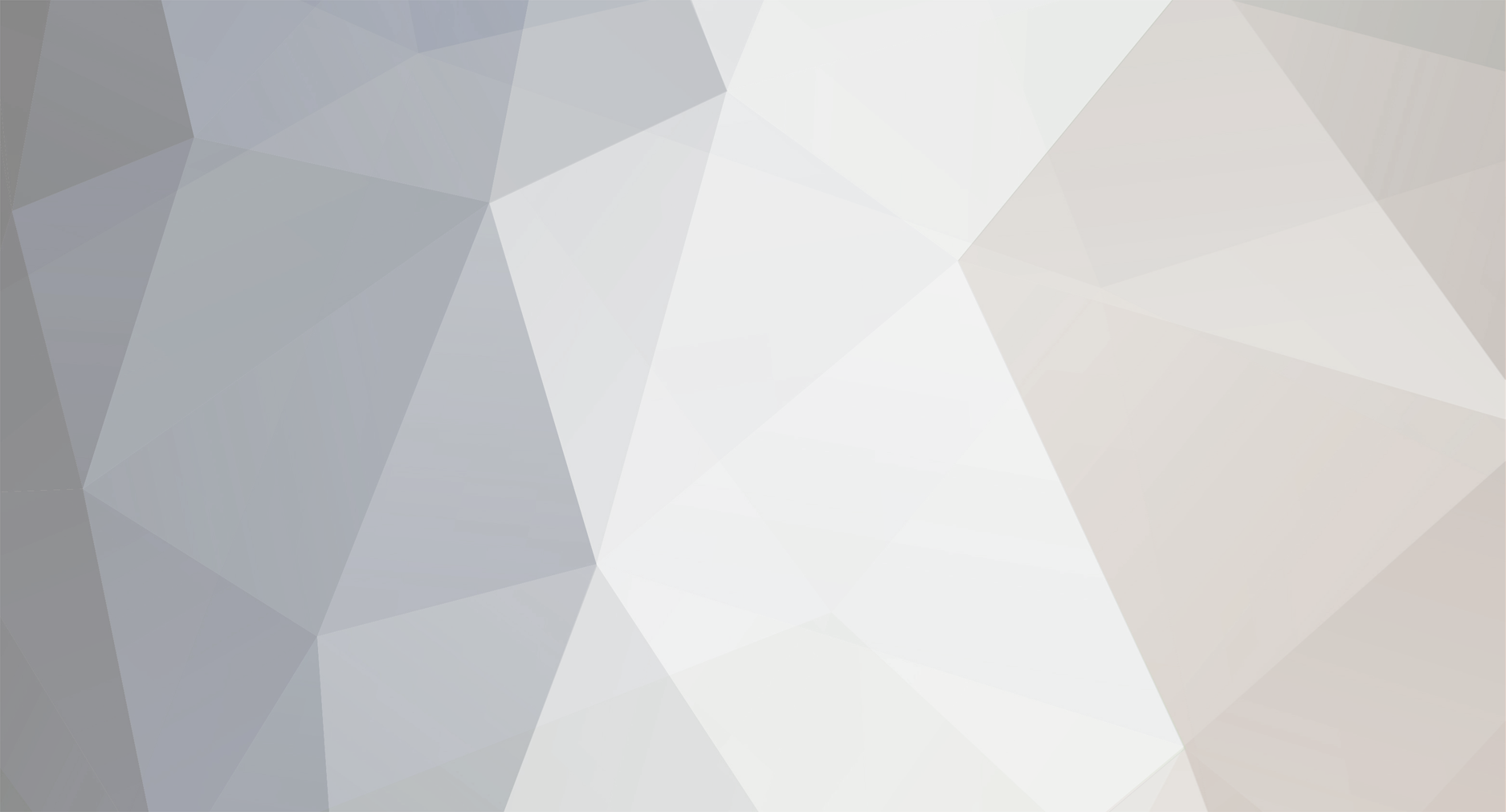
the182guy
Members-
Posts
611 -
Joined
-
Last visited
Everything posted by the182guy
-
I'd do the extension part like this: <?php function getExtension($filename) { $out = false; $pos = strrpos($filename, '.'); if($pos !== false && $pos > 0) { // filename has a . and the . is not the first char $out = substr($filename, $pos + 1); } return $out; } $file = 'test.jpg'; $allowed = array( 'jpg', 'png', 'gif', 'bmp' ); $ext = getExtension($file); if($ext !== false) { // it's a valid extension, does it match a known one? if(in_array(strtolower($ext), $allowed)) { // $ext is one of the allowed extensions echo 'allowed'; } else { // $ext is valid but is not in the allowed list echo 'valid but no allowed'; } } else { // extension is not valid echo 'not valid'; } ?>
-
Firefox 3.6, the same thing happens in Chrome but only on the first step. Fade is is very easy using jQuery: http://api.jquery.com/fadeIn/ Not sure how it would look with the images being a fair size, might need to preload them and use a loading gif.
