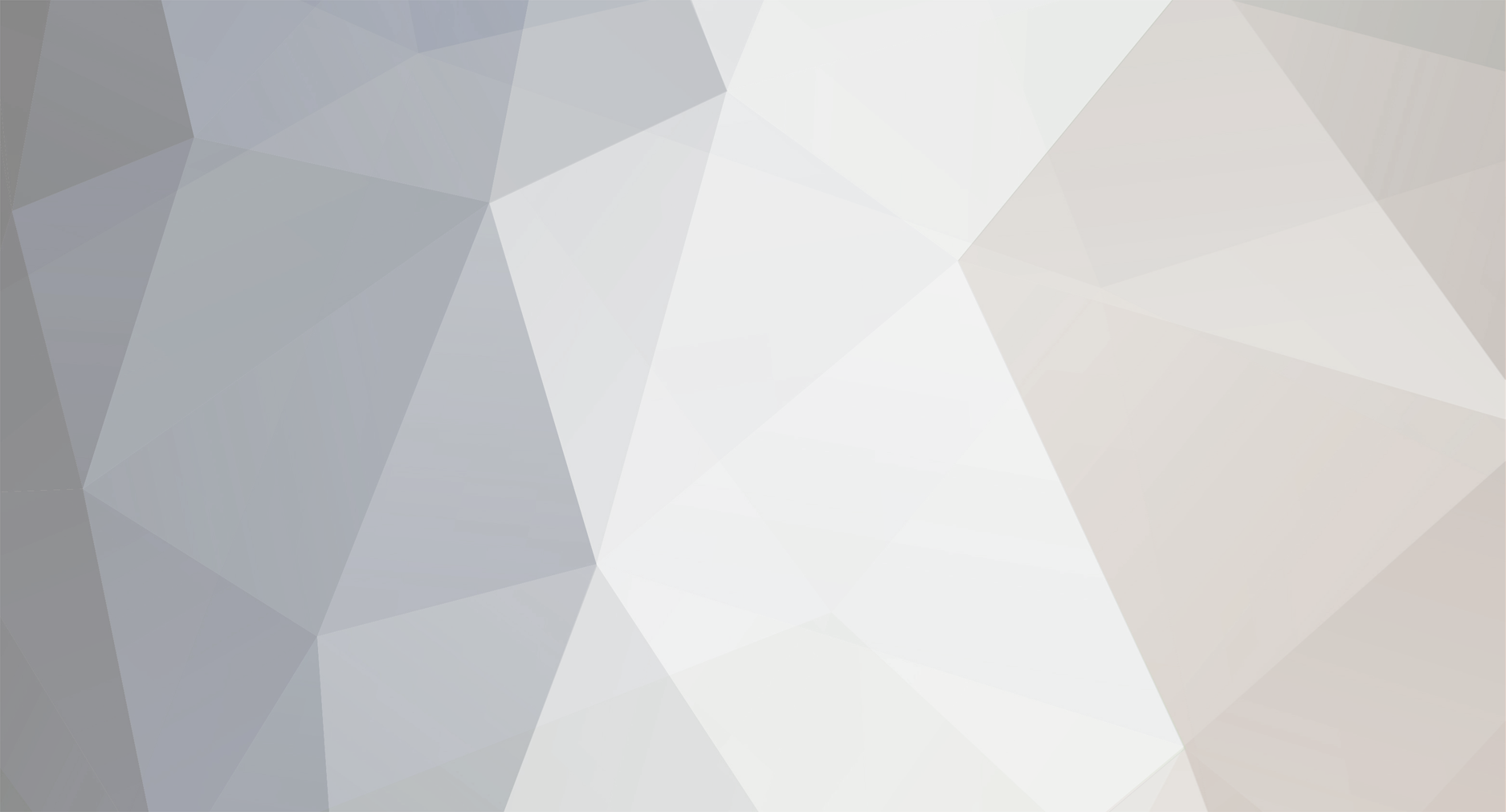
Brian W
-
Posts
867 -
Joined
-
Last visited
Posts posted by Brian W
-
-
do you have error reporting on in your INI?
if not, add this to the top:
<?php error_reporting(E_ALL); ?>
This ran by itself should not work because $result doesn't exist...
