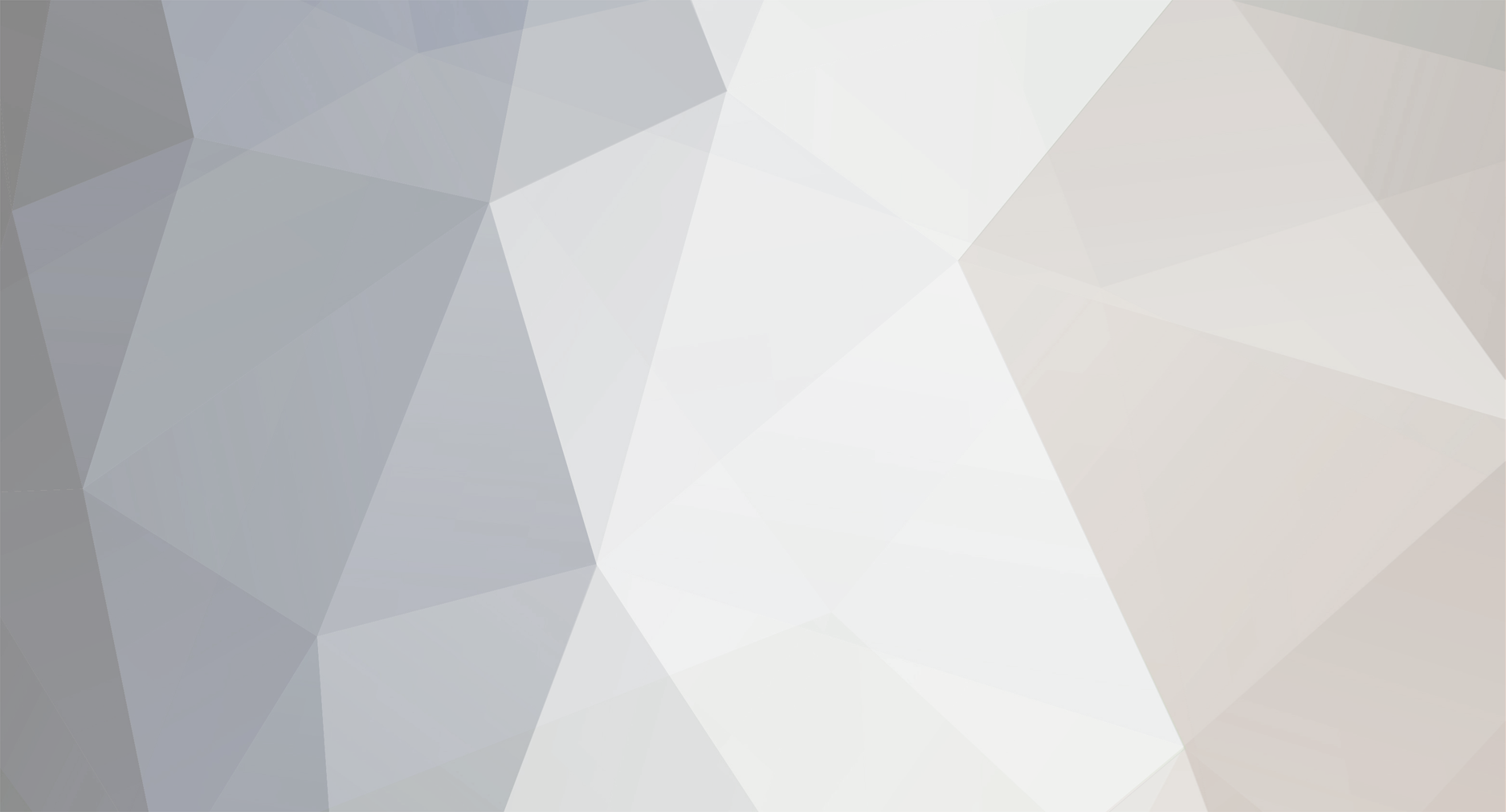
ialsoagree
Members-
Posts
406 -
Joined
-
Last visited
Everything posted by ialsoagree
-
Add Form Errors beside Label/Input on Page?
ialsoagree replied to mfleming's topic in PHP Coding Help
Can't give you a specific example because the code for your form is not present. In general, you accomplish this by modifying the code that writes the form to the browser to include the errors. In order to check for errors somewhere else and know what errors to print, you need to save the relevant errors to a variable (usually an array) and then, when PHP is writing the form, check the array to see if that particular element has an error (and if it does, show the error message next to the element in error, if it doesn't, just write the normal form code for that element). -
Your problem is here: $text = mysql_real_escape_string($_POST[text]); Unless the word "text" has been defined as a constant, you're not accessing an index, you need to do: $text = mysql_real_escape_string($_POST['text']);
-
See mysql_info(): http://www.php.net/manual/en/function.mysql-info.php
-
Quick count suggests that function validateAge($age){ doesn't have a closing brace.
