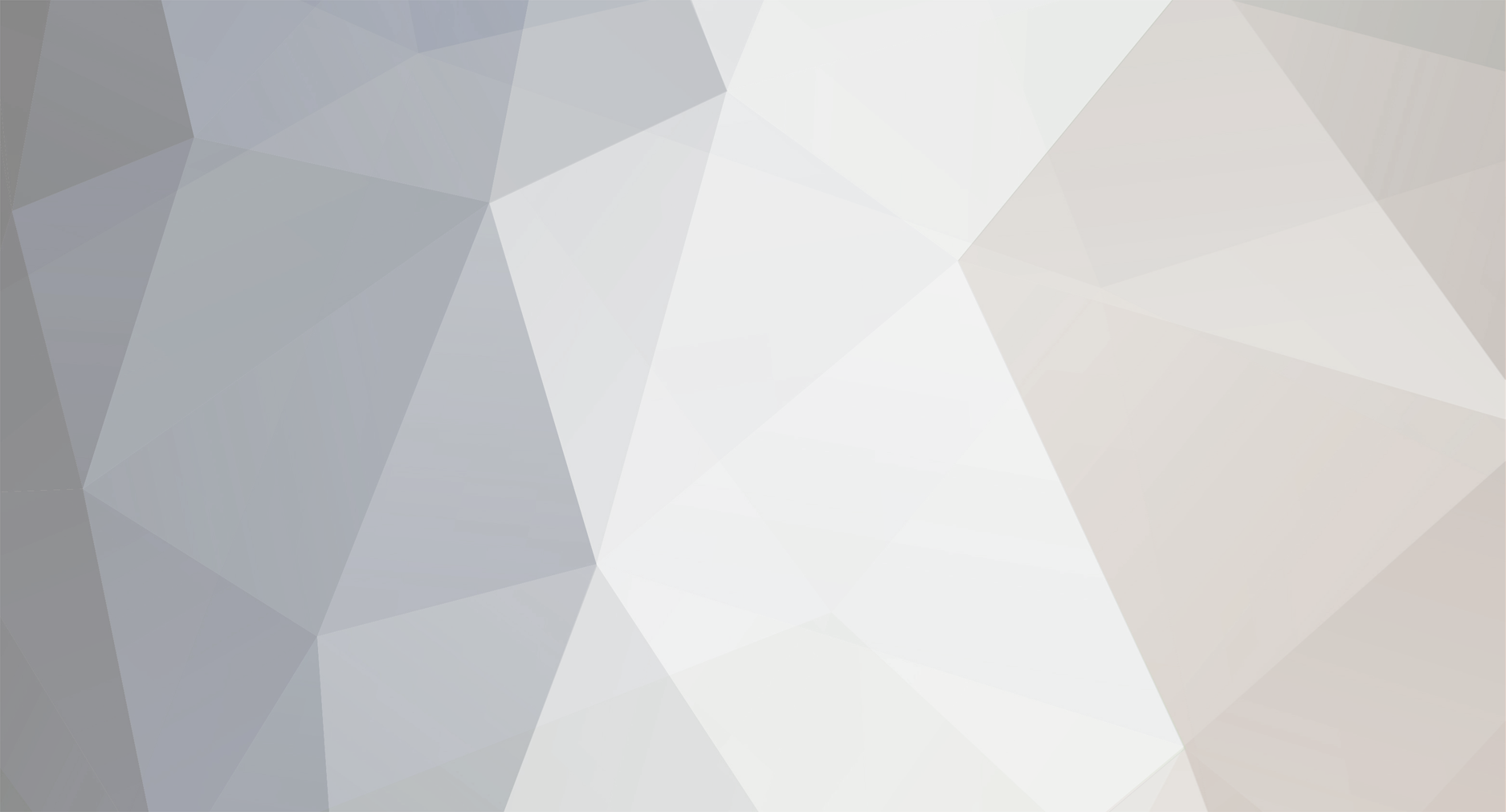
galvin
-
Posts
614 -
Joined
-
Last visited
Posts posted by galvin
-
-
Well, I am getting info from Amazon S3 and the variable I mentioned is the total number of images from a certain bucket. And the div that I sometimes want to hide contains the form to upload new images. Basically, if the user has uploaded a certain number of images (10), then I don't want the upload form to display (so that they can't upload anymore images).
