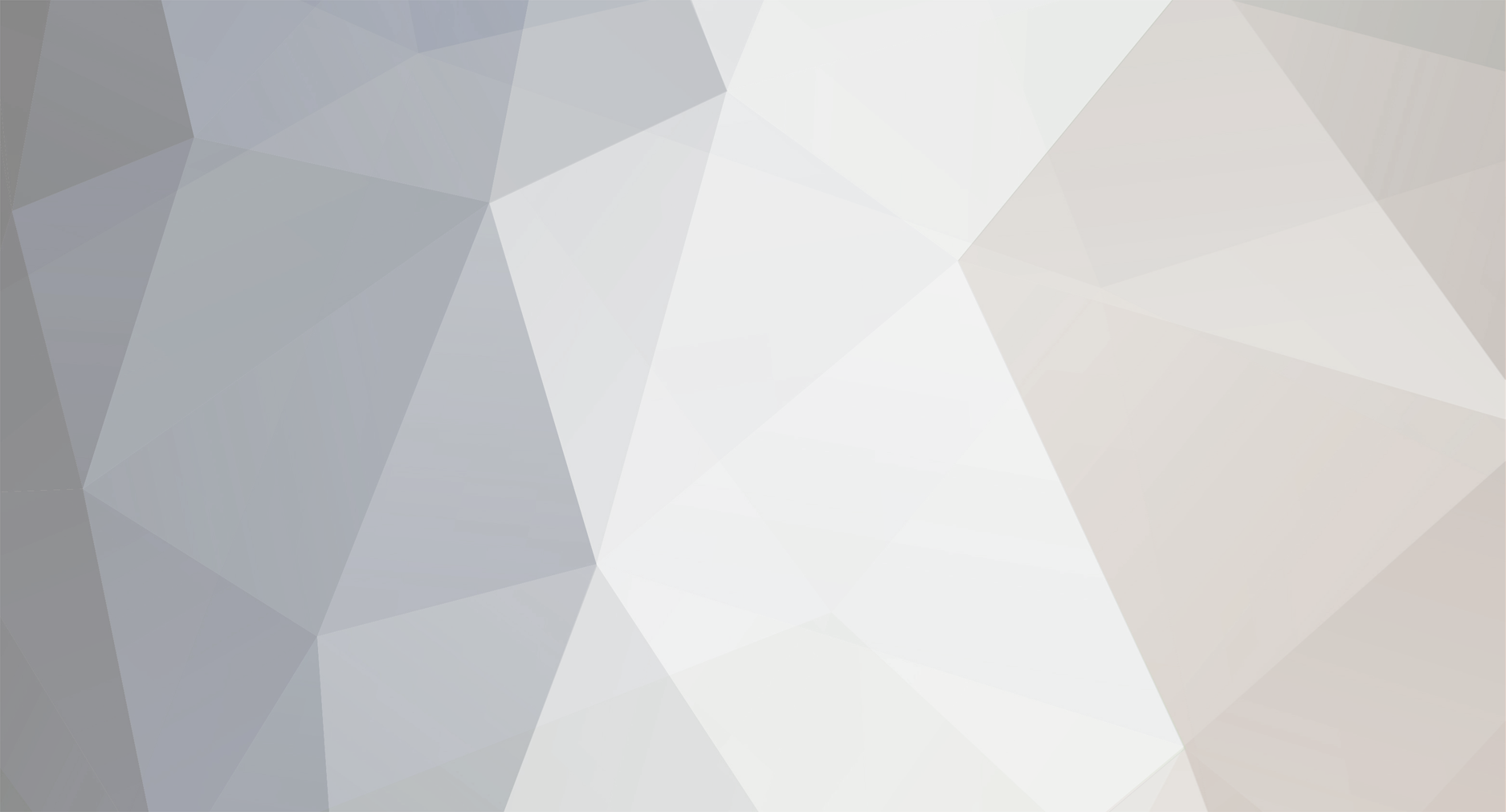
cwarn23
-
Posts
110 -
Joined
-
Last visited
Never
Posts posted by cwarn23
-
-
It's a pitty nobody has used the subforums in the past because on other online communities I know of, I haven't seen a forum with so many new posts in the one forum because of the subforums. I guess people on these forums just don't always have time to think twice about where there post goes.
