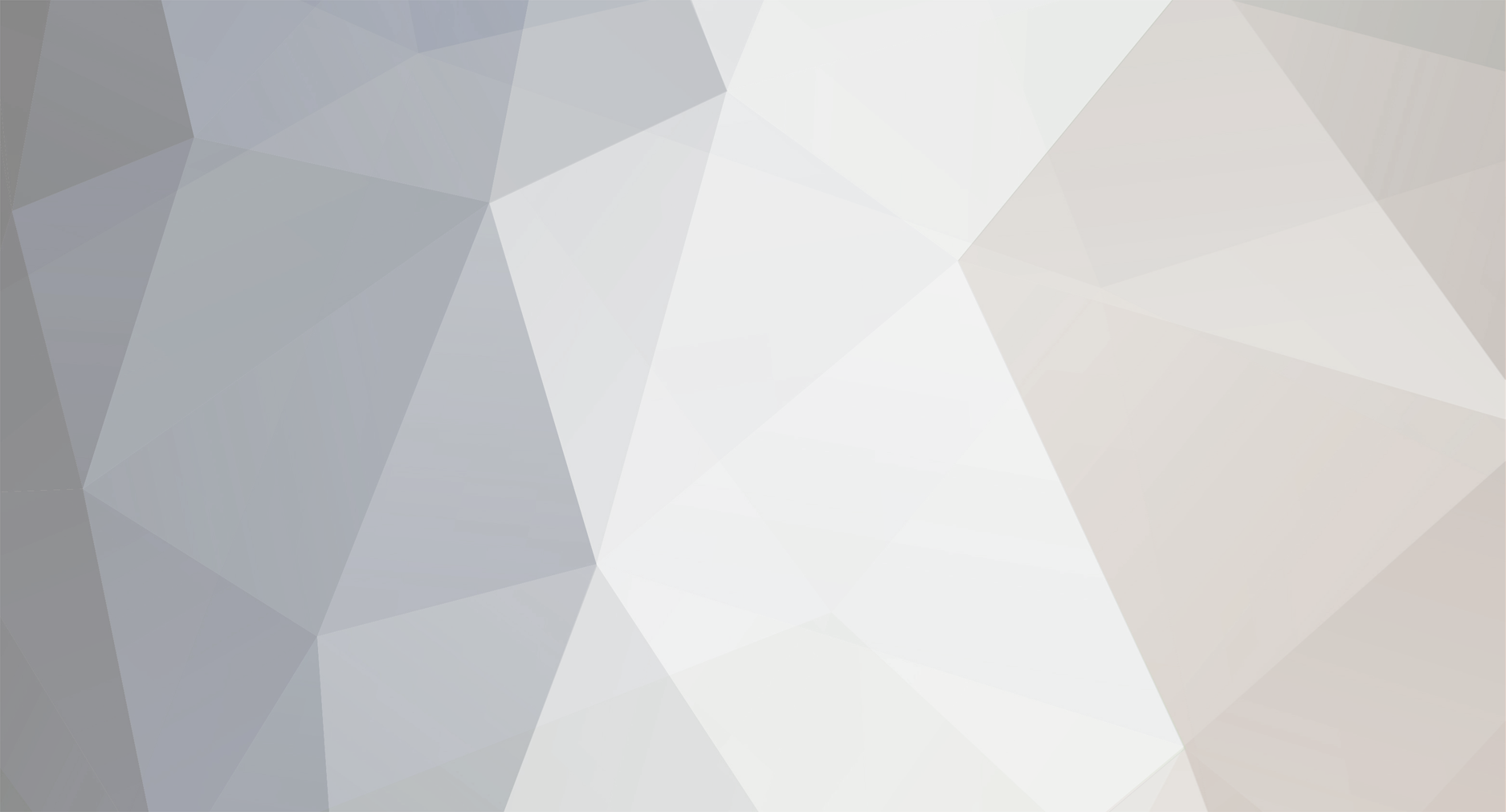
galvin
-
Posts
614 -
Joined
-
Last visited
Posts posted by galvin
-
-
Weird question, but is there a way using PHP to "check" to see if a certain URL brings up an actual real page, or if it brings up a 404 error or something? Any functions do anything like that?