The S3 code gets and displays the images (and also counts them as it's doing that).
I think the upload form needs to be above the S3 code (which is below) since there could be a lot of images listed out below it.
I was thinking maybe putting the upload form after the S3 code and then positioning it above the images using CSS, but that seems hacky, no?
Or could I run the S3 code before it and not display it (i.e. don't use echos until later in the code). This sounds right but not sure how I would store all the image code in a variable that is displayed later in the page.
//include the S3 class require_once('sdk-1.4.4/sdk.class.php'); // Instantiate the class $s3 = new AmazonS3(); $response = $s3->get_object_list('bucket', array('prefix' => $id.'/')); $o=0; foreach ($response as $file){ $furl = "http://mydomain.s3.amazonaws.com/".$file; //output a link to the file echo "<img src='$furl' width='50' />$furl"; ?> <form action="/s3processdelete.php" method="post" enctype="multipart/form-data" name="form2" id="form2"> <input type="hidden" name="file" value="<?php echo $file;?>"/> <input name="SubmitDelete" type="submit" class="delete" value="Delete This Image"> </form> <?php $o=$o+1; }
-
There has to be a simple solution for this problem that I am apparently too dense to figure out...
Say on a single page, I have code like this...
<div id="stuff"> //stuff </div>
and then further down on the page, I have code that runs and produces a variable ($i) that is a number. I then want to check to see if that number is 10 or higher, or not, (which I can handle doing
) and if it is 10 or higher, then I want to hide the "stuff" div that is further up on the page.
What is the best way to do this? It's like I need the code to run and then go back and add something to the "stuff" div to make it not show, if the variable is 10 or higher.
Any thoughts? This has to be easy so I"m feeling particularly newbie tonight :-\
if ($i >=10) { //the number is 10 or higher so let's somehow hide the "stuff" div that is further up on the page. but how? } else { // the number is less than 10 so it's cool to continue to display the "stuff" div }
-
I'll be damned, that worked. I guess this "solution" would be seen as hacky, but it will do for now
Thank you!
-
Ok, I tried file_get_contents() and it's properly recognizing that an image is OK, but if the image doesn't exist, it still gives this warning...
Warning: file_get_contents(http://mysite.s3.amazonaws.com/1/monkey.jpg) [<a href='function.file-get-contents'>function.file-get-contents</a>]: failed to open stream: HTTP request failed! HTTP/1.1 404 Not Found
I really just need a way to essentially say "Hey PHP, if you're going to kick a warning about this specific image URL, run this code instead and DON'T show the warning"
Obviously it's not that easy because Googling about this finds lots of different suggestions, and a lot of people saying the suggestions don't do what they need.
FYI, my new code is...
if (file_get_contents($image)) { //image URL is OK echo "<span class='image'><img src='".$image."' class='image' title = '".$image."' "; list($width,$height) = getimagesize($image); if ($width > $height) { echo "width=50 /></span>"; } elseif ($height > $width) { echo "height=50 /></span>"; } else { //height and width must be equal so just set width = 50, but could just as easily set height to 50 echo "width=50 /></span>"; } } else { //image URL must not be OK echo "<span class='image'><img src='/images/noimage.png' class='image' title = 'no image' /></span>"; }
So if the image doesn't work it does echo that last span (which I want I to do), but it still shows the warning. So I need to get rid of that warning. And I don't want to just turn off warnings
, I want to properly change the code so the warning doesn't happen regardless.
If anyone has any other suggestions, I am more than open to them
Thanks!
-
thanks jcbones, I'll give it a shot. There may be a few external site URLs that are sites not owned by me, but I am likely using Amazon S3 for the majority of my image storage
-
What is the best/proper way to run an image URL from an external site through a check to see if the image actually exists? I am trying to use file_exists() but it's not recognizing image URLs from external sites as existing, so there must be some other way to deal with external URLs. In other words, the image does exist but file_exists() says it doesn't.
Can anyone help?
-
I have the following rule at the end of my htaccess file and its job is to take any url in the format mydomain.com/something and redirect it to mydomain.com/play.php?url=something.
This works fine, but I have images that are not showing up because the src is like "images/monkey.jpg" and I believe this rule is messing those up (because if I remove this rule temporarily, the images work fine).
