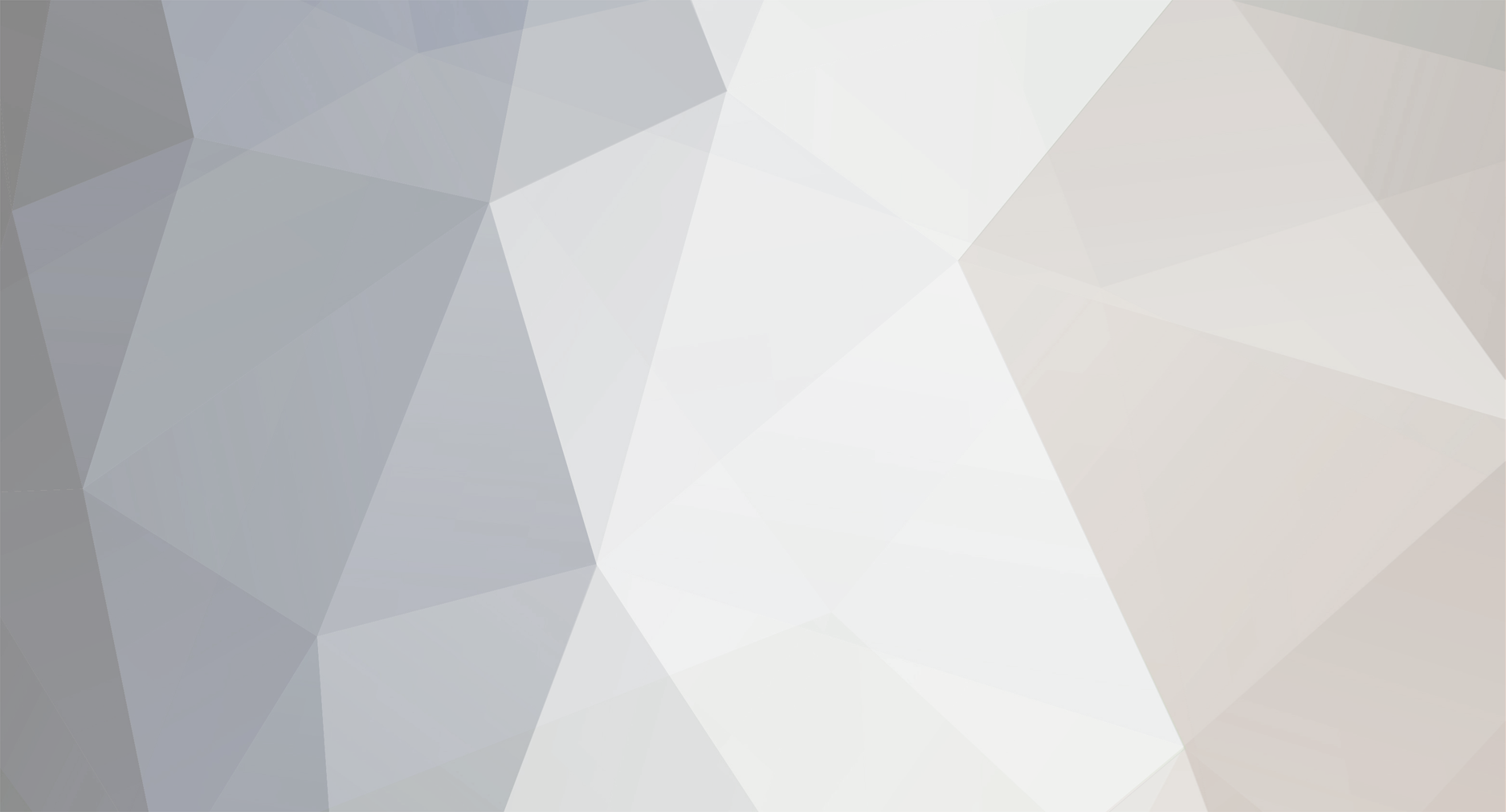
bluesoul
Members-
Posts
149 -
Joined
-
Last visited
Everything posted by bluesoul
-
Made some additional changes and the fact that it's still not working is surprising. expwhois.php: <?php function array_whois($array) { if (!is_array($array)) { die('WHOIS script was not passed an array.'); } else { $count = count($array); $i = 0; include('whoisservers2.php'); // there's a case-switch with TLDs and their corresponding registries. for($i = 0; $i < $count; $i++) { $domain[$i] = $array[$i]; $tempdomain = $domain[$i]; $q = "SELECT tld, exp_date FROM Domain WHERE domain_name = '$tempdomain'"; $query = mssql_query($q) or die(mssql_get_last_message()); $info = mssql_fetch_array($query); $topleveldomain[$i] = strtolower($info["tld"]); // necessary to avoid conflict with $tld for whoisservers.php $tld = $topleveldomain[$i]; // $tld gets used to find the right WHOIS server $exp_date[$i] = $info["exp_date"]; $comnet = 0; getserver(); if($comnet == 1) { // .COM and .NET domains operate differently, Network Solutions will redirect you to another registrar. $j = 0; $fp = fsockopen($server,43); fputs($fp, "$tempdomain\r\n"); $num = 10; // Retrieving the line that specifies the correct whois server, in this case line 10. for ($j=0; $j<$num; $j++) $line = fgets($fp); if (strlen($line) > 1) { // Actual Network Solutions-owned names have a blank line 10 so comparing string length works. $server = substr($line,17); // Snip everything before the start of the correct whois server. $server = substr($server,0,-1); // Snip the trailing linebreak and this is