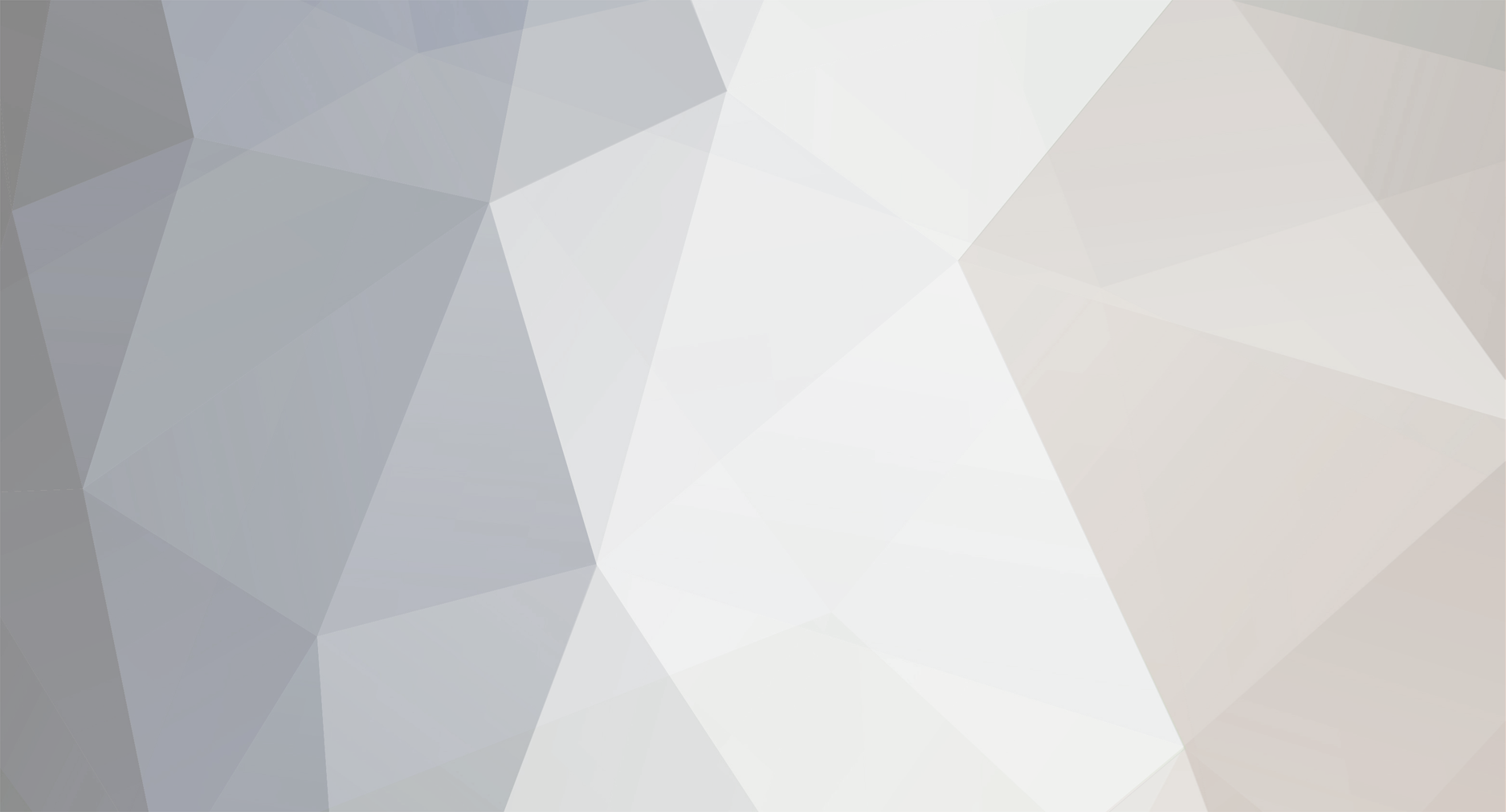
GKWelding
Members-
Posts
268 -
Joined
-
Last visited
Everything posted by GKWelding
-
Weird error: "Unsupported operand types" when I run this script ...
GKWelding replied to MoMoMajor's topic in PHP Coding Help
I think he just copied my code directly rather than integrating it into his own script and I hadn't included the echo command which is why he didn't see anything. -
Weird error: "Unsupported operand types" when I run this script ...
GKWelding replied to MoMoMajor's topic in PHP Coding Help
Well that means it's probably fixed. You just need to add in... echo "views = ". $_SESSION['views']; To view the session views variable. Your final script should look like... <?php session_start(); if (isset($_SESSION['views'])) { if (!is_numeric($_SESSION['views'])) { echo "CRAP!"; } ++$_SESSION['views']; }else{ $_SESSION['views'] = 1; } echo "views = ". $_SESSION['views']; ?> -
Weird error: "Unsupported operand types" when I run this script ...
GKWelding replied to MoMoMajor's topic in PHP Coding Help
P.S - I've seen this error before by the way, what version of PHP are you running? Echoing phpinfo() will tell you this. -
Weird error: "Unsupported operand types" when I run this script ...
GKWelding replied to MoMoMajor's topic in PHP Coding Help
Try this instead and let me know... if (isset($_SESSION['views'])) { if (!is_numeric($_SESSION['views'])) { echo "CRAP!"; } ++$_SESSION['views']; }else{ $_SESSION['views'] = 1; } -
PHP form - from 1 page to 2 pages with a catch
GKWelding replied to gilsontech's topic in PHP Coding Help
Please tell me if I am misunderstanding but what you are looking to do is populate the contact form on the second page with the same information that was submitted on the first page? This is simple if you're calling the second page as the action of the first page, even if you're not it would be easy to change this then submit both pages together. If the action of the first page is the second page then all you need to do is this... <INPUT NAME="fname" TYPE="text" VALUE="<? $_POST['fname']; ?>" > The $_POST['fname'] picks up the submitted value from the last form and populates it in the second. -
Which language is best for Social Networking website
GKWelding replied to sureshp's topic in Applications
I'm currently freelancing for a company that's working on a rather large networking site for the marketing industry. The initial brief was that the site should be scaleable to 5 million users. This is being coded in PHP. As somebody said above, the code is as fast as you make it. Also, about £50,000 worth of server equiptment and communication servers helps... And I agree, if you're a 1 man band and you're coding this on your own, any more than a few thousand users is a bit of a pipe dream. -
Zend is pretty crackable yes, however, if you're looking to secure your source code for resale I'd use something like ioncube instead, as far as I know this hasn't been cracked yet. And yes, if you're worried about people just browsing your php source code from their web browser don't be. It's not possible. They can see the HTML output by the PHP code but not the underlying PHP code, the server just wont allow it.
-
For others who may also want to know the answer to this... What you do is query the 'readers' table for all the articles read by a certain user_id, populating an array with the article_id of the entries they've READ, you then query the 'entries' table for entries that are NOT EQUAL to the article_id's you have in your array from the first query. Simple.
-
[SOLVED] Getting an error when script is rendered
GKWelding replied to ublapach's topic in PHP Coding Help
line 513 is: <option value="IN">Indiana</option> can't see any $ here... Although on line 491 you have this code: <input type="text" name="txtCity" style="width:300px;" value="<? echo $_POST[txtCity] ?>"> Where you're missing a ; -
In the code below, what does the variable $added get populated with? elseif($_POST["action"]=="approve") { $id = $_POST['id']; $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); $sql = "INSERT INTO $db_table (added, password, name, email, site_name, url, description) VALUES ('$added', '$password', '$name', '$email', '$site_name', '$url', '$description')"; $result = mysql_query($sql); $sql = "SELECT * FROM $db_table ORDER BY id DESC LIMIT 1"; $result = mysql_query ($sql); while ($row = mysql_fetch_array($result)) { $id = $row['id']; $to = $row['email']; $subject = "$ring_name Approval"; $message = "Your site has been approved in the $ring_name webring! Thanks for joining!\n\n$admin_name\n$index_php"; if($_POST['password'] == $admin_password) { mysql_query("UPDATE $db_table SET queue='1' WHERE id=$id"); print "<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "Thanks for your approval! We're taking you back to the main page!"; mail($to,$subject,$message,"From: $admin_email"); } else { echo "Wrong password."; } } } To solve this, when adding the date to the database the first time round use this: $added=time(); Then when querying from the database, to get the date in the format you want use this: while ($row = mysql_fetch_array($result)) { $added = date("m-d-y",$row["added"]); echo '<a href="'.$row["url"].'" target="_blank">'.$row["site_name"].'</a> id: '.$row["id"].'<br /> submitted on '.$added.'<br /><br />'; }
-
Spotted it! You have spaces in the filename of your text document, it's looking for a folder called 'new' in your uploads dir. Replace the spaces with underscores.
-
I can't see any reference to a date being added to your db in the code above. However, strtotime() should help solve that error.
-
Check your chmod setting on the folder to make sure it's writable.
-
Firstly, the $id request should be placed as shown below, not outside the while function... elseif($_POST["action"]=="approve") { $id = $_POST['id']; $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); $sql = "INSERT INTO $db_table (added, password, name, email, site_name, url, description) VALUES ('$added', '$password', '$name', '$email', '$site_name', '$url', '$description')"; $result = mysql_query($sql); $sql = "SELECT * FROM $db_table ORDER BY id DESC LIMIT 1"; $result = mysql_query ($sql); while ($row = mysql_fetch_array($result)) { $id = $row['id']; $to = $row['email']; $subject = "$ring_name Approval"; $message = "Your site has been approved in the $ring_name webring! Thanks for joining!\n\n$admin_name\n$index_php"; if($_POST['password'] == $admin_password) { mysql_query("UPDATE $db_table SET queue='1' WHERE id=$id"); print "<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "Thanks for your approval! We're taking you back to the main page!"; mail($to,$subject,$message,"From: $admin_email"); } else { echo "Wrong password."; } } } That was probably my poor explanation rather than your understanding. See if that helps with anything. Get back to me if it doesn't.
-
After you have queried the database for the information you need to add that code to populate the $id variable with the value from the id column in the databse. So put it after the mysql_query() function.
-
Removing slashes from mysql_real_escape_string
GKWelding replied to Canman2005's topic in PHP Coding Help
This is because you have magic quotes enabled on your server I think. Use this instead: <?PHP // Fix a $_POST variable called variable for MySQL $variable = $_POST['variable']; if (get_magic_quotes_gpc()) { // If magic quotes is enabled - turn the string back into an unsafe string $variable = stripslashes($variable); } // Now convert the unsafe string into a MySQL safe string $variable= mysql_real_escape_string($variable); // $variable should now be safe to insert into a query ?> -
Well, once you have that done, to populate your $id variable you should just do this: $sql = "INSERT INTO $db_table (added, password, name, email, site_name, url, description) VALUES ('$added', '$password', '$name', '$email', '$site_name', '$url', '$description')"; $result = mysql_query($sql); $sql = "SELECT * FROM $db_table ORDER BY id DESC LIMIT 1"; $result = mysql_query ($sql); $id = $result['id']; //replace id with whatever the name of your id column is
-
Basically the code isn't finding the entry in the database. Say you have and ID column in your table with values of 1, 2 and 3. If you search for value 4 then it will give you this error as mySQL hasn't found a record with ID 4 and as such there is no array to fetch.