-
I have noticed that the PHP help section has a lot of new posts during recent times. With so many posts, it is easy for many of them to just age back and never be solved. That is why I suggest adding at least 2 subforums to the PHP Help forum and I have thought hard on what would devide some of the posts and below is a list of the subforms (or known as Child forums) that I think will make the difference.
- PHP Strings & arrays
- PHP mysql_query
The first one on the list (PHP Strings & arrays) will separate a large amount of posts and would mainly be used for help with scripts with the main purpose of processing strings and/or arrays. Also the other proposal on the list (PHP mysql_query) would by scripts using mysql databases. And of course most mysql scripts require the function mysql_query. Having a subforum for mysql scripts could also be usefull for when people want to look for solved threads in relation to mysql.
So with those 2 subforums (or Child forums) and if people use them I believe they will make it easier for asking questions and for browsing the recently posted on topics. That is all I have to suggest, any comments?
- PHP Strings & arrays
-
Try the following:
$username=''; $total_files_home = 0; $total_files_home = count(glob("../users/$username/{*.*}", GLOB_BRACE)); if ($total_files_home < 1) $total_files_home = 0; else $total_files_home = $total_files_home; echo '<a href="manager.php?efolder=home">'; echo '<img src="gfx/FolderIconV2.gif" border="0" alt="Home" width="60" height="50"><br>Home<br></a>( '.$total_files_home.' )<br><br></font>'; foreach(glob('../users/'.$username.'/*', GLOB_ONLYDIR) as $dir) { $dir = str_replace('../users/'.$username.'/', '', $dir); $total_files_sub = count(glob("../users/$username/$dir/{*.*}", GLOB_BRACE)); if ($total_files_sub <= 1) $total_files_sub = 0; else $total_files_sub = $total_files_sub; echo '<a href="manager.php?efolder='.$dir.'">'; echo '<font size=2>'; echo '<img src="gfx/FolderIconV2.gif" border="0" alt="'.$dir.'" width="60" height="50"><br>'.$dir.'<br></a>( '.$total_files_sub.' )<br><BR></font></a>'; }
-
There were bugs all above the loop so I have rewritten it and is as follows:
<?php include('dblogin.php'); $host=''; $user=''; $pass=''; $database=''; $connect = mysql_connect($host, $user, $pass) or die(mysql_error()) mysql_select_db($database) or die(mysql_error()."Could not select database"); $mysql="SELECT * FROM `products`"; $result=mysql_query($mysql); if ($_GET["add"]!="") { $_SESSION["cost"]=($_SESSION["cost"]+$price["price"] ); $_SESSION["products"]=$_SESSION["products"]+1; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <!-- Link to stylesheet stylesheet.css within the same folder as the HTML page --> <link rel="StyleSheet" href="inc/css/stylesheet.css" type="text/css"> <html> <head> </head> <body> <div id="container"> <!-- Start of the Container DIV --> <div class="animatedtabs"> <ul> <li class="selected" ><a href="Shop.php" title="Shop"><span>Shop</span></a></li> <li><a href="index.php" title="Home"><span>Home</span></a></li> <li><a href="new.php" title="New"><span>New</span></a></li> <li><a href="Categories.php" title="Categories"><span>Catogires</span></a></li> <li><a href="safty.php" title="Tools"><span>Safty Center </span></a></li> </ul> </div> <div id="HEADER"> <h1>swapit.com</h1> <div class="Visual"> </div> <div id="CONTENT"> <?php while ($row = mysql_fetch_array($result)) { echo '<div id="products">'; echo '<form name="cart" method="post" action="Shop.php?add=true">'; echo '<table><tr>'; echo '<td><h2>'.$row["title"].'</h2><p>'.$row["description"].'</p></td><td>£'.$row["price"].'</td><td><input type="submit" name="submit" value=" Add to Cart"></td>'; echo '</table></tr>'; echo '</form>'; echo '</div>'; } echo '<div id="cart">'; echo '<form name="cart" method="post" action="shop-cart.php" >'; echo '<p class="center">SHOPPING CART</p>'; echo '<p>No of Items: <strong>'.$_SESSION["products"].'</strong><br/>Total Price: <strong>£'. $_SESSION["cost"].'