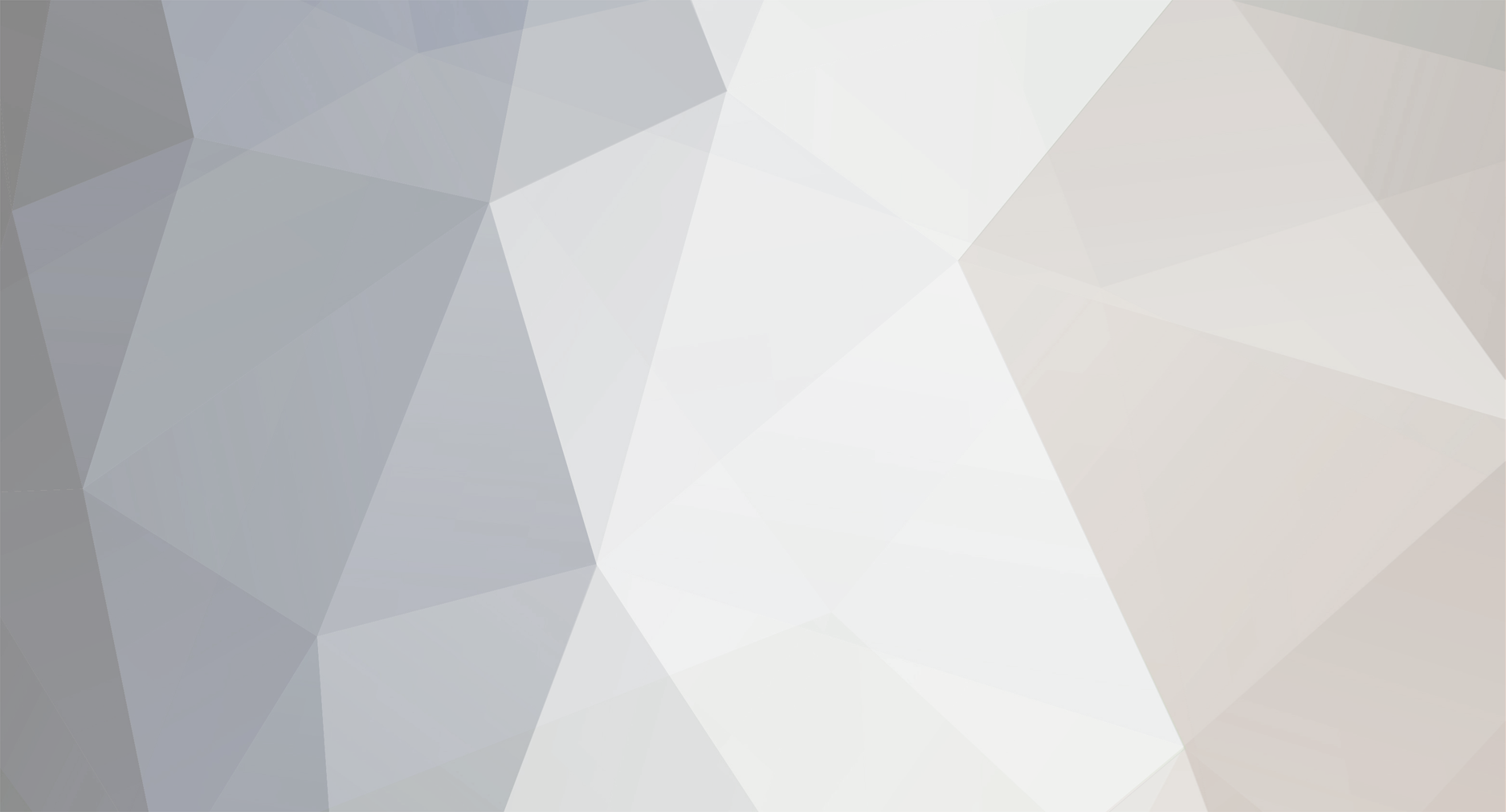
dannyb785
-
Posts
544 -
Joined
-
Last visited
Posts posted by dannyb785
-
-
I honestly don't understand what that does. i have completely ignored function and return and @ up till now. I never really needed them. What does this do? it seems like it would just return true no matter what path you put in there. how do I call the function? how do i use it in a variable or if statement? sorry for being so ignorant. thanks!
