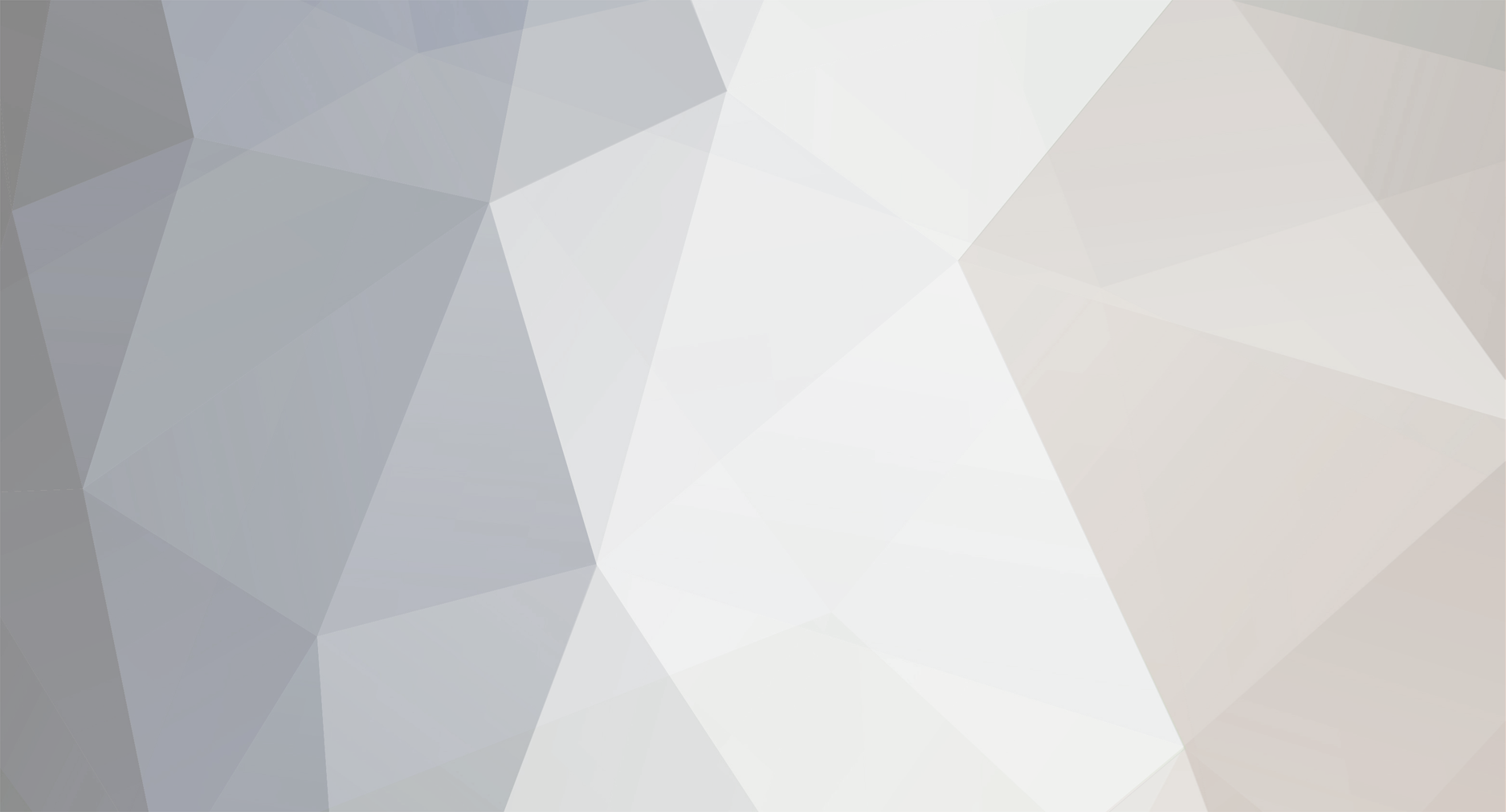
hoopplaya4
Members-
Posts
161 -
Joined
-
Last visited
Never
Everything posted by hoopplaya4
-
[SOLVED] Remove Duplicates from An Array
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
Now that I've finally had some time to look into this issue a bit more, I'm thinking that I haven't explained the issue well enough. So, I'll attempt to re-explain: I have a 'form' where an admin can post a video to a user's account; thus the video is unique to that user. However, the admins may want to post a video to all users (or multiple users) at once. So, for example, let's say that the admin wants to post a video for 4 users: (John, Jane, Jack, & Jill). When he submits the form, the following fields are passed: usrID (this is the usrID of the user who is getting video, e.g., John, Jane, etc). videoURL (this is the URL of the YouTube video) videoTitle (this is the title that the admin gives it, so it is dynamic) videoComments (these are the comments given by the admin, which is also dynamic). videoID (this is an auto-incremented number). Then, the mysql query loops, and does the same thing for the next person, until it finished. Now, when I want to list these videos, it currently would show the following in a table: usrID videoURL videoTitle videoComments videoID John www.youtube.com/abc Funny Clip You will like this clip. 01 Jane www.youtube.com/abc Funny Clip You will like this clip. 02 Jack www.youtube.com/abc Funny Clip You will like this clip. 03 Jill www.youtube.com/abc Funny Clip You will like this clip. 04 However, so that we're not being redundant, I'd like the table to display something like this: usrID videoURL videoTitle videoComments videoID Multiple Users www.youtube.com/abc Funny Clip You will like this clip. 01 It saves a lot of room in the table. -
[SOLVED] Remove Duplicates from An Array
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
Thanks for the replies guys. I'll take a look at these soon, and get back to you. I've got finals today and tomorrow! :-\ -
[SOLVED] Remove Duplicates from An Array
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
Does anyone have any ideas? -
Hey All, I have a form where users can post an item to several users at once. For example, let's say they want to add a video to several users profiles. The following information would be POSTd and then looped: <?php <form class="uniForm" enctype="multipart/form-data" action="addvideo.php" method="POST"> <select multiple style="width:200px" name="name[]" id="name"> $sql = "SELECT usrID, usrFirst, usrLast"; $sql .= " FROM tblUsers"; $sql .= " WHERE usrActive = '1'"; $sql .= " ORDER BY usrFirst"; require("../connection.php"); $rs=mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ print("<option value='" . $row["usrID"] . "'>"); print("" . $row["usrFirst"] . " " . $row["usrLast"] . "</option>\n"); } // end while } else { // No Events found print("No Active players"); mysql_close($link); } // end else ($rs) ?