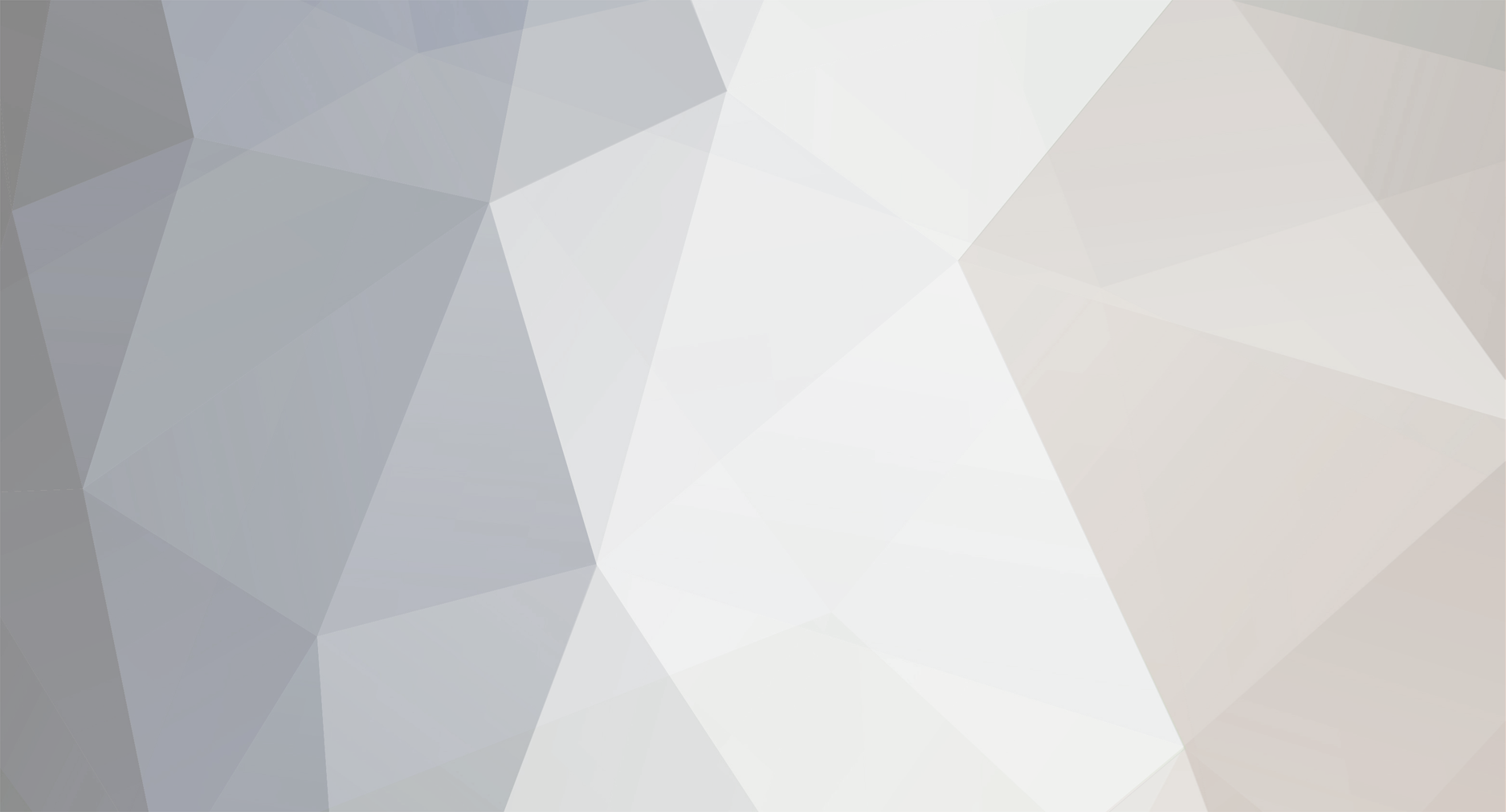
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Bleh, I should have just completely rewritten the entire thing. Bound to make mistakes when I'm working off poor logic in the first place. Anyway, thanks for pointing that out.
-
He never insulted you. Don't get so offended by code critique. Anyway, here's a rewritten copy of your script with comments added to let you know what I did, and why. <?php error_reporting(E_ALL); // Always develop with error reporting turned all the way up session_start(); //make sure this is always the very top before the opening php tag //anytime you want to use a session - this needs to be here //anytime you try and get information on a user that is logged in, make sure you start the session like this //$username = $_POST['username']; //$password = $_POST['password']; //$session = $_SESSION['user']; // No point in explicitly defining a separate variable for $_SESSION['user'], just use that. // When checking if variables are set always use isset(), this is to avoid error messages if the variable is not set. if(!isset($_SESSION['user'])) { //checks to see if the username and password are set if(!isset($_POST['username'], $_POST['password'])) // Again using isset() { //this is the basic form where it allows the users to enter in their username and password echo "You must fill out all the fields before going any further."; echo "<br/><br/><center><form action='index.php' method='POST'>Username: <input type='text' name='username'>"; echo "<br/>Password: <input type='text' name='password'><br/><input type='submit'></form></center>"; } else //if they are set, we continue { //this is the details that we use to connect to the database, make sure yours is matching - it's host, user, pass and then db mysql_connect('', '', ''); mysql_select_db(''); $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); //check to see if the user exists $sql_check_user_exist = mysql_query("SELECT username, password FROM users WHERE username='$username' AND password='$password' LIMIT 1"); //$row_cue = mysql_num_rows($sql_check_user_exist); // Again really no point in defining a variable for this unless you're using it multiple places //makes sure the user exists before trying to see if the details are correct if(mysql_num_rows($sql_check_user_exist) > 0) // You can also just use if(mysql_num_rows($sql_check_user_exist)) because any number other than 1 will evaluate to true, but this way makes things more clear { // No need for these queries, because we can already get the information from the one we did above //since the user exists, we are getting the password and username and making sure they are correct //$dbusername_sql = mysql_query("SELECT password FROM users WHERE username='$username'"); //$dbpassword_sql = mysql_query("SELECT username FROM users WHERE username='$username'"); //$get_pw = mysql_fetch_array($dbpassword_sql); //$get_user = mysql_fetch_array($dbusername_sql); $row = mysql_fetch_assoc($sql_check_user_exist); //making sure the password is the same as the password in the database, the same with the username if($row['password'] == $_POST['password'] && $row['username'] == $_POST['username']) { echo "You have successfully logged in!"; //creates the session so you can know the user and their details $_SESSION['user'] = $_POST['username']; } else { //else the details or incorrect so we give them an error echo "Sorry, the username or password is incorrect. <a href='index.php'>Back</a>"; } } else { //since no user exists with the username they typed in, we give them this message echo "Sorry, no user exists with this username. <a href='index.php'>Back</a>"; } } } else { echo "Your already logged in!"; }
-
There's an example in the manual that deals exactly with this. See "Example #3 Non-obvious Ternary Behaviour" on this page.
-
The YUI DataTable and Flexigrid support this.
-
$str = 'abc'; $arr = str_split($str); str_split
-
That's the ternary operator.
-
Warning: mysqli_query() expects parameter 1 to be mysqli, null given
Alex replied to deemurphy's topic in PHP Coding Help
You should edit that file and remove your connection details immediately. Your problem is that $mysqli is never set, you're also mixing mysql and mysqli functions, you can't do that. -
I'm curious, what was the problem? Plus, it might be helpful for anyone having the same issue in the future.
-
It's pretty self-explanatory; mail takes in 5 parameters at most, and you're supplying it with 6. Check out the manual on mail() for instructions on how to use it.
-
Yes, that's why. You should install something like WAMP locally for development purposes.
-
Quick question: How can I delete in an array like this?
Alex replied to justineaguas's topic in PHP Coding Help
If you want to delete all the elements of $_SESSION['cart']: foreach($_SESSION['cart'] as $key => $val) { unset($_SESSION['cart'][$key]); } -
I've tested the script excluding the update query and it worked fine. Try this separately: <?php $URL="http://sports.nodinelandscaping.com/phplogin_v2.3/Home.php"; header ("Location: $URL"); exit(); ?> And see if it works for you.
-
Post it here, but 430 lines is a bit much. If you can target the problem area a bit more specifically that would be appreciated.
-
Can you post your entire code? You might have a problem somewhere else. Perhaps a logical error that is preventing the header redirect from ever being called.
-
You should put that at the top of the page before anything else. Stupid question, but did you put the semicolon you were missing?
-
$items = array(); while ($item_info = mysql_fetch_array($get_item_sql)) { $items[$item_info['id']] = $item_info; } You can then access items by their id like so: echo $items[5]['price']; // echo the price of the item with the id of 5
-
Here's the blog post you're thinking of: http://www.phpfreaks.com/blog/or-die-must-die
-
You're missing a ; which should be throwing an error, if you have error reporting turned too low so that this error isn't displayed you'll see a white page. For development purposes you should always have error reporting turned on all the way: error_reporting(E_ALL); You should try: $URL="http://sports.nodinelandscaping.com/phplogin_v2.3/Home.php"; header ("Location: $URL"); exit; After a header redirect you should always call exit so that processing is stopped.
-
if(str_word_count($_POST['field'], 0) == 2) { // contained only 2 words } else { // did not contain 2 words } str_word_count If you mean two words at max eg 0, 1, or 2, change the comparison operator == to <=.
-
Quick question: How can I delete in an array like this?
Alex replied to justineaguas's topic in PHP Coding Help
You'll need to know the index of the $_SESSION['cart'] array that you're inserting into. Assuming it's, for example, 1, you can go about it like this: unset($_SESSION['cart'][1]['qty']); unset -
Warning: mysqli_query() expects parameter 1 to be mysqli, null given
Alex replied to deemurphy's topic in PHP Coding Help
After mysqli_connect put something like this to see if it's failing: if (!$mysqli) { die('Connect Error (' . mysqli_connect_errno() . ') ' . mysqli_connect_error()); } -
It comes from Jamacia You'll find it actually originated in Greenland by Ramus Lerdorf originally though of course, all because he wanted to track users to his site then a few other developers took it further and developed it into what now call version 5. He was making a joke Yeah, that's one of the main reasons people pick PHP, it has a low learning curve.
-
Warning: mysqli_query() expects parameter 1 to be mysqli, null given
Alex replied to deemurphy's topic in PHP Coding Help
We can't help much without seeing relevant code. The problem is that when using mysqli_query() in procedural style the first parameter needs to be the mysqli link. To be more specific we'll need to see some code. -
If you're including a local file it doesn't have to be downloaded at all. It just had to be read by PHP. The difference between including a 1kb and 50kb file won't be noticeable.
-
You're defining var $functions twice. First on line 23, and again on line 33.