put the function somewhere at the top of the php page, and instead of typing file_exists(), which is a php library function, call fileExists, which is the custom function, so your code would look like
<?php function fileExists($path){ return (@fopen($path,"r")==true); } //stuff here $jpg_test = fileExists($jpg_path); $jpeg_test = fileExists($jpeg_path); $gif_test = fileExists($gif_path); $png_test = fileExists($png_path); //other stuff ?>
-
well good thing someone understood
-
First I must say great job explaining your problem; seems like many guys just say "I cant do this, help". What you need to have first, is a php page that updates the database row based on which link was clicked, like "changepublic.php" or whatever. It would take a $_GET variable and modify the user's 'public' value based on the $_GET value. then here's the code you could have:
note: I fixed your html code because you didn't seem to put an opening <td> tag in your while loop
while ($row = mysql_fetch_array($result1)) { echo '<td><p id="images"> <a href="images.php?id='.$row['id'].'">Images</a></td> <td><a href="http://' . $row["links"] . '">Link</a> </td> <td><a href="delete.php?id='.$row['id'].'">Delete</a> </td> <td>Public: '; if($row['public'] == "disabled") echo "<a href=changepublic.php?changeto=enabled>Click to Enable</a>"; else echo "Enabled"; echo ' | '; if($row['public'] == "enabled") echo "<a href=changepublic.php?changeto=disabled>Click to Disable</a>"; else echo "Disabled"; echo '</td>'; }
-
try it with the following function, I've had similar problems with file_exists, and I found this function that worked for me:
<?php function fileExists($path){ return (@fopen($path,"r")==true); } ?>
-
So you have a table, which has all the content/html that you want on each page? Like search.php has a row in a table and when someone goes to index.php?location=search.php, it access that row and spits out the corresponding html? I can't imagine that being anymore useful or efficient than just creating a separate page for each php file. I just have a bunch of php files with my config/header/footer files in include() functions in all of them.
-
Sorry, I still have no idea what you're trying to accomplish and what going wrong in the process. I gather you want some kind of script to read what's in between a '[' and ']'? Like with bbcode? But even if that's the case, I don't understand your problem enough to be able to help.
Just post a piece of your code where it's not acting like you want it to act, explain what you want it to do, and explain the wrong output/error you're getting, then we can probably help.
-
Do you that, for example, if your $word is "bob" that all images within the $definition with "bob" in their image name are also being replaced?
This is a great question and I googled it and many other forums had the same question but no concrete answer. I am going to think about this alot bc while there may be very difficult ways to do it(like something that parses every word in the string of text and replaces the word only if it's not within a tag) but there must be an easier way to do it.
-
I'm going to guess that almost noone has any idea what you're even asking. Example code helps the best, but when you say "the code is going to go crazy", you have the describe what you even mean by that.
-
perfect, that fixes it, thanks! but I dunno how to set a topic as solved
-
You can just add the variable in your html page before you include the scripts, using dannyb785 example, you can just replace the javascript part by
<script type="text/javascript"> var my_step_min = <?php echo $stepMinute; ?>; </script> ... later on your js file ... // check if my_step_min is defined, if not, create a default value ..... ..... timeformat:'h:m', stepMinute:my_step_min} .....);})
yessss very true, forgot about that way
-
I've got a search page that spits out results that match the term that was input. Within the results, I want to add a span with a yellow background behind just the search term. I'll show my code, then say my problem
<?php $search = (term in $_GET variable that was searched for); // while loop that goes through all results, $result is the text that the searched term was found in $new_result = str_ireplace($search, "<span style='background:yellow'>$search</span>", $result); echo $new_result; // end while loop ?>
my problem is that if somebody searches for the word "bob", then if the term "Bob" was found, when it outputs the $new_result, it shows as "bob" with the yellow background. Searching for "Bob" would give the correct results("Bob" with a yellow background), but basically when the output is given, the case of each letter is displayed as it was typed in the search field.
-
In most jquery documentation, they recommend making the function call within the html page itself and not in a separate javascript file. There is no way to dynamically change the settings in a .js file. The only way I can think of is to have htaccess create a .js file by using a rewrite rule to access a php file that spits out the javascript code.
-
This doesn't answer your question, but since all resumes are formatted differently, why even have a script than scans them for anything? I mean unless you're scanning them for profanity or something, in which case you should just have a 'notify' option for someone to report the resume in that case. In most jobs sites, you can include your resume, but you're still required to enter your name, address, etc separately so that the info may be inserted into the site's database for indexing purposes.
The main question is "What are you scanning for?" and figure out a way to get that information by other means.
