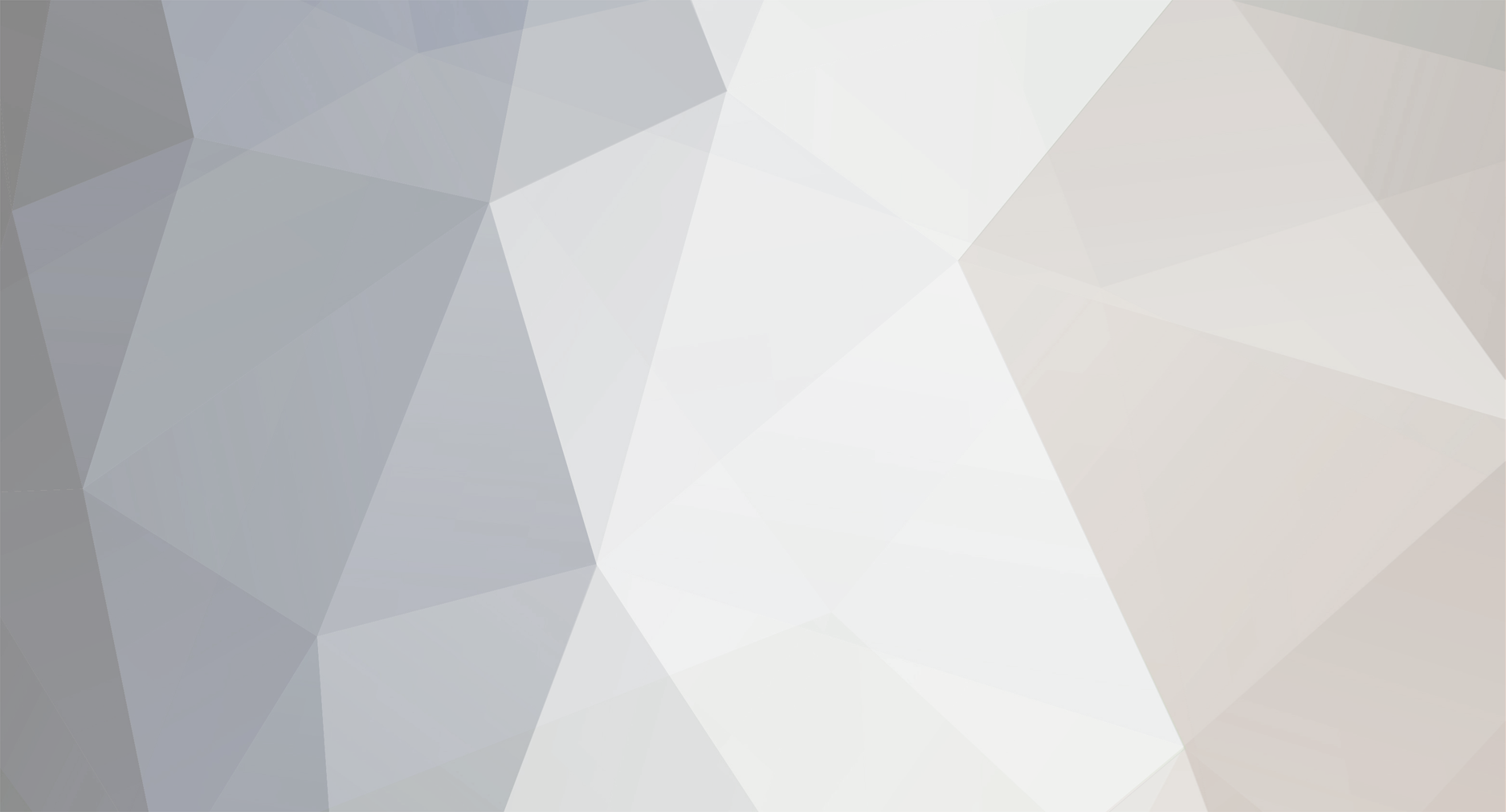
mithras
Members-
Posts
11 -
Joined
-
Last visited
Never
Everything posted by mithras
-
The model is in fact "stupid" and cannot do any actions on other objects. Your view is your template. The model just describes what the element contains: class photo{ var $name; var $id; var $imageLocation; } class album{ var $id; var $name; var $photos = array(); //Array of photo objects } class galleryController{ public function getAlbum( $id ){} public function displayAlbum( $id ){ $album = $this->getAlbum( $id ); $template = new template(); $template->assign( 'album', $album ); echo $template->display( 'album.template.php' ); } public function addPhotoToAlbum( $photo, $album) {} } Here you define two models: a photo and an album. The controller galleryController controls both of them (actually you need for the perfect design two controllers, but this is ok). The template 'album.template.php' is your view.
-
The user class represents (if instantiated) a specific user. The same for a product. Why do you ask the user what the recent added products are? If it's a good design, the user cannot know this information. Actually it's the same for a product. Why should a product know what other products are recently introduced? The best you can use the controller design for this situation: class productController{ public function createProduct(){ return new product(); } public function getProducts(){ $result = db::query( "SELECT * FROM products" ); return $result->fetchArray(); } public function getLatestProducts( $timespan = 3 ){ $result = db::query( "SELECT * FROM products WHERE date > DATE_ADD( NOW(), INTERVAL -1 MONTH) "); return $result->fetchArray(); } } class product{ private $name; private $id; } Now you abstract the product from the controlling product methods.
