', '10', '2', 'coastal')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('64', 'Osumi', 'Kokubu', 'none', 'Shimazu', '121', '10', '1', 'hilly')"); mysql_query("INSERT INTO provinces (Identifier, Province, Capital, Castle, Owner, Wealth, Region, Attitude, Machi) VALUES ('65', 'Satsuma', 'Kagoshima', 'none', 'Shimazu', '605', '10', '1', 'forest')"); ?>
(edited by kenrbnsn to add
tags)
-
this bit doesn't seem to work for me:
$taxuser = $_COOKIE["person"]; $con = mysql_connect("localhost","shugonl","password"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("shugonl", $con); $receiptresult = mysql_query ("SELECT Wealth, SUM(Wealth) FROM provinces WHERE Owner = '$taxuser'"); $taxreceipt = mysql_fetch_assoc($receiptresult); echo "<BR>".$taxreceipt;
-
Didn't know where else to place this question, but here it is.
I recently hosted my site with a cheap host. It worked for a week, till I got an internal server error. It hapens mostly to my .php files, but also a few common .htm files that simply won't show.
Is anyone able to tell me what is wrong and how to solve this?
I'm too much of a noob to figure out what's wrong.
-
I don't think, I quite understand what you're trying to do too ???
why use a header and then be hellbend on not displaying a page
aah nevermind, this is giving me too much a headache. I'm sorry but I can't seem to help you there.
-
ok then p2grace is right
remove the html and enclose the entire bit in php. pass it as an echo. I don't see any other solution to your problem. sorry
-
I once made an html page with javascript this way:
<HTML>
<HEAD>
<TITLE>password checking</TITLE>
<META NAME="keywords" CONTENT="entrance">
<script type="text/javascript">
var password;
var pass1 = "somepassword"; // place password here
password=prompt("Please enter your password:","");
if (password==pass1) {
window.location= "http://www.goodpage.htm"; // file to open if password is correct
} else {
window.location= "http://www.badpage.htm"; // file to load if password is incorrect
}
</script>
</HEAD>
<BODY>
</BODY>
</HTML>
daily auto update problem
in MySQL Help
Posted
so cron job
ok thanks
i've read some tutorials about joins, but i dont see how it possible to do so here. Perhaps with a WHILE i can make it work and run through all the records.