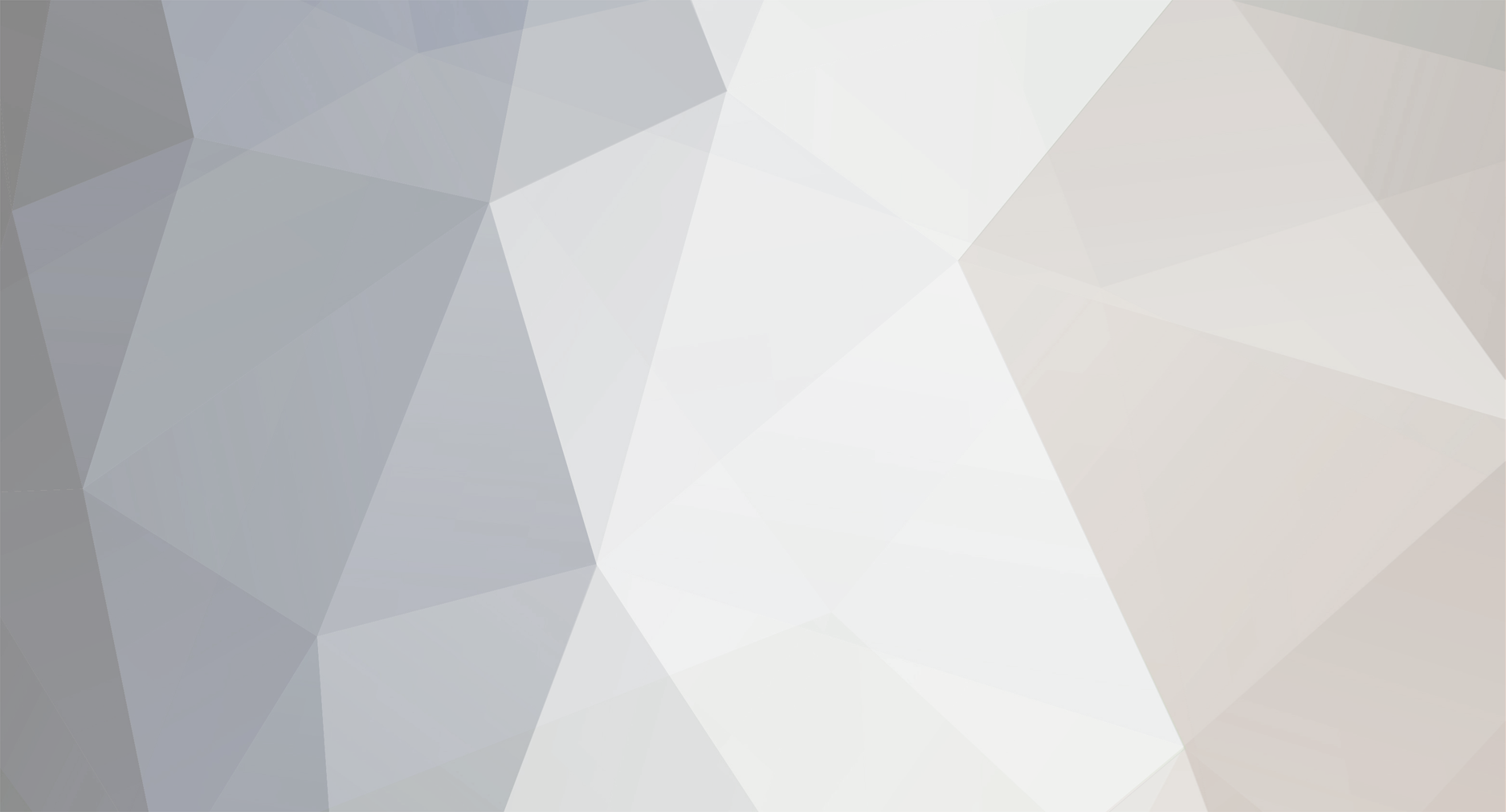
radar
Members-
Posts
645 -
Joined
-
Last visited
Everything posted by radar
-
parseInt is your friend!