<?php echo "<table align=\"left\" border=\"1\" cellspacing=\"0\" cellpadding=\"3\">\n"; echo "<tr><td><font size=\"2\">\n"; for($i=0; $i<$num_rows; $i++){ $uname = mysql_result($result,$i,"username"); echo "<a href=\"userinfo.php?user=$uname\">$uname</a> / "; } echo "</font></td></tr></table><br>\n"; ?>
-
won't slow it down enough to notice... milliseconds maybe.
Its well worth the advantages of not having repeat code.
-
Is it giving you an error? The brackets balance...
-
<?php session_start(); include "db_connect.php"; if($_SESSION['id']) { $sql = "SELECT username FROM `users` WHERE `id`='".$_SESSION['id']."'"; $res = mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($res) != 1) { session_destroy(); echo "<script language=\"Javascript\" type=\"text/javascript\">document.location.href='login.php'</script>"; } else { $row = mysql_fetch_assoc($res); $title = protect($_POST['title']); if(!$title) { echo "<script language=\"Javascript\" type=\"text/javascript\"> alert(\"You must choose a title for your music!\") document.location.href='editmusic.php'</script>"; } $target = $row['username']; if(!is_dir($target)) @mkdir($target); $target = $target . '/music'; if(!is_dir($target)) @mkdir($target); $target = $target."/".basename($_FILES['music']['name']) ; $size = $_FILES['music']['size']; $music = $_FILES['music']['name']; $type = $_FILES['music']['type']; $max_file_size=10485760; if($size > $max_file_size){ echo "<script language=\"Javascript\" type=\"text/javascript\"> alert(\"You may not upload files over 10mb\") document.location.href='editmusic.php'</script>"; } else { if($type == "audio/mpeg" || $type == "audio/x-wav" || $type == "audio/x-ms-wma" || $type == "audio/mp3" || $type == "audio/mpeg3") { $sql2= "INSERT INTO `user_music` (`profile_id`,`title`,`size`,`type`,`reference`) VALUES ('".$_SESSION['id']."','$title','$size','$type','$music'); "; move_uploaded_file($_FILES['music']['tmp_name'], $target); $res2 = mysql_query($sql2) or die(mysql_error()); echo "<script language=\"Javascript\" type=\"text/javascript\"> alert(\"Your Music has been uploaded\") document.location.href='editmusic.php'</script>"; } else { echo "<script language=\"Javascript\" type=\"text/javascript\"> alert(\"Wrong file format. Please upload only .mp3, .wav or .wma files\") document.location.href='editmusic.php'</script>"; } } } } else echo "<script language=\"Javascript\" type=\"text/javascript\">document.location.href='login.php'</script>"; ?>
-
-
Short tags are bad practice for a couple of reasons. Serious application developers recommend not using short tags, which was good enough for me, they know what they are doing.
<?php include("../include/session.php"); if(!$session->isAdmin()){ header("Location: ../index.php"); } else{ ?> <title>MyVee! User Online</title> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <link href="../Style4.css" rel="stylesheet" type="text/css"> <div id="wrapper"> <div id="Layer4"> <div style="color:WHITE;"> <script type="text/javascript"> var d=new Date() var weekday=new Array("Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday") var monthname=new Array("Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec") document.write(weekday[d.getDay()] + " ") document.write(d.getDate() + ". ") document.write(monthname[d.getMonth()] + " ") document.write(d.getFullYear()) </script> </div></div> <div id="Layer2"></div> <div id="Layer1"></div> <div id="Layer3"> <p>[ <a href="http://www.myvee.co.uk">Back</a> ]<br> <p> <?php include("../include/view_active_admin.php"); ?> </html> </div> </div> <?php } ?>
If you try that and you are still getting the error, the problem is in one of your include files.