-
Ok will do, thanks Thorpe!
-
I am using the great script from this site (http://net.tutsplus.com/tutorials/php/how-to-use-amazon-s3-php-to-dynamically-store-and-manage-files-with-ease/comment-page-1/#comments) and I have everything working properly (i.e. I can upload images from a form on my site into my S3 account).
My plan was that a new "bucket" would be created by each user who visits my site. Then I found out S3 limits accounts to only 100 buckets
. So my original plan is no longer an option. I will have to use basically ONE bucket and just carefully name the image files.
Now my problem is figuring how to retrieve only certain images from what is going to be a HUGE bucket of images. Below is the code that grabs ALL images from a bucket and loops through them.
Does anyone familiar with S3 and/or this script know how I could tell it to, for example, only retrieve images with a filename that starts with "11111"?
<?php // Get the contents of our bucket $bucket_contents = $s3->getBucket("jurgens-nettuts-tutorial"); foreach ($bucket_contents as $file){ $fname = $file['name']; $furl = "http://jurgens-nettuts-tutorial.s3.amazonaws.com/".$fname; //output a link to the file echo "<a href=\"$furl\">$fname</a><br />"; } ?>
/* * Get contents for a bucket * * If maxKeys is null this method will loop through truncated result sets * * @param string $bucket Bucket name * @param string $prefix Prefix * @param string $marker Marker (last file listed) * @param string $maxKeys Max keys (maximum number of keys to return) * @return array | false */ public static function getBucket($bucket, $prefix = null, $marker = null, $maxKeys = null) { $rest = new S3Request('GET', $bucket, ''); if ($prefix !== null && $prefix !== '') $rest->setParameter('prefix', $prefix); if ($marker !== null && $prefix !== '') $rest->setParameter('marker', $marker); if ($maxKeys !== null && $prefix !== '') $rest->setParameter('max-keys', $maxKeys); $response = $rest->getResponse(); if ($response->error === false && $response->code !== 200) $response->error = array('code' => $response->code, 'message' => 'Unexpected HTTP status'); if ($response->error !== false) { trigger_error(sprintf("S3::getBucket(): [%s] %s", $response->error['code'], $response->error['message']), E_USER_WARNING); return false; } $results = array(); $lastMarker = null; if (isset($response->body, $response->body->Contents)) foreach ($response->body->Contents as $c) { $results[(string)$c->Key] = array( 'name' => (string)$c->Key, 'time' => strToTime((string)$c->LastModified), 'size' => (int)$c->Size, 'hash' => substr((string)$c->ETag, 1, -1) ); $lastMarker = (string)$c->Key; //$response->body->IsTruncated = 'true'; break; } if (isset($response->body->IsTruncated) && (string)$response->body->IsTruncated == 'false') return $results; // Loop through truncated results if maxKeys isn't specified if ($maxKeys == null && $lastMarker !== null && (string)$response->body->IsTruncated == 'true') do { $rest = new S3Request('GET', $bucket, ''); if ($prefix !== null) $rest->setParameter('prefix', $prefix); $rest->setParameter('marker', $lastMarker); if (($response = $rest->getResponse(true)) == false || $response->code !== 200) break; if (isset($response->body, $response->body->Contents)) foreach ($response->body->Contents as $c) { $results[(string)$c->Key] = array( 'name' => (string)$c->Key, 'time' => strToTime((string)$c->LastModified), 'size' => (int)$c->Size, 'hash' => substr((string)$c->ETag, 1, -1) ); $lastMarker = (string)$c->Key; } } while ($response !== false && (string)$response->body->IsTruncated == 'true'); return $results; }
-
I hope this is enough info, but I have this code (at the bottom) that grabs images from my S3 account (Amazon Web Services)... If there is no bucket for the name given, it gives this warning...
