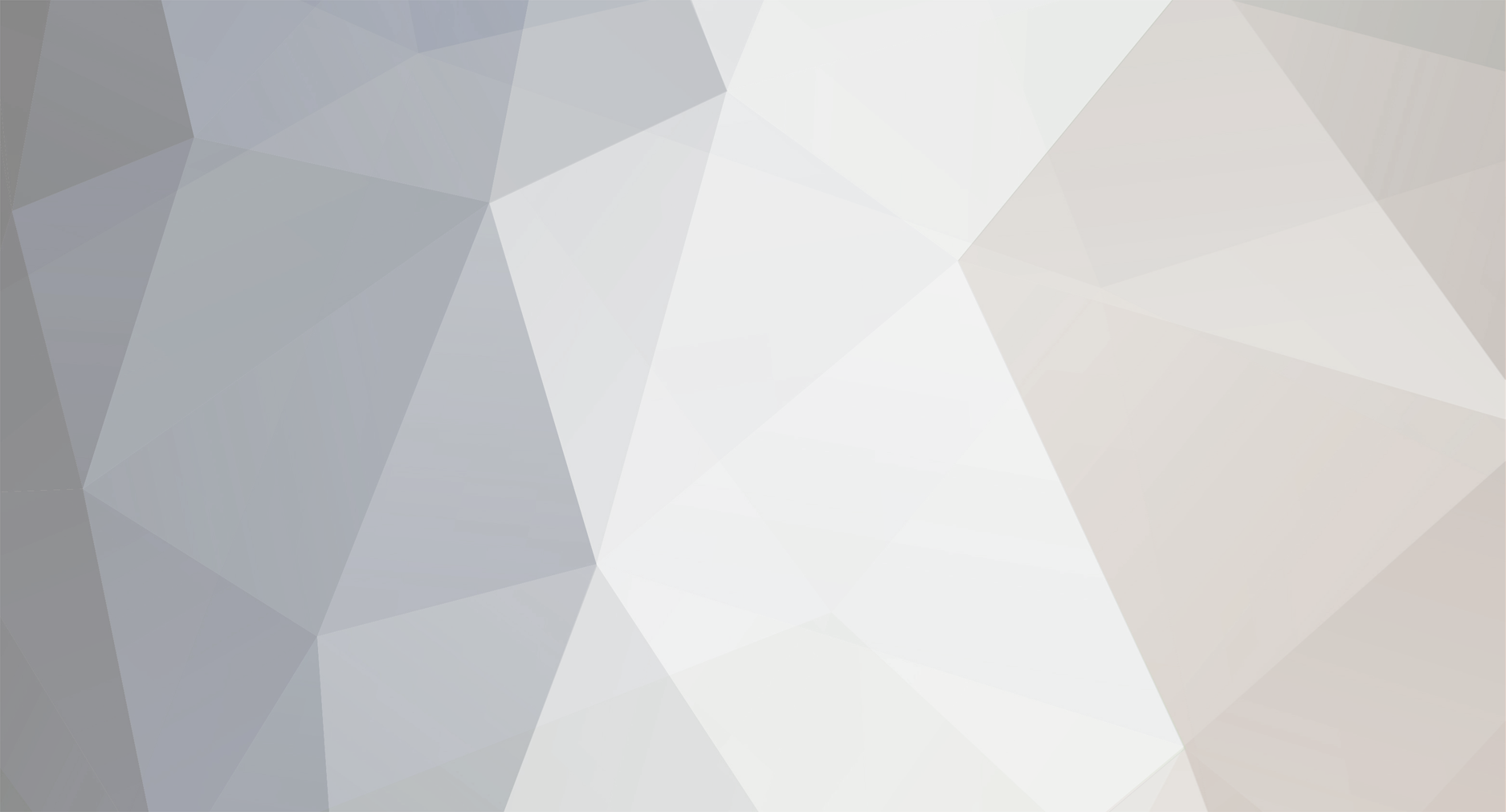
Errant_Shadow
-
Posts
123 -
Joined
-
Last visited
Never
Posts posted by Errant_Shadow
-
-
ah... well that's a lot easier than what I was about to do >>
$includePrefix = ""; if ($_SERVER['REQUEST_URI'] != "/") { $includePrefix = "../"; } include_once $includePrefix . "scripts/functions/scaleImage(imgLink,maxWidth,maxHeight).php"; include_once $includePrefix . "scripts/functions/checkInput(input).php";
Thank you, I really appreciate your help; I wasn't aware that PHP treated full HTML URL's that way.