-
oh, I see what you are talking about. Well, there isn't a good way of doing this without using JavaScript.
Your going to need to grab the input values from your main form and place em in some hidden fields in the add form. One hidden field foreach input in the other form. Then, when they submit the add from, the values of the other from will be in the query string where then you can use em like $_GET['temp_name'] or something like that.
-
you must call session_start(); before anything with the sessions is done and before any output has been made. Pretty much, add this to the top of the page:
<?php session_start(); ?>
-
the $_SESSION array is pulled from a temporary file on the server in which can only be modified by applications on the server. If someone can use an external program to modify the sessions, then you have larger issues. If your on a shared host, I'm not 100% sure that the sessions are safe from someone on the same server grabbing em.
-
use the value attribute in the inputs and save the value in a session
echo 'Name ' . $i . ' : <input type="text" name="name' . $i . '" value="'.$_SESSION['name'].'" /><br />';
-
Since the form submits the user's name, you're going to need to query the table ibf_members for their name.
If the query finds results, use their mgroup as the variable to check it's != 3
Follow?
-
echo'<P>JOB ID:</P>' . $data['job_id']; echo'<P> JOB NAME:</P>' . $data['job_name'];
You had a semi-colon after the echo statement, instead use a period for concatenation and it should work for you.
This is true of all of them... you need to combine the strings using a dot, not a semicolen. But you will need to end those lines with a semi colen. Use the example from cobalt-rose
-
line 60, its fubar
I think you intended
$query = $db->execute("select `id`, `username`, `level`, `money` from `players` order by `level` desc limit 50");
but you are still missing the last_active field in your list.... you need:
$query = $db->execute("select `id`, `username`, `level`, `money`, `last_active` from `players` order by `level` desc limit 50");
-
top of the page add
error_reporting(E_ALL);
-
please use the code tags around your php or even html snipits, you will likely get more help
[ code ]Like this but without the spaces in the tags[ /code ]
would be
Like this but without the spaces in the tags
-
go ahead and get rid of this
$query = "SELECT * FROM players"; $result = mysql_query($query) OR die(mysql_error()); while ($row = mysql_fetch_assoc($result)) { $lastactive = $row['last_active']; }
change the other query to:
"select `id`, `username`, `level`, `money`, `last_active` from `players` order by `level` desc limit 50"
(Added last_active to field list)
See if that works
-
were you doing it inside the loop or is the code you posted literally what you have?
-
Okay, don't group or use sum, my mistake. Order them by dealer (or unique id).
In the loop, only go to the next row and make the first column the dealer if the dealer id or whatever you are using to identify them changed.
-
use count() on the array, if it returns < 1 then there are no adds for the section. walla
-
Try:
mysql_query("INSERT INTO `customers` VALUES ('$company', '$address', '$city', '$state', '$email', '$catagory', '$phone', '$pic')") or die(mysql_error());
More than likely it is a problem with your INSERT statement.
Also, remember to use mysql_real_escape_string() on variables being used in mysql statements other wise you are susceptible to getting a sql injection
-
if you use the code tags around your php or even html snipits, you will likely get more help
[ code ]Like this but without the spaces in the tags[ /code ]
would be
Like this but without the spaces in the tags
In what way did it not work? Did it give you an error or did you simply not get the results you expected?
-
use the one I suggested
lol, by just reading it I don't see whats wrong with it, but I'm not the greatest at regexp
-
is the field called 'id'? By using * in the select statement, you've grabbed all of the fields and you should be able to use the 'id' field with $row['id']
-
I don't see the problem, but this topic should be in the CSS board...
Export to program help
in PHP Coding Help
Posted
In what way does it not work? Do you not get data or does the application find the file invalid or something?