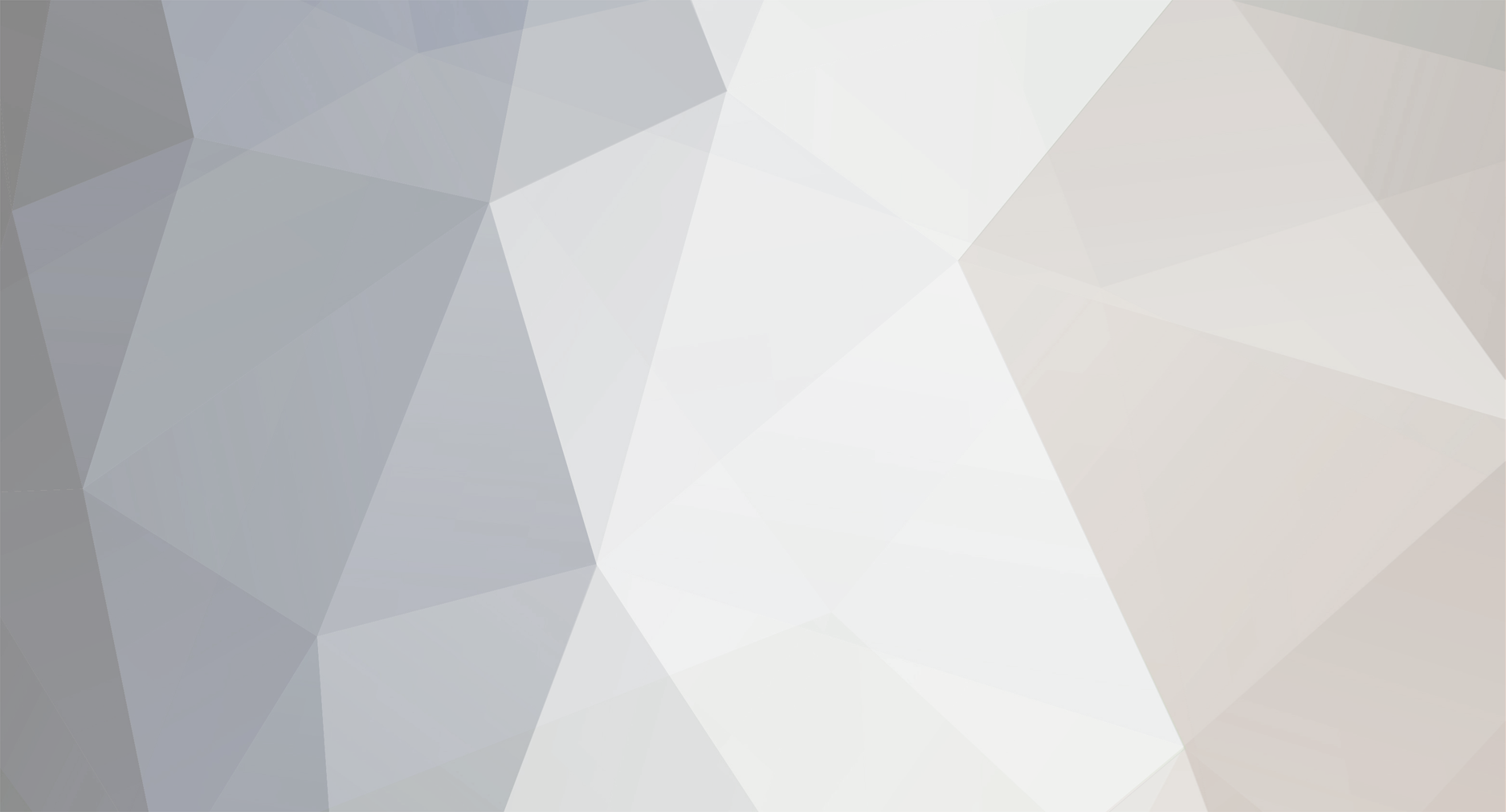
Reaper0167
-
Posts
270 -
Joined
-
Last visited
Never
Posts posted by Reaper0167
-
-
i don't mind if the page reloads itself... as long as it is still the same page name in the url.
-
lets say i have a div with a couple of text fields on my site. after i click on a button,,,, could i have something different display inside that same div instead of the text fields? Can this be done with only using php?
-
One thing that really drives me crazy..... Missing little things like that I know...But I'm still a newb...
Thank You!!!
-
a member helped me out with displaying errors using $_SESSION['message']
on my login page everything works great... if the username is not registered an error displays on screen saying that it is not registered... But on my registration page when i user tries to register a name that is alreay registered, my error doesn't show up on that page. But it will display on my login page if i hit the back button on my browser. here is the code.
this is from the register script <?php if($count > 0) { session_start(); $_SESSION['message'] = "This username is already registered. Please try again."; header("location: http://www.---------.com/registration.php"); exit(); } ?>
this is on my registration.php page where the form is <?php <?php if(isset($_SESSION['message'])){echo $_SESSION['message'];} ?> ?>
i have the exact same setup on my login page and all that works just fine....Am I only able to use a certain number of the echo $_SESSION['message'] statements or is there another problem. i am using header("location: http://www.-------.com/registration.php"); so shouldn't that be the one and only place it should display?
-
thank you,, i fixed it
-
oh sorry....when i try to register a username and/or email that already exists in the database, it lets me register that name.. i would like it to give me an error saying that it already exists.
-
here is my reg script...every works good except for giving an error if the username or email already exists. i can't seem to find the problem.
<?php // connection to your database include ("connection.php"); // connects to server and database mysql_connect("$host", "$username", "$password") or die("Could not connect."); mysql_select_db("$db_name") or die("Could not find database"); // define variables from form register form $username = mysql_real_escape_string($_POST["username"]); $password = md5($_POST["password"]); $email = mysql_real_escape_string($_POST["email"]); // check if username/email is already registered, if so, give error message $sql="SELECT * FROM $tbl_name WHERE username = '$username' and email = '$email' LIMIT 1"; $result=mysql_query($sql); $count=mysql_num_rows($result); if($count == 1) { session_start(); $_SESSION['message'] = "The username and/or password is also registered. Please try again."; header("location: registration.php"); } // insert user into database $sql = "INSERT INTO $tbl_name(username, password, email)VALUES('$username','$password','$email')"; mysql_query($sql) or die(mysql_error()); // direct user to login page with a succuess message session_start(); $_SESSION['message'] = "Thank you for registering $username. You may now log in."; header("location: http://www.------------.com"); // closes your connection mysql_close(); ?>
-
Alright,, the error messages now display properly... Thank you everyone... Here is what ended up working for me..
<?php //login if($count==1) { session_start(); $_SESSION['auth'] = "yes"; $_SESSION['message'] = "Welcome ".$username; header("location: http://www.-------------.com/home.php"); } else { session_start(); $_SESSION['message'] = $username." is not a registered username."; header("location: http://www.-----------.com"); session_destroy(); } ?> Would something like session_unset['message'] on refresh work?
and then the code that goes where you want the message to appear.
<?php if(isset($_SESSION['message'])){echo $_SESSION['message'];} ?>
Everything works good except one small thing..... If someone enters an invalid username the message appears on the page,, which is good... When I hit the refresh button on my browser the message stays there... What should I do to get rid of the session error message when the browser is refreshed????
-
ok,,, so should this...
<?php echo $_SESSION['message'];?>
still go on my pages where i want the message to appear?
I'll try it.
-
Nevermind,,, now I am confused. This is what I thought would work.
<?php // display log in error or success if($count==1) { session_start(); $_SESSION['auth'] = "yes"; header("location: home.php"); $_SESSION['success'] = "Welcome $username"; } else { session_start(); header("location: index.php"); $_SESSION['error'] = "$username is not a registered username."; } ?>
and then for my success or error message
<?php <?php echo $_SESSION['success'];?> //this is located on the members page <?php echo $_SESSION['error'];?> //located on the page with the form ?>
-
Well,,, were getting there... If the name entered is not valid then the $_SESSION['error'] is displayed properly... as for when the name entered and it is a valid username... I am using $_SESSION['auth'] to check that the user is logged in so they can go to the members only page.... So for the success message, what should i use instead of $_SESSION.
-
i don't know a whole of php stuff like lots of others on this site...but one thing i do is set up my code this way, so i can easily spot things out like that. just trying to help
<?php $title = $_POST['title']; if (!file_exists($title)) { echo 'The file you tried to edit does not exist!'; } else { $fp = fopen($title,"a+"); } if(!$fp) { echo 'Error, the file could not be opened or there was an error when creating it.'; } ?>
-
Well, something went wrong with my script and I am back to the same problem as before. My error message for my login displays on the same page as my form. Which is good,,, but it also throws the error message up in the url box on my browser. the code for my login script is below.
<?php[code] // display log in error or success if($count==1) { session_start(); $_SESSION['auth'] = "yes"; $message = "Welcome $username"; header("location: home.php?error=" . urlencode($message)); } else { $message = "$username is not a registered username."; header("location: index.php?error=" . urlencode($message)); } ?>
here is the code that echos the error message
<? echo $_GET['error'];?>
and this is what is in my url box on my browser.
http://----------.com/index.php?error=fdsaf+is+not+a+registered+username.
i guess my question is,, why is it displaying this in the url box of my browser?
-
This is what I have for checking that the fields have atleast something filled in.
<script type="text/javascript" language="javascript"> function validate(form2) { var valid = true; if (form2.username.value) { document.getElementById('username_error').innerHTML = ''; } else { document.getElementById('username_error').innerHTML = 'Please enter a username.'; valid = false; } return valid; } </script>
then next to my textfield is where my error message comes up.. here is the code for that..
