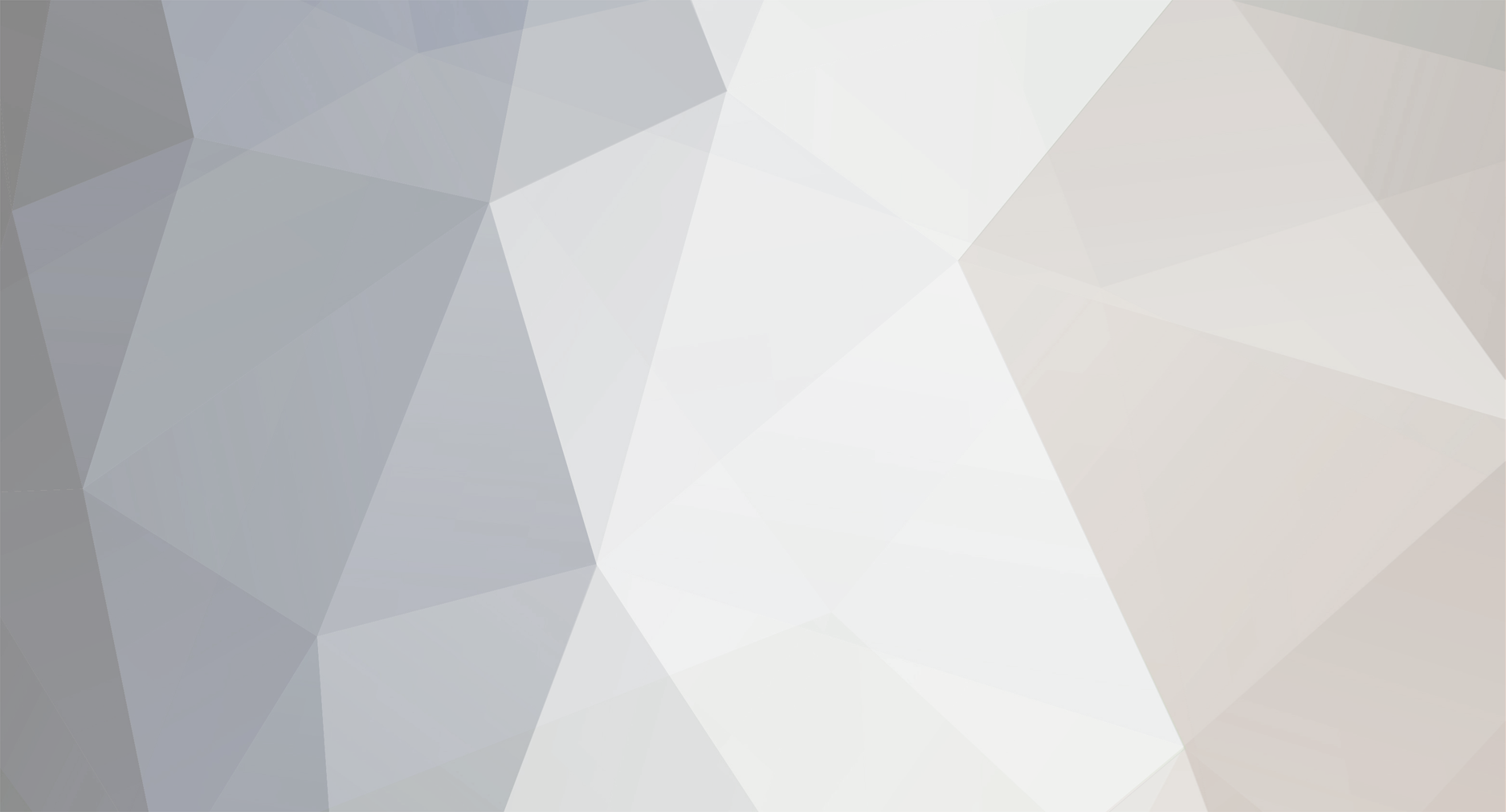
GKWelding
Members-
Posts
268 -
Joined
-
Last visited
Everything posted by GKWelding
-
jEditable will be of no use to you, it allows editing of data when you click it, not en-masse. You will need to write a custom function to do this. I am very proficient with jQuery and if you're really stuck on this then PM me and we can talk about a solution. You will need to select all of the elements you have displayed, use jQuery to replace all of these elements with forms with the correct data and action etc... or alternatively you can replace everything with 1 big form, I think this would be the way to go, but would need to see your layout and data to confirm. This is essentially what jEditable does but you'd be doing ti on a larger scale. The form action would then be a php script which would do the processing of the data. Example: <html> <head></head> <body> <table> <tr><td id="uniqueRowId1" class="editable">Data 1</td></tr> <tr><td id="uniqueRowId2" class="editable">Data 2</td></tr> </table> <script type="text/javascript"> jQuery('table').before('<form action="save.php" method="post">'); jQuery('table').after('<input name="submit" type="submit" value="Save" /> </form>'); jQuery('.editable').each(function(){ var v = jQuery(this).html(); var i = jQuery(this).attr('id'); jQuery(this).replaceWith('<input type="text" name="'+i+'" value="'+v+'" />'); }); </script> </body> </html> example of save.php <?php if(isset($_POST['submit']){ foreach($_POST as $key=>$val){ if($key != 'submit'){ $sql = 'UPDATE {TABLE} SET data = '.$val.' WHERE id = '.$key; mysql_query($sql); } } } ?> please keep in mind that the sql query is not optimised, it could all be done as 1 string rather than a foreach, and none of the values are escaped so somebody could easily SQL Inject this code. Hope this helps though.
-
This is a login class I wrote a long time ago, hope it helps you: class login{ var $response; function set_user_login($username, $password) { $username=mysql_real_escape_string($username); $password=mysql_real_escape_string($password); $query = "select salt from user where username='$username' limit 1"; $result = mysql_query($query); if (mysql_num_rows($result) > 0) { $user = mysql_fetch_array($result); $encrypted_pass = md5(md5($password).