> </select> And here's the PHP that adds the info to the database: <?php require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); $title=$_POST['title']; $name=$_POST['name']; foreach ($name as $nam) { mysql_query("INSERT INTO `tblVideos` (usrID, videoTitle) VALUES ('$nam', '$title')" ); if ($rs) {print" <script> window.location=\"video.php?action=add&msg=2\" </script> "; } else {print" <script> window.location=\"video.php?action=add&msg=1\" </script> "; } } mysql_close($link); And here is the code to display all the videos that were added: <?php $sql = "SELECT * FROM tblVideos, tblUsers WHERE tblVideos.usrID = tblUsers.usrID ORDER BY videoID DESC"; require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link) or die(mysql_error()); if ($rs) { while ($row=mysql_fetch_array($rs)){ print("<tr>\n" ); print("<td>".$row['videoTitle'] ."</td>\n" ); print("<td>". $row['usrFirst'] ." ". $row['usrLast'] ."</td>"); print("</tr>\n"); } //end while } // end if However, this outputs something like this: Really Cool Video Title for John Doe Really Cool Video Title for Jane Doe Really Cool Video Title for John Smith Really Cool Video Title for Jane Smith (etc.) How would I remove the duplicates and have just one instance of: Really Cool Video Title for Multiple Users Please let me know if I'm not making sense! Thanks!
-
[SOLVED] Best way to create Folder Management
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
Thanks msinternet. That helps, I see where you're going with that. -
[SOLVED] MySQL Select (Hopefully Simple)
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
That was it! Thank you very much. Learned something new. -
[SOLVED] MySQL Select (Hopefully Simple)
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
@Flames: The reason was because those numbers are dynamically generated. Thus, I won't always know what the number is. I was only using "32" as an example. Sorry for not being clear. @premiso: That did it, thanks for getting me in the right direction. However, I have one caveat, (and maybe I'm asking too much). In order to pull it off, I had to ORDER BY ASC. But, I'd actually like to display the variables in a descending fashion. Is this possible in my circumstance? -
Hi all, I am looking for some help on selecting variables from MySQL, but leaving out the oldest (or smallest numerical) value. Let's say I have several values (which are auto-incremented) in the DB. For example: 32, 34, 35, 36, 38, 40. When I select these ID's from the Database, I'd like to select and echo all of them except the one which was first created. In the example above, it would be "32". How would I go about achieving something like this? Thanks!
