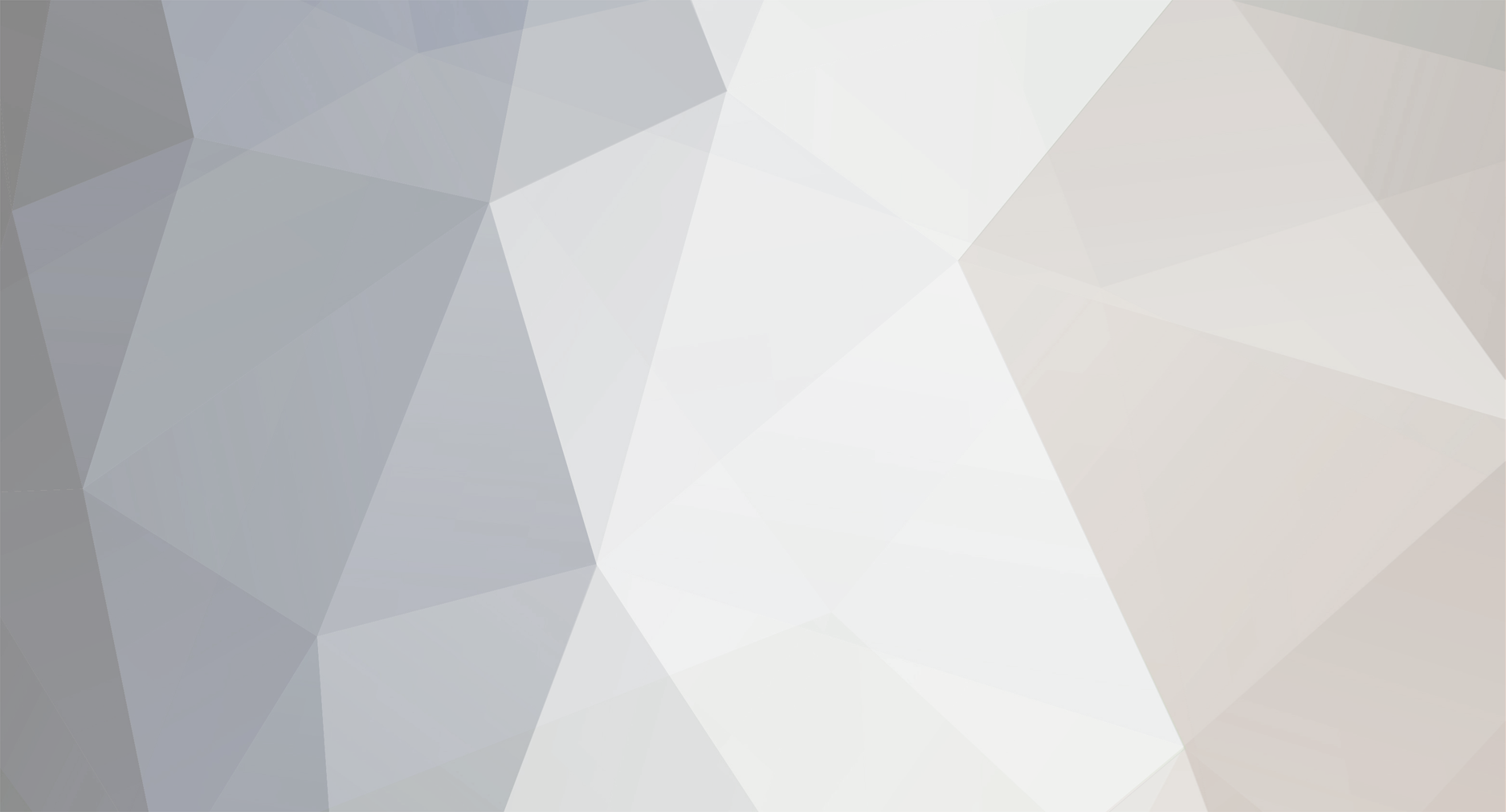
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Warning: mysql_num_rows() expects parameter 1 to be resource, boolean given
Alex replied to Jragon's topic in PHP Coding Help
You're getting that error because mysql_query is returning boolean false as a result of your query failing. You need to use quotes, not back ticks. $login = sprintf("SELECT * FROM user WHERE username='%s' AND password = '%s'", mysql_real_escape_string($post_username), mysql_real_escape_string($post_password)); -
Automatically not accept empty entries or delete empty entries on table
Alex replied to xerox02's topic in PHP Coding Help
Your code could look something like: $con = mysql_connect("","",""); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("my_database", $con); require_once('recaptchalib.php'); $privatekey = "private_key"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { // What happens when the CAPTCHA was entered incorrectly die ("The reCAPTCHA wasn't entered correctly. Go back and try it again."); } else { if(empty($_POST['quotes'])) { // Tell them they missed a required field } else { $sql="INSERT INTO pendingquotes (quotes, email, name) VALUES ('$_POST[quotes]','$_POST[email]','$_POST[name]')"; mysql_query($sql,$con); echo 'Thanks for your submission '; mysql_close($con); } } -
fputcsv is a built in PHP function, you can't declare a function of that name.
-
Automatically not accept empty entries or delete empty entries on table
Alex replied to xerox02's topic in PHP Coding Help
You can validate your input with a function like empty and not submit it to the database if it is empty. For more specific help we'll need to see relevant code. -
You must have output somewhere else before you're doing this. The hash you should get is: e85ea73176c48d8723fb18211c4a9499 Which is in the string you're getting: lujxikk46nx7l2m94e85ea73176c48d8723fb18211c4a9499. The first part must be coming from somewhere else.
-
I think you mean OR instead of AND.
-
Take a look at imagegrabwindow.
-
What version of PHP are you running (you can check this by using phpinfo)?. As you can see from the manual on sha1() it requires PHP 4.3.0+.
-
That's referring to this in the for loop: $i >= $_POST['times']
-
Your script is relying on register_globals. You should instead be accessing the data from the $_POST array. So $times becomes $_POST['times']. Additionally you have a flaw with your logic, the comparison operator you should be using is < not >=. if(isset($_POST['submit'])){ for($i=0; $i < $_POST['times']; $i++){ echo $_POST['text']; echo '<br />'; }
-
You need to put quotes around data that isn't being inserted into a numeric typed column. mysql_query("INSERT INTO subscriptions (email, confirmation number, verified) VALUES ('$email', $confirmation_number, 'no')") or die('Error'); Depending on the data type, you might need to put quotes around $confirmation_number as well, because the variable name is $confirmation_number I assumed it's being inserted into a column with a numeric data type.
-
$img = imagecreatefromjpeg("image2.jpg"); $rgb = imagecolorat($img, $x, $y); // replace $x and $y here to be the point you want.. imagecolorset($img, $rgb, 0, 0, 255); header('Content-type: image/jpeg'); imagejpeg($img, 'simpleimg.jpg');
-
It should work as long as you're inputting the correct information. The script should look something like this: $img = imagecreatefromjpeg("image2.jpg"); $rgb = imagecolorat($img, $x, $y); // replace $x and $y here to be the point you want.. imagecolorset($img, $rgb, 0, 0, 255); header('Content-type: image/jpeg'); imagejpeg($img); That would take image2.jpg and take whatever color is at point ($x, $y) and replace it throughout the whole image with blue, and then display it in the browser.
-
Did you change the $x and $y to the x and y of the pixel containing the color that you want to replace?
-
That code will loop through every color and change that color to blue. Making the entire image blue. Try something like: $img = imagecreatefromjpeg("image2.jpg"); $rgb = imagecolorat($img, $x, $y); // replace $x and $y here to be the point you want.. imagecolorset($img, $rgb, 0, 0, 255); You're also not outputting the new image to see the changes. Add this to the end of the script: header('Content-type: image/jpeg'); imagejpeg($img); imagejpeg
-
Echo Mysql row without repeating; Distinct doesn't seem to work.
Alex replied to xerox02's topic in MySQL Help
Oh sorry, I thought you meant other columns.. To loop through all the rows returned you can do this (you were already provided with this in another topic..): while($row = mysql_fetch_assoc($result)) { echo $row['id']. " - ". $row['quotes'] . "<br />"; } -
What do you mean? I don't see an argument for changing the quality :/ EDIT: nevermind found it, but it requires you to save the file. I don't need to save the file. That's not true: You simply pass null as the second parameter, something like this: imagepng($im, null, 0);
-
Echo Mysql row without repeating; Distinct doesn't seem to work.
Alex replied to xerox02's topic in MySQL Help
echo $row['row name']; But you need to make sure you add it to your select query as well. -
The function is get_class_vars not get_class_variables(), but this won't work for what you're looking for. This gets the default values for a class given the name of the class, not an instance of the class. Instead use get_object_vars.
-
Using the image*() function (depending on your format, png, jpeg, etc.. whichever one you're using) you can specify the quality of the image. Trying increases the quality.
-
Ah, if the data is ordered that way then yeah, that's a better solution.
-
You forgot to take into account that he wants the largest day value to be used if multiple exist.
-
From the sounds of your problem it definitely seems like it can and should be solved through MySQL. You can try: SELECT name, MAX(days) as max_days FROM table GROUP BY name In the case that I'm misunderstanding and this really can't be achieved through MySQL here's a PHP solution: $arr = array( array( 'name' => 'george', 'days' => 20 ), array( 'name' => 'george', 'days' => 10 ), array( 'name' => 'nickie', 'days' => 20 ) ); function getNameIndex($arr, $name) { foreach($arr as $key => $val) { if($val['name'] == $name) { return $key; } } return false; } $new = array(); foreach($arr as $key => $val) { if(($index = getNameIndex($new, $val['name'])) !== false) { if($new[$index]['days'] < $val['days']) { $new[$index]['days'] = $val['days']; } } else { $new[] = $val; } } echo "<pre>" . print_r($new, true) . "</pre>"; Output: Array ( [0] => Array ( [name] => george [days] => 20 ) [1] => Array ( [name] => nickie [days] => 20 ) )