-
if(document.getElementById('order_amount').value >= document.getElementById('cpc').value) { document.getElementById('order_amount').value = document.getElementById('cpc').value; } this works, up until 99 as soon as you type 100 into the order ammount, it doesn't recognize 100 being greater than or equal to 30 any idea's as to why? the 2 form fields for this look like: <input id="order_amount" maxlength="3" name="order_amount" size="3" type="text" value="1" onchange="calPrice('{$data.sale_price}');" /> <input name="cpc" type="hidden" id="cpc" value="{$data.qty_pc}">
-
Ajax get $_POST for preview, but cant submit post.
radar replied to eevan79's topic in Javascript Help
run a body onload command to preload the image. just like you do with JS rollovers. -
since you're combining your HTML, with your Javascript with your PHP, you'll want to create an entirely seperate PHP script to handle any and all ajax calls that you may have... then you will change: var url = "index.php?pg=blah&id="+id+"&int="+myRand to point to the proper place to be run and remember to always add the &int="+myRand to prevent browser caching. my recommendation for you is to create a php file called calls.php then in there you would put: <?php $_pg = isset($_GET['pg']) ? $_GET['pg'] : ''; $_id = isset($_GET['id']) ? $_GET['id'] : ''; switch ($_pg) { case delete_customers: mysql_query("DELETE FROM whatever WHERE id = '".$_id."'"); echo html here; break; } ?> doing this you can add multiple cases and achieve endless ajax calls from one file but doing very different things.... me personally, i keep all my JS in ajax.js, keep all css in style.css, keep all html in page_name.tpl, keep all php in index.php but i use a template engine that allows me to do that properly...
-
alert all of these: form.behandler.options.selectedIndex form.omraede.options.selectedIndex form.navn.options.selectedIndex somewhere along the lines omraede is getting lost.
-
yeah it should be the show_confirm(" . $id .");'>"; instead.
-
Load specific DIV from an external page into another page
radar replied to nummer31's topic in Javascript Help
Really in order to a specific DIV you would have to load the entire external file, and trim it down so the output is only that specific div... good luck -
alright i figured this out... i first tried just a regular select * from table this of course brought all of them... i then tried lowing it down to 1 left join, didnt quite work as planned.. after about 10 different tries with all the same result i decided to try to add cust.id into my group by line, so now i have GROUP BY c.c_id, d.c_id, cust.id and it worked perfectly. i now get all of the results expected.
-
Alright so I am joining 3 tables, 2 tables are only brought in for the count. it counts just fine, but i have 7 records in the database but only 3 of them are being displayed... $sql = "SELECT SQL_CALC_FOUND_ROWS cust.id, cust.f_name, cust.l_name, cust.email, cust.password, cust.status, COUNT( o.id ) AS orders_cnt, COUNT( d.id ) AS deals_cnt FROM customers AS cust LEFT JOIN orders AS o ON o.c_id = cust.id LEFT JOIN deals AS d ON d.c_id = cust.id GROUP BY o.c_id, d.c_id ORDER BY cust.l_name"; and the output in array form from this join is: Array ( [0] => Array ( [id] => 2 [f_name] => [l_name] => => tech@mysite.com [password] => [status] => 1 [orders_cnt] => 0 [deals_cnt] => 0 ) [1] => Array ( [id] => 1 [f_name] => Justin [l_name] => Brown => justin@mydomain.com [password] => li26tuvs [status] => 1 [orders_cnt] => 0 [deals_cnt] => 1 ) [2] => Array ( [id] => 12 [f_name] => k [l_name] => k => keith@mysite.net [password] => y1hiq6oc [status] => 1 [orders_cnt] => 0 [deals_cnt] => 2 ) ) so with that join is there any reason only 3 of them would be displayed? even running is through phpMyAdmin retrieves only 3 of them as well.