now the server we'll check against, and the user never sees any of this. } } echo '$array: '; print_r($array); echo '<br>$domain: '; print_r($domain[$i]); echo '<br>$tld: '; print_r($tld); echo '<br>$exp_date: '; print_r($exp_date[$i]); echo '<br>$server: '; print_r($server); $fp = fsockopen($server,43); // we define $server in whoisservers.php and later refine it if comnet = 1 fputs($fp, "$tempdomain\r\n"); while(!feof($fp)) { echo stristr(fgets($fp),"expir"); } fclose($fp); } //end for loop } //end else }//end function ?> whoisservers2.php: <? function getserver() { //nothing in here should need modifying unless you find a particular whois server is either missing or incorrect. echo "function called"; global $comnet; global $tld; global $server; switch($tld) { case 'ac': $server = 'whois.nic.ac'; break; ... case 'com': echo "hi"; $server = 'whois.internic.net'; $comnet = 1; ... default: echo "default"; $server = 'whois.internic.net'; $comnet = 1; break; A submission of an array containing level-up.com yields this: function called default $array: Array ( [0] => level-up.com ) $domain: level-up.com $tld: com $exp_date: Aug 2 2009 12:00AM $server: Notice: Undefined variable: server in expwhois.php on line 45 Notice: Undefined variable: server in expwhois.php on line 49 Warning: fsockopen() [function.fsockopen]: unable to connect to :43 (A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond. ) in expwhois.php on line 49 Fatal error: Maximum execution time of 30 seconds exceeded in expwhois.php on line 49 ---- So it seems like it's getting stuck somewhere in the case switch.