</strong></p>'; echo '<p class="button"><input type="submit" name="Submit" value=" Check Out >> "shop-cart.php""></p>'; echo '</form>'; echo '</div>'; ?> </div> </div> <?php include('dblogin.php'); if ($_SESSION["userid"]=="") { header ('Location: login.php'); } if ($_SESSION["userid"]!="") { echo '<p><a href="logout.php">Logout</a></p>'; } ?> <!-- Do not edit above this line --> <h2>Products...</h2> <div id="TEXT"> <div id="products"><form name="cart" method="post" action="Shop.php?add=true"><table><tr><td><h2>Hot Fuzz - Limited Edition</h2> <p>1 Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p></td> </td></table></tr><input type="hidden" name="id" value="1"><input type=$price name="price" value="19.99"><td><input type="submit" name="submit" value=" Add to Cart"></form></div> <div id="products"><form name="cart" method="post" action="Shop.php?add=true"><table><tr><td><h2>10000 BC</h2> <p>2 Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p></td> </td></table></tr><input type="hidden" name="id" value="2"> <input type="hidden" name="price" value="24.99"></form></div><div id="products"><form name="cart" method="post" action="Shop.php?add=true"><table><tr> <td><h2>The Matrix</h2><p>3 Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p></td> </td></table></tr><input type="hidden" name="id" value="3"><input type=$price name="price" value="9.99"><input type="submit" name="submit" value=" Add to Cart"></form></div> <div id="products"><form name="cart" method="post" action="Shop.php?add=true"><table><tr> <td><h2>Empire Records</h2><p>4 Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p></td> <td></td></table></tr><input type="hidden" name="id" value="4"> <input type=$price name="price" value="14.99"><<input type="submit" name="submit" value=" Add to Cart"></form></div> <div id="products"><form name="cart" method="post" action="Shop.php?add=true"><table><tr> <td><h2>The Transporter</h2><p>5 Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>< </td></table></tr><input type="hidden" name="id" value="5"><input type=$price name="price" value="12.49"><input type="submit" name="submit" value=" Add to Cart"></form></div> echo '<input type="hidden" name="id" value="'.$row["product_id"].'">'; </div> <!-- Do not edit below this line --> </div> <div id="FOOTER"> <p>swapit.com </p> <p>This website is a university assignment demonstration not a real shop!</p> </div> </body> </html> <!-- CO1505 Assignment 1 Project Example --> <!-- www.000webhost.com Analytics Code --> <script type="text/javascript"> var websiteID='http://swapit.comoj.com'; </script> <script type="text/javascript" src="http://analytics.hosting24.com/do.php"></script> <noscript> <a href="http://www.hosting24.com/"><img src="http://analytics.hosting24.com/do.php?websiteID=chici.org" alt="web hosting" /></a> </noscript> <!-- End Of Code --> <div class="shop"> <li> <!-- the shop--> </div> <!-- End of the Container DIV --> </body> </html>
-
I have just checked the php.ini file and it is a rather simple php.ini file containing only extension entries and one other uncommented line (102 lines including comments) and there seems to be no mention of stripslashes nor magic-quotes so I would assume the interpreter (php.exe part of php-gtk and regular php) would use the defaults.
Its a bit of a mind-twister to work out why the section of code href\\=\\\\\" can escape the quotes after the word echo. I have tried the following code and I still get the error " 'href\' is not recognized as an internal or external command, operable program or batch file."
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std; int main() { string code = "var_dump(preg_split('/(href\\=\\'|href\\=\"|href\\=)/is','adslkfajal;sdfjhref=test.com/test.htm'));"; std::string var = "php -r \" "+code+" \""; system (var.c_str()); system("PAUSE"); return EXIT_SUCCESS; }
Even though in the above code, I replaced href\\=\\\\\" with href\\=\"
Thanks for the reply as on some of my topics I don't get a single reply.