$user['salt']); $query = "select ID, username from user where username='$username' and password='$encrypted_pass'"; $result = mysql_query($query); if (mysql_num_rows($result) > 0) { $user = mysql_fetch_array($result); $userid = $user['ID']; $encrypted_id = md5($user['userid']); $encrypted_name = md5($user['username']); $_SESSION['userid'] = $userid; $_SESSION['username'] = $username; $_SESSION['encrypted_id'] = $encrypted_id; $_SESSION['encrypted_name'] = $encrypted_name; $this->response = 'Correct'; } else { $this->response = 'Invalid Password'; } } else { $this->response = 'Invalid Username'; } } function get_user_login() { return $this->response; } function set_user_logout() { session_unset (); session_destroy (); echo"You have been successfully logged out, you will be redirected shortly."; echo"<META HTTP-EQUIV=\"Refresh\" Content=\"2;URL=index.php\">\n"; } }
-
Bricktop's right, fsockopen will return false if that port isn't open, that's what you need. Don't forget to close afterwards though!
-
I think, basically, the problem is the filters aren't working as the LIKE isn't being formatted properly and all of the entries are being returned, if I read the original post correctly.
-
Try creating a simple session table in MySQL, in this table insert the users session ID and the users user ID (I assume you have an auto-incrememnting id column in the users table). Then, in users.php do a call to your database and return the users userId using their current session ID. As the admin user ID will always be a certain value, probably 1, then you will be able to filter by this in users.php. If you want a working example of this then PM me as this is fairly complicated to achieve securely and successfully.
-
Can you post more from the start of your code as this will assist me in helping you. Anything before #filter settings will help.
-
problem solved, before evey data send i do a socket_read. If the socket_read returns false then I know the sockets down and I reconnect. If the socket returns data then the socket is up, if the socket returns a blank string I also know the socket is up but that the buffer's empty. Either way, socket_read and $socket === false will tell me if he socket's down. Cheers for the help anyway guys.
-
Thanks for your replies, however, I think I may need to explain a little more here. I can connect fine to the socket, however, randomly the socket drops the connection when it shouldn't. Now all I want to do is catch this connection dropping and tell the script to reconnect again. The socket server and is just a tcp listener written by my company using ruby. When the open socket gets randomly disconnected it sends out a message sayign so, now flash can interpret this message and reconnects the socket automatically. However, PHP doesn't seem to have a function for this, is that correct?
