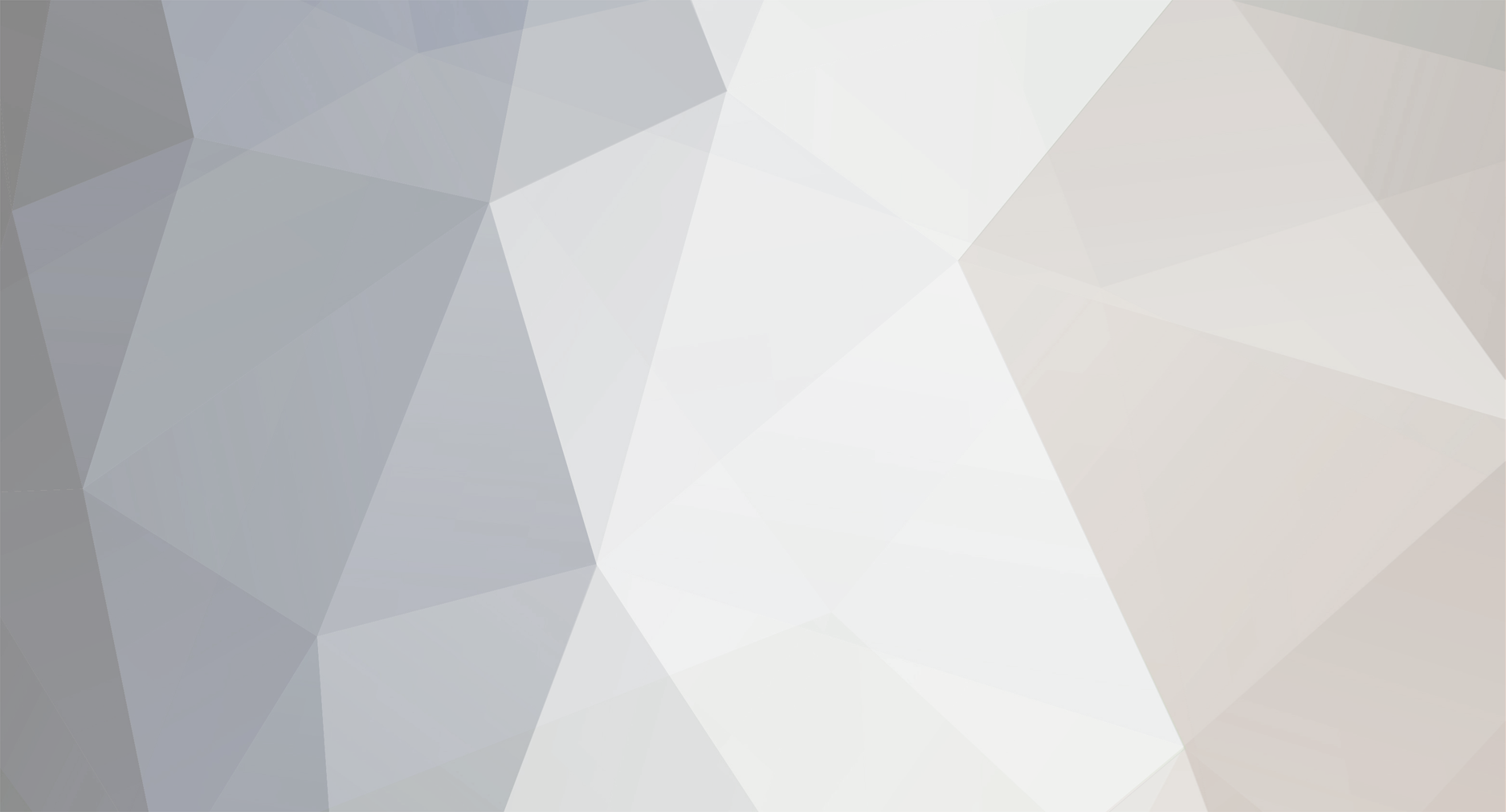
dadamssg
Members-
Posts
729 -
Joined
-
Last visited
Never
Everything posted by dadamssg
-
they're user uploaded photos and will be contacting each user to ask for their permission to use the photos.