-
Hi and I have been writing a script to convert php code (and php-gtk code) into a string that can be used by c++. The only thing is that when I use the string that my script outputs, php fails to execute the code and because it is done via command line (and c++ system function), the error "The system cannot find the file specified." is with at least one script reported. So somewhere in my script I must be adding too many escape slashes or something and perhaps an extra pair of eyes will help as I have been working at this for 2 weeks. But I do know that the classic hello world example works on this script though. Since my php to string/c++ converter is short I shall post it and is as follows:
<? $_POST['code']=stripslashes($_POST['code']); echo "This form will convert yur php-gtk code into a c++ program that if you place in the same directory as the official php-gtk viewer, it will allow you to view your php-gtk application. Note: You cannot use new lines when placing code into c++. Instead you must use \n.<br><form method='post' style='padding:0px; margin:0px;'><textarea name='code' style='width:100%; height:480px;'>".$_POST['code']."</textarea><br><input type='submit' value='Convert PHP-GTK Code into C++ exe command'></form>"; if (isset($_POST['code'])) { //http://www.weanswer.it/blog/optimize-css-javascript-remove-comments-php/ $_POST['code']=' '.$_POST['code']; while ($_POST['code']!=preg_replace("/(\n|\n*\;[^\'\"]+)((?:\/\*(?:[^*]|(?:\*+[^*\/]))*\*+\/)|(?:\/\/.*))(\n)?/", " $1 ", $_POST['code']) ) { $_POST['code']=preg_replace("/(\n|\n*\;[^\'\"]+)((?:\/\*(?:[^*]|(?:\*+[^*\/]))*\*+\/)|(?:\/\/.*))(\n)?/", " $1 ", $_POST['code']); } while($_POST['code']!=preg_replace("/(\n|\n[^\'\"]+)((?:\/\*(?:[^*]|(?:\*+[^*\/]))*\*+\/)|(?:\/\/.*))(\n)?/", " $1 ", $_POST['code'])) { $_POST['code']=preg_replace("/(\n|\n[^\'\"]+)((?:\/\*(?:[^*]|(?:\*+[^*\/]))*\*+\/)|(?:\/\/.*))(\n)?/", " $1 ", $_POST['code']); } $_POST['code']=str_replace('\\r','\r',$_POST['code']); $_POST['code']=str_replace('\\n','\n',$_POST['code']); $_POST['code']=str_replace(" ",'',$_POST['code']); $_POST['code']=str_replace("\r",'',$_POST['code']); $_POST['code']=str_replace("\n",'',$_POST['code']); $_POST['code']=preg_replace("/<\?(php)?/",'',$_POST['code']); $_POST['code']=str_replace('?>','',$_POST['code']); $_POST['code']=str_replace('\\','\\\\',$_POST['code']); $_POST['code']=str_replace('\"','\\"',$_POST['code']); $_POST['code']=str_replace('"','\\\\\"',$_POST['code']); $_POST['code']=str_replace('{','{ ',$_POST['code']); $_POST['code']=str_replace('}',' }',$_POST['code']); $_POST['code']='#include <cstdio> #include <cstdlib> #include <iostream> using namespace std; int main() { //int argc, char *argv[] //http://ubuntuforums.org/showthread.php?t=588559 string code = "'.$_POST['code'].'"; std::string var = "php -r \" "+code+" \""; system (var.c_str()); //system("PAUSE"); return EXIT_SUCCESS; }'; echo "<table border=0 cellpadding=0 cellspacing=0 width=800><tr><td><xmp style='width:800;'> ".$_POST['code']." </xmp></td></tr></table><hr>"; } else { echo "<p>"; } echo "Please note this exe file must be placed in the same folder as the php.exe file (and other php binary files) as can be downloaded from <a href='http://gtk.php.net/download.php'>http://gtk.php.net/download.php</a> (use the binary download)."; ?>
With the c++ code the above script outputs, if you place the executable the c++ code makes in the same directory as php.exe then it will submit the php code to php.exe via command line. The only problem is that sometimes it is reporting an error saying "The system cannot find the file specified.". But with that error, the command line doesn't have anything to do with files other than the fact it uses the php command line. But when I devide my code into smaller bits and put it through the above script, somehow it is able to escape the quotation marks for the system command and cause the standard dos error saying file or command not recognised. Below is an example of the c++ command (no different to how the above script makes it) and can anybody see in the string code line how the middle double quotation mark might escape the quotation mark on the line below it.
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std; int main() { string code = "var_dump(preg_split('/(href\\=\'|href\\=\\\\\"|href\\=)/is','adslkfajal;sdfjhref=test.com/test.htm'));"; std::string var = "echo \" "+code+" \""; system (var.c_str()); system("PAUSE"); return EXIT_SUCCESS; }
Please reply.
-
There is a very good reason for this which must be known for every mysql query with insert/update data containg quotations. Below is an example of what you would have done to assign a variable to a column:
"`message`='$message'"
But there are 2 problems with that code. First is that in most cases, the string $message will be submitted to the database instead of what is inside the variable.
Wrong. Variables in double quotes are evaluated to their values.