-
Ok, I have a custom socket handler for a project I am working on. Now this socket handler has been tested thoroughly and can keep the connection open, and stable for days. However, randomly, it loses the socket connection every now and again for no apparent reason. We've spent a lot of time and monet trying to track the cause of this down and we can't. So now what we're looking to do is just detect when the socket disconnects and reconnect it. Now our flash handler has a function that does this for us, it detects the disconnect and reconnects automatically. However, from the PHP docs I can't seem to find a similar function in PHP. Is there one? Is it possible to automatically reconnect a disconnected socket in PHP? Just a note the socket handler is auto run every 10 seconds or so where it queries our database and z00m server for any changes to pass between the two.
-
I thought that too, so I put a further if statement around $message = "You must enter and entry title and entry text."; that said if $_POST isn't set then do not populate with this text, but alas that didn't work... Still got the same error.
-
Simple if statement causing me problems. Been looking at this too long to figure it out now so I'm not even going to try anymore as it's all just starting to merge into one which means I'm just wasting my time. Ok, so I have a form linked to this script. You put in the title, and the text. hit submit and it posts info to the db for display later on, simple blog. However, hitting post even when you have text in the title and body causes the loop to spit out 'You must enter and entry title and entry text.' however it also posts the data to the database correctly as it should do. So basically it's getting the message from the first part of the if loop whilst doing the action of the else part of the if loop. Any suggestions? $entryTitle = $_POST['entryTitle']; $entryText = $_POST['entryText']; $blogId = $_POST['blogId']; $userId = um()->getCurrentUser()->getUserId(); $myblog = blogconc()->getOneBlog($userId); $blogOwner = $myblog->getBlogOwner(); if($blogOwner != $userId){ $message = "You do not have permission to add to this blog"; } else { if (trim($entryTitle) == "" && trim($entryText) == ""){ $message = "You must enter and entry title and entry text."; } else { $blogcheck = blogconc()->getOneBlog($userId); $blogcheckid = $blogcheck->getBlogId(); if ($blogcheckid != ""){ $entryBlogId = $blogcheckid; $query = blogconc()->addEntry($entryBlogId, $entryTitle, $entryText); //if ($query){ $message = "Entry Added Successfully!"; //} } else { $message = "You are trying to post to a blog that doesn't exist."; } } }
-
No problem mate. Glad it works for you. Enjoy.
-
Try changing your img inks to the folowing <a href="<?php echo $ad['url']['1']; ?>"><img src="<?php echo $ad['ad']['1']; ?>"></img></a><br /> I'l take a look why certain arrays aren't populating too but try that for now.
-
also, regarding your html php problem, go here... http://www.desilva.biz/php/phpinhtml.html
-
ok, replace ad_function.php with the following. <?php function ads(){ mysql_connect("localhost", "****", "****"); @mysql_select_db("****") or die ("unable to connect"); $limit = 8; //number of ad spaces on the page $large = 2; //number of full sized add spaces on your page $small = $limit - $large; $query_large = mysql_query("SELECT * FROM ads WHERE large = \"YES\" ORDER BY Rand() LIMIT $large"); $i=0; while($sql=mysql_fetch_array($query_large)){ if($i < $large){ $ad["ad"][$i]=$sql["full_ad"]; //URL for full sized ad banner $ad["url"][$i]=$sql["add_url"]; //URL for full sized ad banner to redirect to website $i++; } } $query_small = mysql_query("SELECT * FROM ads WHERE large = \"NO\" ORDER BY Rand() LIMIT $small"); $i=0; while($sql=mysql_fetch_array($query_small)){ if($i < $small){ $ad["ad"][$i+$large+1]=$sql["small_ad"]; //URL for small sized banner $ad["url"][$i+$large+1]=$sql["add_url"]; //URL for small sized ad banner to redirect to website $i++; } } return $ad; } ?>
-
do a print_r on the array to check it's being populated correctly and that all the associations are what you are assuming them to be. Post the results of the print_r here. Note, your print_r call needs to be on the HTML page you're trying to display the images on...
-
Technically, MD5 is a hash not encryption. If you encrypted the password using an encryption alogrythm with an encryption key, as long as you had the encryption key stored somewhere safe where your scripts could access it then technically you could decrypt the password. However, the far simpler method is just to MD5 it so it isn't in plain text and have the user reset the password as thorpe suggests.
-
Correct. No probs.
-
What I don't think you'll find in that list is "%0D" and "%0A" are carriage return and line feed.
-
Hex codes.... http://www.ascii.cl/htmlcodes.htm
-
I think the best way to sort that would be to do the following, that way you don't need to change anything else. Add a new column to the database as you said. Then change ad_functions.php to this... <?php function ads(){ $dbconnect; //your code to connect to your database $limit = 6; //number of ad spaces on the page $large = 3; //number of full sized add spaces on your page $small = $limit - $large; $query_large = mysql_query("SELECT * FROM ads WHERE large = \"YES\" ORDER BY Rand() LIMIT $large"); while($sql=mysql_fetch_array($query_large)){ $i=0; if($i < $large){ $ad["ad"]=$sql["full_ad"]; //URL for full sized ad banner $ad["url"]=$sql["add_url"]; //URL for full sized ad banner to redirect to website $i++; } } $query_small = mysql_query("SELECT * FROM ads WHERE large = \"NO\" ORDER BY Rand() LIMIT $small"); while($sql=mysql_fetch_array($query_small)){ $i=0; if($i < $small){ $ad["ad"]=$sql["small_ad"]; //URL for small sized banner $ad["url"]=$sql["add_url"]; //URL for small sized ad banner to redirect to website $i++; } } return $ad; } ?>
-
Just re-read your question. Yes it would be simple. you need to add another column to that DB. ad_url - text data put into this should just be the website like http://www.website.com so that when the <a href> is compiled it looks like a normal href... Change the ads() function to the following: <?php function ads(){ $dbconnect; //your code to connect to your database $limit = 6; //number of ad spaces on the page $large = 3; //number of full sized add spaces on your page $query = mysql_query("SELECT * FROM ads ORDER BY Rand() LIMIT $limit"); while($sql=mysql_fetch_array($query)){ $i=0; if($i < $large){ $ad["ad"]=$sql["full_ad"]; //URL for full sized or large ad banner $ad["url"]=$sql["ad_url"]; //URL for full sized or large ad banner to redirect to website $i++; }else{ $ad["ad"]=$sql["small_ad"]; //URL for small sized or cut down ad banner $ad["url"]=$sql["ad_url"]; //URL for full sized or large ad banner to redirect to website $i++; } } return $ad; } ?> Then in your HTML change your image tags to this... <a href="<?php $ad['url']['0'] ?>"><img src="<?php $ad['ad']['0'] ?>"></img></a><br />
-
Basically, what your DB needs to contain is the following. (column name - column type) ID - Auto Increment Int(11) BusinessName - varChar(128) full_ad - text small_ad - text As an example an entry in the database would be: 1, Amteus Secure Communications Ltd, images/amteus_large.jpg, images/amteus_small.jpg You would then have a folder in your main directory called images, in the images folder you would have 2 images called amteus_large.jpg and amteus_small.jpg. That way, when the php function is run, one of the array values returned would be "images/amteus_large.jpg" or "images/amteus_small.jpg". that way when the HTML file is parsed and displayed the image tags look like <img src="images/amteus_large.jpg"></img> just like regular HTML image tags. simple eh....
-
I also forgot to mention, after <?php require_once("ad_function.php"); ?> but before <img src="<?php $ad['0'] ?>"></img><br /> <img src="<?php $ad['1'] ?>"></img><br /> <img src="<?php $ad['2'] ?>"></img><br /> <img src="<?php $ad['3'] ?>"></img><br /> <img src="<?php $ad['4'] ?>"></img><br /> <img src="<?php $ad['5'] ?>"></img><br /> You need to put <?php $ad=ads(); ?>
-
I forgot to mention, you should have your ad images on your server with a database that has the paths and names of all the images, in large (column name full_ad) and small (column name small_ad) size, which is where the script gets the path of the image from to output to the HTML.