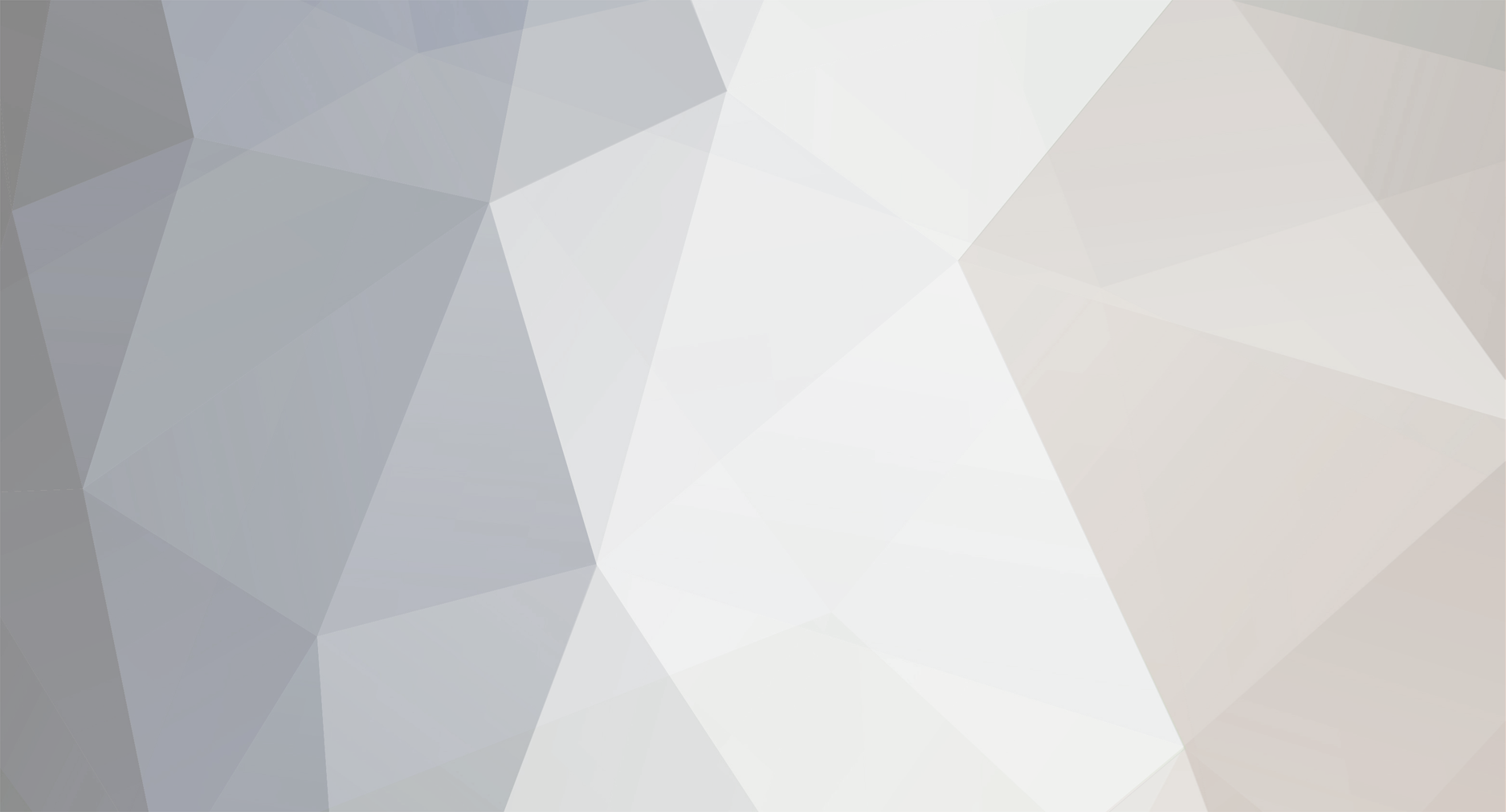
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
<?php $a = '1 2 3 4 5 6 7 8 9 10 11 12 13 14'; $split = explode(' ', $a); for($i = 0;$i < count($split);$i += 5) $arrays[] = array_slice($split, $i, 5); then $arrays will contain all the arrays that you want. So $arrays[0] would be an array containing the numbers 1-5, etc..