-
Hi All, I've never done anything like this before using PHP and MySQL, so I'd very much appreciate if someone could point me in the right direction. I currently have an area where admins can consistently post material into the database. The admins also have the ability to add or create their own "folders" in the database, which helps to categorize the material they're uploading. Right now, the current table structure I have is this: CREATE TABLE `tblMaterials` ( `materialID` int(11) NOT NULL auto_increment, `materialFile` varchar(30) default NULL, `materialFolder` varchar(30) default NULL, `materialTitle` text, `materialDescription` text, `materialCreate` timestamp NOT NULL default CURRENT_TIMESTAMP on update CURRENT_TIMESTAMP, `materialPostby` varchar(30) NOT NULL default '', PRIMARY KEY (`materialID`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=36 ; Now, I don't have any problems posting new material, but I'm not sure how to list all the "folders" and then display the "material" within the folder. It's almost as if I need a "folderID" or something. Here's what I currently have: <? $sql = "SELECT DISTINCT materialFolder"; $sql .= " FROM tblMaterials"; $sql .= " ORDER BY materialFolder"; require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ print ("<tr>\n"); print ("<td width='39px'><img src='../css/images/folder32.png' border='0'></td>\n"); print ("<td><h2><a href=material.php?action=viewMaterial&name='" . $row["materialFolder"] . "'>" . $row["materialFolder"] . "</a></h2></td>\n"); print ("</tr>"); } //end While ?> </table> <? } } function viewMaterial() { $sql = "SELECT * FROM tblMaterials WHERE (materialFolder = " . $_GET['name'] . ")"; require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ echo $row['materialID']; } } }
-
Does anyone have any other ideas on how I might achieve this?
-
Okay, thanks. Any ideas of how I might set this up, or should I take it from a completely different approach?
-
Hi Premiso: Thanks for the reply. My apologies, I posted the wrong code. I've corrected it in my original post above. Perhaps you can take a look at it. Should I put a 'for each' in there? If so, how would I put it in? Thanks.
-
Hi All, Using PHP & MySQL. I've been trying to work out the logic on this one for a while, but I just can't figure it out. But, I think I'm close!! I have several First and Last names in a form, which are in an Option field that is being pulled from the database. Users have the ability to select multiple options at once. As a result, these are being $_POST'd as an array. Once the form is submitted, I'd like to echo back all the First & Last names that were posted. Right now, it only displays one name. Here's the Form: <form name="filterplayer" method="post" action="<?= $_SERVER['PHP_SELF'] ?>?show=2"> <select multiple name="players[]" id="players" size="5"> <?php $sql = "SELECT usrID, usrFirst, usrLast, usrPosition"; $sql .= " FROM tblUsers"; $sql .= " WHERE usrActive = 1"; $sql .= " ORDER BY usrLast"; require("../connection.php"); $rs=mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ print("<option value='" . $row["usrID"] . "'>"); print($row["usrFirst"] . " " . $row["usrLast"] ); print(" (" . $row["usrPosition"] . ")</option>\n"); } // end while } else { // No Events found print("No Active players"); mysql_close($link); } // end else ($rs) ?> </select><br> <input type="submit" value="Show Availability" /> </form> And here is the PHP to Display those options that were Posted: <?php $pname = $_POST['players']; // Get The Form Values $new =implode(' and ',$pname); ////// IMPLODE VALUES //////// $sql = "SELECT usrID, usrFirst, usrLast"; $sql .= " FROM tblUsers"; $sql .= " WHERE usrID = $new"; require("../connection.php"); $rs=mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ $first = $row['usrFirst']; $last = $row['usrLast']; $full = $first . " " . $last; print ("<div>"); print $full; print ("</div>"); } //end while } // end if ?> And all that it prints is: (although I'm selecting multiple values). John Smith
-
Thanks for the reply. The updated PHP code did it for me. date("g:i a F j, Y ", strtotime($info["announceCreate"] .'+2 hours')) Also, thanks for the tip on the SELECT statement. Unfortunately, I'm using MySql 4.12, and apparently "convert_tz" only works with 4.13+. So, I'll have to go with the PHP update for now. Thanks again!
-
Hmm, that seemed to totally throw the hours, minutes, and even the dates. This is what I'm getting back now. (I even tried to use your example of +2 hours). 2:29 pm September 24, 1911 2:24 pm September 24, 1911 1:37 pm September 24, 1911 2:03 pm September 23, 1911 1:45 pm September 23, 1911 When it used to be: 10:55 am November 30, 2008 10:50 am November 30, 2008 10:03 am November 30, 2008 10:29 am November 29, 2008 10:11 am November 29, 2008
-
Thanks for the reply Mchl. using "date_default_timezone_set('America/Los_Angeles');" works in an instance where I will display the current time. For example, "echo date("g:i a")" However, the mysql values remain unchanged. Is there anything else I'd need to add to my statement?
-
Hi All, This is probably an easy question. I have a timestamp in my mysql database that I'd like to display in Pacific (Los Angeles) time. The mysql time appears to be set at GMT. How would I go about displaying the timestamp in Pacific time? Here's my current query right now: <? require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); $data = mysql_query("SELECT * FROM tblAnnouncements ORDER BY announceID DESC LIMIT 5") or die(mysql_error()); while($info = mysql_fetch_array( $data )) { print ("<li>"); print ("<p>" . $info['announcement'] . " "); print ("<br><small>Posted by Johnny on "); print("" . date("g:i a F j, Y ", strtotime($info["announceCreate"])) . "</small></p>"); print ("</li>"); } ?> Any help is appreciated!