-
This code should give you a horizontal line $x1=50; $x2=150; $y1=100; $y2=100; $im = @ImageCreate (200, 200) or die ("Cannot Initialize new GD image stream"); $background_color = ImageColorAllocate ($im, 224, 234, 234); $text_color = ImageColorAllocate ($im, 233, 14, 91); imageline ($im,$x1,$y1,$x2,$y2,$text_color); ImagePng ($im); This code should give you a vertical line $x1=100; $x2=100; $y1=50; $y2=150; $im = @ImageCreate (200, 200) or die ("Cannot Initialize new GD image stream"); $background_color = ImageColorAllocate ($im, 224, 234, 234); $text_color = ImageColorAllocate ($im, 233, 14, 91); imageline ($im,$x1,$y1,$x2,$y2,$text_color); ImagePng ($im);
-
Exactly what it says, you have a syntax error replace } else ( with } else {
-
The error is occuring here $id = $_POST['id']; $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result)) Check the the form is sending the ID correctly as a post and not a get and that it's also called id and not ID or iD or Id.
-
When you added the session_register to the top of the page, you say it didn't work, instead, add session_start(); to the top of the page leaving the rest of the code as is...
-
Try using this instead. You'd gotten a little lost with your if's and elseif's <? require("config.php"); if($_POST["action"]=="submit"){ require_once('recaptchalib.php'); $privatekey = "##################"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { die ("The reCAPTCHA wasn’t entered correctly. Go back and try it again." . "(reCAPTCHA said: " . $resp->error . ")"); } $added=$_POST['added']; $password=$_POST['password']; $name=$_POST['name']; $email=$_POST['email']; $site_name=$_POST['site_name']; $url=$_POST['url']; $description=$_POST['description']; $sql = "INSERT INTO $db_table (added, password, name, email, site_name, url, description) VALUES ('$added', '$password', '$name', '$email', '$site_name', '$url', '$description')"; $result = mysql_query($sql); $sql = "SELECT * FROM $db_table ORDER BY id DESC LIMIT 1"; $result = mysql_query ($sql); while ($row = mysql_fetch_array($result)) { $id = $row['id']; $password = $_POST['password']; $to = $_POST['email']; $from = "From: $admin_email"; $subject = "$ring_name Submission"; $message = "Thanks for joining the $ring_name webring! Here is your login info, keep this in a safe place!\n\nSite ID: $id\nPassword: $password\n\nDon't forget to put up the ring code on your site as soon as possible! You will not be added to the ring until I find the ring code on your site.\n\nHere's the code:\n\n<a href='http://mirrormatter.imokon.com/index.php?id=1&action=prev'><</a> <a href='http://mirrormatter.imokon.com/index.php?action=rand'>?</a> <a href='http://mirrormatter.imokon.com/index.php?action=home'>Mirror|Matter</a> <a href='http://mirrormatter.imokon.com/index.php?action=list'>#</a> <a href='http://mirrormatter.imokon.com/index.php?id=1&action=next'>></a>\n\nThanks!\n$admin_name\n$ring_url"; $name = $_POST['name']; $url = $_POST['url']; $admin_message = "$name has joined $ring_name! To check this site for your ring code, go to:\n$url\n\nTo approve this new site, go to:\n$ring_url/process.php?id=$id&action=approve\n\nAlternatively, to remove this site, go to:\n$ring_url/process.php?id=$id&action=remove"; if(mail($to,$subject,$message,$from)) { mail($admin_email,$subject,$admin_message,"From: $to"); print"<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "Thanks for your submission! We're taking you back to the main page!"; } else { echo "There was a problem with your submission. Please check that you filled in the form correctly."; } } } elseif($_POST["action"]=="modify") { require_once('recaptchalib.php'); $privatekey = "##################"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { die ("The reCAPTCHA wasn’t entered correctly. Go back and try it again." . "(reCAPTCHA said: " . $resp->error . ")"); } $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result)) { $password1 = $row['password']; $password2 = $_POST['password']; if($password1 == $password2) { mysql_query("UPDATE $db_table SET