-
echo "<a href=\"#\" onclick=\"javascript: window.open('barmizva.php?havecritiq=" . $exist . "', 'window_name', 'width = 50, height = 50'); return false;">link</a>"; You shouldn't really stick it all on the <a> tag like that, very difficult to read and debug.
-
Are you trying to do.... echo "<a href="#" onclick=\"javascript: window.open('barmizva.php?havecritiq=" . $exist . "', 'window_name', 'width = 50, height = 50'); return false;"\">link</a>";
-
Please don't create a Goodbye! popup when somebody closes your site, people will start to hate you!
-
It doesn't feel like AJAX, it just feels like a traditional page load because the browser appears to scroll to the top of the page on click through of the demo. What actually happens is the large image is destroyed and for a moment the page resizes itself and that causes the scroll up effect. To solve that put the demo in a containing div that has a height equal to the height of the big graphics. Also it would look good if you faded in the next image.
-
Just use the & sign echo "<a href=\"submit_docs.php?prop_id=".$prop_id."&test=" . $test . "\">Click </a>";
-
Use $_POST['color'] or $_POST['instrument'] to reference posted form inputs not $color or $instrument. Also when printing user inputted data onto a page you need to clean it to stop XSS attacks, use strip_tags() and htmlentites() to remove any HTML (most importably <script> tags).
-
It will work with an array for the params (inputs) aswell, doesn't have to be an object. the SoapClient will convert both arrays and objects into the XML Soap envelope. It's good practice to use a try/catch structure when working with webservices because the SoapClient will throw an exception in your code if there is any problem with the SoapServer, which of course may be out of your control.
-
You don't even need the Zend JSON implementation, all you need is json_encode() and json_decode()
-
You can inject cookies into chrome because it uses a simple SQLite database to maintain it's cookies, the problem would be is Chrome keeps the cookies in memory instead of reading the database on each page request (for speed), so you would have to restart Chrome before the new cookie is picked up. You could echo out the session ID that you need, and then using Opera as your browser, you can manually modify the session ID so change it to the one the php code has echoed. I believe Opera allows you to modify cookies in realtime.
-
Try adding this option to the other cURL options curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
-
You must be printing/echoing the $result variable somewhere?
-
Perfect example here: http://davidwalsh.name/execute-http-post-php-curl Just change the form fields to the ones in your working form (message, token).
-
Using a flat file instead of a RDMS because it's "easier" to modify the data is an invalid reason to use a flat file.
-
php CURL - multiple independent sessions - need help!
the182guy replied to gotts's topic in PHP Coding Help
Personally I would use something like this instead of cURL, but that would need modifying slightly I think. cURL needs a physical cookie file which is not really ideal. You'd be better of just storing the cookies as variables if possible - which it isn't with cURL. -
Just search for a cURL post example, very simple...
-
php CURL - multiple independent sessions - need help!
the182guy replied to gotts's topic in PHP Coding Help
It does make sense, cURL is using the same cookie for all users. You'll need to create a seperate cookie for each user, one way would be to name the cookie with the users user ID in the filename like cookie_[userID].txt -
Can't connect to mysql DB from PHP code on localhost
the182guy replied to MiRed's topic in PHP Coding Help
Well if your db_connect.php does not have the user/pass/host variables in it then of course you'll get the warnings because $DB_SERVER, $DB_USER, $DB_PWD are all undefined and will be treated as blank. -
I don't see the point in pulling the source code for a page, then printing it out so the page displays, then clicking through the links on the page. Why? Anyway about the link, you need to have a look at the source of the human_test() function to see what it's doing. Your web browser will execute the javascript. The reason you are logged out when clicking any links is because cURL has created the session and it has the session ID as a cookie, clicking a link in your browser will be using a seperate session and the browser won't be sending the authenticated session ID.
-
Sounds like you want to create a flat file 'database'. Like a CSV?
-
Can't connect to mysql DB from PHP code on localhost
the182guy replied to MiRed's topic in PHP Coding Help
You're not running the code in your first post, you are running some other code where mysql_connect() is using username 'user' and a blank password. Check you're including the correct dbconnect script. -
To do it script wide so that all your date() calls will be in the specified timezone use: date_default_timezone_set('EST');
-
No AJAX needed, just setup your system to store PHP sessions in the database, this will mean that you have a sessions tabe and there will be a field for the timestamp of the last request. All you need to do is run a query to pull out the number of sessions (rows) that have a timestamp in the last say 10 minutes. There is a way to increase the accuracy with AJAX, which is to (within your page) call one of your scripts every say 1 minute, which will update the session timestamp, then of course if a user closes the page, the AJAX call will no longer run and the timeout won't be updated, so you can assume the user is not online if the timeout is older than a minute. Personally I won't bother with the AJAX though, I would just look at the table and see who was updated in the last 10 minutes.
-
Don't use if(isset($_POST['submit'])) to test if a form has been submitted. Use: if($_SERVER['REQUEST_METHOD'] == 'POST') { // form is submitted } Using the isset way will mean that if the user presses the enter key to submit the form with internet explorer, your code will not pickup the fact that the form has been submitted.