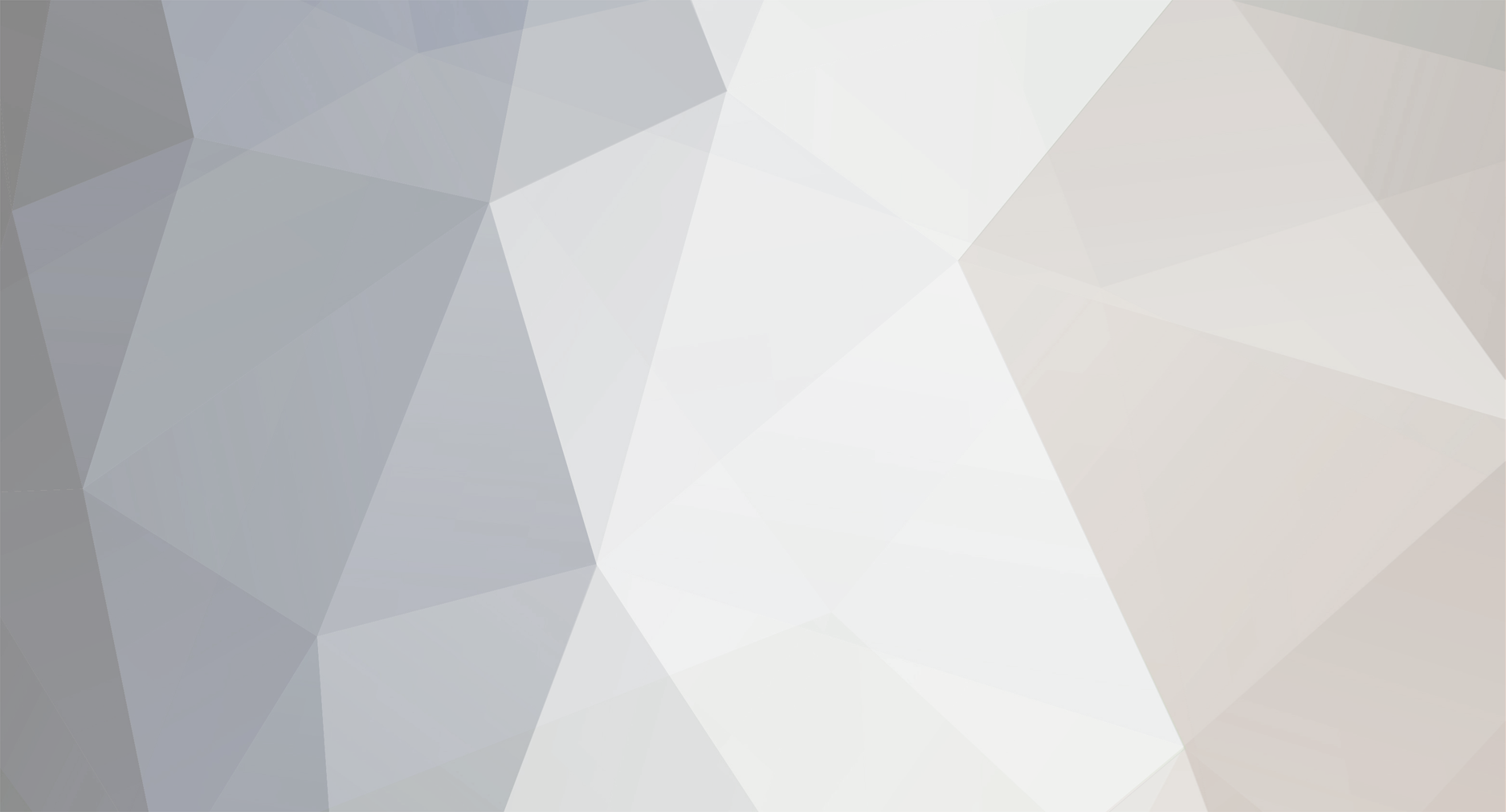
Gighalen
-
Posts
193 -
Joined
-
Last visited
Never
Posts posted by Gighalen
-
-
Yes, sorry.
I assumed that since you already had a login system that you would have an included file that was always conected to the DB
-
Hey guys.