-
So a partial URL, like just "scripts/functions/..." ?
Since header.php is being called from different places, the local path will change. For some pages it will be "scripts/functions/..." but others will have to be "../scripts/function/..."
-
Alright, I'll modify the sql connection; thank you.
The function definition exists in header.php, which is included into every page and the include lines exist at the top of the scripts to be free of all functions and conditionals, and before any html is sent to the page.
After trying a few other things I got it to work when I paste the script directly into the page itself, rather than the header, which is included in the page. I know the header is being included because it formats the page correctly. Additionally, I can access a -different- function that I included in the same way, just not this one for some reason.
-
I can find a lot of resources online to solve unidentified function errors for -specific- things. Mostly built-in functions like mysql_connect. The problem is that it can't find a function that -I- wrote, that it sitting RIGHT THERE in the damn script. I thought maybe it was some stupidness happening with my include_once line, but then I just took that out and put the function itself in there and it -STILL- can't find it...
// include_once "http://".$_SERVER['SERVER_NAME']."/scripts/functions/checkInput(input).php"; // used to strip slashes or escape data function checkInput ($input) { // connect to mySQL $dbc = @mysql_connect("localhost", "NAME", "PASS") or die("&err_msg=MYSQL Error (Could not connect to database)."); // select database ($databaseName) @mysql_select_db("rey619_bigtop") or die("&err_msg=MYSQL Error! " . mysql_error($dbc)); // if the input uses magic quotes... strip the slashes if (get_magic_quotes_gpc()) { $input = stripslashes($input); } // if the input is NOT numeric... escape the data if (!is_numeric($input)) { $input = mysql_real_escape_string($input); } // close mySQL connection mysql_close($dbc); // finally, return the input return $input; } // end function check_input ($input) // 339 lines later, inside some nested IF,THEN,ELSE statements... $test = "What's my line?"; $test = checkInput($test); echo "<p>" . $test . "</p>";
echoes:
Fatal error: Call to undefined function checkinput() in /home/rey619/public_html/register/index.php on line 108So my question is, as always, wtf?
-
I know. My brain is kind of fried trying to track all of the data I'm using, but I'll try to be as simple as I can to convey my problem.
So what I have is an array of arrays. It is called racerArray and it is composed of all the character data for each racer participating in the race that my script runs. This is *technically* ordered by index, but the index starts at 1 rather than 0, so its easier to think about it as an associative array where each key represents a racer's position in the race, from first to last.
The individual characer arrays are assembled from data I fetch from MySQL and contain the character's name, account name, current experience points, etc etc, but more importantly, it contains their skill ranks, their bike's stats, and the damage value for each part (which is in it's own sub array inside the character array).
Additionally, I have a multidimensional associative array much in the same fashion for holding the track. It is called the trackArray and each element (starting at 1) holds the data for that individual segment, which includes the segment's length, the condition, a hazard for the racers to overcome, and an array of stat modifiers to apply to each racer's bike stats.
So basically, what I need to do is walk through the trackArray, and at each segment, use the difficulty mod, the length, the condition and hazard information, and the array of stat modifiers to calculate how each racer did; if they crashed, how much damage each part took on this segment, of a part broke down, and then (if they made it through the segment) how well they did in a way I can compare to each other racer.
Now, I have a model that does all that and worked well enough for my purposes, but i've had to strong-arm, and make work-arounds for a lot of it and I was just hoping that there might be an easier way to accomplish my end goal.
Edit: I will post some code, but I'm swamped at the moment and I have to clean up all my neurotic notes and such first.
-
what the hell... how did this... get here? *confused* I swear to god I hit reply from a different topic, but it posted here for some reason...
-
I know. My brain is kind of fried trying to track all of the data I'm using, but I'll try to be as simple as I can to convey my problem.
So what I have is an array of arrays. It is called racerArray and it is composed of all the character data for each racer participating in the race that my script runs. This is *technically* ordered by index, but the index starts at 1 rather than 0, so its easier to think about it as an associative array where each key represents a racer's position in the race, from first to last.
The individual characer arrays are assembled from data I fetch from MySQL and contain the character's name, account name, current experience points, etc etc, but more importantly, it contains their skill ranks, their bike's stats, and the damage value for each part (which is in it's own sub array inside the character array).
Additionally, I have a multidimensional associative array much in the same fashion for holding the track. It is called the trackArray and each element (starting at 1) holds the data for that individual segment, which includes the segment's length, the condition, a hazard for the racers to overcome, and an array of stat modifiers to apply to each racer's bike stats.
So basically, what I need to do is walk through the trackArray, and at each segment, use the difficulty mod, the length, the condition and hazard information, and the array of stat modifiers to calculate how each racer did; if they crashed, how much damage each part took on this segment, of a part broke down, and then (if they made it through the segment) how well they did in a way I can compare to each other racer.
Now, I have a model that does all that and worked well enough for my purposes, but i've had to strong-arm, and make work-arounds for a lot of it and I was just hoping that there might be an easier way to accomplish my end goal.
Edit: I will post some code, but I'm swamped at the moment and I have to clean up all my neurotic notes and such first.
-
I'm using the array's internal pointer to navigate through my arrays to analyze the data
what I've been doing is setting up a for loop, but using the current() and next() in lieu of the traditional variable ($i). And I know, foreach does exactly that, but I've been having a lot of trouble getting foreach to do what I want. It keeps only running it once as far as I can tell.
Anyway, basically I have an array of arrays, where each sub array contains various character data. What I've been doing is using $r as my navigation variable in a for loop, ending up with blocks of code like $raceArray[$r]['name']. Now, this works, except for some reason it never reads the last element. I think this has something to do with the fact that my array indexes range from 1-10 rather than 0-9; although I've tried adding 1 to sizeof($raceArray) but it doesn't seem to help.
Which brings me to using current() and next() but for the life of me I can't figure out how to use these to navigate a multidimensional array... the one that's really getting me is using this technique inside an additional associative sub array (where I can't even get a traditional for loop to work at all)
what I'm doing now is something like current($raceArray[$r]['damage']) to check the data held in each element of the damage array, which is contained inside the character array inside the race array... any advice?
-
Any advice at all?
-
That makes sense. Sounds like it's easier on the server for larger processes.
-
*nods* I think so. I'll research foreach() some time in this coming week and I suspect I'll implement it.
My usual programming methods are to find some convoluted, overly complicated way to do something, then find out there's an easier way and re-write my code to use that ><
On the plus side, once I find that easier method, I'm slow to forget it
What does flush() and ob_flush() do here?
-
On one last note:
on my method using reset(), current(), and next(). It -will- work, -but- my initial method was flawed.
<?php // here, $r represents the current index in $racerArray // first, I set up an $endof... variable to use in my loop, and then reset the internal pointer $endof_racerArray_this_damage = end($racerArray[$r]['damage']); reset($racerArray[$r]['damage']); // now, using my array as the first arguement of the for() loop, // as long as the current pointer is not set to the end of the array (using our $endof... variable), // I move the pointer to the next index for each successive loop... for ($racerArray[$r]['damage']; current($racerArray[$r]['damage']) != $endof_racerArray_this_damage; next($racerArray[$r]['damage'])) { // unlike array_walk(), you can easily and directly modify your array's values // and even access external arrays and use other variables inside the loop // but this is an important part... // if at any time inside this loop you create some criteria that would disturb the loop, // for instance, such as destroying the array with unset(), or otherwise create an invalid // argument, you -must- break the loop manually, so be careful if ($stuff == "happening") { break; } // end if() } // end for ( ... ) ?>
-
Actually, array_values() worked perfectly. I looked at that in the manual, but I had already read through almost everything else and I just skimmed it; never clicked with me to try it
Thank you very much for you help, MadTechie, I really appreciate it.
-
What I'm doing is setting a few multidimensional arrays from mysql and using the data in a browser game. So when the game calls for it, I need to remove certain elements, such as NPC competitors from those arrays as I process them over and over.
... would it work to use next() last() and current() in lieu of a traditional for() loop?
something like...
<?php // for a given array $myArray = array(1, 2 ... "n"); // find the value of the last element in the array $endof_myArray = end($myArray); // then reset the pointer, checking to make sure the current index does not point to the end, // and moving the pointer ahead by one for each successive loop... for (reset($myArray); current($myArray) != $endof_myArray; next($myArray) { // do some stuff... if (current($myArray) != "foobar") { current($myArray) = "foobar" } // end if () } // end for () ?>
-
As my title suggests, I'm removing an element in an array, but what's happening is that it's leaving a hole in the index sequence.
I start with something like...
$myArray = array("dog", "fish", "cat");
then I remove index number 1 ("fish") with unset...
unset($myArray[1]);
but it leaves the keys in tact, returning
.[0] => "dog"
.[2] => "cat"
what I need it to do is return:
.[0] => "dog"
.[1] => "cat"
because if I were to, say, use a for() loop to increment through the array, it would return an error when it got to index 1, since it doesn't exist, and since the last index of the array is now 1 number higher than it should be, the for() loop won't even check it; it'll hit the end of the loop before the index variable is high enough.
I've read through a lot of array documentation in the PHP manual, but I've found nothing that's worked so far... any advice on this one?
-
It's using an inner join with a bunch of WHERE clauses...
$query = 'SELECT * FROM characters, bikes WHERE characters.name != "' . $_SESSION['character'] . '" AND characters.level = "' . $racerArray[0]['level'] . '" AND bikes.class = "'. $racerArray[0]['class'] . '" ORDER BY RAND() LIMIT ' . $_POST['flaCompetition'];
(simplified)
$query = 'SELECT * FROM table1, table2 WHERE "this" AND "that" ORDER BY RAND() LIMIT #';
-
As made obvious by the title of the thread, I'm trying to retrieve a random number of rows from MySQL.
But more than that I'm trying to retrieve a number of random and *unique* rows from MySQL; see, I'm running into the problem where it will return identical rows. Now, I blame this mostly on the fact that I have a very small number of rows right now, but none the less there are enough there that I shouldn't -have- to see identical rows. So is there any way that I can have it return only unique rows -- never the same row more than once?
-
*sighs* Hopefully next time I'll remember to check reserved words ><
Thank you very much, GingerRobot.
-
Is there even an error as far as anyone can see? Maybe the problem is with the server, and not me...
-
Anyone? As far as I can find, the syntax is:
field_name ENUM('a', 'b', 'c') [NULL | NOT NULL] [DEFAULT default_value] [etc]
I thought it was the default that was messing it up, but even after I took that out, it's still failing.
-
Using MySQL 5.0.45
the SQL query...
CREATE TABLE $TrackName ( segment_id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT COMMENT 'Primary Key. Auto Inremented Index.', length TINYINT UNSIGNED NOT NULL DEFAULT 1 COMMENT 'The length of the segment.', condition ENUM('grass', 'rocks', 'dirt', 'mud', 'snow') NOT NULL COMMENT 'What the segment is made of.', hazard ENUM('corner', 'hill', 'jump', 'cliff', 'ice', 'debris') NOT NULL COMMENT 'What is on the segment.', PRIMARY KEY (segment_id), ) ENGINE = MYISAM;
returns...
Failed to execute SQL : SQL [query] failed : You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'condition ENUM('grass', 'rocks', 'dirt', 'mud', 'snow') NOT NULL COMMENT 'W' at line 1I've looked through manuals, but I can't figure out what I've done wrong. Any advice?
-
I'm making some database tables for a game I'm working on and I've come to be stuck on a decision. The game is based on racing cars, so I'll need to create tracks and save their data in MySQL. Now what I've got so far is an array of segments including the length of the segment, what the segment is made of (dirt, rocks, etc etc), and what hazard exists on the segment (corner, hill, etc etc). So a track is basically an array of these segments, but each track will be different. I know there has to be at least 2 segments for the most basic kind of track (an oval) but more elaborate tracks may have any number of segments...
So my decision is this: do I make one database table with the track name and a maximum number of possible segments, using SET to hold the three segment variables, and allow unused segments to be null, or do I make a new table for each track, where each row contains the three elements in separate rows?
(MySQL version 5.0.22, in case that makes a difference)
-
Okay, so the people I'm working for don't exactly know what they're doing. I'm designing a site for them to run a game I'm also designing. They gave me a server to use to get everything running, but for some reason it is set not to report errors.
Unfortunately, setting and configuring the server is a little bit above me expertise. Any advice?
-----
Nevermind, I found the tool to help me do this.
-
ah, I see. thank you very much
Image resize on upload
in PHP Coding Help
Posted
Is there a simple way to just take an image submitted by a user and scale it to fit within maximum constraints that doesn't involve hundreds of lines and dozens of steps to install?
I need to take images from users, make sure they are no more than 432px wide, 288px high, and no more than 200 kb in size; and if they ARE, then I need to scale them to fit those constraints.
I'm working on a Linux server with PHP version 5.2.9