-
[SOLVED] How to Strip Tags from YouTube Embed Code
hoopplaya4 replied to hoopplaya4's topic in PHP Coding Help
Hey ProjectFear: Thanks for the reply. That worked out perfectly! I'm still pretty new to PHP, so I appreciate the help. -
Hi All, I'm trying to write a PHP script to strip the embed code of a YouTube Video. For example, with a YouTube embed code like this: <object width="425" height="344"><param name="movie" value="http://www.youtube.com/v/5P6UU6m3cqk&hl=en&fs=1"></param><param name="allowFullScreen" value="true"></param><param name="allowscriptaccess" value="always"></param><embed src="http://www.youtube.com/v/5P6UU6m3cqk&hl=en&fs=1" type="application/x-shockwave-flash" allowscriptaccess="always" allowfullscreen="true" width="425" height="344"></embed></object> I'd like to strip it down to just this: 5P6UU6m3cqk I know that I would need to use "str_replace" or perhaps "strip_tags" but I'm not sure how to go about doing so. Any help would be very much appreciated!
-
Thanks for the reply fellas. Well, I tried to make the grammatical changes, and added the debug, and it's still not working. The debug error I'm getting is "Query was empty." I think this has to do with me passing the ID. Any IDeas? (Sorry for the pun).
-
Okay, so I've been trying to figure this one out for the last few hours, and I can't tell what's wrong. I'm trying to Update my database from a few fields, and it doesn't update. Here's my form fields: $sql = "SELECT * FROM tblPractices WHERE (practiceID = " . $_GET["num"] . ")"; require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); if ($rs) { while ($row=mysql_fetch_array($rs)){ print("<h1>Edit Practice - ".$row['practiceDate'] ."</h1>" ); print("<div id='communicate'>"); ?> <form class='uniForm' enctype='multipart/form-data' action="updatepractice.php" method='POST'> <? print("<table width='80%' cellpadding='0'"); print("<div class='ctrlHolder'>"); print("<fieldset>"); print("<legend><a href=uploads/".$row['practiceFile'] ."><img src='../images/dl.png' border='0' /></a> " ); print("<a href=uploads/".$row['practiceFile'] .">Download File</a></legend>" ); print("<div style='float:left'>"); print("<p ><i>Posted By:</i> ".$row['practicePostby'] ."</p>"); print("</div>"); print(" </fieldset>"); print("</div>"); print("<div class='ctrlHolder'>"); print("<fieldset>"); print("<legend>Coach's Comments</legend>"); print("<textarea id='styled' cols='115' rows='6' wrap='soft' type='text' name = 'email'>".$row['practiceComments']."</textarea>"); print("</fieldset>"); print("</div>"); print("<div class='ctrlHolder'>"); print("<fieldset>"); print("<legend>Private Comments</legend>"); print("<textarea id='styled' cols='115' rows='6' wrap='soft' type='text' name = 'phone'>".$row['practicePrivate']."</textarea>"); print("</fieldset>"); print("</div>"); print("<div class='buttonHolder'>"); print("<fieldset>"); print("<br><br>"); print("<input class='submitButton' type='submit' name='submit' value='Add Practice'>"); print("<div style='float:left'>"); print(" « <a href='#' onClick='history.go(-1);return true;'>Go Back</a>" ); print("</div>"); print("</fieldset>"); print("</div>"); print("</table>"); ?> <input type="hidden" name="pid" value="<?= $_GET["num"] ?>"><? print("</form>"); print("</div>"); } //end while } // end if And here's the php: <? require("../connection.php"); $rs = mysql_db_query($DBname,$sql,$link); $comments=$_POST['email']; $private=$_POST['phone']; $practiceid=$_POST['pid']; $sql = "UPDATE tblPractices SET practiceComments = '$comments' "; $sql .= "practicePrivate = '$private'" ; $sql .= "where practiceID = '$practiceid'"; echo $sql; if ($rs) { print ("<p>Complete!</p>"); } // end if else {print ("<br><br>Practice was not updated. <br>\n"); } ?>
-
Those are all great examples. Thanks for the help. I knew there was a way, I just needed to know how to go about doing it. Take care.
-
Here's an easy question (I think). Is it possible, when doing a MySql Query to include a date() string? For instance, if I'm doing: SELECT * FROM table WHERE field LIKE Would it be possible to enter in something like "date(Y) after "LIKE?" If so, what is the syntax? Thanks!
-
Thanks for your help. I went ahead and went with your first suggestion. This should suffice for me to get by right now. Take care!
-
Thanks for the reply. No, the date column is just plain text. For example, 10/22/08. It is posted from a date picker.