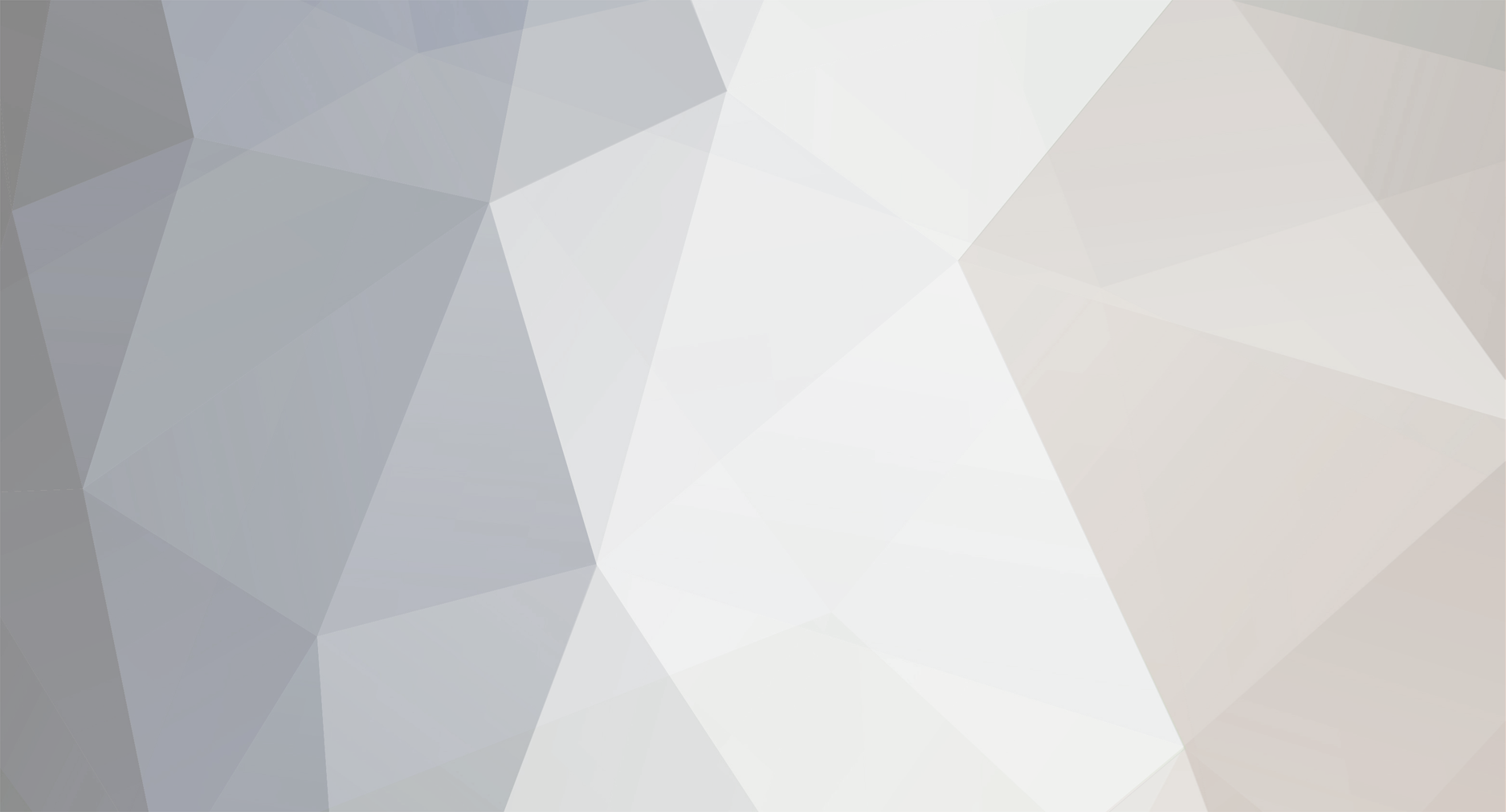
meomike2000
-
Posts
171 -
Joined
-
Last visited
Never
Posts posted by meomike2000
-
-
yes u can, here is an example of a test script that i have written that uses validation:
<html> <head> <script language="javascript" type="text/javascript"> <!-- //Browser Support Code function ajaxFunction(){ var ajaxRequest; // The variable that makes Ajax possible! try{ // Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e){ // Internet Explorer Browsers try{ ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try{ ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ // Something went wrong alert("Your browser broke!"); return false; } } } // Create a function that will receive data sent from the server ajaxRequest.onreadystatechange = function(){ if(ajaxRequest.readyState == 4){ document.myForm.city.value = ajaxRequest.responseText; } } ajaxRequest.open("GET", "secondchoice.php", true); ajaxRequest.send(null); } //--> </script> <script type="text/javascript" src="js/ajax.js"></script> <script type="text/javascript"> var ajax = new Array(); //this will refresh citys function getCityList(sel) { var stateCode = sel.options[sel.selectedIndex].value; document.getElementById('city').options.length = 0; // Empty city select box if(stateCode.length>0){ var index = ajax.length; ajax[index] = new sack(); ajax[index].requestFile = 'mygetcity.php?stateCode='+stateCode; // Specifying which file to get ajax[index].onCompletion = function(){ createCities(index) }; // Specify function that will be executed after file has been found ajax[index].runAJAX(); // Execute AJAX function } } function createCities(index) { var obj = document.getElementById('city'); eval(ajax[index].response); // Executing the response from Ajax as Javascript code } //this will get subcatagory function getSubCategoryList(sel) { var category = sel.options[sel.selectedIndex].value; document.getElementById('subcat').options.length = 0; // Empty subcat select box if(category.length>0){ var index = ajax.length; ajax[index] = new sack(); ajax[index].requestFile = 'mygetsubcat.php?category='+category; // Specifying which file to get ajax[index].onCompletion = function(){ createSubCategories(index) }; // Specify function that will be executed after file has been found ajax[index].runAJAX(); // Execute AJAX function } } function createSubCategories(index) { var obj = document.getElementById('subcat'); eval(ajax[index].response); // Executing the response from Ajax as Javascript code } </script> <title>test</title> <link rel="stylesheet" type="text/css" href="../web1.css" /> <link rel="stylesheet" type="text/css" href="../heading.css" /> <link rel="stylesheet" type="text/css" href="../navigation.css" /> <link