What is the best way to handle this? Is there some kind of rule I could put in place above this other rule that basically says "if anything starts with /images/, then don't try to rewrite it"? Or is there another way to go about this?
RewriteRule ^([^/\.]+)/?$ play.php?url=$1 [NC,L]
-
I found the code below which checks to make sure something the user entered into a text field is an actual URL. I am looking to confirm that something a user enters is an actual link to an IMAGE, so does anyone know how I could adjust this code below to do that?
Basically, this code below would accept http://monkeys.com AND http://monkeys.com/images/monkey.jpg.
I want to alter the code so that http://monkeys.com would NOT be accepted since it's not an image. Essentially, I want to make sure whatever the user enters ends with .jpg, .gif or .png.
Anyone know how to do this?
url": { "regex": /^(https?|ftp):\/\/(((([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(%[\da-f]{2})|[!\$&'\(\)\*\+,;=]|*@)?(((\d|[1-9]\d|1\d\d|2[0-4]\d|25[0-5])\.(\d|[1-9]\d|1\d\d|2[0-4]\d|25[0-5])\.(\d|[1-9]\d|1\d\d|2[0-4]\d|25[0-5])\.(\d|[1-9]\d|1\d\d|2[0-4]\d|25[0-5]))|((([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])*([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])))\.)+(([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])*([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])))\.?)(:\d*)?)(\/((([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(%[\da-f]{2})|[!\$&'\(\)\*\+,;=]|:|@)+(\/(([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(%[\da-f]{2})|[!\$&'\(\)\*\+,;=]|:|@)*)*)?)?(\?((([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(%[\da-f]{2})|[!\$&'\(\)\*\+,;=]|:|@)|[\uE000-\uF8FF]|\/|\?)*)?(\#((([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(%[\da-f]{2})|[!\$&'\(\)\*\+,;=]|:|@)|\/|\?)*)?$/, "alertText": "* Invalid URL" }
-
If I do NOT want to use "Multiviews", how would I write the Rewrite rule for the following...
I want to rewrite www.mydomain.com/profile to www.mydomain.com/profile.php
I assumed it would be this below, but it's not working...
Options +FollowSymlinks RewriteEngine on RewriteRule ^profile$ /profile.php [NC,L]
Any ideas?
-
Using Multiviews is now causing issues with other rewrites.
When I wasn't using Multiviews, the "Create Quiz redirect" below worked fine. Once I started using Multiviews (i.e. once I added "+Multiviews" to the code), that redirect doesn't work and says (in Chrome)... "This webpage has a redirect loop - The webpage at http://www.mysite.com/createquiz/overivew has resulted in too many redirects. Clearing your cookies for this site or allowing third-party cookies may fix the problem. If not, it is possibly a server configuration issue and not a problem with your computer."
Anyone see the possible issue in my code below?...
RewriteBase / Options +FollowSymlinks +MultiViews RewriteEngine on #sending all non-www requests to www RewriteCond %{http_host} ^mysite.com [nc] RewriteRule ^(.*)$ http://www.mysite.com/$1 [r=301,nc] #sending all index.php requests to root RewriteCond %{THE_REQUEST} "GET /index.php HTTP/1.1" RewriteRule index\.php http://www.mysite.com/ [R=301,L] #Create Quiz redirect RewriteRule ^createquiz/overview /createquizoverview.php [NC,L]
-
Had no idea about multiviews, thanks for sharing! I'll definitely use that
-
One more UPDATE. This is from my server's error log. Maybe it will help?....
[Thu Dec 08 12:57:41 2011] [alert] [client 24.187.116.213] /home/me/public_html/mydomain.com/.htaccess: Invalid command '\xa6sending', perhaps misspelled or defined by a module not included in the server configuration
-
UPDATE INFO (which may or may not help)...
I removed the .htaccess file completely and went directly to the profile.php page and it worked.
I then put the .htaccess file back and went directly to the profile.php page again and it gave the 500 internal server error.
So it seems like the rewriting isn't the problem, but the htaccess file clearly is involved since there are no problems until that file exists.
How could the htaccess file break one simple page, while all others in my application work fine?
-
This might not be enough info for anyone to answer, but I am desperate so giving it a shot. I am building an application locally and I am using mod_rewrite to display seo-friendly URLs.
I have a page called profile.php and I simply want the URL to show as mydomain.com/profile instead of mydomain.com/profile.php. I added this info to my .htaccess file...
