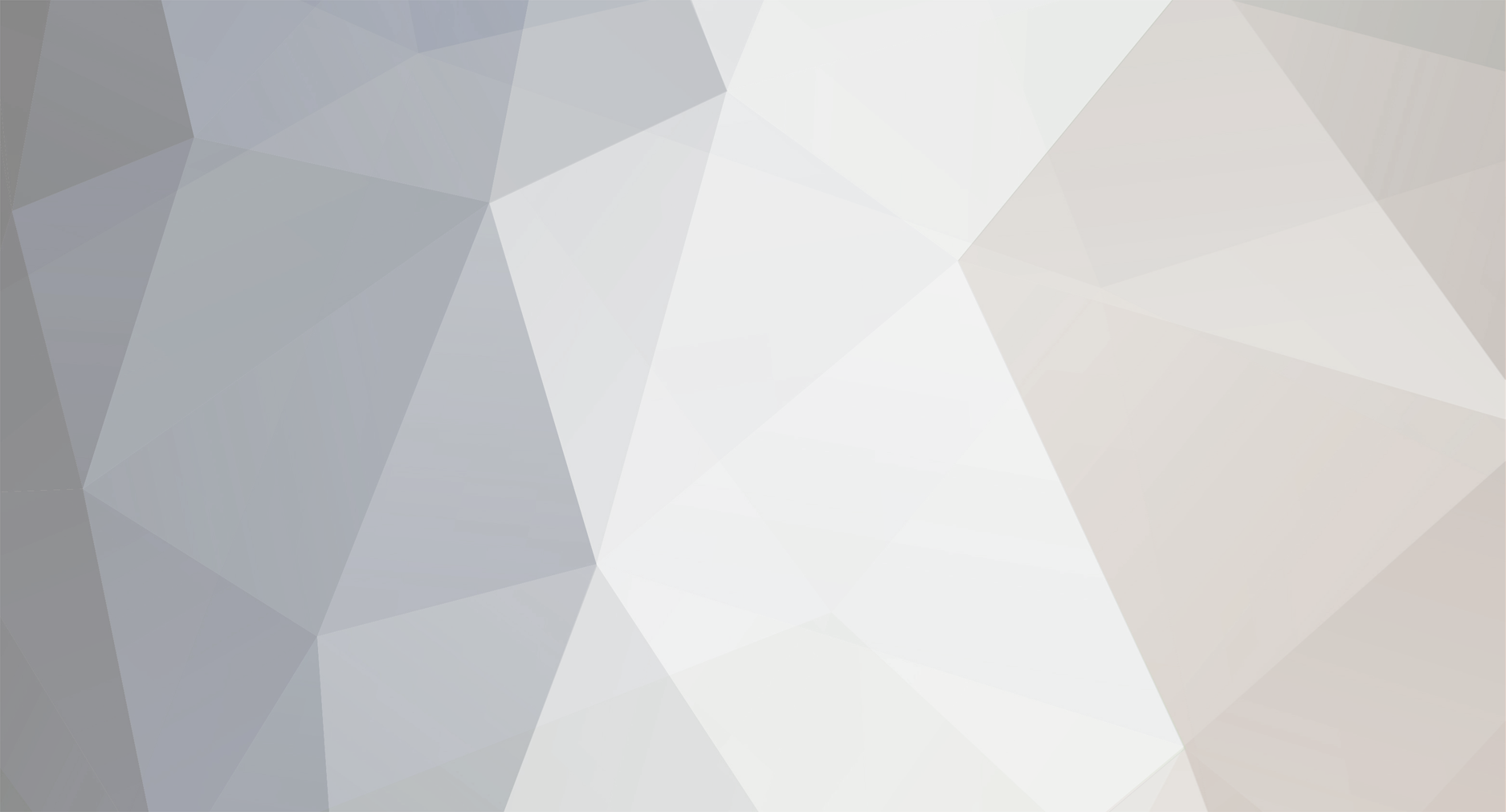
abazoskib
-
Posts
548 -
Joined
-
Last visited
Never
Posts posted by abazoskib
-
-
if ($_POST) ... will always be true, so basing any actions on this is pointless as in your processing code.
also, why are you doing this: $rank = $ranks[$row['rank']];
-
even so, check out http://php.net/manual/en/function.mail.php
you should always use the correct headers.
-
hi,
i have created a search bar for my site which records the users search data into a table.
the table name is `websmart_searches` and its structure is :
`key` bigint(20) NOT NULL auto_increment, `search` varchar(255) NOT NULL, `user` varchar(255) NOT NULL, `blocked` varchar(255) NOT NULL default 'no', `date` varchar(255) NOT NULL, `IP` varchar(255) NOT NULL, `time` varchar(255) NOT NULL,
if the user searches for "phpfreaks" it will add a row like :
INSERT INTO `websmart_searches` VALUES(6, 'phpfreaks', 'me', 'no', '27th November 2009', '192.168.1.1', '13:52pm');
duplicate entries are allowed and needed.
what im trying to do is look at the data in the table and extract out the 10 most popular searches, based on an exact match ? does anyone know if this is possible using an sql query ?
thanks in advance
dave
You could do SELECT count(*) as count,search FROM websmart_searches GROUP BY count ORDER BY count DESC LIMIT 10
Your table is highly inefficient. If you are storing both a date and a time, why are you not using a datetime column? An IP address will NEVER be 255 characters long. Neither will a time, or date, username, or whatever a `blocked` is. Even a search term would become a sentence and a half at that point. You should rethink your schema.
-
i just tested it with a url and i didnt see any errors.
-
SELECT name, email, joined FROM `users` WHERE joined BETWEEN CURDATE()- INTERVAL 51 week AND CURDATE()- INTERVAL 52 week
The query delayedinsanity posted wont work. Try the above query out.
-
no i am doing it for free for him.
-John
if you would like a copy of the script just email me: JayDesigns@live.com
i already saw a copy. i fixed your issue in your other thread.
-
Yes it can be.
-
is this a paid job?
-
you can hire someone to do this for you in the freelancing forum. otherwise do your own homework!
-
Its because your destination image is the smaller of the two.
This looks good to me:
$dst = imagecreatefromjpeg( 'http://img.youtube.com/vi/7Lfyk9yQPYk/default.jpg' ); $src = imagecreatefromjpeg( 'http://www.everyday-taichi.com/image-files/youtube-play.jpg' ); imagecopymerge( $dst, $src, 30, 20, 0, 0, 61, 44, 80 ); header( 'Content-type: image/jpeg' ); imagejpeg( $dst ); imagedestroy( $src ); imagedestroy( $dst );
-
i copy pasted what you have above, and i didnt get that error.
-
i dont see a mkdir error, but i do see a copy() error. Your path must be wrong, or like the error says the file doesnt exist.
-
i'm pretty sure its case sensitive, so try
// Get values from form. $id=$_GET['id'];
if that doesnt work, echo mysql_error() after mysql_query()
-
I removed the html and the mysql query part, but you can fill that in. It is working now. You had a loose '<form>' tag in there, which might have been the problem.
<div id="rightbottom"> <?php error_reporting(E_ALL); ini_set("display_errors", 1); if (isset($_POST['submit1'])){ $MerchantType = $_POST["MerchantType"]; $County = $_POST["County"]; print_r($_POST); }else{ ?> <form name="Results Filter" action="<?php $_SERVER['PHP_SELF']?>" method="POST"> Select Category: <select name="MerchantType"> <option value="antiques">Antiques</option> <option value="Bicycles">Bicycles</option> <option value="B+B's/Hotels">B+B's/Hotels</option> <option value="Beauty Salons/Hairdressers">Beauty Salons/Hairdressers</option> <option value="Bookshops">Bookshops</option> <option value="Bakers/Butchers">Bakers/Butchers</option> <option value="Car Parts/Garages">Car Parts/Garages</option> <option value="Clothing">Clothing</option> <option value="DIY">DIY</option> <option value="Computers/Electronics">Computers/Electronics</option> <option value="Florists">Florists</option> <option value="Furniture/Beds">Furniture/Beds</option> <option value="Groceries">Groceries</option> <option value="Garden Centres">Garden Centres</option> <option value="Gifts/Stationary">Gifts/Stationary</option> <option value="Jewellers">Jewellers</option> <option value="Kitchen/Cookware">Kitchen/Cookware</option> <option value="Model/Hobby Shops">Model/Hobby Shops</option> <option value="Music">Music</option> <option value="Newsagents">Newsagents</option> <option value="Shoe Shops/Shoe Repairs">Shoe Shops/Shoe Repairs</option> <option value="Toys and Games">Toys and Games</option> <option value="Travel Agents">Travel Agents</option> <option selected>Antiques</option> </select> County: <select name="County"> <option value="Carlow">Carlow</option> <option value="Cavan">Cavan</option> <option value="Clare">Clare</option> <option value="Cork">Cork</option> <option value="Donegal">Donegal</option> <option value="Dublin">Dublin</option> <option value="Galway">Galway</option> <option value="Kerry">Kerry</option> <option value="Kildare">Kildare</option> <option value="Laois">Laois</option> <option value="Leitrim">Leitrim</option> <option value="Limerick">Limerick</option> <option value="Longford">Longford</option> <option value="Louth">Louth</option> <option value="Meath">Meath</option> <option value="Monaghan">Monaghan</option> <option value="Offaly">Offaly</option> <option value="Roscommon">Roscommon</option> <option value="Sligo">Sligo</option> <option value="Tipperary">Tipperary</option> <option value="Waterford">Waterford</option> <option value="Westmeath">Westmeath</option> <option value="Wexford">Wexford</option> <option value="Wicklow">Wicklow</option> <option selected>Galway</option> </select> <input type="submit" value="Search" Name="submit1"> </form> <?php } ?> </div> </div> </body>
-
Array ( )
Even I in my infinate newness can tall thats not good lol.
Any one able to help as to why my form isnt POSTing? I have:
action="<?php echo $_SERVER['PHP_SELF'] ?>"
as my form action which i read was the correct thing for a form on the same page. I could be wrong though.
Anyone?
you can remove the action completely. a form's default action is itself.
also, are you using method="POST"? why dont you put post your entire form code, and the code to process it all in one post. this is like a game of tag.
-
yeah i got
Warning: gzuncompress() [function.gzuncompress]: data error in /usr/local/psa/home/vhosts/mysite.co.uk/httpdocs/testtest.php on line 35
Im guessing you didn't read the entire documentation. Somone posted a workaround for the exact problem you are having a little farther down the page.
-
Any error messages?
-
Perhaps the reason that I've never had many issues with MyISAM corruption is that I rarely ever use MyISAM unless I need fulltext indexes.
MyISAM has a bad habit of becoming corrupt from overuse. If you delete too many rows the table becomes unusable. A database table should never become corrupt from be used "too much". Also, I had a nasty index corruption involving dates which left all of my queries involving dates to return zero rows. MyISAM needs work, InnoDB is an industry standard.
-
You should really be using urlencode/urldecode for that GET variable.
-
I gave you the answer in my last post.
-
Hi abazoskib,
Thanks for your suggestions, I have implemented the username and password thing works a treat thank you!
Still struggling with the other, your suggestion unfortunately didnt work. Ive been thinking to open it then copy it from its location as its a zipped csv file to a csv file on my local server but again it need to get it open to begin with lol
Thanks for your help, Phil
Post the code you tried using, without giving away critical information like usernames passwords actual URLs etc etc
-
Hi All,
Im hoping you will be able to help me with these simple issues im having
.
First of im trying to get a file from a merchants server but the directory is protected, I know the user and pass obviously but im wondering how i can pass the login details through to the script automatically as i run the job as a cron.
Secondly i cant seem to find any information on this but i also have a gzip file im trying to open using fgetcsv, do i need to add anything to get this to work, does fopen suffice for this or is there a better function for this?
Thanks, Phil
For the user password try this: http://username:password@website.com/path/to/file
You can't directly open a gzip as far as I know. Try this out though: http://www.php.net/manual/en/function.gzuncompress.php
-
Try shell_exec(), otherwise if it is a permission problem, then you can't do anything until you have permission.
-
also knowing what my code is going to be checking is there any genuine urls that the code would refuse
or is there anything i need to know
Save data in input fields when they press "BACK BUTTON"
in PHP Coding Help
Posted
On the page where your form is, you'll have to use isset() to determine whether each of these $title = $_POST['title'];
$text = $_POST['prayer'];
$name = $_POST['name'];
is set. If it is not already set then define it as $text=''; For ea textfield you could then add $text as its value. A select drop down is a little bit trickier than that, try it out.
This should get you started: