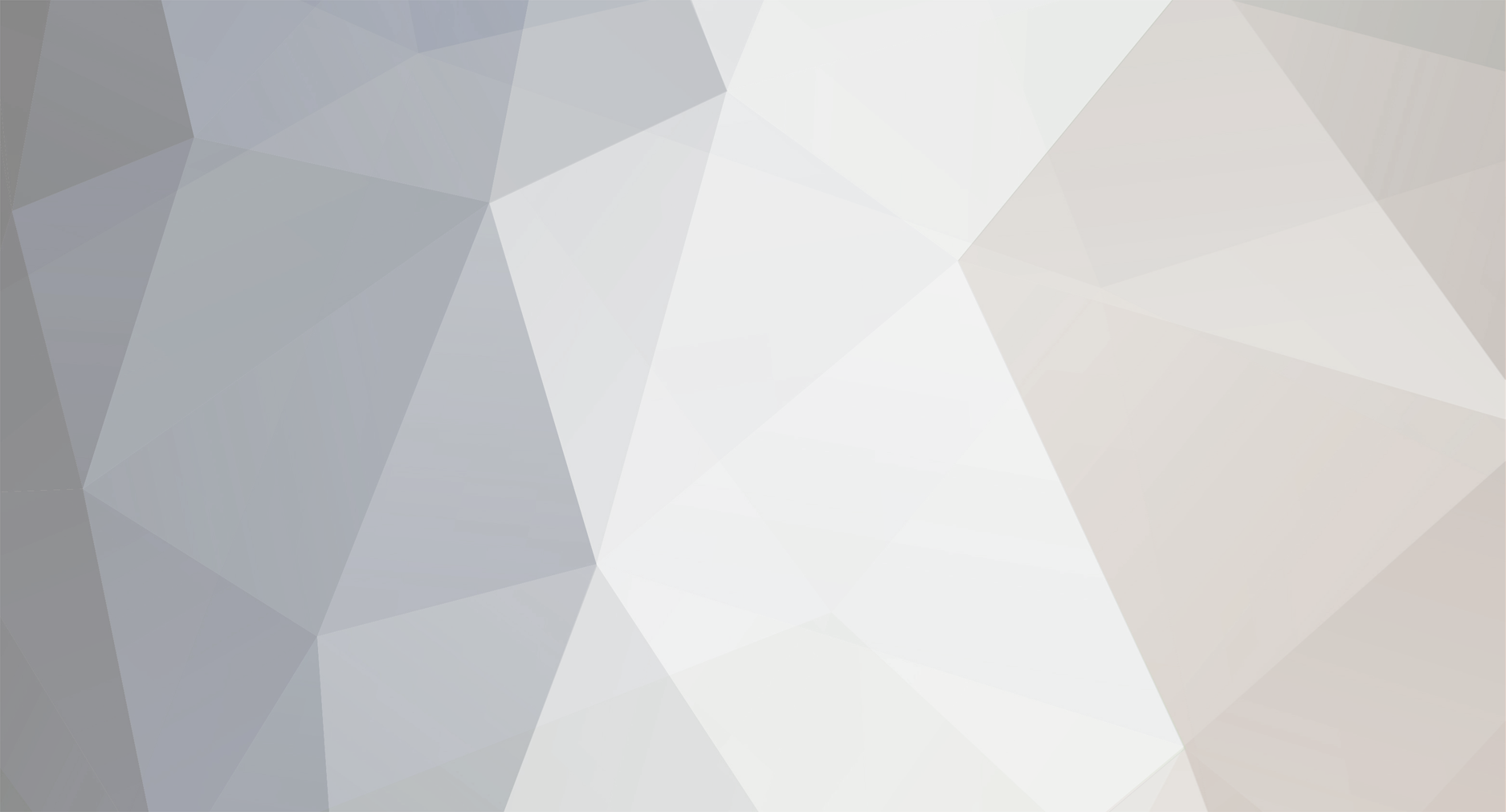
mr_bijae
New Members-
Posts
7 -
Joined
-
Last visited
Never
About mr_bijae
- Birthday 07/12/1972
Contact Methods
-
MSN
mr_bijae@att.net
-
Website URL
http://www.bijae.net
-
Yahoo
mr_bijae
Profile Information
-
Gender
Male
-
Location
AUSTIN, TX
mr_bijae's Achievements

Newbie (1/5)
0
Reputation
-
[SOLVED] String length limited to 255 characters?
mr_bijae replied to mr_bijae's topic in PHP Coding Help
Thank you, kindly! -
I just went through something very similar and found the following code to work: class XMLParser { // raw xml private $rawXML; // xml parser private $parser = null; // array returned by the xml parser private $valueArray = array(); private $keyArray = array(); // arrays for dealing with duplicate keys private $duplicateKeys = array(); // return data private $output = array(); private $status; public function XMLParser($xml){ $this->rawXML = $xml; $this->parser = xml_parser_create(); return $this->parse(); } private function parse(){ $parser = $this->parser; xml_parser_set_option($parser, XML_OPTION_CASE_FOLDING, 0); // Dont mess with my cAsE sEtTings xml_parser_set_option($parser, XML_OPTION_SKIP_WHITE, 1); // Dont bother with empty info if(!xml_parse_into_struct($parser, $this->rawXML, $this->valueArray, $this->keyArray)){ $this->status = 'error: '.xml_error_string(xml_get_error_code($parser)).' at line '.xml_get_current_line_number($parser); return false; } xml_parser_free($parser); $this->findDuplicateKeys(); // tmp array used for stacking $stack = array(); $increment = 0; foreach($this->valueArray as $val) { if($val['type'] == "open") { //if array key is duplicate then send in increment if(array_key_exists($val['tag'], $this->duplicateKeys)){ array_push($stack, $this->duplicateKeys[$val['tag']]); $this->duplicateKeys[$val['tag']]++; } else{ // else send in tag array_push($stack, $val['tag']); } } elseif($val['type'] == "close") { array_pop($stack); // reset the increment if they tag does not exists in the stack if(array_key_exists($val['tag'], $stack)){ $this->duplicateKeys[$val['tag']] = 0; } } elseif($val['type'] == "complete") { //if array key is duplicate then send in increment if(array_key_exists($val['tag'], $this->duplicateKeys)){ array_push($stack, $this->duplicateKeys[$val['tag']]); $this->duplicateKeys[$val['tag']]++; } else{ // else send in tag array_push($stack, $val['tag']); } $this->setArrayValue($this->output, $stack, $val['value']); array_pop($stack); } $increment++; } $this->status = 'success: xml was parsed'; return true; } private function findDuplicateKeys(){ for($i=0;$i < count($this->valueArray); $i++) { // duplicate keys are when two complete tags are side by side if($this->valueArray[$i]['type'] == "complete"){ if( $i+1 < count($this->valueArray) ){ if($this->valueArray[$i+1]['tag'] == $this->valueArray[$i]['tag'] && $this->valueArray[$i+1]['type'] == "complete"){ $this->duplicateKeys[$this->valueArray[$i]['tag']] = 0; } } } // also when a close tag is before an open tag and the tags are the same if($this->valueArray[$i]['type'] == "close"){ if( $i+1 < count($this->valueArray) ){ if( $this->valueArray[$i+1]['type'] == "open" && $this->valueArray[$i+1]['tag'] == $this->valueArray[$i]['tag']) $this->duplicateKeys[$this->valueArray[$i]['tag']] = 0; } } } } private function setArrayValue(&$array, $stack, $value){ if ($stack) { $key = array_shift($stack); $this->setArrayValue($array[$key], $stack, $value); return $array; } else { $array = $value; } } public function getOutput(){ return $this->output; } public function getStatus(){ return $this->status; } } It loads your XML data into an array which I pulled out and echoed back using: foreach($_POST as $k => $v){ $value = $k . $v; $value = $value . "</payload> </data> </NuanceAlarm>" ; $value = str_replace('<?xml_version\"1.0\" encoding=\"UTF-8\"?>','',$value); $times = date('Y-m-d H:i:s'); $p = new XMLParser($value); $y = $p->getOutput(); foreach($y as $a=> $b){ echo $a . "<br>"; foreach($b as $c => $d){ $i = 0; foreach($d as $e=> $f) { $field[$i] = $e; $fvalue[$i] = $f; echo $e . " : " . $f . "<br>"; $i++; } } } Note the str_replace() pulls the XML data from the $_POST variables that I receive from the HTTP POST application that I get the XML data from. Their application did not provide me with a well formatted doc type declaration so i stripped it and got the code to work great! Cheers!
-
Using PHP to protect pdf and other file types
mr_bijae replied to dlcmpls's topic in PHP Coding Help
You could handle this in a couple different ways. One would be enable the security features when you create the PDF and have the PDF doc itself contain a sercurity level that moves along with it. As for hiding where your pdf files are located, you can put them in an include file that is beyond the reach of the apache or IIS server. Have an include file named ./incpath.php and have that file declare the variable $incpath = '/usr/local/pdf'; in the above example my Apache installation is at /usr/local/apache2/htdocs and my php is retrieved by the script but there is no URL in the application that the user can grab. -
need to average values across multiple records which have the same id
mr_bijae replied to qtrimble's topic in PHP Coding Help
Without knowing your table structure I'm not able to write a specific query for you. however it should be something like this; select avg(waterquality) as quality, siteId, sample_date from quality_table group by Sample_date, siteid This will produce a query that gives one average value, per date, per site id -
need to average values across multiple records which have the same id
mr_bijae replied to qtrimble's topic in PHP Coding Help
There are arguments for both scenarios you have suggested. The primary consideration is, will PHP be the only API accessing the data? If so then you're fine building it in PHP. If you're going to have other applications getting the data from the database, then you're better off putting it in view or stored procedure. -
[SOLVED] String length limited to 255 characters?
mr_bijae replied to mr_bijae's topic in PHP Coding Help
Thanks for the reply. Here's the information: function next($row_number="") { if ((!$this->conn_id) || (!$this->result)) return false; if ($row_number=="") $row_number=$this->row_number; //return pg_fetch_array($this->result, $row_number); return mssql_fetch_array($this->result); } -
I have an application that is running PHP 5.2.5 and connecting to a MS SQL 2005 server. I ran into an issue this morning when a report that I built returned only 255 characters of a text field. A manual review of the table in the database verified that the entire 273 character string was stored in the database. I'm using a pretty simple function to get my data from the server. I've never run into this problem before, and have searched only to find that some have stored 2 billion characters in a PHP variable before. So I'm posting to you for help. Here's the entire script: $open_event = recent_closed_events(); foreach($open_event as $o) { $message = $o->message; $len = strlen($message); if($len > 200) echo "$len<br>$message"; } class events{ var $siebelacctid; var $description; var $id; var $subjectline; var $message; var $peripheraldesc; var $custid; var $dateinserted; var $serverip; var $ColorIndex; var $date_text; var $srstatus; var $moddate; var $modby; function events() { } } function recent_closed_events() { $dbmgr = new DBManager(); $sql = "select top 30 Consolidated_Alerts.Message as Message from Consolidated_Alerts"; $dbmgr->execQuery($sql); $num_rows = $dbmgr->numrows(); //echo $sql . "<p>"; $events_array = array(); if(!$num_rows) { return $events_array; } for($i = 0; $i < $num_rows; $i++) { $result = $dbmgr->next($i); $get = new events(); $get->message = $result['Message']; $events_array[$i] = $get; } return $events_array; } The above script produces results similar to this: 255 MEDIUM PNG 77004 - CCM has lost communication to the following Voice Gateway. 237026: Jan 24 02:30:22.798 UTC : %CCM_CALLMANAGER-CALLMANAGER-2-MGCPGatewayLostComm: MGCP communication to gateway lost. Device Name:wmo1rtr001.company.com App ID:Cisco Cal Has any one seen something like this before? I've verified in the database that the string is infact 300 characters in the above instance, however, PHP seems to only load 255 from the database. I'm grateful for any suggestions. Brian