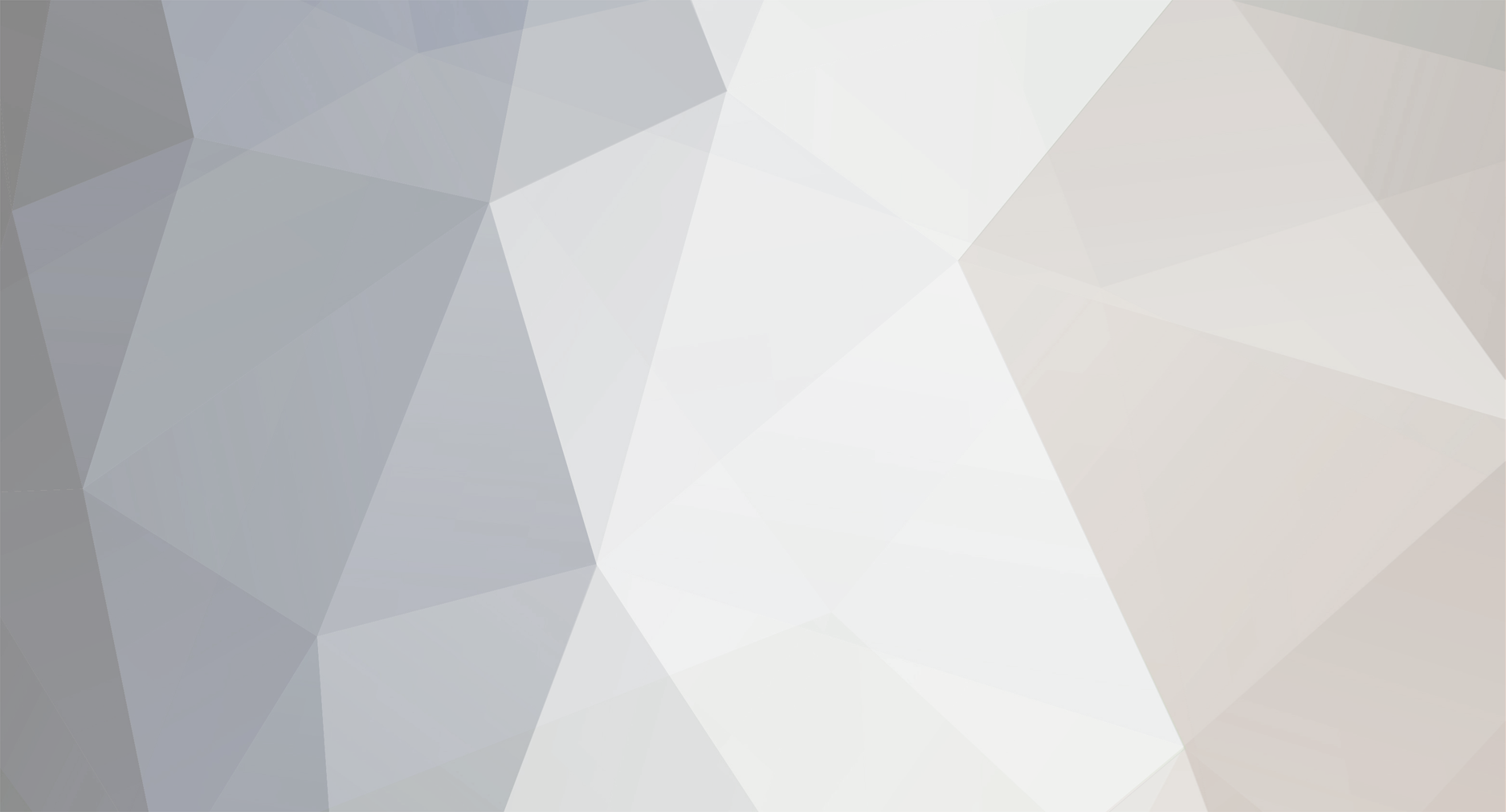
Brian W
-
Posts
867 -
Joined
-
Last visited
Posts posted by Brian W
-
-
please use code tags next time...
try:
if($this->xml->formprocess->contact){ echo $this->xml->formprocess->contact->firstname; $firstname = (string)$this->xml->formprocess->contact->firstname; echo $_POST[$firstname]; }
-
do you get an error message?
-
Look into this quick, easy, well done tutorial: here
-
I agree with Daniel, PFMaBiSmAd, Ken2k7, and probably the majority on phpfreaks... you should upgrade, not downgrade.
If its not your server, change hosts because its not worth it to sit on an old box friend.
Any ways, the one line of code you provided isn't much for us to work off of... does it not work? what does it do when you run it? do you get errors? if so, what are they?
-
I don't know what the JS does because you didn't attach or paste the JS... but I'll venture a guess that variable one in the function DateInput() (in yours, it is 'orderdate') is what the JS uses as the input's name & id. Try:
<?php $mail_msg = "Contact Information\n\n"; $mail_msg .= "Name: ".$_POST["name"]."\n"; //changed the append to .= because its shorter $mail_msg .= "Email Address: ".$_POST["orderdate"]."\n"; //Here I changed "email" to "orderdate" $email = $_POST['email']; mail('email@myemail.com', 'Tour Request', $mail_msg, "From: <".$email.">\n\r"); // Mail to user $mail_user = "Auto-Acknowledgement:\n\n Thank you for contacting Mansion.\n We are in receipt of your e-mail and will respond to your inquiry shortly. \n\n Thank you for visiting at http://mansion.org/weddings/ "; mail($email, "Mansion: Auto-Acknowledgement", $mail_user, "From: Mansion<'weddingcenter@mansion.org'>\n\r"); header("Location: thanks.htm"); ?>
-
if you use the code tags around your php or even html snippets, you will likely get more help
[ code ]Like this but without the spaces in the tags[ /code ]
would be
Like this but without the spaces in the tags
-
In most cases, the answer is yes, its just a matter of what rout you should take.