Then there is your question about why use the addslashes function when you are going to remove them. That is because what if the variable contains a quotation mark? If there is a quotation mark in the variable it will escape one of the quotation marks in the mysql query making a fatal error. Below is a basic example of the error:
$message="asdf'asdf'asdfa'aja\"ads"; "`message`='".$message."'"
With those quotation marks in the variable, for sure it will make the mysql query fail. But if you use the addslashes command (or remove quotation marks completley), it will solve that for you. However if addslashes is used, to get the quotation marks in their origional format you need to use stripslashes.
Any other questions.
That's why you should use mysql_real_escape_string instead of addslashes. You won't need to strip slashes when retrieving data from database.
==AND==
However if addslashes is used, to get the quotation marks in their origional format you need to use stripslashes.No you don't. The slashes simply escape data for use in the query, they are not stored in the database.
I did a few little tests with mysql query's and I found that the following mysql query inserts the data (not variable name) and does not need the use of the stripslashes function.
$var="aaa'aaa\"aaa'aaa"; $var=addslashes($var); mysql_query("INSERT INTO `table` SET `field`='$var'") or die(mysql_error());
So those in the above quotes of this post were right except the part about mysql_real_escape_string() which is optional if you are using addslashes(). However to use the addslashes function, unless you use it on the variables before starting the mysql query, the code will be as follows:
$query3 = mysql_query("INSERT INTO `pms` SET `from`='".addslashes($from)."', `to`='".addslashes($finalto)."', `subject`='".addslashes($subject)."', `message`='".addslashes($message)."', `time`='".addslashes($time)."'") or die(mysql_error());
And as I said in this post (and thorpe said earlier), you do not need to use stripslashes() at all. Also if you really wanted just the one set of double quotes for the mysql query, you could also use the following:
$from=addslashes($from); $finalto=addslashes($finalto); $subject=addslashes($subject); $message=addslashes($message); $time=addslashes($time); $query3 = mysql_query("INSERT INTO `pms` SET `from`='$from', `to`='$finalto', `subject`='$subject', `message`='$message', `time`='$time'") or die(mysql_error());
Hope that helps.
-
Your script was a bit hard to understand because of the use of php4 file read functions. But if you have php5 then the following should work:
$filedata=file_get_contents('names.txt'); $filedata.=' '; $tmp=explode(', ',$filedata); foreach($tmp AS $tmp) { if ($tmp==$user) { echo "FOUND"; $found=1; break; } } if ($found!==1) { echo "not found!"; } unset($tmp);
-
cwarn23: Could please explain, why are you stripping slashes from input variables, just to add them again?
There is a very good reason for this which must be known for every mysql query with insert/update data containg quotations. Below is an example of what you would have done to assign a variable to a column:
"`message`='$message'"
But there are 2 problems with that code. First is that in most cases, the string $message will be submitted to the database instead of what is inside the variable. To solve that you just place an exterior quotation then a dot to connect then the variable then another dot to reconnect then another exterior quotation. The exterior quotation is the quotation mark that surrounds the entire query.
"`message`='".$message."'"
That leaves us with the above code. Then there is your question about why use the addslashes function when you are going to remove them. That is because what if the variable contains a quotation mark? If there is a quotation mark in the variable it will escape one of the quotation marks in the mysql query making a fatal error. Below is a basic example of the error:
$message="asdf'asdf'asdfa'aja\"ads"; "`message`='".$message."'"
With those quotation marks in the variable, for sure it will make the mysql query fail. But if you use the addslashes command (or remove quotation marks completley), it will solve that for you. However if addslashes is used, to get the quotation marks in their origional format you need to use stripslashes.
Any other questions.
-
Does this include everything?
$query3 = mysql_query("INSERT INTO `pms` SET `from`='".addslashes($from)."', `to`='".addslashes($finalto)."', `subject`='".addslashes($subject)."', `message`='".addslashes($message)."', `time`='".addslashes($time)."'") or die(mysql_error());
Also when retrieving the values, say they were fetched from the database as an array called $row, you would need to do the following:
$row['from']=stripslashes($row['from']); $row['to']=stripslashes($row['to']); $row['subject']=stripslashes($row['subject']); $row['message']=stripslashes($row['message']); $row['time']=stripslashes($row['time']);
Hope that helps.
-
Still not working, thanks for the suggestion though errant.
