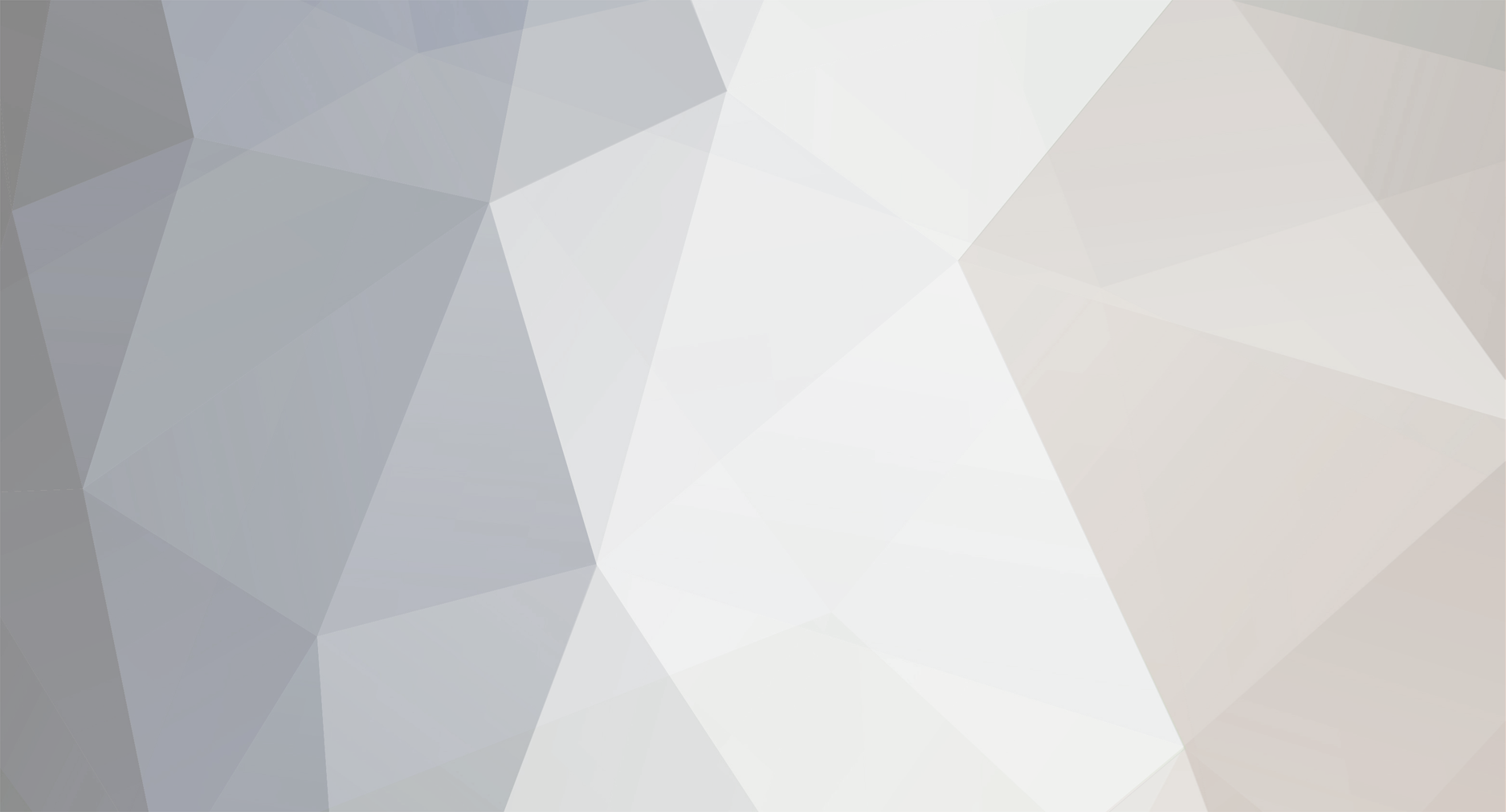
litebearer
-
Posts
2,356 -
Joined
-
Last visited
Posts posted by litebearer
-
-
Just a pre-coffee thought
update indicates the existence of a record to which one wants to add/modify.
it is good practice to populate an update form with any preexisting data
perhaps create a hidden field in the form with the id of the existing record
when processing the form, check for the value of the hidden form field, if=0 new record, if >0 update
-
having never used prepared statement, how would you simply loop thru a result set displaying one particular field from each returned row? You answer may lie there.
-
IMHO
1) store the image names, image titles and image description in a db
2) set the path to the images relative to the calling script
3) create unique names for your images using the timestamp
-
this (not tested a pure guess ) ...
$nextDate = strtotime("{$startDate} +{$weekOffset} weeks"); echo "<td width = '100'>" . date('d/m/y', $nextDate) . "</td>\n"; $startDate = date('d/m/y', $nextDate);
-
Truth be told, I 'cheated'. used excel to create a csv of the combinations. then read the csv into an array
-
perhaps an array like this? http://nstoia.com/bigarray.php
-
hint:
1. put your query in a variable
2. echo the above variable
-
the starting point is to determine how many possible combinations there are.
-
This may help -
<?PHP $w_file = "NAME_OF_YOUR_CSV_FILE"; /* put the contents into an array */ /* presumes the file does NOT contain Long,Lat,id,gName,fId,fName,fldId,fldName,fldAcers,featureID,objID,fInsu,fFSA,fBid */ $lines = file($w_file); /* loop thru the array capturing the desired info into a new array */ $x = count($lines); for($i=0; $i<$x; $i++) { $temp_data = explode(",", $lines[$i]); $data = $data . $temp_data[2] . ", " . $temp_data[3] . ", " . $temp_data[5] . "\n"; } $new_array = explode("\n", $data); echo "<PRE>"; print_r($new_array); echo "</pre>"; ?>
-
show your most recent coding
-
Did you read the last coding I posted?
$query01 = "SELECT id, salt FROM companies WHERE username = '$username'";
-
do not hash/salt the username, just the password
-
Print this out on a piece of paper and look it over carefully.
<?PHP include ('db.php'); /* set some validation variables */ $error_message = ""; /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; }else{ $username = mysql_real_escape_string(trim($_POST['username'])); } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['password'] is NOT set 2) if length of password is less than 5 3) if password has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>"; }else{ $password = trim($_POST['password']); } /* END validating password */ /* =============================================== */ /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: login.php"); exit(); } /* =============================================== */ /* =============================================== */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } /* check to see if username is in the table if not send back to login */ $query01 = "SELECT id, salt FROM companies WHERE username = '$username'"; $result01 = mysql_query($query01) or die(mysql_error()); if(mysql_num_rows($result1 != 1)) { header("Location: login.php"); exit(); } $row = mysq_fetch_array($result01); $salt = $row['salt']; $password = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $query02 = "SELECT id FROM companies WHERE username = '$username' AND password = '$hash'"; $result02 = mysql_query($query02) or die(mysql_error()); if(mysql_num_rows($result2) !=1){ /* not found send back to login */ header("Location: login.php"); exit(); } /* =============================================== */ /* success!!! send them where you want */ ?>
-
Since you have hashed the password using a salt when inserting, you also NEED to use the same hash/salt technique when checking the password on login.
ie.
1. get the salt from the table for the appropriate user
2. hash/salt the login password just like you did for the insert BUT use the salt recovered in step 1 above.
3. NOW query the table to make sure the newly hashed/salted password matches the password in the table
clear as mud?
(look at the password in the table using phpadmin. you will see what the hash/salted passwords look like. That is 'abcd' as a password will NOT be 'abcd; in the table)
-
show us the code that you used for inserting the data
-
1. as stated above remove the $ from companies, and;
2. did you by chance use any hashing etc when you originally inserted the data into the table?
-
How critical is this information to you?
How detailed do you want the information to be?
How intrusive to a user's "private" information do you feel is appropriate?
Might take at look here http://roshanbh.com.np/2008/07/getting-country-city-name-from-ip-address-in-php.html
-
perhaps seeing the code that creates the array may help
-
Not all of us do.
-
you execute the query BUT you aren't retrieving the row ie mysql_fetch_array
-
hint: look at when and where your single quotes open/close
-
easier to use mysql's date formating function
http://www.electrictoolbox.com/article/mysql/format-date-time-mysql/
-
use single quotes not backticks for your variables in you query
-
Also seriously need to validate/sanitize your post values BEFORE you attempt to use them
PHP automated gallery
in PHP Coding Help
Posted
for question 1. remove the var_dump line
for question 2. read this http://developer.loftdigital.com/blog/php-utf-8-cheatsheet