name='$name', email='$email', site_name='$site_name', url='$url', description='$description' WHERE id='$id'"); print"<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "Thanks for your modification! We're taking you back to the main page!"; } else { echo "There was a problem with your submission. Please check that you filled in the form correctly."; } } } elseif($_POST["action"]=="remove") { require_once('recaptchalib.php'); $privatekey = "##################"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { die ("The reCAPTCHA wasn’t entered correctly. Go back and try it again." . "(reCAPTCHA said: " . $resp->error . ")"); } $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result)) { $password1 = $row['password']; $password2 = $_POST['password']; $to = $row['email']; $url = $row['url']; if($password1 == $password2) { mysql_query("DELETE FROM $db_table WHERE id=$id"); $from = "From: $admin_email"; $subject = "Site Deleted From $ring_name"; $message = "Your site has been deleted from $ring_name upon your request. Sorry you decided to go!\n\n$admin_name\n$index_php"; $admin_message = "The site located at $url has been deleted upon the owner's request."; if(mail($to,$subject,$message,$from)) { mail($admin_email,$subject,$admin_message,"From: $to"); print"<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "You've been deleted from $ring_name. Sorry to see you go!"; } else { echo "There was a problem with your submission. Please check that you filled in the form correctly."; } } else { echo "There was a problem with your submission. Please check that you filled in the form correctly."; } } } elseif($_POST["action"]=="approve") { $id = $_POST['id']; $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result)) { $to = $row['email']; $subject = "$ring_name Approval"; $message = "Your site has been approved in the $ring_name webring! Thanks for joining!\n\n$admin_name\n$index_php"; if($_POST['password'] == $admin_password) { mysql_query("UPDATE $db_table SET queue='1' WHERE id=$id"); print "<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "Thanks for your approval! We're taking you back to the main page!"; mail($to,$subject,$message,"From: $admin_email"); } else { echo "Wrong password."; } } } elseif($_POST["action"]=="delete") { $id = $_POST['id']; $sql = "SELECT * FROM $db_table WHERE id=$id"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result)) { $to = $row['email']; $site = $row['site_name']; $subject = "$ring_name Deletion"; $message = "Your site has been deleted from the $ring_name webring! If you have any questions about why your site was deleted, respond to this email.\n\n$admin_name\n$index_php"; if($_POST['password'] == $admin_password) { mysql_query("DELETE FROM $db_table WHERE id=$id"); print "<meta HTTP-EQUIV=\"REFRESH\" CONTENT=\"3; URL=$index_php\">"; echo "$site has been deleted from the webring!"; mail($to,$subject,$message,"From: $admin_email"); } else { echo "Wrong password."; } } } elseif($_GET["action"]=="approve") { echo "Please enter your admin password:<br><br><form method=\"post\" action=\"$PHP_SELF\"><input type=\"hidden\" name=\"id\" value=\"$id\"><input type=\"password\" name=\"password\"><input type=\"submit\" name=\"action\" value=\"approve\"></form>"; } elseif($_GET["action"]=="remove") { echo "Please enter your admin password:<br><br><form method=\"post\" action=\"$PHP_SELF\"><input type=\"hidden\" name=\"id\" value=\"$id\"><input type=\"password\" name=\"password\"><input type=\"submit\" name=\"action\" value=\"delete\"></form>"; } else { echo "No variable passed."; } ?>
-
Although I'm not familiar with PDO myself, I know for a fact that prepared queries DO NOt protect against SQL injection. I promise you that. Even if it does you should still sanitize all data JUST IN CASE... Adding mysql_real_escape_string() or addslashes(trim()) (<--- although I would use real_escape_string over this) isn't a big deal.
-
Set your set_time_limit() to something like 45 seconds. Have a HTML meta refresh set up for 30 seconds on your page that redirects to whatever the next page is you want to run a script from.