The only other 2 things that I have experienced in the past are an invalid email address and incorrect php.ini configurations. Both of which you should be able to change with php code. So first is first, I see in your code a copy of the email is saved in the database. Varify that the mysql password update process took place during the loading of the page. If it did then you can eliminate to possibility of it being the email address which leaves one other option in my experience of the mail function. And that is to alter the ini directives that tell php what server processes the mail. You will want an external server such as gmail to process the mail for you since it seems your web host may not support email or haven't got php configured to their email client. Below is the code used to configure the email server and in the code they are set to their defaults:
ini_set('SMTP','localhost'); ini_set('smtp_port','25'); ini_set('sendmail_path','/usr/sbin/sendmail -t -i'); //this line may or may not work
Below is what you can try setting them to but am unsure weather it will work or not.
ini_set('SMTP','smpt.gmail.com'); ini_set('smtp_port','465');
Other than that, you may want to submit a support ticket to your web host.
-
I check your script and your syntax usage of the function curl_setopt() seems to be incorrect. Try the following and if it works, you can try uncommenting the commented line in the following:
<?php ini_set('display_errors', 1); error_reporting(E_ALL); $user = $_POST['username']; $pass = $_POST['password']; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,"WEBSITEURLGOESHERE?"); //curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS,"username=" . $user . "&password=" . $pass .); $pagedata = curl_exec($ch); curl_close($ch);
Hope that helps.
-
I have altered the script so that it will make the + symbol as 0.
<? $ValResult=0; $Valstring='hulu+'; preg_match_all('/^[a-zA-Z- .,]{2,200}$/', $Valstring, $matches); foreach($matches[0] as $value){ if(!in_array($value,array('','0','null','+'))){ $ValResult=1; } else{ $ValResult=0; } } return $ValResult; ?>
The way I altered the if function makes more sense.
-
I have tested your script and it works perfectly for me. Make sure that your $timeStart variable is not changed during the comment saying Hundreds of lines of code... Also try running the following as a test script and you will see what I meen:
//Top section of code $timeStart = microtime(true); sleep(1); $timeEnd = microtime(true); echo substr($timeEnd - $timeStart, 0, 6);
-
Hey All,
I was wondering if anyone knew a way to check if a submitted url is a domain or subdomain. For example possible entries are:
cname.somedomain.com
somedomain.com
cname.somedomain.on.ca
somedomain.on.ca
I have just read the question and I had already made a function that strips a url to the domain+subdomain so I have extended it to determine the real domain. My code is as follows:
<? function domain($domainb) { $bits = explode('/', $domainb); if ($bits[0]=='http:' || $bits[0]=='https:') { $domainb= $bits[2]; } else { $domainb= $bits[0]; } unset($bits); $bits = explode('.', $domainb); $idz=0; while (isset($bits[$idz])) { $idz+=1; } $idz-=3; $idy=0; while ($idy<$idz) { unset($bits[$idy]); $idy+=1; } $part=array(); foreach ($bits AS $bit) { $part[]=$bit; } unset($bit); unset($bits); unset($domainb); if (strlen($part[1])>3) { unset($part[0]); } foreach($part AS $bit) { $domainb.=$bit.'.'; } unset($bit); return preg_replace('/(.*)\./','$1',$domainb); } //Now to use the function echo domain('http://www.subdomain.google.com.au/'); ?>
And that is all it takes. Not much regex. Mainly the explode function does the job.
Enjoy.
-
hmm, i had a little gander, i would suggest making them sign in, if the can then its valid, if they cant then... well, they dont have a windows live id.
There is no way to tell wether the windows live id is in fact valid and working without attempting to use the service.
You found what you were looking for imho, you ust eed to find a way to implement it into your code (btw this code is written in OOP if you didnt know, if your code isnt, i would start now
).
Just as I feared because the msn address/live id field I am using in my form is an optional field and though there might be an easy way for 100% accuracy validation. So I guess I will need to do it the old fashion way and use the preg_match method.
BTW: I have been learning oop for php-gtk recently so using that msn download in my first post for other things on my website will be like a breeze as I should be able to isolate the oop programming to certain sections of the page by just calling objects. Or at least that is how I think it works. Thanks for the reply as there was no mention of it on the web.
-
I would use the ini_set command to set it to whatever timezone you have. For example, if your timezone is Antarctica/South_Pole then use the following:
ini_set('date.timezone','Antarctica/South_Pole');
A full list of timezones in catagories can be found at http://au.php.net/manual/en/timezones.php
Note: The links within the web address are not timezones. You need to click the links within that web address to get a full list of timezones for that area.
