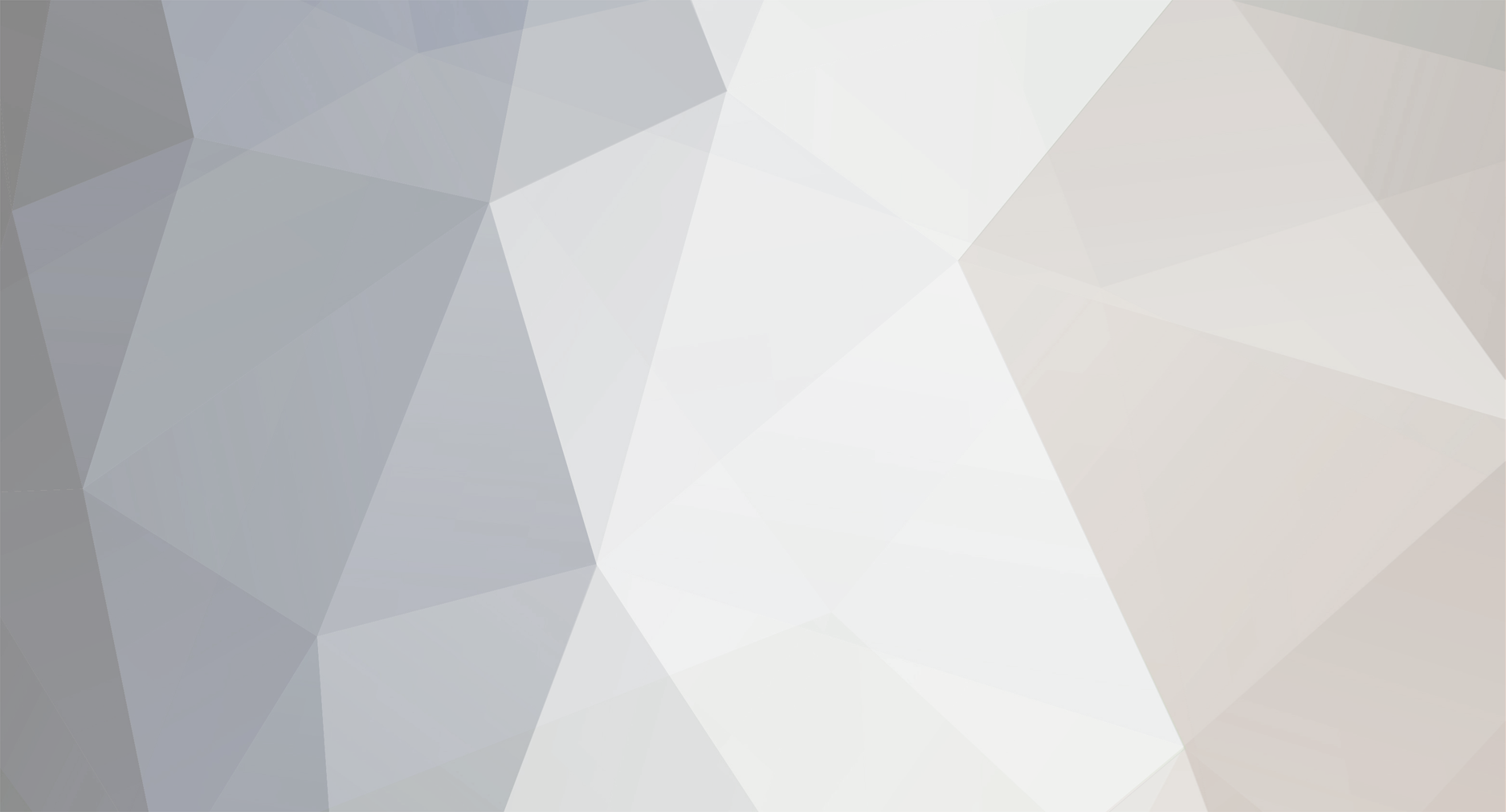
taquitosensei
Members-
Posts
676 -
Joined
-
Last visited
-
Days Won
2
Everything posted by taquitosensei
-
While Loop / Variable Access Question
taquitosensei replied to chordsoflife's topic in PHP Coding Help
I would put the number of rows before the loop $shows = mysql_query("SELECT * FROM tblConcert WHERE fkBandID = '$pk' AND fldConcertDate >= CURDATE() ORDER BY fldConcertDate", $db); $numRows = mysql_num_rows($shows); if($numRows == 0){ echo "<p>No upcoming shows for $show[fldBand]. <a href='#'>Know of one?</a></p>"; } else { while($show = mysql_fetch_array($shows, MYSQL_ASSOC)){ $date = date("F j, Y",strtotime($show[fldConcertDate])); } -
if it's a date or datetime field in MySQL it's Y-m-d H:i:s format it before you insert $date_field=date("Y-m-d", strtotime($date)); $sql="insert into yourtable(datefield) values('$date_field')";
-
Problems configuring WAMP to send emails...
taquitosensei replied to aarbrouk's topic in PHP Installation and Configuration
I would use a pre-built class for sending mail, makes it much easier, you can configure an SMTP server with authentication. I use pear's http://pear.php.net/package/Mail -
That's exactly how you do it.
-
Try this $sql = "SELECT clients.company, clients.client_id, contacts.client_id, contacts.name, contacts.contact_id, contact_coms.contact_id, contact_coms.com_content ". "FROM clients JOIN contacts on clients.client_id=contacts.client_id LEFT JOIN contact_coms on contacts.contact_id=contact_coms.contact_id where clients.ctype=2"; the easiest way to think of a left join is, you're selecting all records on the table listed to the left of "LEFT JOIN" and the table listed to the right of LEFT JOIN will show an empty record if there isn't a match.
-
If you're going to get more than 1 result. If Sect1_6 isn't a unique index. Then you need to loop through the mysqlresults. But that doesn't quite work with the header redirection. list($name, $domain) = explode("@", $email); $error = mysql_query("SELECT `Sect1_6`,`Sect1_7d`,`CompanyID` FROM tblDirectory2 WHERE `Sect1_6`='$email'") or die(mysql_error()); $errorID = mysql_fetch_array($error); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $emailcheck=$row['Sect1_6']; list($name, $domaincheck) = explode("@", $emailcheck); $postcode = strtoupper($_POST["Sect1_7d"]); $postcodecheck = strtoupper($errorID["Sect1_7d"]); if ($domaincheck == $domain || $postcodecheck == $postcode) { header("Location: /new_directory/completed.php?ID=".$errorID["CompanyID"].""); exit(); } [/code] I would also put the code exploding $email outside of the loop so that you're not exploding it every instance through the loop. If it's going to be a single result then this would probably get you close $email = $_POST["Sect1_6"]; $error = mysql_query("SELECT `Sect1_6`,`Sect1_7d`,`CompanyID` FROM tblDirectory2 WHERE `Sect1_6`='$email'") or die(mysql_error()); $errorID = mysql_fetch_array($error,MYSQL_NUM); // This gives you a numeric indexed array $emailcheck = $errorID[0]["Sect1_6"]; // notice the 0 list($name, $domain) = explode("@", $email); list($name, $domaincheck) = explode("@", $emailcheck); $postcode = strtoupper($_POST["Sect1_7d"]); $postcodecheck = strtoupper($errorID[0]["Sect1_7d"]); // Notice the 0 if ($domaincheck == $domain || $postcodecheck == $postcode) { header("Location: /new_directory/completed.php?ID=".$errorID["CompanyID"].""); exit(); }
-
That's the point of the function I posted it's recursive and checks to see if the folder exists or not.
-
They're probably tabs try str_replace("\t","", $s);
-
You're missing a step check out http://www.tizag.com/mysqlTutorial/mysqlquery.php This should help
-
use sessions at the top of each page that's not an include put session_start(); then just do $_SESSION['whatever']="whatever"; then you can access that from any page that you put the session_start on.
-
[SOLVED] Quick Question, Remove Comma
taquitosensei replied to hoopplaya4's topic in PHP Coding Help
I would do it this way <?php $sql = "SELECT * FROM tblUsers"; require("../connection.php"); $rs=mysql_db_query($DBname,$sql,$link) or die(mysql_error()); while($row = mysql_fetch_assoc($rs)) { $users[] = $row['usrID']; } echo implode(",", $users); -
Splitting a string - with a difference.