-
something like... $query1 = "INSERT INTO users(id, username, password) VALUES (NULL, '$username', '$password')"; ... $lastid = mysql_insert_id($conn); //where $conn is the resource identifier you used in mysql_connect $query2 = "INSERT INTO work (id, job, user_id) VALUES (NULL, '$job', '$lastid')";
-
2. I tried foreach but I've never used it before and made a mess of it. Guess I didn't do much better here but I at least got a result with 1 element. 1. <?php function array_whois($array) { if (!is_array($array)) { die('WHOIS script was not passed an array.'); } else { $count = count($array); $i = 0; include('whoisservers2.php'); // there's a case-switch with TLDs and their corresponding registries. for($i = 0; $i < $count; $i++) { $domain[$i] = $array[$i]; $tempdomain = $domain[$i]; $q = "SELECT tld, exp_date FROM Domain WHERE domain_name = '$tempdomain'"; $query = mssql_query($q) or die(mssql_get_last_message()); $info = mssql_fetch_array($query); $topleveldomain[$i] = strtolower($info["tld"]); // necessary to avoid conflict with $tld for whoisservers.php $tld = $topleveldomain[$i]; // $tld gets used to find the right WHOIS server $exp_date[$i] = $info["exp_date"]; $comnet = 0; $server = getserver(); if($comnet == 1) { // .COM and .NET domains operate differently, Network Solutions will redirect you to another registrar. $j = 0; $fp = fsockopen($server,43); fputs($fp, "$tempdomain\r\n"); $num = 10; // Retrieving the line that specifies the correct whois server, in this case line 10. for ($j=0; $j<$num; $j++) $line = fgets($fp); if (strlen($line) > 1) { // Actual Network Solutions-owned names have a blank line 10 so comparing string length works. $server = substr($line,17); // Snip everything before the start of the correct whois server. $server = substr($server,0,-1); // Snip the trailing linebreak and this is now the server we'll check against, and the user never sees any of this. } } /*echo '$array'; print_r($array); echo '$domain'; print_r($domain[$i]); echo '$tld'; print_r($tld); echo '$exp_date'; print_r($exp_date[$i]); echo '$server'; print_r($server); */ $fp = fsockopen($server,43); // we define $server in whoisservers.php and later refine it if comnet = 1 fputs($fp, "$tempdomain\r\n"); while(!feof($fp)) { echo stristr(fgets($fp),"expir"); } fclose($fp); } //end for loop } //end else }//end function ?> And a relevant snippet of whoisservers2.php: <? function getserver() { //nothing in here should need modifying unless you find a particular whois server is either missing or incorrect. global $comnet; global $tld; switch($tld) { case 'ac': $server = 'whois.nic.ac'; break; //etc } return $server; } ?>
-
mysql_insert_id() will get the last generated ID.
-
Good catch on the second $i, I completely overlooked it. I've turned the case-switch in whoisservers.php into a function and call it now, and I'm having the same error. Notice: Undefined variable: server in expwhois.php on line 49 Warning: fsockopen() [function.fsockopen]: unable to connect to :43 (A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond. ) in expwhois.php on line 49 Fatal error: Maximum execution time of 30 seconds exceeded in expwhois.php on line 49 EDIT: It's now not working for even one element.
-
I'm not sure where the exact problem is here. I'm trying to make a script to compare expiration dates of domains in our database against the dates on the actual WHOIS servers, it takes an array (which appears to be fine in print_r) and does the following function array_whois($array) { if (!is_array($array)) { die('WHOIS script was not passed an array.'); } else { $count = count($array); $i = 0; for($i = 0; $i < $count; $i++) { $domain[$i] = $array[$i]; $tempdomain = $domain[$i]; $q = "SELECT tld, exp_date FROM Domain WHERE domain_name = '$tempdomain'"; $query = mssql_query($q) or die(mssql_get_last_message()); $info = mssql_fetch_array($query); $topleveldomain[$i] = strtolower($info["tld"]); // necessary to avoid conflict with $tld for whoisservers.php $tld = $topleveldomain[$i]; // $tld gets used to find the right WHOIS server $exp_date[$i] = $info["exp_date"]; include('whoisservers.php'); // there's a case-switch with TLDs and their corresponding registries. if($comnet == 1) //$comnet is defined as 0 in whoisservers.php and set to 1 in the instances of com and net. { // .COM and .NET domains operate differently, Network Solutions will redirect you to another registrar. $fp = fsockopen($server,43); fputs($fp, "$tempdomain\r\n"); $num = 10; // Retrieving the line that specifies the correct whois server, in this case line 10. for ($i=0; $i<$num; $i++) $line = fgets($fp); if (strlen($line) > 1) { // Actual Network Solutions-owned names have a blank line 10 so comparing string length works. $server = substr($line,17); // Snip everything before the start of the correct whois server. $server = substr($server,0,-1); // Snip the trailing linebreak and this is now the server we'll check against, and the user never sees any of this. } } /*echo '$array'; print_r($array); echo '$domain'; print_r($domain[$i]); echo '$tld'; print_r($tld); echo '$exp_date'; print_r($exp_date[$i]); echo '$server'; print_r($server); */ $fp = fsockopen($server,43); // we define $server in whoisservers.php and later refine it if comnet = 1 fputs($fp, "$tempdomain\r\n"); while(!feof($fp)) { echo stristr(fgets($fp),"expir"); } fclose($fp); } //end for loop } //end else }//end function This works fine with an array with 1 element but any more than that and the script times out. Any ideas?