Warning: S3::getBucket(): [NoSuchBucket] The specified bucket does not exist in /home/xxxxxxx/public_html/xxxxxxx.com/S3.php on line 126
Assuming it's not specific syntax to S3 and is general PHP syntax, how could I prevent that warning from showing, by saying in plain English "If a bucket by that name doesn't exist, I'm gonna do something else and NOT spit out this warning?"
Basically, I want to echo a message like "There is no bucket yet" instead of having that ugly warning.
// Get the contents of our bucket $contents = $s3->getBucket("mybucket"); foreach ($contents as $file){ $fname = $file['name']; $furl = "http://mybucket.s3.amazonaws.com/".$fname; //output a link to the file echo "<a href=\"$furl\">$fname</a><br />"; echo "<img src='$furl' width='50' /><br />"; echo "$furl<br />"; }
-
Interesting. So since there sometimes will be zero values that I do NOT want to be seen as empty, I guess I can't use the empty syntax in this case. I'll try !=="" instead...
Thanks!
-
Ok all set now. Totally forgot to specify the mysql field (called "result"), i.e. instead of if(!empty($isaresult)) , it needed to be if (!empty($isaresult['result'])).
Sorry for wasting your time. Although, thank you for telling me about using "!empty" to check. I will use that syntax going forward
Thanks!
-
Wait, I think I just realized my stupid mistake...
-
I think this below is all that is necessary (this is all within a larger loop, but it's all working right...my only issue is figuring how to properly say in plain english "If $isaresult has nothing in it, do this. But if $isaresult has any type of value in it (including 0), do this". FYI, the "result" column in the database is blank except for one of them that has the number "1" in it (for testing purposes)...
$sql = "SELECT result FROM picks WHERE picks.userid = '{$users["userid"]}' AND picks.teamid = '{$nextpick["teamid"]}' AND picktype='high'"; $istherearesult= mysql_query($sql, $connection); if (!$istherearesult) { die("Database query failed: " . mysql_error()); } else { $isaresult = mysql_fetch_array($istherearesult); if ($isaresult !== '') { $class="done"; } else { $class="notyet"; } }
When i do that, the class variable is showing as "done" for all of them, even though I put the number "1" in one of them. That one should be coming up with a class of "notyet"
-
If I do a mysql query from a database on a field that either has a number or is blank, how can I write php to check that.
In other words, I am getting that value back into a variable called $isaresult and doing...
if ($isaresult !== '') { //value exists } else { //no value yet }
It's that first line of code that doesn't seem to be working. Should I be using "null" or something instead?
I'm not real experienced with checking if a value exists, so let me know the proper way to do it (since I assume there are multiple ways).
Thanks!
-
Ahhhh, ok, so the process of uploading the images will still put some stress on my server. I was thinking the images could somehow be uploaded directly to Amazon, but apparently not. Anyway, I'm sure the hosting of all the images on a separate server will still prove to be worth it in the end.
Thanks!
-
Thanks for the reply requinix! I'll read about Amazon's service more on their site, but just curious, I assume I would be able to have an upload image area on my site where users could upload images directly to my Amazon S3 account. I could fully customize that area to match my website design right? (colors, font, etc).
-
I am building a website that will essentially have information in table-like grids. Many of the tables will have images for each piece of info. The images will only be like 100x100 at most, but each table (i.e. each page of the site) may have between 0 and 100 images. And by its nature, there is no limit on how many tables/pages there will be total. It will constantly be growing, well into the thousands, because a lot of the info will be user-generated/uploaded.
So, all along, I was assuming I'd use a site like imageshack and either implement their API on my site, or have detailed instructions explaining the very simple steps to upload an image to imageshack and get the "direct link. FYI, I would pay the $5 a month premium price at ImageShack which they confirmed allows me to hot link as many images as I want. This would obviously keep all the image bandwidth off of my server.