Options +FollowSymlinks RewriteEngine on RewriteRule ^profile /profile.php [NC,L] RewriteRule ^create/data /createdata.php [NC,L]
The "create/data" rewrite rule works perfectly. But the profile one will not work. I tried deleting every rule except that one, I tried commenting out the "Options" line, etc. and everytime, I get this 500 Internal Server Error message...
The server encountered an internal error or misconfiguration and was unable to complete your request. Please contact the server administrator, webmaster@mydomain.com and inform them of the time the error occurred, and anything you might have done that may have caused the error. More information about this error may be available in the server error log. Additionally, a 500 Internal Server Error error was encountered while trying to use an ErrorDocument to handle the request.
I tried deleting everything out of the profile.php page and it still happens, so pretty sure that means there is nothing wrong with profile.php page itself.
Anyone have any idea what I can check? This is driving me absolutely nuts.
I'd rather it not work for any rules, but the fact that every other rule except this one works fine makes no sense.
Any suggestions on what to try would be incredibly appreciated!
-
ok, thanks very much for the info!!
-
Can you include a file and append info to be grabbed by using GET? For example, can you have an include file like this...
include('file.php?id=1');
And then in the file "file.php" I would use $_GET['id'] to do other things in the code.
I tried it and it's not working. I'm getting this error... "Warning: include(file.php?id=1) [function.include]: failed to open stream: No such file or directory in..."
Just want to find out if this should be doable before I continue testing why it's not working for me.
If it should work, should I be using something other than include maybe? (like require or require_once?)
-
Awesome, i will totally do that. Thanks so much!
-
Meaning if ever a visitor clicked a link to a page on my site that didn't exist, they wouldn't see a nasty 404 error, but would simply be sent to the home page.
I know I should still do everything possible to make sure there are no 404s, but if there are, the rewrite rule would at least help (if there is one).
-
Is there a way to send any URL requests that don't actually bring up a page to just go to the homepage?
If so, wouldn't this solve any 404 problems? Or is the fact that the page originally being looked for doesn't exist still a problem, even if the visitor is redirected to the home page? I assume the latter, but still let me know if the first question is doable?
-
Got it to work with this below. Hope I did it right...
RewriteRule ^admin admin.php [L]
-
I have a "clean" url of mysite.com/admin and I simply want that to bring up the "actual" page mysite.com/admin.php. I tried the code below and several variations, but it's not working. Anyone tell me what I'm doing wrong?...
<IfModule mod_rewrite.c> RewriteEngine On RewriteRule ^([^/\.]+)/admin$ admin.php [L] </IfModule>
Anyone?
-
Thanks, after some tinkering I got it to do the bold properly. Not really sure what I did wrong at first, but it's working as I expected now. At one point, the code was getting echoed back into an h2 tag, so EVERYTHING was bold and that was throwing me off as well :-\
Also, i noticed these strange characters in the MySQL table where the stuff is stored. I assumed this is normal and just how MySQL stores it. If this is bad for some reason, please let me know
<p>Prove you’re a loyal member of the team and “the leagueâ€
Anyway, thanks for answering!
-
Say I have the following text stored in a MySQL database...
<b>Classic Quote from movie</b>
and I retrieve it into a variable called $text, how do I properly echo that so that it keeps the bold tags and actually display the text "Classic quote from movie" in BOLD?
I'm doing something wrong somewhere along the line (simply doing "echo $text;") because it displays on the page as...
<b>Classic Quote from movie</b>
Instead of...
Classic Quote from movie
Any info on properly storing and echoing back HTML would be very appreciated.
-
I have code that I want to increase a column in my products table by 1. So far I have this for my query...
$sql = "UPDATE products SET orderclicks = (??????) WHERE productid= '$productid'";
I just need help with that part in question marks. In plain english, it would say "SET orderclicks = orderclicks+1"
I tried that and it didn't work. I could easily run a query to get the value of 'orderclicks' first and then add one to that and then run another query to update it to the new value, but I imagine there is a way to do it in just ONE query, so it's more efficient
Ordering issue
in PHP Coding Help
Posted
Sorry, I figured it out. Instead of echoing all the image code, I stored it in $imagecode (i.e. $imagecode .=) and then echoed it later on. Sorry for wasting your time with something so easy :/