-
it's not as difficult as it seems... the first javascript function is left alone... the showConfirm javascript function is where the changes will happen... first change: show_confirm(id){ (i missed that in my edit) now to explain the code a little.. req=AjaxObjectCreateGeneral(); this is taking the ajax request into a variable is really all it is doing if(req) { if the ajax connection is there do the following var myRand = parseInt(Math.random()*99999999); we create a random number to prevent browser caching var url = "index.php?pg=blah&id="+id+"&int="+myRand - here we are setting up our request url (the PHP script) notice the id="+id, this is the id that is passed through to the function req.onreadystatechange = function() { ajax.onreadystatechange -- not sure what it means (i dont use this method myself), but it seems to work (when i used to use it) if(req.readyState == 4) { if readyState = 4 (forget what 4 means) if(req.status == 200) { if status = 200 (200 is basically saying everything is ok and im done) //alert(req.responseText); - with this you could do a multitude of things.. if you are outputing HTML you can do document.getElementByID(page).innerHTML = req.responseText; or you can even do window.location.href="eatit.html"; or whatever you wish to do really. you can also further your checking like: if(req.responseText == 0) { alert('error! could not do something'); } else { document.getElementByID('page').innerHTML = req.responseText; } } } }; req.open("GET", url, true); -- this is the open command, you can use GET, or POST, the url is the page we're opening, and the last means asynchronys or not asynchronys -- when i used this method i always used true. req.send(); this sends the .open command and you can also use this when using a POST command like: req.send(formPars) or something of the like. } hope this makes you a little less confused, and a bit more knowledgable about what the code does.
-
ajax is definately the way... you would do an onChange="doSomething();" on the drop down.. then you'd run regular ajax to call/read the output from a php file.... you'd probably be best @ taking the results and putting them in a delimited form such as: name:address:city:state:zip:grade:gpa etc.. then in javascript exploding that into an array and using document.getElementByID(id).value = to set each individual value... otherwise you could throw your entire form into a span with an id or a div with an id and using PHP to echo out the entire HTML for the form and then using this inside javascript: document.getElementByID(id).innerHTML = returnText
-
you're right this is easy... your html button: <input type="submit" name="button" id="button" value="do something" onClick="dothis(<?php echo $id; ?>);"> your javascript: function AjaxObjectCreateGeneral() { var req; // The variable that makes Ajax possible! try{ // Opera 8.0+, Firefox, Safari req = new XMLHttpRequest(); } catch (e){ // Internet Explorer Browsers try{ req = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try{ req = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ // Something went wrong alert("Your browser broke!"); return false; } } } return req; } function show_confirm(){ var r=confirm("Do you really wish to delete this table?"); if (r==true) { req=AjaxObjectCreateGeneral(); if(req) { var myRand = parseInt(Math.random()*99999999); var url = "index.php?pg=blah&id="+id+"&int="+myRand req.onreadystatechange = function() { if(req.readyState == 4) { if(req.status == 200) { //alert(req.responseText); } } }; req.open("GET", url, true); req.send(); } alert("Database was succesfully deleted.."); }else { alert("Database was not deleted."); } } that should get you started.. though look @ using prototypejs for your ajax (you still have to write your own functions) it's functionality is hard to surpass. I modified my code to fit within your requirements of needing a confirm box prior to running the PHP code.
-
http://php.net/manual/en/control-structures.switch.php will get you started with the switch... i think it makes for easier reading down the road... not as many if elseif elseif elseif elseif else statements needed (if any at all).