-
You need to redeclare the session_start() function at the beginning of editprofile.php. You're trying to access $_SESSION['id'] in the UPDATE query, but since the session_start() wasn't executed, the session isn't the same as the previous page.
Personally, I always have session_start() in my config.php file so that I never forget to do it
-
Is there a reason you can't just use the width/height properties within the image tag? I guess if you're resizing huge files, I'd understand but otherwise, thats the easiest
-
When you say what you tried hasn't worked, what have you tried? You didn't mention anything about htaccess, which is what would solve this problem, since it is relating a special url with what is displayed on the page. You would put this code in your htaccess file. This code probably isn't exact, but it's the right idea
RewriteEngine On RewriteRule ^([a-zA-Z0-9_-]+)/([a-zA-Z0-9_-]+)$ specialpage.php?variable=$2 [NC]
-
Are you saying that the page with the datepicker form would have a $_GET['equip'] variable(like page.php?equip=xxx), and that you would check something in a database using the 'equip' value, and depending on the results, you need the stepMinute would be 15, 30, or whatever? That's how I read your question.
If so, you would call the datetimepicker() function anywhere on the page and then when you get to the 'stepMinute:' part of the function call, you would echo the value, based on whatever your results were from the sql call. So something like
// page header stuff <body> // html stuff here <?php $equip = addslashes($_GET['equip']); $query = "QUERY TO ACCESS TABLE BASED ON EQUIP VALUE"; // process query // figure out the needed stepMinute value based on the info grabbed $stepMinute = whatevervaluehere; ?> $(document).ready(function(){ $('#res_start').datetimepicker({ ampm:true, timeformat:'h:m', stepMinute:<?php echo $stepMinute; ?> }); }) // rest of html of page
-
I figured it out. You have $_FILES['Uploadfile']['Name'], but the names of the values in the array of the $_FILES variable are all lowercase and you have "Name". Change it to "name" and you should be good.
You have $_POST[Name] which is correct because is corresponds to the input fields you specified
-
I want key words on my website to be linked for efficiency reasons for my customers and for SEO purposes. When people are reading an article and they come across a word they don't know, I want it to be linked the way they are in wikipedia.
You may not understand what he(who posted above you) meant. Anytime the text is displayed on a web page, it would convert the keyword into a link, so any web crawler would see the hyperlink. It's just in the table itself that there would be no link; and crawlers don't access database tables to index their results so his way is totally fine
-
It's a plugin. Plugins are registered as Wordpress loads. If after it has loaded there are any attempts to send headers there will be an error because this plugin outputs data when it is loaded into Wordpress.
Sorry, I don't work with wordpress, I assumed that the code specified was the beginning and end of the page trying to be viewed. I didn't realize the code itself was a plug-in. Oh well, the thought was still there, search for when headers are sent and eliminate all text that comes before it
-
I honestly don't know what any of that stuff is. But based on their brief descriptions, they all seem web-related. oh well, this is a question about semantics, and the person asking the original question clearly had no idea what they were asking
-
Thanks DannyB, I am getting closer.I have a better error now.
Error: target=documents/, tmp_name=/var/tmp/phpdv7Xk9Data has saved in the database except the file location. File did not find it's way to the folder.
I may be incorrect, but I don't believe you need the slash at the end of 'documents'. Try removing it. Also, this may be crazy, but make sure that folder actually exists. A last thing to check is that 'documents' is a folder in the directory that this php script is located
-
Indeed the problem would be trying to send headers after output has been sent, which is why a plugin should not send output until it is asked to do so.
obviously in his code, there are headers sent after output(somewhere in the functions that were called), but there's no attempt to send headers after those last 7 lines so I don't see how they are any part of the problem
-
You can have html without javascript but you can't have javascript without html.
Um, indeed you can. That is like saying PHP is useless without HTML, but you can build applications with PHP that have nothing at all to do with HTML or the web. Same goes for JavaScript.
Can you give an example of javascript being used without html?
And what applications in php are used that are unrelated to the web? PHP means "Hyptertext preprocessor", so I'd say in its definition it's used for html(hypertext markup language)
EDIT: now that I think of it, I suppose php can be used to move files around and do server-side things that don't require html to be output, but it'd still require a browser or similar program to begin execution(even with cron jobs)
file_exists issue?
in PHP Coding Help
Posted
Look at the function, if it determines the file to exist, it returns true. Yeah you shouldn't do your statements like
do them like