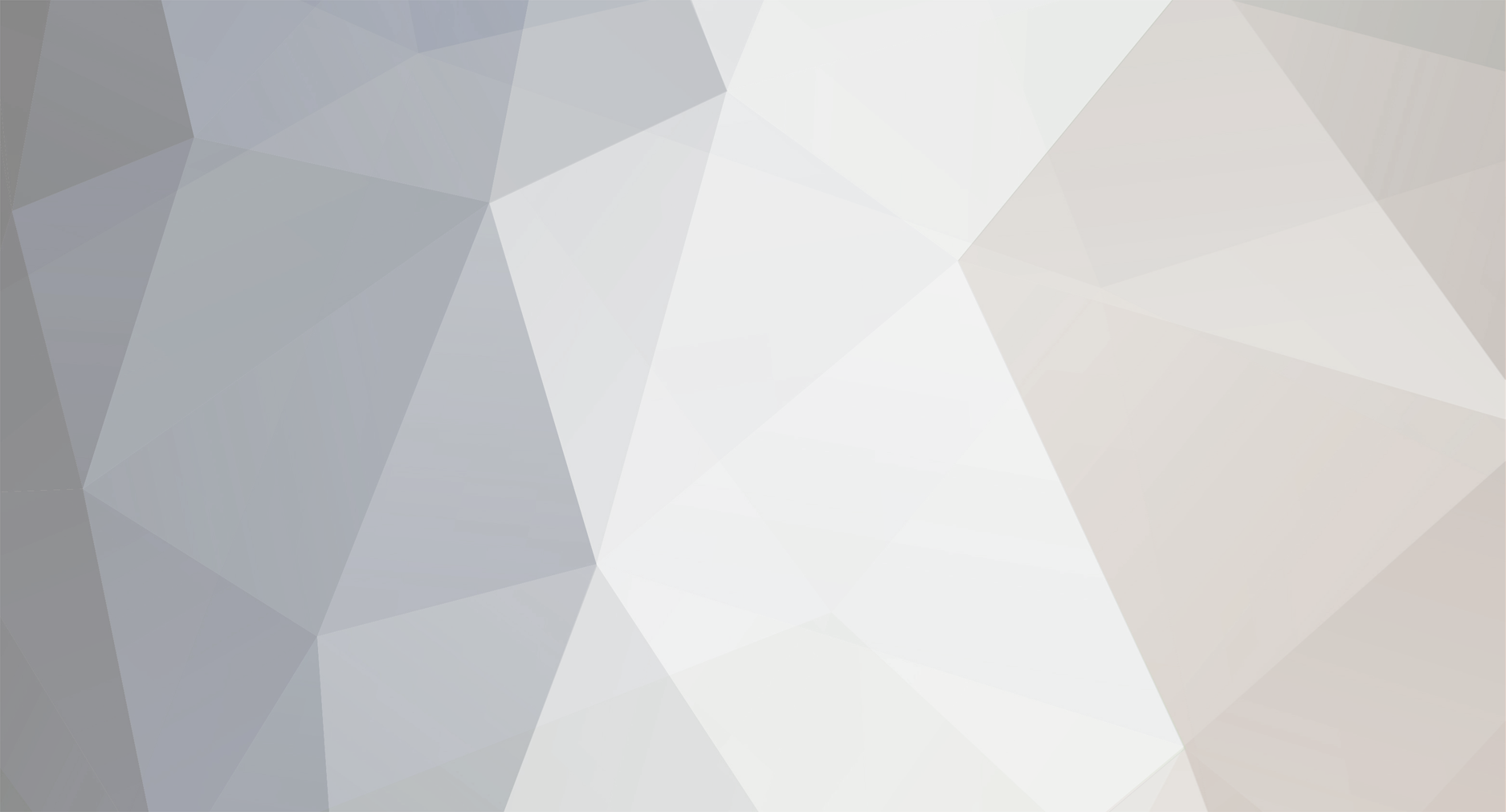
anupamsaha
Members-
Posts
251 -
Joined
-
Last visited
Never
Everything posted by anupamsaha
-
Please try this. <? include 'header.php'; if (isset($_POST['submit'])) { $avatar = $_POST["avatar"]; $quote = $_POST["quote"]; $username = $_POST["username"]; // Check whether username is already taken or not $sql = "SELECT COUNT(*) AS `user_count` FROM `grpusers` WHERE `username` = '$username' LIMIT 1"; $rs = mysql_query($sql); // If the SELECT query failed if ( $rs === FALSE ) { // Do your error handling stuff here } else { $row = mysql_fetch_assoc($rs); mysql_free_result($rs); if ($row['user_count'] > 0) { $message = "<strong>$username</strong> already exists with us. Please try a new one."; } } //insert the values if (!isset($message)){ $result = mysql_query("UPDATE `grpgusers` SET `avatar`='".$avatar."', `quote`='".$quote."', `username`='".$username."' WHERE `id`='".$user_class->id."'"); echo Message('Your preferences have been saved.'); die(); } } ?> <? if (isset($message)) { echo Message($message); } ?> <tr><td class="contenthead"> Account Preferences </td></tr> <tr><td class="contentcontent"> <form name='login' method='post'> <table width='50%' border='0' align='center' cellpadding='0' cellspacing='0'> <tr> <td height='28'><font size='2' face='verdana'>Avatar Image Location: </font></td> <td><font size='2' face='verdana'> <input type='text' name='avatar' value='<?= $user_class->avatar ?>'> </font></td> </tr> <tr> <tr> <td height='28' align="right"><font size='2' face='verdana'>Quote: </font></td> <td><font size='2' face='verdana'> <input type='text' name='quote' value='<?= $user_class->quote ?>'> </font></td> </tr> <tr> <tr> <td height='28' align="right"><font size='2' face='verdana'>Username: </font></td> <td><font size='2' face='verdana'> <input type='text' name='username' maxlength="20" value='<?= $user_class->username ?>'> </font></td> </tr> <tr> <tr> <td> </td> <td><font size='2' face='verdana'> <input type='submit' name='submit' value='Save Preferences'> </font></td> </tr> </table> </form> <? include 'footer.php'; ?>
-
Please try this. <?php $sql="SELECT * FROM category"; $result=mysql_query($sql); $options=""; while ($row=mysql_fetch_array($result)) { $catname=$row['cat_name']; //$cat_id=$row["cat_id"]; $options.="<OPTION VALUE=\"$catname\">$catname</option>"; } if (isset($_POST['list']) && is_array($_POST['list'])) { $items = implode(", ", $_POST['list']); } ?> Category: <SELECT NAME="list[]" multiple><?php echo $options?></SELECT> And, the INSERT statement will be: $sql="INSERT INTO `markers` (`name`, `street`, `city`, `state`, `zip`, `url`, `lat`, `lng`, `marker_cat`) VALUES ('$_POST[name]', '$_POST[street]', '$_POST[city]', '$_POST[state]', '$_POST[zip]', '$_POST[url]', '$_POST[lat]', '$_POST[lng]', '$items')"; It should work
-
Object Oriented Question, Abstract Vs Static Vs Singleton
anupamsaha replied to JustinK101's topic in PHP Coding Help
Well, Static classes have no instance level variables. They are best suited for utility type classes where you can group like functions together - like a String utility class. Or they are good if you want to have them as an entry point for a factory type of approach where they take care of generating the items. Singletons and Statics both provide a "global" type of entry point in terms of referencing them, but a singleton is more effective if you need to keep track of stateful items. I also asked the same question to a bunch of people and the responses were varied. Somebody had proposed the idea of stateless vs stateful as a distinction between the two. Static classes, although they can retain information about state are better off retaining information that is stateless. Singletons are better of taking care of information that is stateful. Of course, all this is just paraphasing. I hope that the article http://www.devshed.com/c/a/PHP/The-Singleton-and-Factory-Patterns-in-PHP-Building-objectoriented-forms/ will help you a lot. -
$upload_dir = realpath(dirname(__FILE__) . '/..');
-
Please change '$_POST[items]' To: $items In the INSERT query.
-
Object Oriented Question, Abstract Vs Static Vs Singleton
anupamsaha replied to JustinK101's topic in PHP Coding Help
You can go with Singleton class. It will prevent creating multiple instances inside of a script. Do not forget to prevent cloning of the class. Thanks. -
Thanks for your input... notice how I was asking for a good caching method in this situation... @dreamwest: what does that do etc? Could you explain it? Will Cache_lite help you? A good article is at: www.devshed.com/c/a/PHP/Caching-With-PHP-Cache-Lite
-
Try Eclipse with PDT. Free, lightweight and simply great. http://www.eclipse.org/pdt/downloads/
-
[SOLVED] What is the best way to let out specific data?
anupamsaha replied to redknight's topic in PHP Coding Help
You mean in data.php? That file collects in this format, so I don't see how I can use preg_match(). <a href=\x22$RefUrl\x22>$aPartOfRefUrl</a><br> Please visit http://www.phpfreaks.com/forums/index.php/board,43.0.html -
[SOLVED] What is the best way to let out specific data?
anupamsaha replied to redknight's topic in PHP Coding Help
Did you try regular expression matches through preg_match()? -
[SOLVED] POST data with ajax. But this & stops the data
anupamsaha replied to aliento's topic in PHP Coding Help
Yes. Also, read this: http://www.dangrossman.info/2007/05/25/handling-utf-8-in-javascript-php-and-non-utf8-databases/ This will answer your query. Cheers! -
You can easily do it through .htaccess file in the root. Please read: http://www.askapache.com/htaccess/apache-htaccess.html http://corz.org/serv/tricks/htaccess2.php
-
[SOLVED] POST data with ajax. But this & stops the data
anupamsaha replied to aliento's topic in PHP Coding Help
Change: var params = 'html='+html; To: var params = 'html='+escape(html); -
Try this: <?php $sql = "SELECT COUNT(*) AS `phone_count` FROM `request` WHERE `request_tel` = '" . $_POST['request_tel'] . "'"; $rs = mysql_query($sql) or die(mysql_error()); $row = mysql_fetch_assoc($rs); mysql_free_result($rs); if ( $row['phone_count'] >= 3 ) { echo 'Phone number <strong>' . $_POST['request_tel'] . '</strong> entered more then 3 times.'; } ?> Put this code above the INSERT SQL statement and do the required checks in it. Hope this will help.
-
You can do a test like this. Copy the code to "notepad" or any other plain text editor and save it as the "php" file. Run this file. If it is not showing any error, then surely, you are having an issue with the script editor that you are using.
-
The code: <html> <head> <title>Sample</title> <style type="text/css"> <!-- #idName { display: block; } --> </style> <script type="text/javascript"> <!-- function doToggleTextBox(objSelect, theId) { var obj = document.getElementById(theId); if ( objSelect.value == '2' ) { obj.style.display = 'none'; } else { obj.style.display = 'block'; } } //--> </script> </head> <body> <form id="idForm" name="fromReg" method="post" action="register.php"> <select name="items" onChange="javascript:doToggleTextBox(this, 'idName')"> <option value="1">Item 1</option> <option value="2">Item 2</option> </select> <br /> <div id="idName"><label>Enter name: <input type="text" id="Name" /></label><br /></div> <div id="idSubject"><label>Enter subject: <input type="text" id="Subject" /></label><br /></div> </form> </body> </html> The idea in the above code is, on selection of "Item 2" from the select box, the line, "Enter name" will be hidden. Does it help?
-
Also change: if ( $_GET['view'] == create ) To: if ( $_GET['view'] == 'create' ) All those lines will produce Notice internally and bypasses few lines. Always do this while you develop a script: error_reporting(E_ALL); ini_set('display_errors', '1'); ini_set('log_errors', '0'); in top of your every script.
-
Please provide your HTML / Form part that is posted to the problematic script. <?php include ("include/config.php"); echo "<font face='verdana' color='#D2D2D2'> <style type='text/css'> Body { Background: transparent; } </style> <center>"; if ( $_GET['view'] == create ) { echo " <form action='register.php?view=process' method='post'> <table border='0'> <tr> <td colspan='2' align='center'> <b><font face='verdana' color='#D2D2D2'>Registration</font></b> </tr> <tr> <td><font face='verdana' color='#D2D2D2'>Username:</font></td> <td><input type=text name=user size=20 maxlength=12></td> </tr> <tr> <td><font face='verdana' color='#D2D2D2'>Password:</font></td> <td><input type=password name=pass size=20 maxlength=20></td> </tr> <tr> <td><font face='verdana' color='#D2D2D2'>GP (millions)</font></td> <td><input type=text name=cash size=20 maxlength=4></td> </tr> <tr> <td><font face='verdana' color='#D2D2D2'>Secret Question</font></td> <td><input type=text name=question size=20 maxlength=20></td> </tr> <tr> <td><font face='verdana' color='#D2D2D2'>Secret Answer</font></td> <td><input type=text name=answer size=20 maxlength=20></td> </tr> <tr> <td colspan='2' align='center'> <b><input type=submit value=Proceed></b> </tr> </table>"; } if ( $_GET['view'] == process ) { // Gathered Information $Zuser = $_POST['user']; $pass = $_POST['pass']; $cash = $_POST['cash']; $Zquestion = $_POST['question']; $Zanswer = $_POST['answer']; // Lowercase gathered information $user = strtolower($Zuser); $question = strtolower($Zquestion); $answer = strtolower($Zanswer); // Check whether $user exists or not $sql = "SELECT `username` FROM `users` WHERE `username` = '$user'"; $rs = mysql_query($sql) or die(mysql_error()); // If number of rows returned is more than 1, it means username is duplicated if ( mysql_num_rows($rs) > 1 ) { echo "Duplicate username"; } else { mysql_query("INSERT INTO users (username, password, money, current, rank, secretquestion, secretanswer) VALUES('$user', '$pass', '$cash', '0', 'noob', '$question','$answer' ) ") or die(mysql_error()); echo "User Created!"; } } ?> I have already quoted this. First change this: if ( $_GET['view'] == process ) To: if ( $_GET['view'] == 'process' ) Also: if ( $_GET['view'] == 'create' ) All those lines will produce Notice internally, if the error messages are turned off. Always do this while you develop a script: error_reporting(E_ALL); ini_set('display_errors', '1'); ini_set('log_errors', '0'); in top of your every script.
-
Please provide your HTML / Form part that is posted to the problematic script. Also, the table structure.
-
If "delnewguy" is a submit type button in the form posted, the answer is yes, that it will tell you that the button is not clicked or not. Generally, unless it is clicked, the form will not be posted.
-
Not clear.
-
Change this: $result=mysql_query($sql, $conn); To: $result=mysql_query($sql) or die("Failed: $sql <br>Reason: " . mysql_error()); This will help understand you about the actual issue.
-
Change this: <SELECT NAME=items multiple>Category<?php echo $options?></SELECT> To <SELECT NAME="list[]" multiple>Category<?php echo $options?></SELECT> The above HTML will enable PHP to collect $list as an Array in the POST. Your code will work now. Cheers!
-
[SOLVED] How do I write this If Statement?
anupamsaha replied to webref.eu's topic in PHP Coding Help
If ($_POST['processform'] == 1 OR isset($_COOKIE['cookname'])) -
It means $results !== FALSE, i.e. whether $results contains any data or not. It does not necessarily mean that $results is true.