But lately some people have warned me that it's risky to have all your images under someone else's control, especially "shady" image hosting sites.
So based on that, I looked into the possibility of allowing users to upload images directly onto on my own "shared hosting" server with HostGator (under a different sub-domain to help with parallel downloading). This would give me full control of the images and be the easiest option for users, but my plan is for the site to have thousands and thousands of images within a few years, so I feel like it will just crash my shared hosting server eventually and I'd have to go bigger. So that made me consider going right to a VPS server. I just feel like that will be unnecessary for at least a few years, so maybe I should start on shared hosting and then move once I run into bandwidth problems (not sure that's they right way to think though).
And of course, I'm also beginning to research a THIRD option of using CDNs (like Amazon's S3 and Cloudfront). Never having done a site with tons of images before, it's hard for me to know if that type of service is even necessary or not.
Anyway, I'm just looking for anyone's general feedback or experiences they've shared/knowledge gained on this confusing subject of where to host images when your site will have A LOT of them. I need to make a decision soon since most of my site is done EXCEPT for the whole aspect of how I'm going to let people get their images onto the site. I'm terrified to make the wrong decision up-front that may cause me massive headaches down the road.
Any insight would be appreciated.
Thanks!
-
Yes, I meant subtract, sorry
Anyway, your info helped me make it do what I needed, thank you so much!
-
Say I do a query from a database that only asks for one field in return (which always has a number in it). How do you write the PHP to take the first number returned and add it to the next number returned (NOTE: There may be more than two numbers returned, but for now, I'm always dealing with two)
For example, say I do a query that returns the number 27 first and then the number 10 second. FYI, these numbers will be available in the variable $diff['result']. So the code after the query would look something like this, but obviously i'm struggling with the part in comments...
if (!$numbers) { die("Database query failed: " . mysql_error()); } else { while ($diff = mysql_fetch_array($numbers)) { //this would bring back 27 first, in the variable $diff['result'] and then on the 2nd loop through, it would bring back 10 in the $diff['result'] variable //ultimately, i just want the difference between the numbers (which in the case would be 17). It's just a simple addition, so Im obviously an idiot because I can't figure out the syntax for adding the first returned number to the next returned number:) } }
-
Ok, I believe I got this working. I included my code below. If you see anything wrong with it, please let me know, but it handles both of these situations, so I think I'm in good shape...
1. If someone goes to mysite.com, it will redirect them to www.mysite.com
2. If someone goes to any page in the structure of mysite.adifferentsite.com/anypage.php, it will redirect to www.mysite.com/anypage.php
Options +FollowSymlinks RewriteEngine on rewritecond %{http_host} ^mysite.adifferentsite.com [nc] rewriterule ^(.*)$ http://www.mysite.com/$1 [r=301,nc]
-
Figured it out, had to reverse the order of allow,deny in the first line to say "deny,allow" instead. Works now. Thanks!
-
Thanks both of you! MarPlo, I did what you said and it blocked me from getting there (which is what I wanted). Then I added my IP address per your instructions, yet I still can't access it from that IP. I added...
order allow,deny deny from all allow from XXX.XXX.XX.X
(with the X's being my actual IP of course).
So just curious if you know of anything that might prevent this from working as intended?
-
Is there an easy way to have a site live, but only have it visible to me. In other words, I'll be building the site while it's live and don't want anyone going to it accidentally until it's ready.
Before everyone jumps down my throat
, I know all developing/testing should be done locally, but I'm just curious if there is an easy way to keep a site private if it must be developed while live.
I guess making a simple login would do it. But then I'd have to have code on every page that says "if not logged in, don't allow to see this page". I guess i could put that code in a header include file to avoid having to add it to every page manually.
Ok, sorry for that, but I think I just talked myself into how to do it.
But I'm going to post the question anyway in case there is a better/smarter way.
Thanks everyone!