-
You can place it anywhere, but it should be interpreted as javascript. You should have an element with id "nav" and in that element you should have several list elements (li's) for drop-down's which won't work in IE. There are four possible options: directly in your html file or in an external file. You can also place it into you head or body from the html file. <html> <head> <script> //You can place it here.... </script> <script src="script.js" type="text/javascript"></script> //Or place it in the external file script.js </head> <body> <div id="nav"> <ul><li>a</li></ul> </div> <script> //You can place it here.... </script> <script src="script.js" type="text/javascript"></script> //Or place it in the external file script.js </body> </html> The better is to place it in an external file, because browsers can cache the script easy. Furthermore you better place the script tag at the end in your body. Browsers interpret the html and let the client view the content already. At the end they see a script and will download it. If you place it in your head, the browser must do by each page request another (http) request for the script. After that script request the browser can render the html. So: the best option is to place a <script src="here_your_script.js"></script> tag at the end in your body of the html.
-
It's always a good idea to separate your media files from your controlling php files. For example, you can use a www directory for templates and non-www for coding stuff. In the non-www you have folders for each plugin. In the www there are also folders for each plugin. Each plugin has to maintain it's "own" directory. /non-www/members/core (e.g. controller.php and group.php) /non-www/members/profile (e.g. controller.php and profile.php) /non-www/skins/core (e.g. controller.php and template.engine.php) /www/members/core/ (don't know what to place here) /www/members/profile (e.g. profile.tpl and profile.edit.tpl) /www/skins/classic/ (e.g. core.tpl) etc
-
It depends on the system you want to create. In most complex systems, mvc applications are a good way to go. If you want to make a framework, an abstraction layer or a simple script, it isn't necessary. If you explain more about you're ideas, we could say if its applicable for a mvc system.
-
If you use a database abstraction layer, it might support pagination. If it don't, you can add the pagination feature yourself or maybe switch from database abstraction layer. I start about a database abstration layer, because it's a nice way to abstract pagination from your code in the controlling object. Example: $query = "SELECT name, description FROM items"; database::enablePagination( '5' ); //5 items per page $result = database::query( $query ); while( $pageSet = $result->getNextPage() ){ echo "Current page: " . $result->getCurrentPage(); foreach( $pageSet as $item ){ echo $item->name . " - " . $item->description; } }
-
It's true that sessions are with the default php session handler using cookies following the http protocol. But still sessions and cookies are a safe way for user logins. If you use a challenge/response system (with a non-javascript fallback system) you can safely (enough...) login. If you store the session id in a session and user specific information in a database session table (e.g. id, session, user id, last visit and ip) it's a really stable system. I you sent critical data (credit card numbers, username/password for critical systems) it's better to use https, but it cost more (development, certificates etc) to use it.
-
Some php code may help, but do you use a certain pattern. I think a factory pattern is quite usable in your case: class icalGenerator{ public function __construct(){} public function createIcal(){ $ical = new iCal(); $ical->doSomething(); return $ical; } } class ical{ public function __construct(){} public function __toString(){} public function doSomething(){} } You can use the classes in a loop: $result = array( 12, 14, 19, 40, 89 ); //A list of event ids, for example. $generator = new icalGenerator(); if( $result ){ foreach( $result as $event ){ $ical = $generator->createIcal( $event ); echo $ical; } }else throw new Exception( 'No events to display!' );
-
A license will help so your code cannot be stolen "legally". The company has still the readable php code so they can use it. If you're sure you want to keep control you can use something like Zend Guard or another encryption software.
-
For my cms i have an index.php, init.php and config.php in the root. All other basic code is in the folder 'include' (database etc). Furthermore there are two folders 'plugins' and 'media'. Both are organized by plugin: /plugin/blog/blog.class.php is the blog controller /media/contact/form.php is the template for the contact form I'd like to rewrite it soon to www and non-www. The non-www folder contains the models and controllers. The www folder contains all media files like images, templates, scripts and so on.
-
I want a RBAC system with seperated objects in different domains. Each plugin has its own domain: for domain "blog" there are objects "article", "reaction", "tag" and "setting" and so on. I'm using MySQL 4.0.21 and created these tables: users id (int) name (varchar) groups id (int) name (varchar) user_in_groups group_id (int) user_id (int) actions id (int) name (varchar) domains id (int) name (varchar) object_table (varchar) permissions id (int) domain_id (int) object_id (int) group_id (int) action_id (int) In the field domains.object_table is for each domain the table listed which contains the objects for that domain. The table looks like this: object_table_X id (int) name (varchar) Now I'm trying to get all the objects and their actions for a specific user. Getting domain, action and object_id is no problem: SELECT a.name action, d.name domain, p.object_id object FROM permissions p LEFT JOIN actions a ON p.action_id = a.id LEFT JOIN groups g ON p.group_id = g.id LEFT JOIN domains d ON p.domain_id = d.id LEFT JOIN user_in_groups u ON u.group_id = g.id WHERE u.user_id =1 But I don't want the object_id but the object name. I tried this query: SELECT o.name object, d.name domain, a.name action FROM permissions p LEFT JOIN actions a ON p.action_id = a.id LEFT JOIN groups g ON p.group_id = g.id LEFT JOIN domains d ON p.domain_id = d.id LEFT JOIN user_in_groups u ON u.group_id = g.id LEFT JOIN (SELECT object_table FROM domains d2 WHERE d2.id = p.object_id) o ON p.object_id = o.id WHERE u.user_id =1 But it failed with the following error: Unknown table 'p' in where clause I thought the p in the subquery is allowed; it's also mentioned at this manual page: http://dev.mysql.com/doc/refman/5.0/en/comparisons-using-subqueries.html. Does somebody know how to get the object name? For me, the difficult part is getting the table name from a field in the same query as where you use this table name.