taquitosensei replied to kickstart's topic in PHP Coding Help
best way would be to write your own functions $response=myOwnSplit($string, $count, $interval); $arrays function myOwnSplit($string,$count,$interval) { $array=split(" ",$string); $counter=floor(count($array)/$count); for($a=0;$a<$counter;$a++) { $response=""; for($b=1;$b<=$interval;$b++) { $response.=$array[$a][$b]; } $responses[]=$response; } return $response; } that should get you pretty damn close. You might have to tweak it a bit. That's just off the top of my head. -
this if($getdate = "20day") { $day = 30; $sql = "SELECT * FROM comments WHERE date(CURDATE(), INTERVAL '$day' DAY) <= $todaysdate"; //date = column field in table echo $sql; } should be this if($getdate == "20day") { $day = 30; $sql = "SELECT * FROM comments WHERE date(CURDATE(), INTERVAL '$day' DAY) <= $todaysdate"; //date = column field in table echo $sql; } as a comparison the equals sign needs to be a double or if you're also comparing type it's a triple. Not sure about the syntax on the query.
-
how about posting all the code, because at some point the Name equals "-4"
-
echo out your variables and make sure they're right. Because in windows you can't create a "-4" folder. That's what causing the problem. The function I put up there would work fine if the folder name was valid. echo "Username: ".$username."<br>"; echo "Name_Artist: ".$name_artist."<br>";
-
$sql = mysql_query("SELECT * FROM consultant WHERE email_address = '$email_address'"); if (mysql_num_rows($sql) == 0) { // This is if the e-mail is not in the database } else { $error=True; $errormessage="That e-mail is already in the database"; header("Location: addconsultantform.php?error=".$error."&message=".$message); } [/code] then in addconsultantform.php check for the error and message if(isset($_GET['error']) && $_GET['error']) { echo $_GET['error']; }
-
That's fine is_dir(dirname($pathname)) || mkdir_recursive(dirname($pathname), $mode); if it's a directory or it can make directory it moves on to the next
-
Here's a function to handle this function mkdir_recursive($pathname, $mode) { is_dir(dirname($pathname)) || mkdir_recursive(dirname($pathname), $mode); return is_dir($pathname) || @mkdir($pathname, $mode); } then you would use it like this $old=umask(0); $path="users/".$username."/".$name_artist."/images/tumbs",0777); mkdir_recursive($path, 0777); umask($old);
-
[SOLVED] Whats wrong with my code? Please help
taquitosensei replied to matt.sisto's topic in PHP Coding Help
You're not passing $error in the header try this $error = $_POST['error']; header("Location: addconsultantform.php?error=".$error); otherwise your $_GET['error'] doesn't exist -
something along these lines $sql="select field1,field2 from table where date_format(datecolumn,'%Y-%m-%d') > '".date("Y-m-d", strtotime("-7 days"))."'"; would get you all the posts in the past 7 days "-1 Days" would get you yesterday "-30 Days" would get you last 30 days this would get last months $sql="select field1,field2 from table where date_format(datecolumn,'%Y-%m-%d')='".date("Y-m",strtotime("-1 Month"))."'";
-
Need some date expiration help please
taquitosensei replied to nocniagenti's topic in PHP Coding Help
Just before the if state put this echo "10 Days from now: ".date("m-d-Y",strtotime(+10 Days))."<br>"; echo "Expiration Date: ".$expire; to make sure that the expiration date variable is 10 days or 15 days whichever you're using, from today and that they're the same format. -
Need some date expiration help please
taquitosensei replied to nocniagenti's topic in PHP Coding Help
I would do this if(date("Y-m-d", strtotime("+10 days"))==date("Y-m-d", strtotime($expire))) { echo 'Renew Link'; } or +15 days -
try print_r($_POST) at the top of your page to make sure it's being posted. if it's not then make sure that your form is set to post and not get <form action='whatever.php' method='post'> double check to make sure your form opening and closing tags are in the right place
-
If you change your checkboxes to this echo "<input type='checkbox' value='' name='room[]' />$row[Room_ID]<br/> "; then it will be an array so you can do this foreach($_POST['room'] as $room) { echo $name; }
-
Try this $result_actions = mysql_query("SELECT *,actions.id as actionsid, contacts.id as contactid FROM actions JOIN contacts ON(actions.contact_id = contacts.id) WHERE date_of_reminder = '$today' AND send_reminder='Y' AND complete='N' ORDER BY contact_id"); while ($row_actions = mysql_fetch_assoc($result_actions)) { echo $row_actions["actionsid"] . $row_actions["contactid"]; }