What OS are you running and what version of that OS is it? Unix, MS Windows (xp, vista, etc) ?
-
But if you are just looking to have it done when you update a score from your application, then you just need another query and run it after the one for updated the grade. Average the scores and UPDATE the grade, right?
-
try
$db = mysql_pconnect("host", "user", "pass");
-
you could set your time zone in the ini or on the fly per page (before anything else) by using date_default_timezone_set('America/Phoenix'); and replace Phoenix with your supported local city found here.
Else, you can use the unixtimestamp returned by strtotime("-10 hours")
(Sorry, reply is for changing timezone in php, please excuse me... I ended up in the wrong board)
-
I'm going to venture a guess that PFMaBiSmAd is going to say something along the lines of "well, that means there is no such file or directory as C:\Program Files\Apache Software Foundation\Apache2.2\htdocs\Counter.php"
-
counting words, not strlen... right?
$words = count(explode(" ", $_POST['text'])); if($words > 100){ //fail code }else { //other code
just a thought
-
No offense man, but you need to start with some basics before jumping into queries and such.
Thanks for your help.
Intended to be sarcastic I'm guessing. I'll be on for about another 15minutes, post your entire page and any include pages and I'll try running through it... but my opinion of starting small stands.
-
Do you have phpMyAdmin? if so, through this into a query (slight mod of kickstart's, no guarantee it will work)
SELECT a.post_id, COUNT(b.comment_id) as `count`FROM posts a
LEFT OUTER JOIN comments b
ON a.post_id = b.post_id
ORDER BY `count`
GROUP BY a.post_id
"LEFT OUTER JOIN" means you are linking the table with whatever is on the left side of the "ON" statement's "=" having to be in existence (if your post is deleted, it won't try counting from the comments for that post). The on statement is how you specify what fields need to be equal. in this case, the post id fields in both tables. "COUNT()" counts how many results were returned from the table comments. If you notice that the tables are names then have "a" or "b" after them, that is called an "alias" and it just simply makes it easier/shorter to write.
Help?
-
No offense man, but you need to start with some basics before jumping into queries and such.
-
can you post all of the code, it may help us figure out the problem.
-
You don't get any errors? do you have error_reporting(E_ALL) on for testing?
$function_to_write($destination_handle, $dest);
That throws up a flag with me at least when I read through it.
-
You need to increment $row
$row = 0; foreach($search_rows as $row) { $alt = ($row % 2 == 0)? 'd4e4fe' : 'bfd4f8'; echo '<tr>'; echo '<td bgcolor="#' . $alt . '">' . $row->branch_name . '</td>'; echo '<td bgcolor="#' . $alt . '">' . $row->address . '</td>'; echo '<td bgcolor="#' . $alt . '">' . $row->city . '</td>'; echo '<td bgcolor="#' . $alt . '">' . $row->state . '</td>'; echo '<td bgcolor="#' . $alt . '">' . $row->zip . '</td>'; echo '<td bgcolor="#' . $alt . '">' . format_phone($row->phone_number) . '</td>'; echo '</tr>'; $row++; }
-
delete the file you are writing to (clean start), and post to it again. How many news items do you have? should be one...
Post a new one. How many do you have? should be two...
do that a few times and see what you get and let us know.
btw, I suggest this code for saving:
<?php if(isset($_POST['author'])){ $author = $_POST["author"]; $zeth = file_put_contents("test.php","<center>Author: " . $author . "<br>" . wordwrap($_POST["news"], 75, "<br />") . "<br><hr><br></center>", FILE_APPEND); } ?>
and this code to output the file's content:
<?php $file = filesize("test.php"); $news = file_get_contents("test.php"); print $news; ?>
-
conditional statement needed...
<?php if(isset($_POST['author'])){ $author = $_POST["author"]; $zeth = file_put_contents("test.php","<center>Author: " . $author . "<br>" . $_POST["news"] . "<br><hr><br></center>", FILE_APPEND); } ?>
-
let me get this right, your include file has links that are relative? When you include it, the links relativity is broken and you obviously can't have that. Right?
-
Bit off a little more than you could chew? I suggest starting with tutorials or the freelance board (hire someone). You'll likely want to do this yourself, so here is some links to start out with:
-
lol, I noticed mine is broken anyways. its returning them in the wrong order...
-
wow, you make that look hard... lol
<?php $x1 = (string) rand(1001, 9999);//lowest number, highest number $digits = str_split($x1);//spilits it into digits echo "The number $x1<br><br>"; $places = array("Ones", "Tens", "Hundreds", "Thousands", "Ten thousands", "Hundred thousands"); foreach($digits as $place=>$digit){ echo $places[$place]." is ".$digit."<br>"; } ?>
Have fun with that one, let me know if you want it to work differently. The educational system needs more interaction with computers.
Retrieving Image Data From a mysql Database
in PHP Coding Help
Posted
Assuming that the image is being stored in the same folder as the file getImage.php, this is what you need I think...