-
$comment = mysql_real_escape_string(strip_tags(htmlentities(preg_replace('/[^A-Za-z0-9\s]/', '', $_POST['comment']))));
-
He was talking about PHP GD Library. I'm sure you can find more than adequate examples there. Especially here.
-
You're asking for the value, he gave you the way to get the value. You're asking the question wrong, so expect a wrong answer. Simply put, you can't do what you want. Why don't you just make the value "Dogs" if that's what you want?
-
Just preform the same functions on the data before inserting it into the database. You should also be using mysql_real_escape_string() on all data being passed into mysql_query().
-
http://livedocs.adobe.com/flash/9.0/ActionScriptLangRefV3/
-
Np. Just make sure to mark the topic as solved, there's a button on the bottom left.
-
Use isset(). if(isset($_SESSION['myusername'])) echo "logout";
-
You're trying to echo a mysql resource. echo $sql;
-
You could store the number of users redirected in a flat-file. $num = file_get_contents('counter.txt'); file_put_contents('counter.txt', $num + 1); if($num <= 50) header('Location: a.html'); else header('Location: b.html');
-
That's an error returned by class; are you sure you have PHP GD installed on your server?
-
Your problem is that you're not closing your first form: <form action = "'.$_SERVER['PHP_SELF'].'" method="post"> with </form>. So that's the action being used. By the way, it's a bad practice to use $_SERVER['PHP_SELF'] for form actions as it leaves you open for an XSS attack. Rather, if you want a form to be submitted back to the same page just leave action blank (action="").
-
The standard or 'correct' way to do it is to use getters and setters. The use of getters and setters, and many other programming standards, isn't necessary for you. It's for other programmers. Other people using your code shouldn't have to understand how everything works internally. They should just have to understand what method X, and what method Y does. Even though you're not going to be sharing your code with other programmers it's good to stick to a constant way of doing things, it will only help you. If you come back to this class 6 months later are you going to completely remember exactly how everything works? Chances are you won't, so it's better to make things easy to use.
-
Do you mean like a slideshow? Because you can rotate an image resource by using imagerotate(). Either way I don't think creating the slideshow is the problem here. Anyway, I created an Image Manipulation Class that can do what you want easily. I never really 'finished' it though, I just add stuff here and there. class Image { private $_image, $_ext, $_file; const CENTER = 0, CENTER_LEFT = 1, CENTER_RIGHT = 2, TOP_CENTER = 3, TOP_LEFT = 4, TOP_RIGHT = 5, BOTTOM_CENTER = 6, BOTTOM_LEFT = 7, BOTTOM_RIGHT = 8; public function __construct($im=NULL) { if(empty($im)) return true; $info = @getimagesize($im); if(!$info) $this->throw_error("'$im' is not a valid image resource"); $this->_ext = end(explode('/', $info['mime'])); $func = 'imagecreatefrom' . $this->_ext; $this->_image = @$func($im); return $this->_file = $im; } public function set($im) { $info = @getimagesize($this->_file); if(!$info) $this->throw_error("'$im' is not a valid image resource"); $this->_ext = end(explode('/', $info['mime'])); $func = 'imagecreatefrom' . $this->_ext; $this->_image = @$func($im); return $this->_file = $im; } public function resize($width, $height, $porportional=FALSE, $percent=NULL, $max=NULL) { if(empty($this->_image)) $this->throw_error('Invalid call to Image()->resize, no image is set'); $info = Array(imagesx($this->_image), imagesy($this->_image)); if($porportional) { if(!empty($percent)) { $new_width = $info[0] * ($percent/100); $new_height = $info[1] * ($percent/100); } else if(!empty($max)) { if($info[0] < $max && $info[1] < $max) return false; $new_width = ($info[0] > $info[1]) ? $max : ($info[0]/$info[1]) * $max; $new_height = ($info[0] > $info[1]) ? ($info[1]/$info[0]) * $max : $max; } else { if(!empty($width) && !empty($height) || empty($width) && empty($height)) return false; $new_width = (!empty($width)) ? $width : $height/$info[1]*$info[0]; $new_height = (!empty($height)) ? $height : $width/$info[0]*$info[1]; } } else { $new_width = $width; $new_height = $height; } $new_image = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($new_image, $this->_image, 0, 0, 0, 0, $new_width, $new_height, $info[0], $info[1]); return $this->_image = $new_image; } public function crop($width, $height, $x, $y, $position=NULL) { if(empty($this->_image)) $this->throw_error('Invalid call to Image()->crop, no image is set'); $info = Array(imagesx($this->_image), imagesy($this->_image)); if($width > $info[0] || $height > $info[1]) return false; switch($position) { case Image::CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height)/2; break; case Image::CENTER_LEFT: $x = 0; $y = ($info[1] - $height)/2; break; case Image::CENTER_RIGHT: $x = ($info[0] - $height); $y = ($info[1] - $height)/2; break; case Image::TOP_CENTER: $x = ($info[0] - $width)/2; $y = 0; break; case Image::TOP_LEFT: $x = 0; $y = 0; break; case Image::TOP_RIGHT: $x = ($info[0] - $width); $y = 0; break; case Image::BOTTOM_CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height); break; case Image::BOTTOM_LEFT: $x = 0; $y = ($info[1] - $height); break; case Image::BOTTOM_RIGHT: $x = ($info[0] - $width); $y = ($info[1] - $height); break; default: break; } $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->_image, 0, 0, $x, $y, $width, $height, $width, $height); return $this->_image = $new_image; } public function watermark($wm, $position=NULL, $alpha=50) { if(empty($this->_image)) $this->throw_error('Invalid call to Image()->crop, no image is set'); $info = @getimagesize($wm); if(!$info) $this->throw_error("'$wm' (watermark) is not a valid image resource"); elseif(end(explode('/', $info['mime'])) != $this->_ext) $this->throw_error("The watermark must have the same mime type as the base image"); $func = 'imagecreatefrom' . end(explode('/', $info['mime'])); $wmr = $func($wm); $info = Array(imagesx($this->_image), imagesy($this->_image)); $wm_info = Array(imagesx($wmr), imagesy($wmr)); switch($position) { case Image::CENTER: $x = ($info[0] - $wm_info[0]) / 2; $y = ($info[1] - $wm_info[1]) / 2; break; case Image::CENTER_LEFT: $x = 0; $y = ($info[1] - $wm_info[1]) / 2; break; case Image::CENTER_RIGHT: $x = $info[0] - $wm_info[1]; $y = ($info[1] - $wm_info[1]) / 2; break; case Image::TOP_CENTER: $x = ($info[0] - $wm_info[0]) / 2; $y = 0; break; case Image::TOP_LEFT: $x = 0; $y = 0; break; case Image::TOP_RIGHT: $x = ($info[0] - $wm_info[0]); $y = 0; break; case Image::BOTTOM_CENTER: $x = ($info[0] - $wm_info[0]) / 2; $y = ($info[1] - $wm_info[1]); break; case Image::BOTTOM_LEFT: $x = 0; $y = ($info[1] - $wm_info[1]); break; case Image::BOTTOM_RIGHT: $x = ($info[0] - $wm_info[0]); $y = ($info[1] - $wm_info[1]); break; default: $x = ($info[0] - $wm_info[0]); $y = ($info[1] - $wm_info[1]); break; } return imagecopymerge($this->_image, $wmr, $x, $y, 0, 0, $wm_info[0], $wm_info[1], $alpha); } public function save($loc, $compression=100) { if(empty($this->_image)) $this->throw_error('Invalid call to Image()->save, no image is set'); $func = 'image' . $this->_ext; return $func($this->_image, $loc, $compression); } public function