-
I'll give it a go, thanks! And FYI, I just realized i wrote the wrong thing. If my main domain is mysite.com, then my subdomain (that I don't like and want to direct traffic away from) is actually mysite.adifferentsite.com (NOT adifferentsite.mysite.com). I don't think that changes anything, so I'll see what happens.
If you're wondering why there is that subdomain, I have shared hosting on HostGator and you only have one MAIN domain and a bunch of ADDON domains. But for some reason, HostGator makes all pages that you put on addon domains also show up via addondomain.maindomain.com.
So if my main domain is mainsite.com, I might have a completely different site using one of my addon domains at addonsite.com. But again, the site that shows up at addonsite.com also shows up at addonsite.mainsite.com.
I'm sure HostGator has good reason for it, but they should probably make it more obvious that it does this. I just discovered it after months.
i'll let you know what happens.
-
Ok, so the 2nd rule is wrong
. So how should I write the 2nd rule to say, in plain english...
If anyone tries to go to any page that has the root domain of adifferentsite.mysite.com (whether it's adifferentsite.mysite.com/content.php or adifferentsite.mysite.com/monkeys.php or whatever), redirect them to mysite.com?
I tried requinix's suggestion but it didn't work (and cags is saying that it is not doing what I intended anyway)
Bottom line is that I want to send all non-www requests to the www version. That is the first rule and it works fine. Now I just need to figure out how to do the 2nd part explained again above, i.e. forwarding any page with that subdomain in it (i.e. adifferentsite.mysite.com) to my main domain (mysite.com)
Thanks!
-
That didn't work (kicking an "internal server error"). My htaccess file already has this info in it...
Options +FollowSymlinks RewriteEngine on rewritecond %{http_host} ^mysite.com [nc] rewriterule ^(.*)$ http://www.mysite.com/$1 [r=301,nc]
So based on your reply, I added it to be this. Do you see anything wrong with how I wrote this? Can you have two separate rules in there? ...
Options +FollowSymlinks RewriteEngine on rewritecond %{http_host} ^mysite.com [nc] rewriterule ^(.*)$ http://www.mysite.com/$1 [r=301,nc] RewriteCond %{HTTP_HOST} !^mysite.com$ RewriteRule ^ - mysite.com%{REQUEST_URI} [L]
-
Say I have a site with the main URL of mysite.com
And let's say my hosting company for some strange reason automatically creates a subdomain of adifferentsite.mysite.com which shows the exact same content as what shows at mysite.com
How would I write the 301 redirect (in the htaccess) file to say in plain english...
If anyone tries to go to any page that has the root domain of adifferentsite.mysite.com (whether it's adifferentsite.mysite.com/content.php or adifferentsite.mysite.com/monkeys.php or whatever), redirect them to mysite.com?
-
Great, thanks so much for the info!
-
Thanks fry2010. I was also thinking about doing a 301 redirect of the adifferentsite.mysite.com URL to the mysite.com URL
Do you know which method would be better (i.e. 301 redirect or your canonical suggestion)? Or does it not really matter, as long as one of them is done
Shopping Cart of choice?
in Applications
Posted
I've never been steered wrong by anyone on this site, so I want to ask...
I have a client whose website I did and he is ready to begin selling products on the site. I have yet to do anything with eCommerce, but now is the time to learn
. Money is not an issue, so if the best solution is a paid one, that is fine with him. With that said, which shopping cart solution do you recommend for someone in this situation...
1. Fairly experienced with PHP/MySQL
2. Website is coded from scratch in HTML/PHP (i.e. it is NOT a CMS)
3. Money is not an issue, although if you truly feel the best solution is a free solution, we'd certainly entertain that option
After doing some googling, it seems like the decision may be between Zen Cart and Volusion. If so, let me know which you think I should go with. And if there is another one I should consider, I'm all ears
One last question: He would likely want to accept all standard Credit Cards and PayPal, so is this handled via whatever eCommerce solution you suggest, or is billing done through a separate solution (like maybe PayPal itself?)? I realize this question may not make sense because as I said, I have never done any eCommerce work before
Thanks!