-
I'm trying to scrape images from the mark-up of certain webpages. These webpages all have a slideshow. Their sources are contained in javascript objects on the page. I'm thinking i need to get_file_contents("http://www.example.com/page/1"); and then have a preg_match_all() function that i can input a phrase(ie. '"LargeUrl": ', or '"Description":') and get whatever's in the quotes directly after those instances. var photos = {}; photos['photo-391094'] = {"LargeUrl": "http://www.example.org/images/1.png","Description":"blah blah balh"}; photos['photo-391095'] = {"LargeUrl": "http://www.example.org/images/2.png","Description":"blah blah balh"}; photos['photo-391096'] = {"LargeUrl": "http://www.example.org/images/3.png","Description":"blah blah balh"}; I have this function, but it returns the entire line after the input phrase. How can i modify it to look for whatever's in quotes directly after the input keyword? Or am i doing it all wrong and theres an easier way? $page = file_get_contents("http://www.example.org/page/1"); $word = "\"LargeUrl\":"; if(preg_match_all("/(?<=$word)\S+/i", $page, $matches)) { echo "<pre>"; print_r($matches); echo "</pre>"; }
-
awesome. thanks. Here's my function function get_phrase_after_string($haystack,$needle) { //length of needle $len = strlen($needle); //matches $needle until hits a \n or \r if(preg_match("#$needle([^\r\n]+)#i", $haystack, $match)) { //length of matched text $rsp = strlen($match[0]); //determine what to remove $back = $rsp - $len; return trim(substr($match[0],- $back)); } }
-
thanks for the that, xyph. I did the following but it is returning "Property: " with it. Anyway to cut that out? $body = "Dear John Doe, your information is below Check in date: 07/23/2012 Check out date: 07/26/2012 Property: The best inn around \n Alternate Check in date: 07/27/2012 Alternate Check out date: 07/29/2012"; echo "$body<br/><br/>"; if(preg_match('/(?<=Check in date: )\S+/i', $body, $match)) { echo $match[0]."<br/><br/>"; //returns '07/23/2012' } if(preg_match('#Property: [^\r\n]+#i', $body, $match)) { echo $match[0]; //returns 'Property: The best inn around' }
-
I have this function to search for a 'word'(string with white spaces around it) after a specified string and it works great. I need to modify it to where it looks for all characters after the specified string until it hits a new line. The following returns "07/23/2012". But i would like to do is modify it so if i input "Property: " into the preg_match function it returns "The best resort in town". I suck at regex though. Any help would be much appreciated. thanks! $body = "Dear John Doe, your information is below Property: The best resort in town Check in date: 07/23/2012 Check out date: 07/26/2012 Alternate Check in date: 07/27/2012 Alternate Check out date: 07/29/2012"; if(preg_match('/(?<=Check in date: )\S+/i', $body, $match)) { echo $match[0]; }
-
so i've been developing in the CodeIgniter framework and i have yet to code an object. My app deals with bookings. So in the database i have columns like: start, end, created, created_by, title, description, etc. I pull the data out and put it into an array. I use my controller functions to validate data and model functions to delete/edit/create the bookings. Should i write a class that creates a Booking object? i don't see how that would benefit me though. How do you guys determine what needs to be an object?
-
perfect. thanks!
-
I found this preg_match() function which searches the parts of a string and uses the checkdate() function to see if it is a date. It expects the format of the date to be "YYYY-MM-DD". I would like to rewrite it to expect the format to be "MM/DD/YYYY". <?php function checkDateFormat($date) { //match the format of the date if (preg_match ("/^([0-9]{4})-([0-9]{2})-([0-9]{2})$/", $date, $parts)) { //check weather the date is valid of not if(checkdate($parts[2],$parts[3],$parts[1])) return true; else return false; } else return false; } I'm pretty sure the following is write to change the string length of each part but i don't know how to change the expected "-"'s to be a "/"'s. <?php if (preg_match ("/^([0-9]{2})-([0-9]{2})-([0-9]{4})$/", $date, $parts)) Any help would be greatly appreciated!
-
perfect @barand. exactly what i needed. thanks!
-
Say i have two arrays with custom keys. What would be the easiest way to write a function that would determine if all the keys in arrayOne are in arrayTwo? The example below would return false because keys 3 and 4 are missing in arrayTwo. Any help would be much appreciate. thanks! <?php $arrayOne['1'] = "adg"; $arrayOne['2'] = "a4g"; $arrayOne['3'] = "346"; $arrayOne['4'] = "etwe"; $arrayTwo['1'] = "xcb"; $arrayTwo['2'] = "acbr"; $arrayTwo['6'] = "yiy"; $arrayTwo['7'] = "mmm"; ?>
-
this is great. Thank you so much! I'm in Texas as well!
-
I'm trying to figure out how i should be storing itemized order information. My orders require a down payment and has multiple taxes, each with their own rate. What should my database look like? I know i should be storing the base price. Then, should i store the tax rates for each order or tax amounts with each item in a row? Then for the transactions(deposit and remainder)...should i store the exact amount of the base prices and taxes each transaction covers? or just the total amount of the transaction to keep it simple? I'd like to be able to edit as much information as possible if need be, base price, tax rates/amounts, etc. And i'd also like to generate reports on the data(how much of product be was sold, the tax amounts owed, average price each product sold for, etc.) How should i organize all this in my database?