-
HELP!! - PEAR SMTP Mail, on Windows XP - OPEN TO OTHE OPTIONS...
bluesoul replied to lmaster's topic in PHP Coding Help
Eh, the best thing I can tell if you if you prefer to go with that script is to check online references for TLS and tweak it until it does as it should, that was why I had the echo of both sent and received messages. The methodology to add/change entries is pretty straightforward. -
overview of php secuirty. what should i be doing?
bluesoul replied to daydreamer's topic in PHP Coding Help
Also if you need to know what your host has enabled don't forget about ini_get(). -
I have to agree, even though I was already comfortable with MySQL I took an Intro to Databases class at the local junior college and a good foundation really helped write good SQL later on, and I even learned a few new tricks. Learning normalization and indexing will really pay off in the long run.
-
HELP!! - PEAR SMTP Mail, on Windows XP - OPEN TO OTHE OPTIONS...
bluesoul replied to lmaster's topic in PHP Coding Help
It is possible to connect via telnet and send mail that way, it's not nearly as elegant as using a PEAR module but I didn't have PEAR available to me when I made the following. <html><body> <pre> <? function authSendEmail() { global $to; global $from; global $subject; global $message; $localhost = "localhost"; $username = "smtp_username"; // set to your smtp username $password = "smtp_password"; // and password $smtpConnect = fsockopen('192.168.161.142', 25); // change to your SMTP server, leave the port socket_set_blocking($smtpConnect,1); $smtpResponse = fgets($smtpConnect, 515); $newLine = chr(10); if(empty($smtpConnect)) { $output = "Failed to connect: $smtpResponse"; return $output; } else { echo('Opening Connection to Mail server...<br>>>'.$smtpResponse.'<br>'); } $str = "AUTH LOGIN" . $newLine; //Request Auth Login $test = fputs($smtpConnect,$str,strlen($str)); $smtpResponse = fgets($smtpConnect); echo('AUTH LOGIN<br>>>'.$smtpResponse.'<br>'); //Send username fputs($smtpConnect, base64_encode($username) . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo (base64_encode($username).'<br>>>'.$smtpResponse.'<br>'); //Send password fputs($smtpConnect, base64_encode($password) . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo (base64_encode($password).'<br>>>'.$smtpResponse.'<br>'); //Say Hello to SMTP fputs($smtpConnect, "HELO $localhost" . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo ('HELO localhost<br>>>'.$smtpResponse.'<br>'); //Email From fputs($smtpConnect, "MAIL FROM: $from" . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo ('MAIL FROM: '.$from.'<br>>>'.$smtpResponse.'<br>'); //Email To fputs($smtpConnect, "RCPT TO: $to" . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo ('RCPT TO: '.$to.'<br>>>'.$smtpResponse.'<br>'); //The Email fputs($smtpConnect, "DATA" . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo ('DATA<br>>>'.$smtpResponse.'<br>'); //Construct Headers $headers = "MIME-Version: 1.0" . $newLine; $headers .= "Content-type: text/plain; charset=iso-8859-1" . $newLine; fputs($smtpConnect, "To: $to\r\nFrom: $from\r\nSubject: $subject\r\n$headers\r\n\r\n$message\r\n.\r\n"); $smtpResponse = fgets($smtpConnect, 515); echo ('To: '.$to.'<br>From: '.$from.'<br>Subject: '.$subject.'<br>'.$headers.'<br><br>'.$message.'<br>.<br><br>>>'.$smtpResponse.'<br>'); // Say Bye to SMTP fputs($smtpConnect,"QUIT" . $newLine); $smtpResponse = fgets($smtpConnect, 515); echo ('QUIT<br>>>'.$smtpResponse.'