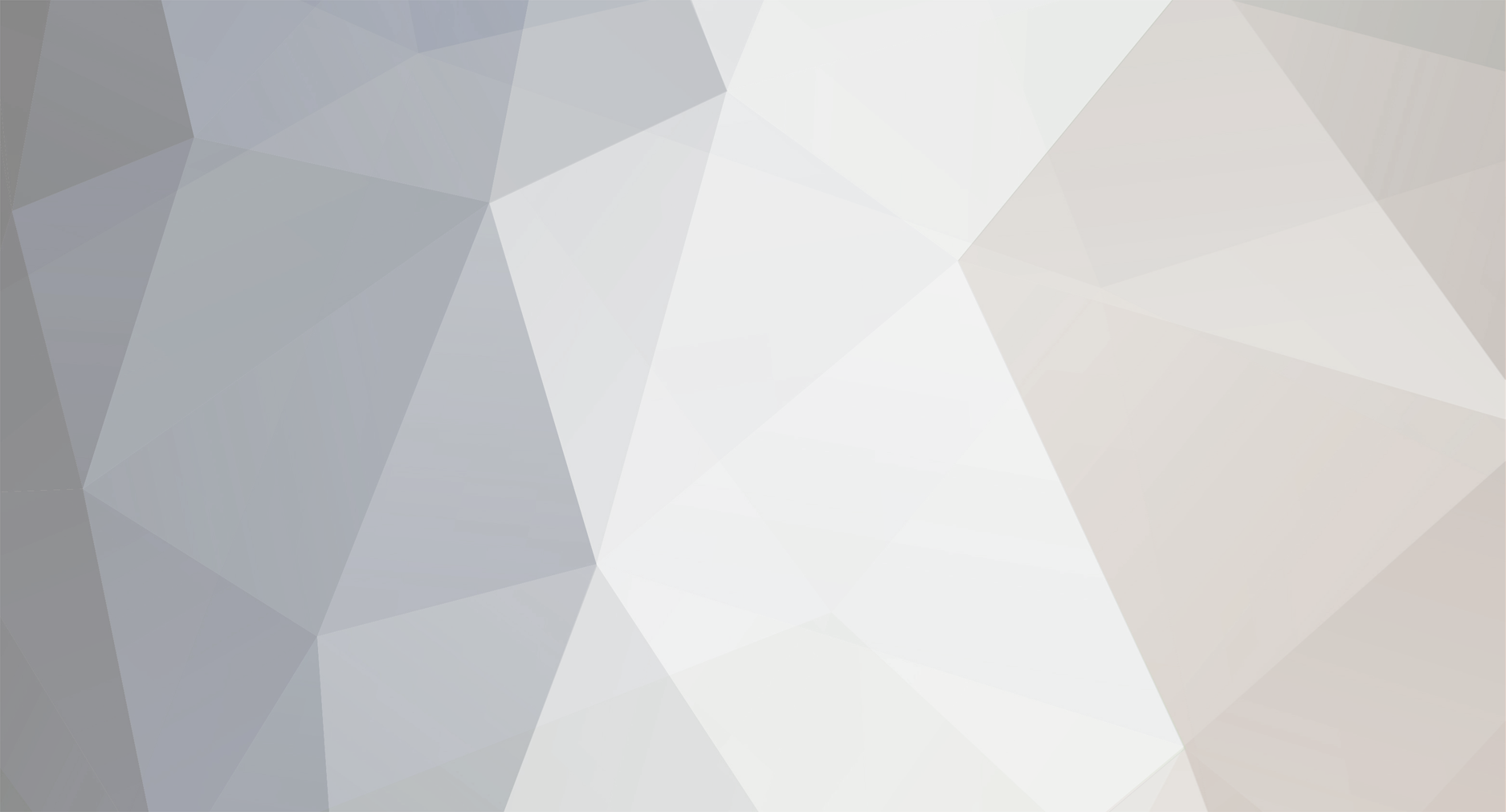
Errant_Shadow
-
Posts
123 -
Joined
-
Last visited
Never
Posts posted by Errant_Shadow
-
-
there's 2 ways I can think of off the top of my head
if( !empty($_POST['year']) ) { $year = $_POST['year']; $month = $_POST['month']; $day = $_POST['day']; $hourFrom = $_POST['hourFrom']; $hourTo = $_POST['hourTo']; $url = "/bla/bla/$year$month$day/"; if (!$dir = opendir($url)) // if the operation returns nothing... { echo '<p>No Such Directory ('.$url.')</p>'; } else { while ($file = readdir($dir)) { // display files } } }
or
if( !empty($_POST['year']) ) { $year = $_POST['year']; $month = $_POST['month']; $day = $_POST['day']; $hourFrom = $_POST['hourFrom']; $hourTo = $_POST['hourTo']; $url = "/bla/bla/$year$month$day/"; if (!file_exists($url)) // if the specific file does not exist on that server... { echo '<p>No Such File ('.$url.')</p>'; } else { $dir = opendir($url); while ($file = readdir($dir)) { // display files } } }
though I've never tested that second one on files not on my own server.
-
My question is not now to verify an e-mail address; I've got most of that down. My question is regarding fsocketopen, and communicating with a mail server.
Basically, the script I'm designing will run a series of tests to verify that a given e-mail address meets all of the syntax requirements it needs to, then it checks the domain name, finds the mail server, and makes sure the given address is actually registered.
The problem I'm having is with opening the socket. I keep getting a connection refused error (No 111). Since then I switched to udp protocol and it's not giving me the error, but I think its still not connecting. I can't actually get anything from it with either fread() or fgets().
this is what I got:
$emailDomain = "google.com"; // the domain I'm testing against $dnsr = dns_get_record($emailDomain, DNS_MX); // fetch the DNS records for mail exchange servers if (count($dnsr) < 1) { // if there are no DNS records // return array('isValid' => false, 'reason' => 'Invalid E-Mail DNS Entry! ('.$emailDomain.')'); echo '<p>'.$emailDomain.' is invalid...</p>'; } else { // find the highest priority server $index = 0; $priority = $dnsr[0][pri]; foreach ($dnsr as $i => $array) { if ($dnsr[$i][pri] < $priority) { $priority = $dnsr[$i][pri]; $index = $i; } } $reply = array(); $err = array ('no' => NULL, 'str' => ""); if (!$socket = fsockopen($dnsr[$index]['target'], 25, $err['no'], $err['str'], 30)) { // return array('isValid' => false, 'reason' => 'Connection Failed (Error No. '.$err['no'].': '.$err['str'].')'); echo '<p>Connection Failed (Error No. '.$err['no'].': '.$err['str'].')</p>'; } else { fwrite($socket, "HELO ".$_SERVER['HTTP_HOST']); while (!feof($socket)) { $reply[] = fgets($socket,255); } fclose($socket); } print_r($reply); }
and this is what it gives me:
Connection Failed (Error No. 111: Connection refused)If I add 'udp://' to the fsocketopen command (fsockopen('udp://'.$dnsr[$index]['target'], 25, $err['no'], $err['str'], 30)) it stops giving me an error, but when I run it, the page will sit there and load forever and never actually return anything.
-
What are you actually trying to do here? And what is it doing instead?
(Are you trying to insert a new field or update an existing field?)
Try this after running the query, but before you close the connection:
echo $mysql_error();
-
because isset() checks to see if that variable is set.
So, if $_GET['God'] is set, then isset($_GET['God']) will translate to "true" but it won't give you the value of $_GET['God']
if you want to check to make sure $_GET['God'] is not only set, but set to something specific, use:
if ($isset($_GET['God']) && $_GET['God'] == "Enter")
so it checks to see if $_GET['God'] is set at all, and if it is (only if it is) it will then check the value.
-
Okay, what I'm trying to do is to use a form to append an already existing GET data string.
It goes something like this:
REQUEST_URI="/page.php?var1=foo&var2=bar"
Now, what I want to do is just add a new variable to the end there without replacing the whole thing. I try using the URI as the form action, but it just replaces the string entirely, returning "/page.php?var3=wtf" rather than "/page.php?&var1=foo&var2=bar&var3=wtf"
Any ideas how to make this work?
-
as a note: mysql_insert_id() MUST be called immediately after the insert query, even if the only other query run was not an insert. It will return 0 otherwise, rather than the insert id.
-
Ah, that's awesome. No, none of my tables are BIGINT sized so there shouldn't be a problem.
Thank you very much
-
I've been having a hard time finding this because I'm not sure how to ask it succinctly.
Basically, I'm entering new data rows into a database, but I then need to retrieve the auto-incremented primary key for use immediately after. I can't know what the key will be before I create the row (that I'm aware of), so I was wondering if there was anything I could do to retrieve that value without creating and running a separate query.
-
I've read through tons of resources and tutorials and as far as I can tell, my code is accurate, but for some reason it's not working...
My HTML Form:
<form action="script.php" name="update" method="post"> <input type="file" name="update_banner" size="22" /> <br /> <input type="submit" name="update_submit" value="Update Subscription" /> </form>
My Handling Script:
// if there is an update submitted... if (isset($_POST['update_submit'])) // if the update form has been submitted { // if there is a file to upload... if (isset($_FILES['update_banner']) && !empty($_FILES['update_banner']['name'])) { // it should give me all the info about that file... die(print_r($_FILES)); } else { die("No file has been uploaded"); } } else { die("No upload has been submitted"); }
It should output all the info about that file, but I load the form, select an image from my computer, hit submit, and it tells me there is no file.
Any ideas why?
-
Cool. That's pretty much exactly what I'm after; fetching all current subscriptions, and doing something for each existing subscription.
As a side question, what's the difference between "!=" and "!==" and when would it be appropriate to use one over the other? Like, I know "!=" means "NOT EQUAL TO", so what additional limitations does "!==" include?
-
I see.
so, to save on lines and make it all easier to update each query...
$ID = 4 $_SCRIPS = array(); $myDatabases = array('red','blue'); for ($i = 0; $i < count($myDatabases); $i++) { $result = mysql_query("SELECT * FROM $myDatabases[$i].subscriptions AS SCRIP WHERE SCRIP.ID = $ID"); if ($result) { while ($row = mysql_fetch_assoc($result)) { $_SCRIPS[$myDatabases[$i]][] = $row; // would this need that extra [] to work right? } } }
might come out something like...
$_SCRIPS = array ( ['RED'] => array ( 'ID' => 4, 'Name' => John Doe, 'Title' => PHP Weekly, 'StartDate' => 2009-10-01, 'ExpireDate' => 2010-09-30 ), ['BLUE'] => array ( 'ID' => 4, 'Title' => PHP Weekly, 'PaidDate' => 2008-09-12, 'IssueCount' => 12 ) )
So not only would I be able to change the script and effect each query, I could aslo do something like:
if (isset($_SCRIPS['RED']['ID'])) { do something... }
-
What if the rows aren't all the same? Each subscription will contain different data, and even different data types, there are a few common denominators, but the bulk of each table will be unique.
Would I be better off just running separate queries for simplicity's sake?
-
would it just be like this?
SELECT * FROM `red`.`subscriptions`, `green`.`subscriptions`, `blue`.`subscriptions` WHERE `account_id` = "$num"
... or would I need to describe each separate database in the WHERE clause?
like this?
WHERE `red`.`subscriptions`.`account_id` = "$num" OR `green`.`subscriptions`.`account_id` = "$num" OR `blue`.`subscriptions`.`account_id` = "$num"
-
I'll try to be as simple as I can in my description.
Basically, I have 4 databases; for simplicity's sake we'll call them `global`, `red`, `blue`, and `green`.
`global` has a table called `accounts`, with `id` as it's primary field.
Now, `red`, `blue`, and `green` each have a table named `subscriptions`, with `id` as their primary key and `account_id` as a foreign key that references `global`.`accounts` (`id`).
Let's say your account id is 3, and you wanna see a list of all of your subscriptions. What I need to do is have my script fetch ALL the data held in the `subscription` table from any database where `account_id` matches a given `id` from the `accounts` table in the `global` database.
So I need to query all of the databases for the same field in the same table for a single value, and if that value is true, I need all of the data.
-
Oh MySQL, you uppity whore =_=
Found the problem... I'm using phpMyAdmin to create all these.
It wouldn't create the database because I was sending the command from the database that the table is being CREATED in but it wanted me to send it from the database that the FOREIGN KEY is REFERENCING.
I got it to work:
CREATE TABLE `db2`.`subscriptions` ( `id` SMALLINT(5) UNSIGNED NOT NULL AUTO_INCREMENT COMMENT 'Primary Key. Used to link users to their PMs, posts and feedback.', `account_id` MEDIUMINT( UNSIGNED NOT NULL COMMENT 'Foreign Key. Used to link dealers back to their accounts.', ... PRIMARY KEY (`id`), FOREIGN KEY `account_id` (`account_id`) REFERENCES `db1`.`accounts`(`id`) ON DELETE CASCADE ) ENGINE = InnoDB;
but I had to execute that from db1, rather than db2 where it's being made
-
I've been able to create a foreign key when the tables are all on the same database, so this problem must have something to do with them being on separate databases...
-
Unfortunately, same error =_=
-
I mean, should I be making subscriptions.account_id reference account.id
(like this?)
... or should I make a separate foreign key for accounts.id that references subscriptions.account_id on each other database?
(like this?)
so, rather than using what I posted way back at the beginning, doing something like this (for the account table, rather than the subscription table):
CREATE TABLE `accounts` ( `id` MEDIUMINT( UNSIGNED NOT NULL AUTO_INCREMENT COMMENT 'Primary Key. Used to link users to their PMs, posts and feedback.', `banned` BOOLEAN NOT NULL DEFAULT 0 COMMENT 'Records wether or not the account is banned; 0 = NO, non 0 = YES.', `gender` ENUM('Mr.','Ms.') NOT NULL COMMENT 'The users title.', `name` VARCHAR(31) NOT NULL COMMENT 'The users first name.', `email` VARCHAR(63) NOT NULL COMMENT 'The users email address.', `state` VARCHAR(2) NOT NULL COMMENT 'The state of the dealership the user works for.', `county` VARCHAR(31) NOT NULL COMMENT 'The city of the dealership the user works for.', `PWORD` VARCHAR(127) NOT NULL COMMENT 'The users password.', `REMOTE_ADDR` VARCHAR(15) NOT NULL COMMENT 'The users ip address.', `reg_date` TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT 'The date and time of the users registration.', PRIMARY KEY (id), FOREIGN KEY `db1_account_id` (`id`) REFERENCES `db1`.`subscriptions` (`account_id`) ON DELETE CASCADE, FOREIGN KEY `db2_account_id` (`id`) REFERENCES `db2`.`subscriptions` (`account_id`) ON DELETE CASCADE, FOREIGN KEY `db3_account_id` (`id`) REFERENCES `db3`.`subscriptions` (`account_id`) ON DELETE CASCADE, UNIQUE (email, REMOTE_ADDR) ) ENGINE = InnoDB;
-
Hmm... good question. There is exactly 1 record in the accounts table, but since I'm creating the subscription table now, there are 0 records in it. Could this cause the problem?
Not every account will have a subscription, but every subscription will have an account. Will this work the way I'm designing it?
-
Now, while that solved the question here (thus solving the thread) it did not fix my problem...
So if anyone would like to continue helping me to find a solution to my rather persistent bug, I've made a new thread: The Indestructable Session
-
Okay, so my problem is thus:
I have a session working across (almost) all my subdomains (I know this much is working), but when I try to destroy the session (to log out) I can't seem to even find it.
It looks like it works across all of my subdomains, EXCEPT "www"
What I did to get it working across my subdomains was I opened up my php.ini file and added ".communitybigtop.com" to "session.cookie_domain"
What's getting me is that it's working for everything EXCEPT "www"...
-
Well, I've found a few methods to do this...
The php.ini configuration contains a line that, by default, is blank; your server fills this in with your full domain name when a session is created. Unfortunately, that includes the subdomain, thus limiting that session to ONLY that subdomain.
To fix this you can either open the php.ini and change it to read as:
"session.cookie_domain = .yourdomain.com"(the dot separator there at the beginning is important for cross-browser compatibility)
Or, if you can't, or don't want to access the php.ini file, you can use the ini_set method to do pretty much the same thing:
ini_set('session.cookie_domain', substr($_SERVER['SERVER_NAME'],strpos($_SERVER['SERVER_NAME'],"."),100));
And this can be included in an init file that should be included in every page; or it can be included by itself and it appears to run fine.
Alternatively, you can use the session_set_cookie_params() method before every call to session_start(). The parameters available include int $lifetime [, string $path [, string $domain [, bool $secure = false [, bool $httponly = false ]]]]. The brackets mean that in order to set the domain, you must first set the life time and the path (in that order); keeping in mind that the default life time is zero (0) and the default path is "/".
So this method would read:
session_set_cookie_params(0, "/", ".yourdomain.com")
-
I need to make a session that will stay active across all of my sub domains, but so far all I can make is a session that works only on the sub domain it was created on.
-
That'll work. Thank you
if structure off
in PHP Coding Help
Posted
The code you have here should display whatever data is stored in the message variable, if the message variable exists. Are you saying that your script is /not/ showing the message when it /does/ exist?