<span id="username_error" class="error">
How would I incorporate that method for checking that both fields are the same.
-
i have two text fields... password and password confirmation..... i know how to use php to check that both fields are the same and display the error.... i also know that this thread is not posted in the right section but the javascript section seems,, well,,, it just seems like no one is ever there..... question is what is a good way to to check two fields are the same using javascript... i can use javascript to make sure that the fields have something in it...... but i'm a little confused on the fields being the same.
-
with the way that it is set up right now,,,, even if all the fields are filled in and the passwords do not match,, it will not register them until the passwords are the same..... like i was saying at the beginning of this thread,,, all i have to do is take out the md5 and the "()" in that line of code and everything works fine... so somewhere in both scripts with the md5 is where it is going wrong..Could there be something wrong with the way i have my database setup??? i don't think there is but it is worth asking.
-
What could be wrong here???? This is what i got. md5 is put before both $_POST....
register script
<?php //connection to your database include ("connection.php"); // connects to server and database mysql_connect("$host", "$username", "$password") or die("Could not connect."); mysql_select_db("$db_name") or die("Could not find database"); // define variables from form register form $username = mysql_real_escape_string($_POST["username"]); $password = md5($_POST["password"]); $email = mysql_real_escape_string($_POST["email"]); // inserting data into your database $sql = "INSERT INTO $tbl_name(username, password, email)VALUES('$username','$password','$email')"; $res = mysql_query($sql) or die(mysql_error()); if ($_POST['password'] != $_POST['password_conf']) { $message = "Passwords must be the same. Please try again."; header("location: registration.php?error=" . urlencode($message)); } // closes your connection mysql_close(); ?>
login script
<?php // datbase information include "connection.php"; // connects to server and database mysql_connect("$host", "$username", "$password") or die("Could not connect."); mysql_select_db("$db_name") or die("Could not find database"); // pull username and password from the form $username = mysql_real_escape_string($_POST['username']); $password = md5($_POST['password']); // searching for username and md5password in database $sql="SELECT * FROM $tbl_name WHERE username ='$username' and password = '$password' LIMIT 1"; $result=mysql_query($sql); $count=mysql_num_rows($result); // display log in error or success if($count==1) { session_start(); $_SESSION['auth'] = "yes"; $message = "Welcome $username. You are now logged in."; header("location: home.php?error=" . urlencode($message)); } else { $message = "$username is not a registered username. Please register first."; header("location: index.php?error=" . urlencode($message)); } ?>
-
i went in a registered new usernames and passwords and still can not log in...
i am now thinking that the method i used for registering and loggin in a md5 password is not correct...
-
now i am baffled... not but what everyone here is saying about security stuff... but the fact that my login worked just fine a few minutes ago... but now it will not work,,,,AGAIN..... i don't understand what is going on... i did not change anything.
-
cool, thanks for the info
so is this an ok method for keeping people out of member only pages???
-
first off,,, sorry about all the questions... but in my login script i have
<?php if($count==1) { session_start(); $_SESSION['auth'] = "yes"; $message = "Welcome $username. You are now logged in."; header("location: home.php?error=" . urlencode($message)); } ?>
and on my members page i have this
<?php session_start(); //Checking if user is logged in. If not, re-direct them to login form. if (!isset($_SESSION['auth'])) { header("Location: index.php"); } ?>
I did accomplish what i was trying to do. I wanted only a logged in user to be able to access my members page. If your logged in and and type the address in you can access the page. But if you close your browser and type in the address it takes you directly back to the login/register page... Which is great. Works perfect for me...Here is my question,,,,, i am setting my session auth to yes... so how is the !isset working with session auth set to yes... Basically could someone explain how the !isset is talking to the session being set to yes..... The way i see it,,, i would do something like this...
if $_SESSION['auth'] != "yes"
{
header ("Location: index.php");
}
How does that !isset work with a yes and a no like i have here????
Hopefully someone understands.
-
i set max characters to 50 and everything works now... thanks.... now what else could i do to make the names and passwords more secure???
-
if i take out the md5 in the reg and log everything works fine.. just when i insert the md5 it registers as a md5password but the login is telling me that i am not registered.
-
it registers the username and the md5 password just fine... i checked my database... but when i go to log in,,, it keeps telling me that i am not registered......how much further could i go with security past md5 once i get the md5 to work?
upload script question
in PHP Coding Help
Posted
i found this basic upload script. it works, very simple. but when the picture is uploaded to mysql,,, it creates its own row,, a new row... how would i have it upload to the row of whoever is signed in at that time? here is the simple little script i found online. also,,, what is the "rb" in the line below??
better yet,,, instead of uploading the image in the same row of the user that is signed in. Would it be better to create a new table for all the images and then somehow link images to users that upload them.. Can i do that???