-
do it something like this $_sk = isset($_REQUESt['sk']) ? $_REQUEST['sk'] : ''; $_id = isset($_REQUEST['id']) ? $_REQUEST['id'] : ''; switch ($_sk) { default: // the main page of the website break; case vid: // selected video page.. select * from table where id = $_id echo html here... or stop PHP display HTML with php elements added into it, and then restart PHP... break; }
-
if onselect is 'other' then un-disable next field.
radar replied to jasonc's topic in Javascript Help
onChange="check_select(); return false" function check_select() { id = document.form1.elements["selection"].selectedIndex; vel = document.form1.options[id].value if (vel == other) { document.getElementByID['textbox'].disabled = false; } else { document.getElementByID['textbox'].disabled = true; } } something like that could work.. though it's not tested. -
how to change value of hidden field using javascript?
radar replied to jasonc's topic in Javascript Help
document.formname.getElementById('hiddeninput').value = 'boo!'; should do the trick. much like the code above me states, except in a shorter fashion. also keep in mind that users who are using ie8 will get a less than perfect response from your code... reasining behind this it seems as though IE8 doesn't negate a href command when it runs into an onclick command.. and so you may want to do something like: href="index.php" onclick="changeHidden(); return false" that way it forces ie8 to not use the href. -
thats because you dont have a $quer5, your $noticia5, uses $quer4 so try to echo that out and see what you come up with.
-
Well aside from this not being true ajax, since the point of ajax is to not have the screen refresh and you have reload(this.form) repeatedly... you should have a few different functions for each dropdown in a different javascript file that you include into your html file.. then you have your functions that the javascript file calls, in a php file that isn't called by anything but the javascript file... i personally use switches for that with that out of the way, have you tried to echo out $noticia5? perhaps putting in (after the last echo"</select>" echo "<pre>"; print_r($noticia5); echo "</pre>" will give you some insights as to what is going on with it...
-
Ajax get $_POST for preview, but cant submit post.
radar replied to eevan79's topic in Javascript Help
why not just do <input type="submit" value="Preview" name="preview" class="signsub" id="preview" onClick="showPreview(); return false"/> <input type="submit" name="submit" id="submit" value="Submit" /> one form, 2 buttons.... add 10 more that way if you want -- it's all in the way you handle the processing of those buttons. also when using ajax, you don't need an action because the action should be in the ajax itself or passed into the ajax through a parameter. -
By looking at your code you've got an odd way that you're doing your error checking... might i suggest doing your error checking all at once like: if($_SESSION['pass_phrase'] != $phrase) { $e[] = "Pass Phrase doesn't match"; } then when you get all your error checking done like so, to check if there are any errors you simply: if(sizeof($e == 0) { // everything is good } else { // there is at least 1 error } this makes it easier to debug, and makes it easier to read. A sample of this would be like this, i have the sneaking suspicion i am missing a } somewhere in the code probably right @ the very end..... You may also want to look and make sure I have all the error checking done... but doing something like this instead of how you had it would theoretically work a bit better than how you had it coded. <?php session_start(); error_reporting(E_ALL); require_once('cons.php'); require_once('aprs.php'); // Get the variable sent by AJAX script if it is typed in // Connect to the database $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); $_un = isset($_REQUEST['username']) $_REQUEST['username'] : ''; if ($_un != '') { $ok = 'okay'; $den = 'denied'; // Secure the information $passname = mysqli_real_escape_string($dbc, trim($_REQUEST['username'])); // Lookup the username in the database $query = "SELECT username FROM info WHERE username = '$passname'"; $data = mysqli_query($dbc, $query); // Allow some time to get the response sleep(3); // If 1 is returned that name exsists, if 0 then we can move forward if (mysqli_num_rows($data) == 0) { // Send okay back to the ajax script so it knows