<br>'); } /* * * * * * * * * * * * * * SEND EMAIL FUNCTIONS * * * * * * * * * * * * * */ //Authenticate Send - 21st March 2005 //This will send an email using auth smtp and output a log array //logArray - connection, $to = "destination@example.com"; $from = "sender@example.com"; $subject = "Test"; $message = "Message goes here."; authSendEmail($from, $to, $subject, $message); ?> </pre></body></html> I wrote that so I could see my errors as they encountered and make fixes accordingly. If you're using ESMTP it should work perfectly (tested on an IMail platform), feel free to adapt it to your needs (removing the echoes etc). -
Good to know since I didn't actually test that code.
-
=== also checks for the variable type. $i = string(1); $j = int(1); $i === $j ? echo true : echo false; $i == $j ? echo true : echo false; Would return: false true
-
$result=mysql_query($query,$id) or die ('<META HTTP-EQUIV="Refresh" CONTENT="0;URL=error.php">');
-
Have you tried.. $root = $_SERVER['DOCUMENT_ROOT']; // defined outside the loop $thelist .= '<a href="'.$root.'/'.$file.'">'.$root.'/'.$file.'</a>'.'<br>';
-
How do u do to avoid data being lost from page to page this way?
bluesoul replied to alvarito's topic in PHP Coding Help
Sure you can, $_SESSION can store anything $_GET or $_POST can, it's just a variable. -
How secure is this user/membership code?
bluesoul replied to your.syndrome's topic in PHP Coding Help
As long as you understand what's going on with the code, I don't see anything obviously bad about it. There's only so many ways to make a login script. -
I guess the obvious question is why not just use phpMyAdmin rather than a wheel reinvention?
-
How secure is this user/membership code?
bluesoul replied to your.syndrome's topic in PHP Coding Help
Not quite, from the site: It's nothing more than a dictionary search. MD5 is somewhat vulnerable to hash collisions especially with a database of that size. I believe there's still a distributed computing effort going on to outright crack MD5. -
Ah, I suppose I'm afraid of losing my place in the loop but I suppose with a $_GET or something it could keep going. Thanks for the advice.
-
How secure is this user/membership code?
bluesoul replied to your.syndrome's topic in PHP Coding Help
Yeah the form could be a little better but the functionality's pretty standard, md5 is far and away the most common form of password encryption on php-powered sites. -
How do u do to avoid data being lost from page to page this way?
bluesoul replied to alvarito's topic in PHP Coding Help
If you've already received the information once you could store them in a $_SESSION variable, then check for it in your step 2 code. -
I thought it might be something like that, unfortunately I haven't gotten around to learning AJAX yet. How are you meaning to refresh the page, through javascript or some function I'm not aware of?
-
Okay, I'm trying to give a summary of a loop in progress in the browser window, and the results are unexpected to say the least. The relevant code is: for($i = 0; $i < $j; $i++) { if($i%10) { sleep(3); echo("Processed $i domains of $j...\r\n"); flush(); set_time_limit(30); } (do stuff here) } Generally it'll only spit out updates every 30 seconds or so instead of every 3 seconds which should be all it takes to get to 10 iterations of the loop, it involves sending an email and I don't want to overload the server, hence the sleep(). I'm sure there's a better function than flush for this but I haven't been able to get any results out of anything else. Thanks.