rel="stylesheet" type="text/css" href="directory.css" /> </head> <body> <div class="navigation" id="navigation"> <a class="navjust" href="../index.php">Home</a><br /> <br /> <a class="navjust" href="http://mail.meo2000.net">Check Mail</a><br /> <br /> <a class="navjust" href="../web/webservice.php">Web Services</a><br /> </div> <div id="justify"> <div id="h"> <h1>meo2000 listing service</h1> <h3>want to get listed, click <a href="getlisted.htm">here</a> to find out how.</h3> <h3>This directory is under construction!</h3> <br/> </div> </div> <?php Function DisplayForm($errors = null){ $error_string = $errors; //state, you could use $error_string = implode ("<br />",$errors); if you want to save performance by using an array insead. echo(' <div id="left"> <span class="error"> '.$error_string.'<br /> </span> <form method="post" action="'.$_SERVER['PHP_SELF'].'"> State: <select id="state" name="state" onchange="getCityList(this)"> <option value="">Select a state</option> <option value="Alabama">Alabama</option> <option value="North Carolina">North Carolina</option> <option value="Texas">Texas</option> </select><br /> <br /> City: <select id="city" name="city"> </select><br /> <br /> Category: <select id="category" name="category" onchange="getSubCategoryList(this)"> <option value="">Select a catagory</option> <option value="Auto">Auto</option> <option value="Hotel">Hotel</option> <option value="Restaurant">Restaurant</option> </select><br /> <br /> SubCategory: <select id="subcat" name="subcat"> </select><br /> <br /> <input type="submit" name="submit" value="Select"> </form> </div> '); } //check if form has been submitted if (!$_POST['submit']) { //if not display form DisplayForm(); } else { //form has been submitted //items selected, check that number was entered, //Declare it so we dont get notices 'undeclared variable' $error = null; //check state has been selected if (trim($_POST['state']) == "") { $error .='ERROR: Please select a state.'; } //check category has been selected elseif (trim($_POST['category']) == "") { $error .='ERROR: Please select a category.'; } //if there was an error, show it. if ($error != null){ DisplayForm($error); } else { //otherwise carry on? echo '<div id="right">'; $state = $_POST['state']; $city = $_POST['city']; $cat = $_POST['category']; $subcat = $_POST['subcat']; echo 'Here is your selections: <br />'; echo "<i>$state</i><br />"; echo "<i>$city</i><br />"; echo "<i>$cat</i><br />"; echo "<i>$subcat</i><br />"; echo '</div>'; } } ?> <div class="addspace" id="addspace"> <p class="none" id="addmargin"> your<br > add<br > could<br > be<br > filling<br > this<br > spot! </p> </div> </body> </html>
-
As I am still fairly new at scripting with php, and have never used sessions before, I could use some help.
What I have is created a form, and would like to add sessions so that if there is an error in the user filling out the form, when the form reloads and displays the error, they do not have to choose all options again....
here is the options form that i have created, this is just a test form:
<html> <head> <script language="javascript" type="text/javascript"> <!-- //Browser Support Code function ajaxFunction(){ var ajaxRequest; // The variable that makes Ajax possible! try{ // Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e){ // Internet Explorer Browsers try{ ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try{ ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e){ // Something went wrong alert("Your browser broke!"); return false; } } } // Create a function that will receive data sent from the server ajaxRequest.onreadystatechange = function(){ if(ajaxRequest.readyState == 4){ document.myForm.city.value = ajaxRequest.responseText; } } ajaxRequest.open("GET", "secondchoice.php", true); ajaxRequest.send(null); } //--> </script> <script type="text/javascript" src="js/ajax.js"></script> <script type="text/javascript"> var ajax = new Array(); //this will refresh citys function getCityList(sel) { var stateCode = sel.options[sel.selectedIndex].value; document.getElementById('city').options.length = 0; // Empty city select box if(stateCode.length>0){ var index = ajax.length; ajax[index] = new sack(); ajax[index].requestFile = 'mygetcity.php?stateCode='+stateCode; // Specifying which file to get ajax[index].onCompletion = function(){ createCities(index) }; // Specify function that will be executed after file has been found ajax[index].runAJAX(); // Execute AJAX function } } function createCities(index) { var obj = document.getElementById('city'); eval(ajax[index].response); // Executing the response from Ajax as Javascript code } //this will get subcatagory function getSubCategoryList(sel) { var category = sel.options[sel.selectedIndex].value; document.getElementById('subcat').options.length = 0; // Empty subcat select box if(category.length>0){ var index = ajax.length; ajax[index] = new sack(); ajax[index].requestFile = 'mygetsubcat.php?category='+category; // Specifying which file to get ajax[index].onCompletion = function(){ createSubCategories(index) }; // Specify function that will be executed after file has been found ajax[index].runAJAX(); // Execute AJAX function } } function createSubCategories(index) { var obj = document.getElementById('subcat'); eval(ajax[index].response); // Executing the response from Ajax as Javascript code } </script> <title>test</title> <link rel="stylesheet" type="text/css" href="../web1.css" /> <link rel="stylesheet" type="text/css" href="../heading.css" /> <link rel="stylesheet" type="text/css" href="../navigation.css" /> <link rel="stylesheet" type="text/css" href="directory.css" /> </head> <body> <div class="navigation" id="navigation"> <a class="navjust" href="../index.php">Home</a><br /> <br /> <a class="navjust" href="http://mail.meo2000.net">Check Mail</a><br /> <br /> <a class="navjust" href="../web/webservice.php">Web Services</a><br /> </div> <div id="justify"> <div id="h"> <h1>meo2000 listing service</h1> <h3>want to get listed, click <a href="getlisted.htm">here</a> to find out how.</h3> <h3>This directory is under construction!</h3> <br/> </div> </div> <?php Function DisplayForm($errors = null){ $error_string = $errors; //state, you could use $error_string = implode ("<br />",$errors); if you want to save performance by using an array insead. echo(' <div id="left"> <span class="error"> '.$error_string.'<br /> </span> <form method="post" action="'.$_SERVER['PHP_SELF'].'"> State: <select id="state" name="state" onchange="getCityList(this)"> <option value="">Select a state</option> <option value="Alabama">Alabama</option> <option value="North Carolina">North Carolina</option> <option value="Texas">Texas</option> </select><br /> <br /> City: <select id="city" name="city"> </select><br /> <br /> Category: <select id="category" name="category" onchange="getSubCategoryList(this)"> <option value="">Select a catagory</option> <option value="Auto">Auto</option> <option value="Hotel">Hotel</option> <option value="Restaurant">Restaurant</option> </select><br /> <br /> SubCategory: <select id="subcat" name="subcat"> </select><br /> <br /> <input type="submit" name="submit" value="Select"> </form> </div> '); } //check if form has been submitted if (!$_POST['submit']) { //if not display form DisplayForm(); } else { //form has been submitted //items selected, check that number was entered, //Declare it so we dont get notices 'undeclared variable' $error = null; //check state has been selected if (trim($_POST['state']) == "") { $error .='ERROR: Please select a state.'; } //check category has been selected elseif (trim($_POST['category']) == "") { $error .='ERROR: Please select a category.'; } //if there was an error, show it. if ($error != null){ DisplayForm($error); } else { //otherwise carry on? echo '<div id="right">'; $state = $_POST['state']; $city = $_POST['city']; $cat = $_POST['category']; $subcat = $_POST['subcat']; echo 'Here is your selections: <br />'; echo "<i>$state</i><br />"; echo "<i>$city</i><br />"; echo "<i>$cat</i><br />"; echo "<i>$subcat</i><br />"; echo '</div>'; } } ?> <div class="addspace" id="addspace"> <p class="none" id="addmargin"> your<br > add<br > could<br > be<br > filling<br > this<br > spot! </p> </div> </body> </html>
-
error console????????????
-
solved, the problem was with permissions...... what ya know....
-
i use ubuntu and firefox. like i said the image file is in the same folder as the page. and i have tryed multiple images....... no image no matter what.
never had this problem before.....
-
i am trying to add an image to my webpage. the image tag is outside the <?php ?> tags, and the image is a .jpg. i know that the src is correct but image will not display. the image is in the same folder that the page it is to be on. all that displays is the alt. text. here is the code that i used. <img src="image.jpg" height="100" width="100" alt="image" />. but only the alt text displays.........
never had this problem before but i have never added an image to a page with a .php extension.
thanks mike....
-
the problem was with the link to the js file. i fixed this link and now the sample is working and i can experiment with it to try to get it to do what i want.
original:
<script type="text/javascript" src="ajax.js"></script>
after fix:
<script type="text/javascript" src="js/ajax.js"></script>
this was just a copy past from the link above and i thought that i followed the instructions exactly???!!!! not sure.
thanks mike.......
-
i have copy and pasted the sample form, php script, and the ajax.js files, i followed the instructions, the form comes up but doesnt function. what could be wrong......
i am trying to learn how to use ajax but can not figure this out.
here is the link: http://www.dhtmlgoodies.com/index.html?whichScript=ajax_chained_select
i am running ubuntu 8.04, have apache2, and php5 on machine. that all works as my other scripts work......
please help......
mike.......
-
thank you very much, that is will help me alot.....
mike....
-
i have read the tutorial and one at tigzag.com that is very similar to the one at w3.
but nether of them give much detail on how to do what i want to do. can somebody give me a link to a tutorial that would show more on how to do what i am trying to do.
thanks mike......
-
thank you for the link, i would prefer to learn.....
been learning php/mysql, now trying to learn more about how to use javascript, and ajax would be another great tool to learn to use.
thanks again mike.....
-
What I am trying to do is create a sample, or test, form.
I have created 2 sample forms with html/php as follows:
<html> <head></head> <body> <?php //display form to choose state ?> <form method="POST" action="state.php"> Choose a state: <select name="state"> <option value="test1.1">test1.1</option> <option value="test1.2">test1.2</option> <option value="test1.3">test1.3</option> </select><br /> <br /> <input type="submit" name="submit" value="Select"> </form> </body> </html>
This form gives me a way to make my first selection......
and the second:
<html> <head> <title>state</title> </head> <body> <?php if (!$_POST['submitcity']) { //the form has been submitted //look for and process $state = $_POST['state']; //display state selected echo "$state <br />"; //select which list to display next ?> <form action="<?=$_SERVER['PHP_SELF']?>" method="post"> <?php switch ($state) { case 'test1.1': echo (' Select from test2: <select name="city"> <option value="test2.1.1">test2.1.1</option> <option value="test2.2.1">test2.2.1</option> <option value="test2.3.1">test2.3.1</option> </select><br /> <br /> <input type="hidden" name="state" value="test1.1" /> Select from test3: <select name="cat"> <option value="test3.1">test3.1</option> <option value="test3.2">test3.2</option> <option value="test3.3">test3.3</option> </select><br /> <br /> <input type="submit" name="submitcity" value="Select"> '); break; case 'test1.2': echo (' Select from test2: <select name="city"> <option value="test2.1.2">test2.1.2</option> <option value="test2.2.2">test2.2.2</option> <option value="test2.3.2">test2.3.2</option> </select><br /> <br /> <input type="hidden" name="state" value="test1.2" /> Select from test3: <select name="cat"> <option value="test3.1">test3.1</option> <option value="test3.2">test3.2</option> <option value="test3.3">test3.3</option> </select><br /> <br /> <input type="submit" name="submitcity" value="Select"> '); break; case 'test1.3': echo (' Select from test2: <select name="city"> <option value="test2.1.3">test2.1.3</option> <option value="test2.2.3">test2.2.3</option> <option value="test2.3.3">test2.3.3</option> </select><br /> <br /> <input type="hidden" name="state" value="test1.3" /> Select from test3: <select name="cat"> <option value="test3.1">test3.1</option> <option value="test3.2">test3.2</option> <option value="test3.3">test3.3</option> </select><br /> <br /> <input type="submit" name="submitcity" value="Select"> '); break; } } else { //if "submit" variable exist //the form has been submitted //look for and process $state = $_POST['state']; $city = $_POST['city']; $cat = $_POST['cat']; //display state selected before echo "$state <br />"; //display city selected echo "$city <br />"; //dislay the cat selected echo "$cat <br />"; } ?> </body> </html>
this second form script uses your choice from the first form to determine which set of choices to let you choose from....
I would like to create a form that would let you choose a state from the first drop down menu, and that would determine the list of cities that would display in the second drop down list.
hope somebody understands what I am trying to say and can help me or point me to a tutorial that can help me....
thanks mike....
-
i understand thanks....
mike...
-
i have created a sample script with drop down list in my form. all works well exept that i want to be able to make the selection from the first drop down list affect the choices of the second drop down list. here is what i have so far.
<html>
<head></head>
<body>
<?php
Function DisplayForm($errors = null){
$error_string = $errors; // you could use $error_string = implode ("<br />",$errors); if you want to save performance by using an array insead.
echo('
'.$error_string.'<br />
<form method="POST" action="'.$_SERVER['PHP_SELF'].'">
Select from test1:
<select name="state">
<option value="test1.1">test1.1</option>
<option value="test1.2">test1.2</option>
<option value="test1.3">test1.3</option>
</select><br />
<br />');
// assign value $state = <select name="state">
this is were i seem to have the problem, getting the value from first drop down list into a string
$state =//not sure what to do here
switch ($state)
{
case 'test1.1':
echo ('
Select from test2:
<select name="city">
<option value="test2.1.1">test2.1.1</option>
<option value="test2.2.1">test2.2.1</option>
<option value="test2.3.1">test2.3.1</option>
</select><br />
<br /> ');
break;
case 'test1.2':
echo ('
Select from test2:
<select name="city">
<option value="test2.1.2">test2.1.2</option>
<option value="test2.2.2">test2.2.2</option>
<option value="test2.3.2">test2.3.2</option>
</select><br />
<br /> ');
break;
case 'test1.3':
echo ('
Select from test2:
<select name="city">
<option value="test2.1.3">test2.1.3</option>
<option value="test2.2.3">test2.2.3</option>
<option value="test2.3.3">test2.3.3</option>
</select><br />
<br /> ');
break;
default:
echo ('
Select from test2:
<select name="city">
<option value=" "> </option>
</select><br />
<br /> ');
break;
}
echo ('
Select from test3:
<select name="cat">
<option value="test3.1">test3.1</option>
<option value="test3.2">test3.2</option>
<option value="test3.3">test3.3</option>
</select><br />
Please enter a number between 1 and 10:
<input type="text" name="num" size="2">
<br />
<input type="submit" name="submit" value="Select">
</form>
');
}
//check if form has been submitted
if (!$_POST['submit'])
{
//if not display form
DisplayForm();
}
else
{
//form has been submitted
//items selected, check that number was entered,
$error = null; //Declare it so we dont get notices 'undeclared variable'
//check number range
if (!isset($_POST['num']) || !is_numeric($_POST['num']))
{
$error .='ERROR: Please enter a number between 1 and 10';
}
elseif ($_POST['num'] < 1 || $_POST['num'] > 10)
{
$error .='ERROR: The number must be between 1 and 10';
}
//if there was an error, show it.
if ($error != null){
DisplayForm($error);
}
else
{
//otherwise carry on?
$state = $_POST['state'];
$city = $_POST['city'];
$cat = $_POST['cat'];
echo 'Here is your selections: <br />';
echo "<i>$state</i><br />";
echo "<i>$city</i><br />";
echo "<i>$cat</i><br />";
}
}
?>
</body>
</html>
i am still fairly new at php... and any help with this would be great
i tried to put submit buttons in the first list but they do not show up in the list, they always show up net to the list. but that seems to work, problem is in a real script i want to be able to select a state and then have a selection of cities for that state show up in the second choice box..... hope somebody can understand what i am trying to ask....
thanks mike....
-
I have read a book called "how to do everything with php&mysql" by Vakram Vaswani.
very good book, explains most of the basics and gives good examples, that is how i learned how to write the script above and how to use validation to check the input.
i will look at the site that you suggest, but i do how a good understanding of the basics.
thanks for the info and the link.
-
i only had to change the validation part of your script to:
//check number range
if (!isset($_POST['num']) || !is_numeric($_POST['num']))
{
$error .='ERROR: Please enter a number between 1 and 10';
}
elseif ($_POST['num'] < 1 || $_POST['num'] > 10)
{
$error .='ERROR: The number must be between 1 and 10';
}
//if there was an error, show it.
from:
//check number range
if (isset($_POST['num']) && is_numeric($_POST['num']) && $_POST['num'] < 1 || $_POST['num'] >10)
{
$error .= 'ERROR: The number must be between 1 and 10';
}
if i used the validation that you show here i could still leave the number input blank it would pass validation......
but still thanks for the help...
now what if i wanted to make a selection in the first box affect my choices in the second box.
say i wanted the first box to let me choose a state, well then my second box would be
to choose a city within that state.
how would something like that look.....
can somebody please help with that.....
thanks mike
-
yes it makes sense, thank you very very much. now i can test this out, and move on with my development.
-
could you show me an example against the sample script that i have included in the original post, i am very new at this, or at least give me a link to examples i can see.
thanks mike
-
i would like to put the error on the same form that way it can be corrected and resubmitted.
-
A am new to php and have been trying to create a form to collect information. I can get the form to post and can even validate the input, the problem comes when there is a failure in validation, how do you get the form to reload and display the error that needs to be fixed. All I seem to be able to do is let the script die and display the error.
Here is a sample form that I have created to test this on:
<html>
<head></head>
<body>
<?php
//check if form has been submitted
if (!$_POST['submit'])
{
//if not display form
?>
<form method="POST" action="<?=$_SERVER['PHP_SELF']?>">
Select from test1:
<select name="state">
<option value="test1.1">test1.1</option>
<option value="test1.2">test1.2</option>
<option value="test1.3">test1.3</option>
</select><br />
<br />
Select from test2:
<select name="city">
<option value="test2.1">test2.1</option>
<option value="test2.2">test2.2</option>
<option value="test2.3">test2.3</option>
</select><br />
<br />
Select from test3:
<select name="cat">
<option value="test3.1">test3.1</option>
<option value="test3.2">test3.2</option>
<option value="test3.3">test3.3</option>
</select><br />
Please enter a number between 1 and 10:
<input type="text" name="num" size="2">
<br />
<input type="submit" name="submit" value="Select">
</form>
<?php
}
else
{
//form has been submitted
//items selected, check that number was entered,
$num = (!isset($_POST['num']) || trim($_POST['num']) == " " || !is_numeric($_POST['num']))
? die ('ERROR: Please enter a number between 1 and 10') : trim($_POST['num']);
//check number range
if ($num < 1 || $num >10)
{
die ('ERROR: The number must be between 1 and 10');
}
//if so, display them
$state = $_POST['state'];
$city = $_POST['city'];
$cat = $_POST['cat'];
echo 'Here is your selections: <br />';
echo "<i>$state</i><br />";
echo "<i>$city</i><br />";
echo "<i>$cat</i><br />";
}
?>
</body>
</html>
any help with this would be greatly appreciated......
mike
Is it possible to validate radio buttons and Select list/menu with PHP?
in PHP Coding Help
Posted
here is the part that does my validation: