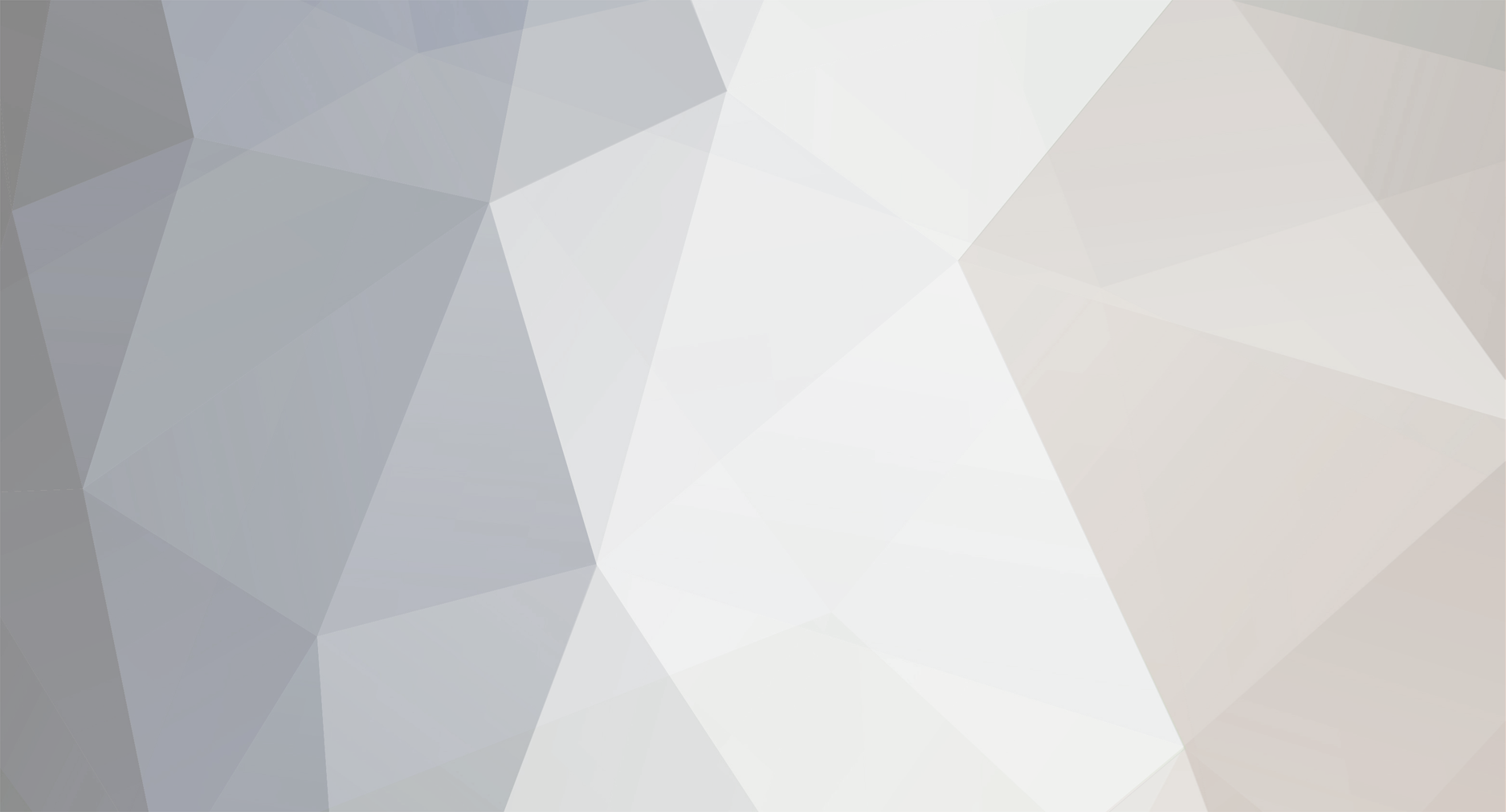
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Using array_intersect with a master array and two sub arrays?
Alex replied to CarmenH's topic in PHP Coding Help
That method is fine, but you could clean it up a bit without using all those temporary variables. $arr = array_unique( array_merge( array_intersect($arrA, $arrB), array_intersect($arrA, $arrC) ) ); -
Well, that's one way to do it, but if you utilize some of PHP's built in functions I think you can make things easier on yourself. Here's my solution to the given problem: function getEquilibriums($arr) { $equilibriums = array(); for($i = 0, $n = sizeof($arr);$i < $n;++$i) { if(array_sum(array_slice($arr, 0, $i)) == array_sum(array_slice($arr, $i + 1))) { $equilibriums[] = $i; } } return $equilibriums; } $arr = array(-7, 1, 5, 2, -4, 3, 0); print_r(getEquilibriums($arr)); It's pretty simple really. Essentially it does the same thing as yours, it just does it in a shorter procedure. It simply loops through each position in the array with the for loop and checks if the sum of the lower part equals the sum of the upper part utilizing array_sum and array_slice.
-
Yeah, you can do that but you're pattern will have to be a bit different. In regex [ and ] are special characters so you'll need to escape them. preg_match_all('~\[youtube\](.+?)\[/youtube\]~', $_POST['Post'], $matches);
-
If you don't have a choice you can validate the input the way you initially wanted to before it goes to the parser. Something like this should work: $matches = array(); preg_match_all('~.+?):~', $text, $matches); if(sizeof($matches[0]) > 10) { // too many }
-
Try: $default_smileys = Array( '' => 'smile.gif', ':-)' => 'smile.gif', '=)' => 'smile.gif', '=-)' => 'smile.gif', '' => 'frown.gif', ':-(' => 'frown.gif', '=(' => 'frown.gif', '=-(' => 'frown.gif', '' => 'bigsmile.gif', ':-D' => 'bigsmile.gif', '=D' => 'bigsmile.gif', '=-D' => 'bigsmile.gif', ''=> 'angry.gif', '>:-('=> 'angry.gif', '>=('=> 'angry.gif', '>=-('=> 'angry.gif', 'D:' => 'angry.gif', 'D-:' => 'angry.gif', 'D=' => 'angry.gif', 'D-=' => 'angry.gif', ''=> 'evil.gif', '>:-)'=> 'evil.gif', '>=)'=> 'evil.gif', '>=-)'=> 'evil.gif', '>'=> 'evil.gif', '>:-D'=> 'evil.gif', '>=D'=> 'evil.gif', '>=-D'=> 'evil.gif', '>'=> 'sneaky.gif', '>;-)'=> 'sneaky.gif', '>'=> 'sneaky.gif', '>;-D'=> 'sneaky.gif', 'O:)' => 'saint.gif', 'O:-)' => 'saint.gif', 'O=)' => 'saint.gif', 'O=-)' => 'saint.gif', '' => 'surprise.gif', ':-O' => 'surprise.gif', '=O' => 'surprise.gif', '=-O' => 'surprise.gif', ':?' => 'confuse.gif', ':-?' => 'confuse.gif', '=?' => 'confuse.gif', '=-?' => 'confuse.gif', ':s' => 'worry.gif', ':-S' => 'worry.gif', '=s' => 'worry.gif', '=-S' => 'worry.gif', ':|' => 'neutral.gif', ':-|' => 'neutral.gif', '=|' => 'neutral.gif', '=-|' => 'neutral.gif', ':I' => 'neutral.gif', ':-I' => 'neutral.gif', '=I' => 'neutral.gif', '=-I' => 'neutral.gif', ':/' => 'irritated.gif', ':-/' => 'irritated.gif', '=/' => 'irritated.gif', '=-/' => 'irritated.gif', ':\\' => 'irritated.gif', ':-\\' => 'irritated.gif', '=\\' => 'irritated.gif', '=-\\' => 'irritated.gif', '' => 'tongue.gif', ':-P' => 'tongue.gif', '=P' => 'tongue.gif', '=-P' => 'tongue.gif', 'X-P' => 'tongue.gif', '' => 'bigeyes.gif', '8-)' => 'bigeyes.gif', 'B)' => 'cool.gif', 'B-)' => 'cool.gif', '' => 'wink.gif', ';-)' => 'wink.gif', '' => 'bigwink.gif', ';-D' => 'bigwink.gif', ''=> 'anime.gif', '^^;' => 'sweatdrop.gif', '>_>'=> 'lookright.gif', '>.>' => 'lookright.gif', ''=> 'lookleft.gif', '<.<' => 'lookleft.gif', 'XD' => 'laugh.gif', 'X-D' => 'laugh.gif', '' => 'bigwink.gif', ';-D' => 'bigwink.gif', ':3' => 'smile3.gif', ':-3' => 'smile3.gif', '=3' => 'smile3.gif', '=-3' => 'smile3.gif', ';3' => 'wink3.gif', ';-3' => 'wink3.gif', '<g>' => 'teeth.gif', '<G>' => 'teeth.gif', 'o.O' => 'boggle.gif', 'O.o' => 'boggle.gif', ':blue:' => 'blue.gif', ':zzz:' => 'sleepy.gif', '<3' => 'heart.gif', ':star:' => 'star.gif', ':locked:' => 'dancinglock.gif', ':baby:' => 'baby.gif', ':hug:' => 'hug.gif', ':rofl:' => 'rofl.gif', ':sniper:' => 'sniper.gif', ':rifle:' => 'rifle.gif', ':banana:' => 'banana.gif', ':zzz:' => 'sleep4.gif', ':santa' => 'santa.gif', '' => 'cry3.gif', ':hello:' => 'hand.gif', ':love:' => 'heart2.gif', ':kiss:' => 'kuss.gif', ':blush:' => 'blush.gif', ':wallbash:' => 'wallbash.gif', ':thumbsup:' => 'thumbsup.gif', ':uglyhammer:' => 'uglyhammer.gif', ':fume:' => 'ranting.gif', ':argue:' => 'icon_argue.gif', ':uglystupid' => 'uglystupid1.gif', ':drool:' => 'drool.gif', ':angel:' => 'angel.gif', ':flamethrower:' => 'flamethrower.gif', ':hail:' => 'hail.gif', ':headscratch:' => 'headscratch.gif', ':run:'=> 'Bolt.gif', ':RUN:' => 'Bolt.gif', ':evil:' => 'devil.gif', ':cuss:' => 'cuss.gif', ':modwork:' => 'modwork.gif', ':help:' => 'help.gif', '' => 'ph34r.gif', ':mad:' => 'mad.gif', //@@ '' => 'mellow.gif', '' => 'wub.gif', '' => 'unsure.gif', '::' => '.gif', ':help:' => 'help.gif', '-->' => 'icon_pointr.gif', '<--' => 'icon_pointl.gif' ); You should have "var" before defining variables. You also had an extra comma on the end of the last element of the array.
-
What are you using to replace the text? If you're using str_replace you can use the optional 4th parameter to determine how many matches were replaced. ex: $smilies = array( ':uglyhammer:' => 'uglyhammer.png' ); $count = 0; $text = str_replace(array_keys($smilies), array_values($smilies), $text, $count); if($count > 10) { // too many smilies } else { // less than 10 smilies } Similarly, you could use preg_replace to match :anythinghere: and use the optional 3rd parameter to count the occurrences, but I think the over is a better idea because not every instance of :somethinghere: will match a smiley.
-
You can't do that with a foreach loop, but assuming both arrays are the same length you can use a for loop like so: for($i = 0;$n = sizeof($arr1);$i < $n;++$i) { echo $arr1[$i] . " - " . $arr2[$i] . "<br />\n"; }
-
Are your sure you're not accidentally running multiple queries to update the same information?
-
Regardless of if it's a homework question or not (it sure sounds like one to me..) you should try it on your own, or show us what you've tried so we can help out. Specifically you might want to look into these functions: array_sum and array_slice. Once you've shown some effort I'll post my solution
-
Those don't count towards your total. Yes they do.
-
Well I'm pretty sure that he had around 2,000 posts sometime within the last week, and those topic moved posts do show on the "Your posts" page.
-
I just realized that KingPhilip has over 8,000 posts. Last I noticed he had significantly less, somewhere around 2,000. If you view the list of his posts there's only 134 pages, at 15 per page that's about 2010. Is this a bug with SMF, or was someone messing with the database, or what?
-
my code wont insert data from form into the database
Alex replied to silverglade's topic in PHP Coding Help
Because the values you're inserting are not numeric they need quotes around them. mysql_query("INSERT INTO users (password,email) VALUES ('$Password', '$Email')"); -
my code wont insert data from form into the database
Alex replied to silverglade's topic in PHP Coding Help
Well... what does happen? You should always develop with this at the top of the page: error_reporting(E_ALL); Something could be throwing an error and you might not know. -
I'm creating a relatively small game with a flash client and I'm having some problems with the design of a certain part. In the game you have a character and you can walk around a map. It's multi-player so there are other users walking around as well. Because the game is isometric you can walk in front of and behind other users, so the order of the layers needs to be readjusted every time something moves; however, this isn't possible due to another design decision I made. Because as you walk around the game you character doesn't actually move, it's always positioned in the center, and the maps and other players move instead. I thought it would be a good idea to have 3 layers: 1. a layer for yourself, 2. A layer for the map, and 3. A layer for other players. This makes it simple because when you move the map and "other players layer" can be moved. The problem with this is that because the other players are on a separate layer from yourself you can't be positioned between 2 other users, that would require your layer to be somewhere in between the "other players layer", which just isn't possible. Anyone have any ideas on another way? I've been trying to come up with solutions for this problem for a while and tried a few different things with no success. I've also tried posting on more game oriented forums where I'd be more likely to find help, but again I haven't gotten a solution.
-
Are you sure? I was curious as to which method would be faster so I was going to do a test, and I received an "invalid use of group function" error. I also recall encountering a similar problem when attempting to use MAX() in a similar situation a while ago.
-
[SMALL ERROR] Why do I get this error? Everything is correct as I can see.
Alex replied to 3raser's topic in PHP Coding Help
"to" is a reserved keyword, you need to use back-ticks. SELECT * FROM answers WHERE `to`='$to' -
[SMALL ERROR] Why do I get this error? Everything is correct as I can see.
Alex replied to 3raser's topic in PHP Coding Help
mysql_query is returning false. Change your query to: $query = mysql_query("SELECT * FROM answers WHERE to='$to'") or trigger_error(mysql_error()); To see what's wrong. -
You can't use MAX() like that, and even if you could if you deleted a record it could potentially break the code. Instead you can try: $style = mysql_query("SELECT borderbg, bg1, bg2 FROM ".PREFIX."cup_baum ORDER BY cupID DESC LIMIT 1,1");
-
Well, first off you're not defining $row in the loop, but that doesn't even matter because you don't need a loop if there's only going to be at max 1 result returned. function getmd5($code){ include("connect.php"); $code = $code; $query = sprintf("SELECT decipher_code FROM codes WHERE md5_code = '%s'", mysql_real_escape_string($code)); $numrows = mysql_num_rows(mysql_query($query)); $result = mysql_query($query); if($numrows = 1){ return mysql_fetch_assoc($result); }else{ return false; } }
-
You can center text using PHP GD using some simple math. Here's a tutorial on it: http://www.bitrepository.com/php-how-to-center-a-text-on-an-image-using-gd.html
-
how does php interpreter work? (Trying to optimize code)
Alex replied to Goat's topic in PHP Coding Help
That's not really a good idea either. You're really not going to see much of a speed difference anyway, nothing noticeable. As Mchl said, there are other ways that you should be seeking to optimize your code. -
how does php interpreter work? (Trying to optimize code)
Alex replied to Goat's topic in PHP Coding Help
That wouldn't help; it would still interpret the included file(s) as well.