-
Would need to see more of the code before I could tell you what's going on. Specifically, how is the variable $text being defined?
-
What does your code currently look like? Have you made the change I suggested?
-
You're not using "mysql_real_escape_string" properly. You need to escape the data that is going into the database. Your use of mysql_real_escape_string actually doesn't do anything, because you don't save the result to a variable. In fact, the entire "addslashes" list at the beginning of your code is not needed, you can simply do: if (isset($_POST['add'])) { $e1 = mysql_real_escape_string($_POST['element_1']); // etc.}$update = '/* your update statement here - no need for mysql_real_escape_string anywhere here! */';mysql_query($update) or die('/* your error message here */');
-
As to your first set of code. empty($text) will return a boolean (true or false), there's no reason to compare that to "": if (empty($text) || strlen($text) < 1) { In fact, if you're going to check the string length, there's no need to check if the variable is empty at all: if (strlen($text) < 1) { As to the 2nd chunk of code, that creates an infinite loop. The first part of the if reads: if (empty($text) || !empty($text) If empty($text) is true, then the or is true, if empty($text) is false, then !empty($text) is true and the or is still true. Thus, the if is ALWAYS true (the statement is a tautology, it cannot be false). The if just runs the same function over again, which will of course result in another true, and will result in the function running again ad infinitum.
-
The extra parenthesis don't appear to be necessary per: http://php.net/manual/en/language.operators.comparison.php However, I tend to always use parenthesis myself, I think it helps to make clear the exact expression that is being evaluated. And just to warn, while ternary expressions can be great time savers and space savers, they're also a huge pain in the behind to go back and read. If you're coding something that others may have to look at, I highly suggest only using ternary expression for extremely simple evaluations, and expanding to an if statement for anything that requires even a little bit of thought.
-
We would need to see some code in order to help you.
-
Check field content depending on other field entries
ialsoagree replied to keyboarder's topic in PHP Coding Help
This isn't doing what you think it is: if(!isset($data_start) && !isset($data_end) && empty($pax) && empty($comment)){ If $data_start is set (IE. if the user did input a start), !isset($data_start) will return FALSE. Therefor, if the user inputs a start date and/or an end date, your "if" is ignored (because you have FALSE && FALSE && TRUE && TRUE which equals FALSE which means: do not follow the "then" look for an "else"). What you want is: if (!empty($data_start) && !empty($data_end) && empty($pax) && empty($comment)) { Whenever possible, it's good to conditionalize the "accepted" input, and make the default action (if all the if statements are ignored) an error message. That way if there's a mistake in your if statements, the result will usually be an error message. -
help in storing a database value to a php variable
ialsoagree replied to fsl4faisal's topic in PHP Coding Help
$select_copies = 'SELECT copies FROM book WHERE bookid= \''.$bookid.'\''; $select_result = mysql_query($select_copies, $linkID1); if ($select_result && mysql_num_rows($select_result) > 0) { $copies_array = mysql_fetch_assoc($select_result); $num_of_copies = $copies_array['copies']; // $num_of_copies is the variable that contains the number of copies } else { // Invalid $bookid, process error here } This code assumes that every $bookid is for a unique book and therefor will only return 1 or 0 results (0 results if that $bookid does not exist). That what you're looking for? -
When you say "checking the stats" are you using a PHP script to check if a new user is signed up, or are you going into the database and looking to see if a new record was created?
-
As to the identifier, check if it is set where ever you handle your session creation: session_start(); if (!isset($_SESSION['identifier']) $_SESSION['identifier'] = 1; /* Note the lack of brackets? It's okay, the 1st line after the if will only be executed when the if is true, everything after that will be executed normally. */ Before I get into the loop, I'm a bit confused. You're using $_SESSION['cushArray'] up until now, but suddenly I see $_SESSION['pillowArray'] - are these the same thing? If so, why have two names? If not, what exactly is the difference? That being said, I'm going to use 'cushArray' since that's what you've been using up until now. Also, you should break the habit now of writing strings with double quotes, and then using a period, it's redundant and makes PHP waste time (although a really small amount, never-the-less it adds up, and it's more work for the processor). $test = 'test'; echo "This is a $test"; // This is a test echo "This is a ".$test; // This is a test - but this one takes longer for PHP to parse! echo 'This is a '.$test; Now, on to the loop: foreach ($_SESSION['cushArray'] as $key => $value) { echo '<tr>'; echo '<td>'.$value['Cushion'].'</td>'; /* etc. down to where you want to have the delete form */ echo '<td><form name="delete" id="delete" enctype="multipart/formdata" method="post" action="removePillowOrder.php">\n <input name="cushionID" type="hidden" value="'.$key.'" /><input name="remove" type="submit" value="Remove" /></form></td></tr>'; // Note that I had to change all your single quotes in your HTML to double quotes // because I'm using single quotes for the PHP echo command. } In your script that processes removals, you're looking for $_POST['cushionID'] and the value of that variable will be the index you need to remove from $_SESSION['cushArray'].
-
Depends exactly on how you wind up looping, this would be my suggestion: foreach ($_SESSION['cushArray'] as $key => $value) { // Write/prepare your HTML that will let the user choose what they want to delete // $value is the variable that's the array with all your data in it, like: $value['Cushion'] or $value['Fabric'] // $key will be the numeric identifier for that particular cart item. }
-
I'd make the following changes. Firstly, in order to remove stuff from the array you're going to need to give cart items some kind of identifier. My suggestion would be to create an incrementing variable you store in your session: $_SESSION['identifier'] = 1; Then when you make your $cushArray, use this identifier as the index (and then make sure to increment the identifier so that you don't reuse it!). That brings me to my next point, whenever you're checking if the $_SESSION['cushArray'] exists, don't declare an array(array(, just use that opportunity to initialize the $_SESSION variable, this will break your need to do the assignment stuff in both the if and the else, here's an example: if(!isset($_SESSION['cushArray'])){ $_SESSION['cushArray'] = array(); }//Actually, the brackets aren't necessary here, when an "if" statement has no brackets // it will automatically only do the following line when the if statement is true, and will skip // the 1st line after the if when the if is false. $stuffToAddArray = array(Cushion=> $cushion, Fabric=> $fabric, Fill=> $fill, Button=> $button, ContWelt=> $contWelt, ContWeltFabric=> $contWeltFab, Zipper=> $zip, Quantity=> $quantity) ); $_SESSION['cushArray'][$_SESSION['identifier']] = $stuffToAddArray; $_SESSION['identifier']++; // Don't forget this step!! Notice that the else statement is entirely eliminated, and now you've shortened your code by only having to write the creation of the new array in 1 place? To delete items from the cart, you now merely have to write a script that unsets $_SESSION['cushArray'][$identifier_to_be_deleted].
-
As noted above, your problem is here: $sub = array(Cushion=> $cushion, Fabric=> $fabric, Fill=> $fill, Button=> $button, ContWelt=> $contWelt, ContWeltFabric=> $contWeltFab, Zipper=> $zip, Quantity=> $quantity ); $_SESSION["cushArray"] = $sub; The last lines tells PHP to REPLACE $_SESSION["crushArray"] with $sub. If you want to add $sub to $_SESSION["crushArray"] as a new index, use the code provided above or: $_SESSION['crushArray'][] = $sub; Just as an aside, you should use single quotes when you don't want PHP to parse a string: $test = 'test'; echo 'This is a $test'; // This is a $test echo "This is a $test"; // This is a test echo 'This is a '.$test; // This is a test
-
determining which words are searched the most
ialsoagree replied to Smudly's topic in PHP Coding Help
Just to clarify, my solution is to establish a current listing of search terms for a database that is already in use. It is not a solution that should be run repeatedly on a live server. -
determining which words are searched the most
ialsoagree replied to Smudly's topic in PHP Coding Help
$common_words = array(); // An array of words you don't want to include in the top search results.$search_count = array();$result = mysql_query('SELECT phrase FROM search', $db); // Change $db to your database variable.while ($result_array = mysql_fetch_assoc($result)) { $search_array = explode(' ', $result_array['phrase']); $search_array = array_diff($search_array, $common_words); foreach ($search_array as $value) { $search_count[$value]++; }}sort($search_count, SORT_NUMERIC);reset($search_count);for($i=0; $i < 10; $i++) { if (key($search_count)) echo key($search_count); // This is where the top $i+1'th search term is outputted. else $i=99; next($search_count);} That should do it, but it's untested. -
I'm not sure that PHP can be made to interpret images like this. Perhaps I'm just not familiar enough with image processing in PHP, but I'm not sure you're going to be able to use PHP to convert an image of a barcode with the barcode number into a text number that PHP can understand. It sounds like you need a barcode reader and software that will send the read barcodes to a PHP script as an integer or string.
-
It isn't clear what's going on. A user goes to the index page logged out. They then login using a script you haven't provided. They then get redirected... where? And are they still logged in at this point? Is "memberheader.php" a file that only has the script require_once('scripts/auth.php');? If so, why not just have require_once('scripts/auth.php'); in each of the pages that need it, and ditch memberheader.php entirely?
-
$start_00 = substr($start, 0, -2);$start_00 = $start_00.'00';$end_59 = substr($end, 0, -2);$end_59 = $end_59.'59';
-
Since there doesn't appear to be any difference in the segment of code you've provided, the problem is somewhere else in the files.
-
Well, for one thing... $email_body = "you have recived an event registration from $Surname.\n". "Here are his details:\n $message". //change the way this shit is presented ...doesn't have a colon after the closing quotation: $message". // There may be other things wrong, but that should cause a parse error.
-
You would run a select statement on your database with no where or limit clauses. Beyond that, I don't think we can help you without seeing relevant code.