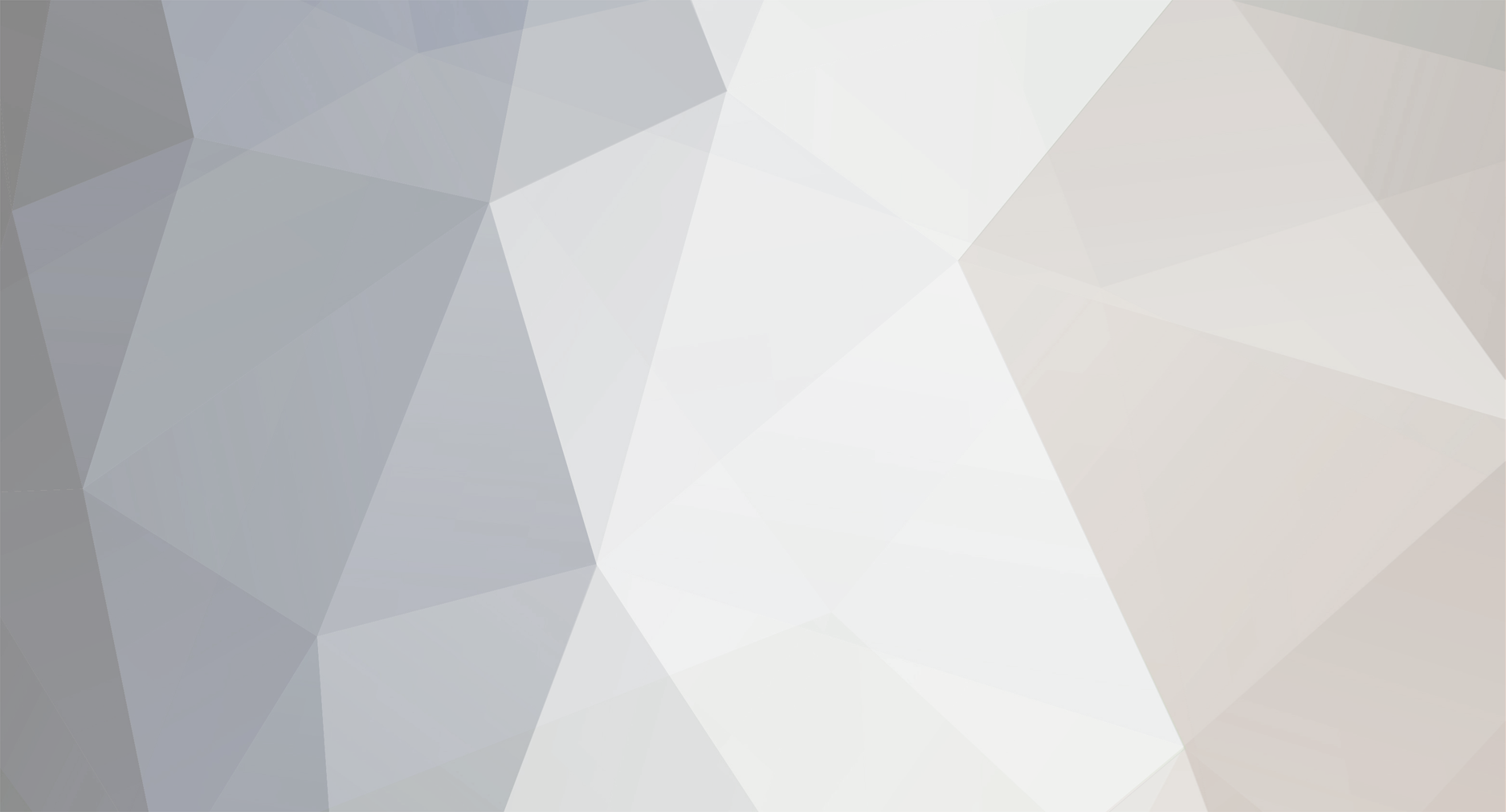
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Well with the current method if you type "comp" a row with "computer something something" should be returned. But if you want a more comprehensive search you'll have to look into more advanced techniques than just MySQL wildcards.
-
Why don't you add a "post id" column to the media table to specify which post it was uploaded in. Then when you're fetching the posts you can use a simple join query to fetch all the media in that post as well.
-
Did you bother to read any of the posts besides the first one in this topic? If you did you would know that inversesoft123 has already tried this with no avail, so it must be something else.
-
Are you sure that one of the rows contains "computer", or whatever other keyword you're trying?
-
In what ways have you tried wildcards? This is more of a MySQL question, but something like this should work: $get_items = "SELECT * FROM tablename WHERE category='$category' AND i_name LIKE '%$result%'"; Using the % wildcard, which means match 0 or more of anything.
-
First you should start off my fixing your database design. Storing multiple items in a single column usually connotes bad database design.
-
You can't truly secure them from being downloaded. If they can't download it then they can't view it.
-
You can also do it like this (using the built in function to get the extension): $allowed = array("jpg", "gif", "bmp"); if(in_array(pathinfo($path, PATHINFO_EXTENSION), $allowed)) { // valid } else { // not }
-
URL from /index.php?node=home To>>> /index?node=home using php?
Alex replied to shortysbest's topic in PHP Coding Help
This can't be done in PHP because without a properly formatted URL the request won't even get to the PHP code. Instead you need to look into mod_rewrite. -
JQuery is JavaScript, look it up.
-
You mean like this? class Foo { public $bar; public function __construct() { $this->bar = function($var) { echo $var; }; } }
-
Hm.. I was thinking of a better solution where you don't have to check any points on the line at all. What you could do is take your first point and from that through some simple calculations you can determine where on that grid box it will hit. If it hits on the vertical line then you know the next box that the line will occupy is the current box + 1 on the x scale. If it hits the horizontal line then you know that the next box is the current box + 1 on the y scale, and if it hits on the corner perfectly then the next one is +1 on both the x and y scales. You can just continue to loop through until you've reached the ending point on your line. Using this method you wouldn't have to deal with any repetitive calculations that might tell you that a point on the line occupies a box you already know.
-
If you have it available, look into exec.
-
Or use ctype_alnum which doesn't have all the overhead of the PCRE engine.
-
Imagine your graph part of a typical coordinate plane like shown in the attached image. Now we'll example line this step by step, since it's where you're confused: return $xScale[floor(abs($x))] . $yScale[floor(abs($y))]; First we'll show what $xScale and $yScale contain: $xScale = array('A', 'B', 'C', 'D', 'E'); $yScale = array(1, 2, 3, 4, 5); The values in these arrays correspond to your graph. Let's look at how the $xScale value is picked. $xScale[floor(abs($x))] First we take the absolute value of $x (|$x|). If $x is -1.5 it becomes 1.5, if it's 2.0 it stays 2.0. Then floor that value. We do this because say the value is 4.5, 4.9, or 4.65, 4.xx, ... that means that it's located somewhere in the 4th box, which is what we're trying to determine. So if the math came out and we determined that it was in box 1, that would be $xScale[1], which is B. Essentially the arrays are just lists of the names for each scale. floor(abs($x)) or floor(abs($y)) determines which box we're dealing with. The by plugging that into the corresponding array we get the name for that box. [attachment deleted by admin]
-
You can create a custom function to do this, something like this: function strpos_many($haystack, $needle) { foreach($needle as $val) { if(strpos($haystack, $val) !== false) { return true; } } return false; } What this does is check to see if any of the words in the array are found in the string. If one is, it returns true. If none are, it returns false. strpos Then use it like this: if(strpos_many($pdcD, $ignoreWords)) { $newDescription = "For further information please click <b><a href=\"$url\" target=\"_blank\">here</a></b>."; } else { $newDescription = $pdcD; }
-
Okay so here's a rough example on how you could do it: class Point { private $_x, $_y; public function __construct($x, $y) { $this->_x = $x; $this->_y = $y; } public function getX() { return $this->_x; } public function getY() { return $this->_y; } } function slope($p1, $p2) { return ($p2->getY() - $p1->getY()) / ($p2->getX() - $p1->getX()); } function getBoxAt($x, $y, $xScale, $yScale) { if($y == (int) $y && $x == (int) $x) { return; // On a line, not in a box } return $xScale[floor(abs($x))] . $yScale[floor(abs($y))]; } $xScale = range('A', 'E'); $yScale = range(1, 5); $p1 = new Point(0.5, -0.5); $p2 = new Point(1.5, -4.5); // y = mx + b // m = deltaY / deltaX $m = slope($p1, $p2); // m = -4 // b = y - mx $b = $p1->getY() - $m * $p1->getX(); // b = 1.5 // y = -4x + 1.5 $boxes = array(); for($i = $p1->getX();$i <= $p2->getX();$i += 1/8) { if(($box = getBoxAt($i, -4 * $i + 1.5, $xScale, $yScale)) !== false) { $boxes[] = $box; } } print_r(array_unique($boxes)); Output: Array ( [0] => A1 [1] => A2 [3] => A3 [4] => B3 [5] => B4 [7] => B5 ) And now I'll break everything down.. This defines a simple Point object, if you're not familiar with Object-Oriented PHP don't worry, you really don't need to know anything to understand this. All you need to know is that it represents a point, so it has an X and Y property. class Point { private $_x, $_y; public function __construct($x, $y) { $this->_x = $x; $this->_y = $y; } public function getX() { return $this->_x; } public function getY() { return $this->_y; } } This function takes 2 points as parameters and returns the slope of the resulting line they form. function slope($p1, $p2) { return ($p2->getY() - $p1->getY()) / ($p2->getX() - $p1->getX()); } This function will take standard graph points and determine which box on your graph it would fall in. function getBoxAt($x, $y, $xScale, $yScale) { if($y == (int) $y && $x == (int) $x) { return; // On a line, not in a box } return $xScale[floor(abs($x))] . $yScale[floor(abs($y))]; } Here we define and create the two points we're dealing with. $p1 = new Point(0.5, -0.5); $p2 = new Point(1.5, -4.5); We know that the standard equation for a line is y = mx + b, so now, given the two points, we need to figure out the 2 variables in this equation, m (slope) and b (y-intercept). Now we simply plug in our 2 points into our slope function that we declared earlier to easily determine the slope between the tow points, we find that m = -4, we declare that to be the value of $m. // y = mx + b // m = deltaY / deltaX $m = slope($p1, $p2); // m = -4 Now we find b, the y-intercept value. To do this we simply plug in x, y (both taken from one of the two points we were given) and m, the slope we already determined. We find b = 1.5. // b = y - mx $b = $p1->getY() - $m * $p1->getX(); // b = 1.5 Now we have the equation y = -4x + 1.5 for our line. Now what we want to do is take this equation and find out all possible boxes that the points of this line fall in, that are within the small segment we have (between point 1 and point 2). We start the loop at our first point, and go to the second point incrementing the X by 1/8 each time (this increment will depend on the slope of the line you're dealing with). Within this loop we call the getBoxAt function we defined earlier on each point to determine which point that box lands on in your graph. After checking to make sure the function didn't result false (if it did return false it means that it did not land in a box, but rather directly on a line) and store it in the $boxes array. After all this we print_r out our array after calling array_unique on it to ensure that no box is listed twice. $boxes = array(); for($i = $p1->getX();$i <= $p2->getX();$i += 1/8) { if(($box = getBoxAt($i, -4 * $i + 1.5, $xScale, $yScale)) !== false) { $boxes[] = $box; } } print_r(array_unique($boxes));
-
You have curly brackets around the table name that you shouldn't, try: $result1 = pager_query(db_rewrite_sql("SELECT n.nid, n.created FROM node n WHERE n.type = '$content_type' AND n.status = 1 ORDER BY n.created DESC LIMIT 3"));
-
Add a limit clause to the query: $result1 = pager_query(db_rewrite_sql("SELECT n.nid, n.created FROM {node} n WHERE n.type = '$content_type' AND n.status = 1 ORDER BY n.created DESC LIMIT 3"));
-
Yeah, I should have explained the code in my post, sorry about that. I was a little busy at the moment. gizmola pretty much covered everything
-
$student_name isn't a property of the Student class. I'm not sure but I think you want to do something like this: class Student extends Classroom { static public $student_count = 0; private $_student_name; public function __construct($student_name) { $this->_student_name = $student_name; echo "Hello {$this->_student_name}! <br />"; parent::__construct(); echo "The number of students in your class is: " . parent::$class_attendance . "<br /><br />"; self::$student_count++; } public function getName() { return $this->_student_name; } } $student1 = new Student('sfc'); echo $student1->getName(); By the way, your hierarchy doesn't seem to make sense. Why should student extend Classroom? If you want help with your logic with that as well post the class definition for Classroom.
-
Cookies are domain specific. If you set them using the domain, then use the ip address to access the website to see if they're being cleared it's only giving you the illusion that they're being cleared on the same request because they were never set. That's my best guess at least, I'm not entirely sure I understand your situation.
-
If more than 255 characters echo 255 characters then "..."
Alex replied to dezkit's topic in PHP Coding Help
if(strlen($str) > 255) { $str = substr($str, 0, 255) . '...'; } substr -
Are you sure that 1. You're looking at the correct row and 2. You've spelled the column name correctly?