-
I have a nested array. I'm trying to write code to produce the minimum value foreach first key of the $test array. So, ideally it would produce what's in the $result array. I don't know why i can't figure out how to do this. The multiple nests are throwing me off. I think i'm over thinking this. <?php $test['abcd']['0'] = array('10','100','300'); $test['abcd']['1'] = array('1000','100','3000'); $test['abcd']['3'] = array('23','89','700'); $test['efgh']['0'] = array('3','55','300'); $test['efgh']['1'] = array('160','160','9000'); $test['efgh']['3'] = array('900','89','200'); //$result['abcd'] = 10; //$result['efgh'] = 3; ?>
-
What's the proper way to check if a file exists on a remote server? I know file_exists() works for you local files. I think i need to use something like the following. BUT if the file is not found PHP throws a warning. I know i can put a '@' before fopen() but that just masks the error. <?php if(fopen("http://www.othersite.com/imageXYZ.jpg", "r")) { echo "File exists."; } else { echo "File does not exist."; } ?>
-
Please help me oh yee magical programming masters :)
dadamssg replied to kac001's topic in PHP Coding Help
i would change the very first part to this...for sql injection reasons if (!$_GET['pid']){ $pageid = '1'; } else{ $pageid = (int) $_GET['pid']; if($pageid == 0) { $pageid = "1"; } } So if they do write letters/words in that parameter this would convert it to 0 and then set it to 1 -
to get the value in the php script it would be $_POST['userGroup']. And depending on which button they selected you would get whatever $group1, $group2, $group3, $group4, $group5, or $group6 is.
-
shouldn't all the names of the radio buttons be the same? <input type="radio" name="userGroup" value="<?php echo $group1; ?>" /><?php echo $group1; ?> <input type="radio" name="userGroup" value="<?php echo $group2; ?>" /><?php echo $group2; ?> <input type="radio" name="userGroup" value="<?php echo $group3; ?>" /><?php echo $group3; ?> ..and so forth
-
perfect. Thanks!
-
Isn't there a way to get the last record entered into a table? I have a blog post that i enter into a table and then once that happens i want to redirect them to that blog but i need to get the id of that entry. Is it necessary for me to enter a datetime field in the table to do this?
-
yes...like every wednesday or the 1st of every month or the first friday of every month...along those lines.
-
I have a database table that has events(title, description, location, start and end datetimes) stored to display in a calendar. I want the ability for a user to specify an event that reoccurs. I'm trying to wrap my head around the best way to achieve this is. My PHP script cycles through the days of a month to produce the table cells for the month and then queries the database if the day it's cycling through has an event. I'm not sure how i should put the reoccurring events in the table. I figure i need to add some other fields to the table to capture the reoccurrence pattern the user specifies. ANOTHER thing i'm trying to figure out is the fact that if one of those reoccurring events needs to be changed(like the location). How the heck i should arrange that. Anyone have any suggestions on how i should set up my table and query the reoccurring events? This is the query i have that pulls up the events for each day. $quer = "SELECT * FROM Events WHERE '$day' BETWEEN date(start) AND date(end) AND username = '$username' ORDER BY start ASC";
-
does anyone know of a decent color picker? I've come across the YUI color picker and the color square from Colorjack.com. Both require quite a bit of customization for i what i want to do. What i want to do: display a text input when clicked pulls up a color picker. The selection updates the input with the hex value and closes the color picker. Then i can write a javascript function to grab the value of the input and do something with it.
-
change the source of the javascript function?
dadamssg replied to dadamssg's topic in Javascript Help
hey thanks...i figured out a way to do what i want. I have a javascript that has a php file as its source to spit out javascript. Then i have a function in the head that updates the div with a php script that spits out html. <html> <head> <script src="http://code.jquery.com/jquery-1.4.4.js"></script> <style type="text/css"> body{ background: url(http://localhost:8888/css/lightgreenstripe.png); } </style> <script type="text/javascript"> function ChangeMonth(X,Y){ var changemonthlink = "http://localhost:8888/calendars/calendar_html.php?m=" + X + "&y=" + Y; $("#relEVENTz").load(changemonthlink); }; </script> <link type="text/css" href="http://localhost:8888/css/dynamic.php?color=blue" rel="stylesheet" /> </head> <body> <br> <div id="relEVENTz"> <script type="text/javascript" src="http://localhost:8888/calendars/calendar_javascript.php?m=11&y=2010"></script> </div> </body> </html> -
I have a color picker on my page that puts the hex value in an input box. I'm not familiar with jquery or javascript in general. I would like to be able to take the hex value and use it in updating a style of a div...say the border color for example. Something like this...but this isn't working.... any help would be awesome! <input type="text" id="colorfield1" onFocus="ddcolorposter.echocolor(this, 'colorbox1')"> <div id="colorbox1" class="colorbox"></div> <br><br> <div id="testbox"> Some Text </div> <script> $("#colorfield1").keyup(function () { var value = $(this).val(); $("#testbox").style.border="1px solid "+ value; }).keyup(); </script>
-
Ok..this is kind of complicated. I wrote a php script that spits out a calendar in javascript. I use it as the source of a javascript function. heres the javascript code that will be placed in a html webpage <script type="text/javascript" src="http://localhost:8888/calendars/calendar_javascript.php?m=11&y=2010"></script> as you can see the script uses the $_GET['m'] and $_GET['y'] to determine which month to display. Again it all works but i want to have the script produce Next and Previous month links. and then when clicked it would change the source of the script to have different m & y values. I kinda doubt this is possible but can anyone recommend a way to achieve this?