output() { if(empty($this->_image)) $this->throw_error('Invalid call to Image()->output, no image is set'); header('Content-type: image/' . $this->_ext); $func = 'image' . $this->_ext; $func($this->_image); } private function throw_error($err) { $backtrace = debug_backtrace(); die('<b>Fatal error:</b> ' . $err . ' <b>on line ' . $backtrace[1]['line'] . ' [' . $backtrace[1]['file'] . ']</b>'); } } To do what you want here's an example: <?php require_once 'image.class.php'; $image = new Image('http://thewalkingtree.files.wordpress.com/2009/07/children-1.jpg'); $image->resize(900, null, true); $image->crop(900, 250, null, null, Image::CENTER); $image->output(); //$image->save('path/to/new/location'); ?> If you test this you'll notice it outputs exactly what you posted in your example.
-
[SOLVED] "rtrim" specific length from sentence
Alex replied to salman_ahad@yahoo.com's topic in PHP Coding Help
I can't speak for your specific case, but it seems more logical that you limit the amount of characters starting from the left. If you have strings that vary in length then you'll have different results. Wouldn't you want to have the strings with the 'Read more..' be the same length all the time? At least that's normally how it is. -
[SOLVED] "rtrim" specific length from sentence
Alex replied to salman_ahad@yahoo.com's topic in PHP Coding Help
Then you should go with substr() as Thorpe said. ex echo substr($sentence, 0, $a) . ' Read more...'; The problem with that is it will cut in the middle of words. Alternatively to get around this you can use this: echo current(explode("\n", wordwrap($sentence, $a))) . ' Read more...'; wordwrap() -
[SOLVED] "rtrim" specific length from sentence
Alex replied to salman_ahad@yahoo.com's topic in PHP Coding Help
Do you want to preform rtrim on characters on the right of the sentence or do you want to completely remove them? -
You still need to wrap your PHP inside included files with appropriate php tags. <?php $phone = "1.877.894.8555"; $email = "info [at] mobirecycle.com"; ?> Otherwise it'll be read like any other file, not parsed by PHP, so those variables are never stored.
-
Add a name the name 'submit' to your button. Then wrap the foreach statement inside of a isset() conditional, like.. if(isset($_POST['submit'])) { // Foreach here.. }
-
Alternatively you can use file_put_contents() which essentially does the same as the 3 functions that haku stated, just in 1 step making things easier for you.
-
[SOLVED] Page refresh as part of a function
Alex replied to Errant_Shadow's topic in PHP Coding Help
You can use a meta refresh. -
What error are you getting?
-
So basically you're wanting to put in a phrase like 'Adobe Software' and get back LIKE '%Adobe%' OR LIKE '%Software%'? You can use: function like_format($str) { return implode(' OR ', array_map(create_function('$a', 'return "LIKE \'%$a%\'";'), explode(' ', $str))); }
-
Addressing Daniel0's suggestions won't be as simple as "change this line, and this line". Essentially you'd need to rewrite the entire code. For example, error handling is a key part of application design, and using die() is a bad habit, so you'd really need to rethink your entire logic. Implementing some form of SoC (like MVC) would be even more drastic, and isn't something you'll master over-night.
-
Your problem is a scope issue. $class2 isn't a property of class1, it's just a variable who is only available in the scope of class1's constructer. You'd need to do it like this: <?php class class1 { public $class2; public function __construct() { $this->class2 = new class2(); } } class class2 { public function test() { echo 'Hello world!'; } } $class1 = new class1(); $class1->class2->test(); ?>