to stop bothering the user echo $ok; return $ok; } else { // Send denied back to the ajax script so the user knows that name is taken echo $den; return $den; } // Close If / Else statement } // Close (isset($_REQUEST['username'])) { // Start of Main Submit Function Script if (isset($_POST['submit'])) { // Grab the profile data from the POST and secure it $avatar = mysqli_real_escape_string($dbc, trim($_POST['avatar'])); $usern = mysqli_real_escape_string($dbc, trim($_POST['username1'])); $password1 = mysqli_real_escape_string($dbc, trim($_POST['password1'])); $password2 = mysqli_real_escape_string($dbc, trim($_POST['password2'])); $email = mysqli_real_escape_string($dbc, trim($_POST['email'])); $side = mysqli_real_escape_string($dbc, trim($_POST['side'])); $class = mysqli_real_escape_string($dbc, trim($_POST['class'])); $vercap = mysqli_real_escape_string($dbc, trim($_POST['verify'])); // convert username to all lowercase $userna = strtolower($usern); $username = stripslashes($userna); // Check the CAPTCHA pass-phrase for verification $phrase = SHA1($vercap); if ($_SESSION['pass_phrase'] != $phrase) { $e[] = "<div id=\"signconf\">The verification text didn't match the picture.</div>"; } if(!preg_match('/[^0-9A-Za-z]/', $username)) { $e[] = "<div id=\"signconf\">The email you have entered is invalid, please try again.</div>"; } if(!preg_match('/^[^@]+@[a-zA-Z0-9._-]+\.[a-zA-Z]+$/', $email)) { $e[] = "<div id=\"signconf\">The email you have entered is invalid, please try again.</div>"; } if(!empty($avatar) && !empty($username1) && !empty($password) && !empty($password2) && ($password1 == $password2) && !empty($email) && !empty($side) && !empty($class)) { $query = "SELECT * FROM info WHERE username = '".$username."'"; $data = mysqli_query($dbc, $query); if(mysql_num_rows($data) != 0) { $e[] = "<div id=\"signconf\">An account already exists for this username. Please use a different address.</div>"; } } else { $e[] = "<div id=\"signconf\">You must enter all of the sign-up data, including the desired password twice.</div>"; } if(sizeof($e) == 0) { // all is good $hash = md5(rand(0, 1000) ); query = "INSERT INTO"; mysqli_query($dbc, $query)); echo '<div id="signconf">'; echo '<p>Your new account has been successfully created. Please check your Email for the final verification.'; echo '</div>'; // Send the registration e-mail so the user can activate thier account $to = $email; // Send email to our user $subject = 'Signup | Verification'; // Give the email a subject $message = ' Thanks for signing up! Your account has been created, you can login with the following credentials after you have activated your account by clicking the url below. ------------------------ Username: '.$username.' Password: '.$password1.' ------------------------ Please click this link to activate your account: http://www.mydomain.com/verify.php?email='.$email.'&hash='.$hash.' '; // Our message above including the link $headers = 'FroM: me@mydomain.com' . "\r\n"; // Set from headers mail($to, $subject, $message, $headers); // Send our email mysqli_close($dbc); exit(); } else { // all is not so good // here you could foreach through all of the error results above.. i think i got em all. } // Connection to the database is no longer needed so we can close it mysqli_close($dbc); // We no longer need php so let's close that and start the submit form ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title> Registration</title> <link type="text/css" rel="stylesheet" href="tlstyle.css" /> <script src="scripts/utils.js" type ="text/javascript"></script> <script src="scripts/validation.js" type="text/javascript"></script> </head> <body> <div id="csignup"> <div id="signform"> <p>Please enter all the information below to sign up.</p> <form enctype="multipart/form-data" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <label for="username1">Username:</label> <input type="text" id="username1" name="username1" value="<?php if (!empty($username1)) echo $username; ?>" maxlength = "7" /><br /> <label>Password:</label> <input type="password" id="password1" name="password1" value="<?php if (!empty($password1)) echo $password1; ?>" class="jcinput"/><br /> <label>Password (retype):</label> <input type="password" id="password2" name="password2" value="<?php if (!empty($password2)) echo $password2; ?>" class="jcinput"/><br /> <label>E-Mail Address:</label> <input type="text" id="email" name="email" value="<?php if (!empty($email)) echo $email; ?