Hope that helps.
-
It is possible to make a link submit a Javascript variable to php at any time but for php to process it in the main page would require a page reload. So basically you setup a link to an invisible iframe and add to the iframe url variables. Then when the iframe loads, it gets the url variables and converts them to session variables. Then those session variables are available to any new page that loads. An example is as follows:
<? //index.php echo "<a href='iframe.php?myvar=test&var2=example' target='iframe'>test link.</a>"; echo "<iframe width=1 height=1 scrolling='no' name='iframe' frameborder=0 src='iframe.php'></iframe>"; ?>
<? //iframe.php session_start(); foreach ($_GET AS $key => $val) { $_SESSION[$key]=$val; } unset($key); unset($val); ?>
In the example above, as soon as the user clicks the link, the following variables will be set:
$_SESSION['myvar']=test
$_SESSION['var2']=example
So it is possible to make variables set at any time but it is just processing or displaying them which is the real trouble.
-
I have searched all over the web but cannot find a php script that will check if an email address is a valid Windows Live ID address (msn address). The only download that I have found that comes close is at http://www.microsoft.com/downloads/details.aspx?FamilyId=E565FC92-D5F6-4F5F-8713-4DD1C90DE19F&displaylang=en#filelist but can't find any direct function within that script to detect weather the email address is a valid Windows Live ID. Does anybody know where I can find documentation or scripts for to check if an email address is a valid Windows Live ID?
Thanks
-
Dont forget http://www.jumba.com.au/hosting/personal - They have a wide range of plans for both personal, business and virtual private servers. Also to search for australian hosts by stastics you can use the website at http://www.webhostingchoice.com.au/
Enjoy.
-
Try the below code:
<?php $thiz = array(); $this_array = array(); $this_array[] = "value1"; $this_array[] = "value2"; foreach ($this_array as $compare){ $thiz[] = "testvalue"; } print_r($thiz); ?>
The reason why you couldn't retrieve the values from what I can see is that functions cannot call variable/array values from outside the function. So if you have a variable and you want to pass it to a function, you can 1, use the function brackets or 2, use sessions. But the easiest thing to do in your case was just to remove the entire function since it did not contain much.
Hope that helps.
-
Well I have tested the script again and for me it works. So as Daniel said it could be just that you haven't got curl but then again you should get a fatal error if that is the case. So try setting up a page with the following test script to test your curl version to see what you can see. With the below script your should be able to see the google translator homepage and your curl version information at the bottom. If you can't see that then it is not the script that is failing but rather the php.ini extensions configuration that is failing. Also be sure that you have php version 5 as the file_get_contents function is only available in php5.
<? $curl_handle=curl_init('http://translate.google.com/translate_t'); curl_setopt($curl_handle, CURLOPT_HEADER, false); curl_setopt($curl_handle, CURLOPT_FAILONERROR, true); curl_setopt($curl_handle, CURLOPT_HTTPHEADER, Array("User-Agent: Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.15) Gecko/20080623 Firefox/2.0.0.15") ); // request as if Firefox curl_setopt($curl_handle, CURLOPT_POST, false); curl_setopt($curl_handle, CURLOPT_NOBODY, false); curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,false); curl_setopt($curl_handle,CURLOPT_RETURNTRANSFER,1); $buffer = curl_exec($curl_handle); curl_close($curl_handle); echo $buffer."<xmp>"; var_dump(curl_version()); echo "</xmp>"; ?>
Let me know of the results of the above test script.
-
In your first code box check this line:
<form action="test.php" method="POST">
Is test.php the filename of the processing page or is it processtest.php. If I am not reading your question wrong then it should be as follows:
<form action="processtest.php" method="POST">
-
Google has a full API for their translator.
You can find it here:
http://code.google.com/apis/ajaxlanguage/
That is an ajax api and if you wanted to use the translation for server-side processing then using that ajax method, you would need to do something like write the translation to a mysql database for the server to read. Also what if a user hasn't got ajax/java enabled, then they wouldn't be able to view the translation. So that is why I am making a php (server side) script that will process that data for the user. I have been working hard on this and I manage to make a script that will automatically detect the hidden fields and include them into the server side post. So my new script (hopefully will last longer) is as follows:
<?php function translate($sentence,$languagefrom,$languageto) { $homepage = file_get_contents('http://translate.google.com/translate_t'); if ($homepage==false) {$homepage='';} preg_match_all("/<form[^>]+ction=\"\/translate_t\".*<\/form>/",$homepage,$botmatch); $botmatch[0][0]=preg_replace("/<\/form>.*/",'',$botmatch[0][0]); preg_match_all("/<input[^>]+>/",$botmatch[0][0],$botinput); $id=0; while (isset($botinput[0][$id])) { preg_match_all("/value=(\"|'|[^\"'])[^\"']+(\"|'|[^\"'])?( |>| )/",$botinput[0][$id],$tmp); $tmp[0][0]=preg_replace('/((\'|")?[^\'"]+)/','$1',$tmp[0][0]); $tmp[0][0]=preg_replace('/(\'|")/','',$tmp[0][0]); $tmp[0][0]=preg_replace('/.*value=/i','',$tmp[0][0]); $len=strlen($tmp[0][0]); $len-=1; $tmp[0][0]=substr($tmp[0][0],0,$len); preg_match_all("/name=(\"|'|[^\"'])[^\"']+(\"|'|[^\"'])?( |>| )/",$botinput[0][$id],$tmpname); $tmpname[0][0]=preg_replace('/((\'|")?[^\'"]+)/','$1',$tmpname[0][0]); $tmpname[0][0]=preg_replace('/(\'|")/','',$tmpname[0][0]); $tmpname[0][0]=preg_replace('/.*name=/i','',$tmpname[0][0]); $len=strlen($tmpname[0][0]); $len-=1; $tmpname[0][0]=substr($tmpname[0][0],0,$len); if (strlen($tmpname[0][0])>0 && !in_array($tmpname[0][0],array('text','sl','tl'))) { $vars.=$tmpname[0][0]."=".$tmp[0][0].'&'; } unset($tmp); unset($tmpname); $id+=1; } $curl_handle=curl_init('http://translate.google.com/translate_t'); curl_setopt($curl_handle, CURLOPT_HEADER, true); curl_setopt($curl_handle, CURLOPT_FAILONERROR, true); curl_setopt($curl_handle, CURLOPT_HTTPHEADER, Array("User-Agent: Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.15) Gecko/20080623 Firefox/2.0.0.15") ); // request as if Firefox curl_setopt($curl_handle, CURLOPT_POST, true); curl_setopt($curl_handle, CURLOPT_POSTFIELDS, $vars.'text='.$sentence.'&sl='.$languagefrom.'&tl='.$languageto); curl_setopt($curl_handle, CURLOPT_NOBODY, false); curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,false); curl_setopt($curl_handle,CURLOPT_RETURNTRANSFER,1); $buffer = curl_exec($curl_handle); curl_close($curl_handle); $buffer=strip_tags($buffer,'<div>'); preg_match_all("/\<div id\=result_box dir\=\"[^\"]+\"\>[^<]+\<\/div\>/",$buffer,$match); $match[0][0]=strip_tags($match[0][0]); return $match[0][0]; } echo translate('This is the text','en','iw'); ?>
Good luck. And just let me know if it stops working because I would like to use the same script too.
sha1 to text
in PHP Coding Help
Posted
And I have created a script that just does that. If you have 2 Petabytes (or 2147483648 Megabytes which is 2048 Terabytes) then you should be able to dehash and hash contain data up to 30 or 40 digits. So first you will need to create a mysql database with a table called 'dehasher' (without the quotes) and inside that table you need 2 text columns name 'word' and 'hash' (without the quotes). After creating that mysql database, create a file named db.php and place in it the following contents:
And of course you will need to configure the above variables to your mysql database. Then below is index.php
Then create a file named generator.php with the following contents and this file will generate the database contents for the dehashing.
Now that is the script, next is the use the dehasher. If you intend to use the whole 2 Petabytes then setup a cron job to run generator.php or if you only want to use a portion of that space then just run the generator.php file in the browser and monitor the space used in phpmyadmin table structure tab. After you have generated the database then load index.php and enter a hash and it will dehash it. But for best results you will need to use the full 2 Petabytes.
So it is possible to dehash sha1 and the only hash I have come accross that this method will not work on is whirlpool. That is why I recommend the following method of hashing:
Hope that script helps with your dehashing if you have 2 Petabytes of disk space.