For the past week or so, I have been working on a text-based MMORPG which I have temporarily named "Trinity Conquest". It's written in PHP / MySQL. Here are a few details:
1. You are in charge of your own little 'space empire'.
2. You start out with 1 'system' and 5 or so 'planets'.
3. You assign the population of your planets to do various tasks, such as mine, commerce, industy, etc.
4. Players can buy / sell minerals & items to each other via trade or the Marketplace.
5. You can gain more systems / planets by building scouts and then sending colonists in their wake.
6. You can capture systems / planets from other players by building a fleet and fighting it out.
7. You can buy upgrades for each planet to improve productivity and defense.
8. You can research different technologies to allow for better upgrades, ships, productivity, etc.
9. You can do various different missions/quests to gain planets, resources, etc.
Of course, those are just a few of the features I am planning. I already have the GUI worked out and have the basic functions build for a "Warehouse", or inventory, and I am currently scripting the "Marketplace".
As you can imagine, there is going to be A LOT of scripting invloved, and I don't exactly feel like writing it all myself, so if anyone would like to help contribute, I would greatly appreciate it.
There is no pay involved what-so-ever (for you or me), because if it ever gets finished it will be a free game that everyone can play. However, if you help contribute (coding, images, storyline, security-checking, etc), we can arrange for a credits page of some sorts.
If you would like to help, and show me that you can contribute, PM me on AIM (Gighalen) or E-mail me at Admin@2five6.com
Thanks,
Tom
-
You'll need to implement a hits tracker of sorts.
I have a table like
id ip hits forumid time
When a user hits the forum, it will check to see if they have viewed that forum within the past 24 hours. If they have NEVER visited before at all, it will add a new row with all their corresponding data. If they HAVE, but HAVEN't visited in the past 24 hours, it will UPDATE their row (by IP address) and set hits to $hits + 1 and update the time. If they HAVE visited and HAVE visited in the past 24 hours, it will do nothing, as to try and keep stats pretty accurate.
Then to add up the stats you just do a SUM(hits) thingie with mysql.
Hope I wrote that to where you can understand it lol.
-
Ah, I didn't see that part about the edit password. I would assume you would have two files: profile.php and useredit.php.
The coding required for useredit is more than I feel like writing at the moment, but to change a password you need 3 text input fields: current password, new password, confirm new password. You would pass those three variables off to a process which will convert 'current password' to md5 (You ARE using md5, aren't you?), retrieve the users current password from the database, and compare them. If they are the same, it will convert the new password and confirm new password to md5 and compare those. If THEY match, you need simply to run a UPDATE query inserting the new password into the database.
Check out this tutorial http://evolt.org/node/60384 if you need help. It's pretty advanced, but if you can understand php-mysql to an extend, it's great. I just download the files and use them any time I need a login system - it's super uber easy to add new fields and it says A LOT of coding.
-
Let's say your table for 'users' looks like this:
id username password name aim yahoo icq website address email
Now, for a basic structure of your profile.php page, you would do something like so:
/*Retrieve the data*/ $id = $_GET['id']; $q = mysql_query("SELECT * FROM users WHERE id = '$id'"); $data = mysql_fetch_array($q); /*To display the data*/ echo "Username: ".data['username'];."<br>"; echo "Name: ".data['name'];."<br>"; echo "AIM: ".data['aim'];."<br>"; echo "Yahoo: ".data['yahoo'];."<br>"; echo "ICQ: ".data['icq'];."<br>"; echo "Website: ".data['website'];."<br>"; echo "Address: ".data['address'];."<br>"; echo "E-mail: ".data['email'];."<br>";
And so on. Now to view a profile, you would go to profile.php?id=x , with x being the id of the profile you wish to view.
-
E-mail me at Admin@2five6.com... I have some source codes you can have.
-
Blueman,
Was it really necessary to post that last link
-
If it uses a PHP function like copy(), it could be because you have PHP sade-mode on. Though it should tell you so in an internal-error output if it is turned on.
-
Did you try changing
if("$pw1" !== "$pw2" ){ header( "Location:Messages1.php?msg=5" ); exit();
to
if((pw1) == !($pw2) ){ header( "Location:Messages1.php?msg=5" ); exit();
-
Never use $_GET unless you have to. The more variables you have in the URL, the bigger chance you have of security explotion.
I would have a hidden field inside a form to hold the value of the message ID, and then when a user pressed 'Reply', the form will submit the id of the message that you are replying to onto the reply.php page.
<?php $q = mysql_query("QUERY TO SELECT ALL DATA FOR THE MESSAGE"); $data = mysql_fetch_array($q); $id = $data['id']; ?> <form action="reply.php" method="post"> <input type="hidden" name="id" value="<?php $id ?>" <input type="submit" value="reply"> </form>
From there, you can access the body of the original message and insert it into the body of the new message, as such:
<?php $id = $_POST['id']; $q = mysql_query("SELECT * FROM messages WHERE id = '$id'"); $data = mysql_fetch_array($q); $message = $data['message']; ?> <textarea name="body"><?php echo $message; ?></textarea>
Or something like that.
-
Opps, my bad.
And thank you darkfreaks.
-
Just let me know if you need anything else.
-
You can use the $_POST method to retrieve values from a submitted form.
So if you have a variable name $subject on your confirm page, you can retrieve them on your action page by doing $subject = $_POST['subject'];
-
This is just an idea I have, and I'm going to write the example off the top of my head, but hopefully it will help. Essentially its a number verification system.
Have this on your page inside the form where the user signs the guestbook. It generats a number from 1-100000 and saves it as a variable. It then asks the user to type the number in the textbox.
$number = rand(1, 100000); echo "$number"; echo "<input type=\"hidden\" value=\"$number\" name=\"realnumber\">"; echo "<input type=\"text\" name=\"usernumber\">";
Here, the script compares the two numbers. If they match, it executes the guestbook script. If else, it well, doesnt.
$number = $_POST['number']; $usernumber = $_POST['usernumber']; if($number == $usernumber){ execute guestbook script } else { echo "Incorrect Verification Code!"; }
Crude example, but I hope it helps.
-
No no no. If you did an update query, all it would do is update the original message, which is not what we want because in order to have a proper Inbox/Outbox, you need to be able to view all messages that were sent in their original state.
What you want to do is do a query to return the 'body' of the first message and then insert it after the body of the new message, so that the contents of the new message and first message will sent to the database together when sending the new(reply) message.
-
Ahh thanks BlueSky, Can I ask your views on purchasing scripts? are they fairly easy to implement or?
I have a huge task ahead in building the website I want and initially i thought this would be the perfect opportunity to learn php, however I seem to have dived in at the deep end :-\
You should never never never buy a script. If you can't code it yourself, you can always find it via Google.
As far as your Reply feature goes, you need to have your script retrieve the first message body from the database and then insert it into the body after the new reply.
Ex:
$id is the ID of the message you are replying to.
$body is the contents of the message you are replying to
The textarea is the data for the body of the new message. Users will type the reply before the $message.
$q=mysql_query("SELECT * FROM messages WHERE id='$id'"); $data = mysql_fetch_array($q); $message_suffix = $data['body']; echo " <textarea name=body>$message</textarea> ";
Very crude example, but I believe that should give you a pretty good idea as to what you asked.
-
I'm not sure if this is the correct category for this, and if it's not can someone please move it?
Anyways
Hey guys, I recently started working on a space-oriented, text-based MMORPG. The game is written in PHP/MySQL, and for now I am calling it "Trinity Conquest". You start off with 1 system and 5 planets and you can expand your empire by capturing / buying new systems and planets. You can assign your population to do various different tasks (mine, commerce, industry, military, etc) and you collect 'resources' which will be used to research new technologies and all that good stuff. Players will also have to ability to buy/sell resources to one another as well as chat.
I just started the project a few days ago, but it is already coming along quite nicely. But as you can imagine, it is A LOT of coding, so I am looking for one or two 'code buddies' to help me along.
I'm looking for people with decent php/mysql skills, and owning a version of Dreamweaver would be a plus.
Email me at Admin@2five6.com or Admin@runecommand.com if you're interested. There is no pay involved for any of us, I just thought it would be nice to make a little web-based game.
simple question on include.
in PHP Coding Help
Posted
If the code required in the include in only required inside an IF ELSE statement, I would assume you could include the file INSIDE the statement.
Ex:
Since it will not execute the code inside the brackets if the statement proves false, I would assume it will not load the file, hence freeing up some processing.
But then again, that's just my theory - I've never tried it.
Can anyone prove me right / wrong?