>" class="jcinput"/><br /> <label for="verify">Verification:</label> <img src="captcha.php" alt="Verification" /><br /> <label for="verify">Verification:</label> <input type="text" id="verify" name="verify" class="jcinput" /><br /> <label>Choose Your Avatar:</label> <input type="radio" name="avatar" value="/images/smile.png" /> <img src="/images/smile.png" alt="1" /> <input type="radio" name="avatar" value="/mages/smile.png" /> <img src="/images/smile.png" alt="1" /> <input type="radio" name="avatar" value="/mages/smile.png" /> <img src="/images/smile.png" alt="1" /> <input type="radio" name="avatar" value="/images/smile.png" /> <img src="/images/smile.png" alt="1" /><br /> <label>Choose Your Side:</label> <input type="radio" name="side" value="A" />A <input type="radio" name="side" value="B" />B <div id="signclassb"> <div id="signclass"> Choose a Class: <ul><li><input type="radio" name="class" value="A" /> A</li> <li><input type="radio" name="class" value="B" /> B</li> <li><input type="radio" name="class" value="C" /> C</li></ul> </div> </div> <input type="submit" value="Sign Up" name="register" class="signsub" /> </form> </div> </div> </body> </html> as far as checking email, use this function instead function validEmail($email) { $isValid = '1'; $atIndex = strrpos($email, "@"); if (is_bool($atIndex) && !$atIndex) { $isValid = '0'; } else { $domain = substr($email, $atIndex+1); $local = substr($email, 0, $atIndex); $localLen = strlen($local); $domainLen = strlen($domain); if ($localLen < 1 || $localLen > 64) { // local part length exceeded $isValid = '0'; } else if ($domainLen < 1 || $domainLen > 255) { // domain part length exceeded $isValid = '0'; } else if ($local[0] == '.' || $local[$localLen-1] == '.') { // local part starts or ends with '.' $isValid = '0'; } else if (preg_match('/\\.\\./', $local)) { // local part has two consecutive dots $isValid = '0'; } else if (!preg_match('/^[A-Za-z0-9\\-\\.]+$/', $domain)) { // character not valid in domain part $isValid = '0'; } else if (preg_match('/\\.\\./', $domain)) { // domain part has two consecutive dots $isValid = '0'; } else if (!preg_match('/^(\\\\.|[A-Za-z0-9!#%&`_=\\/$\'*+?^{}|~.-])+$/', str_replace("\\\\","",$local))) { // character not valid in local part unless // local part is quoted if (!preg_match('/^"(\\\\"|[^"])+"$/', str_replace("\\\\","",$local))) { $isValid = '0'; } } if ($isValid && !(checkdnsrr($domain,"MX") || checkdnsrr($domain,"A"))) { // domain not found in DNS $isValid = '0'; } } return $isValid; } usage: $vemail = ValidEmail($_POST['email']); if($vemail == 0) { // error } else { // success }
-
Wordpress might be a great product for your website, and other websites you go. However as a custom programmer, my clients have the websites that are so absurdly difficult to do in any pre-built CMS that it only makes sense to build 100% custom. With that being said, I am however seeing trends in my client websites and i am working on my own CMS that will allow me to do all the things I need to do, such as having the option to use an ajax navigation menu, or to not use an ajax navigation menu. to use smart folders or to use ?pg=blah&i=blah type url system... as well as the ability to add ajax checking and submitting to some form buttons but not other form buttons, and allow me to choose which ajax function to add into the particular button... allowing the ability to add ajax countdown's for items remaining, ajax calendars, etc.. these are things that i think you'd be hard pressed to find WP doing.
-
ImageMagick is still used, but not as frequently as the GD Lib.
-
i still take the stance of 100% custom programming as apposed to using drupal, or joomla, or yes even wordpress.. sure it takes a little longer, but the client gets only the functions they need and/or want.
-
http://www.phpfreaks.com/forums/index.php/topic,115581.0.html is the link to the basic working ajax example. Then of course you'll need to modify it to do what it is you want. I personalled learned ajax using prototype, from the Sams Teach Yourself Ajax, Javascript and PHP all in one book. It gives you a basic understanding of ajax, but gives you the knowledge to be able and expand that to do whatever you want to do.
-
would require ajax. onchange("select_week(); return falase") then the ajax commences, there is an ajax tutorial on this site that can show you how to do this.